Chapter 6: Class, Identifiers, Reserved Keywords, Data Types in Java
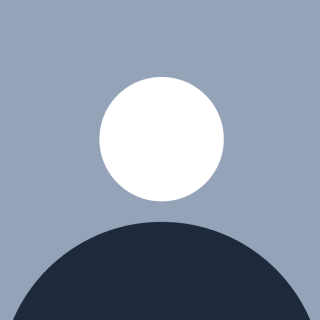
Table of contents
- Today's Topics of Discussion:
- Object-Oriented Programming (OOP) Principles
- Understanding Classes and Objects
- Identifiers in Java
- Examples of Identifiers in Java
- Rules for Writing an Identifier in Java (With Respect to JVM + Compiler)
- Rule 1: Allowed Characters in Java Identifiers
- Rule 2: Using Any Other Characters Results in a Compile-Time Error
- Rule 3: Identifiers Cannot Start with a Digit
- Rule 4: Identifiers Are Case-Sensitive
- Rule 5: No Length Limit for Identifiers
- Special Cases: Identifiers Can Start with $ or _
- 2.2 Reserved Words in Java
- Data Types in Java
- Data Types Overview
- Compiler Role
- Primitive Data Types in Java
- Tree Diagram for Primitive Data Types
- Data Type Info
- Reserved Words for Data Types (8):
- 1. byte Data Type:
- 2. short Data Type:
- 3. int Data Type:
- 4. long Data Type:
- Example Scenario: Byte Arithmetic and Type Conversion
- Conclusion
- Further Reading and Resources
- Related Posts in My Series:
Today's Topics of Discussion:
Basic Introduction to OOP (Object-Oriented Programming)
Identifiers and Variables
Reserved Words in Java
Data Types and Data Type Chart
Object-Oriented Programming (OOP) Principles
Object-Oriented Programming (OOP) is a programming paradigm centered around the concept of "objects." It enables developers to model real-world entities in code. Every object in OOP consists of two main components:
What it has (Attributes/Properties)
What it does (Methods/Behaviors)
Example: Car
Let's consider the example of a car:
What it has:
Brand Name: The manufacturer of the car (e.g., Toyota).
Number of Wheels: Most cars have four wheels.
Model: The specific version of the car (e.g., Camry).
Speed: The maximum speed the car can achieve.
What it does:
Move: The ability to travel from one place to another.
Accelerate: The ability to increase speed.
Brake: The ability to slow down or stop.
Java Code Representation
To express these concepts in Java, we use classes. A class is a blueprint for creating objects. Here’s how we can represent the car in Java:
class Car {
// Attributes (Has part of an object)
String brandName; // Brand of the car
int noOfWheels; // Number of wheels
// Methods (Does part of an object)
public void move() {
// Logic for moving the vehicle
System.out.println("The car is moving.");
}
public void accelerate() {
// Logic for accelerating the vehicle
System.out.println("The car is accelerating.");
}
}
In this code:
Attributes like
brandName
andnoOfWheels
represent what the car has.Methods like
move()
andaccelerate()
represent what the car does.
Understanding Classes and Objects
Class: A class is a template from which objects are created. It defines the properties and behaviors common to all objects of that type. For instance,
Car
is a class that defines what attributes and methods every car object will have.Object: An object is an instance of a class. Each object can have its own unique values for the properties defined in the class. For example, a specific car like a "Toyota Camry" is an object of the
Car
class.
Example: Student Class
Let’s consider a Student
class to illustrate this further:
class Student {
// Attributes (Has part)
String name; // Name of the student
int id; // Student ID
float height; // Height of the student
// Methods (Does part)
public void study() {
// Logic for studying
System.out.println(name + " is studying.");
}
public void play() {
// Logic for playing
System.out.println(name + " is playing.");
}
}
In this example, each student has a name, ID, and height, and can perform actions like studying and playing.
Identifiers in Java
Identifiers are names given to various elements in Java code, such as classes, methods, and variables. They help distinguish different parts of the code.
Types of Identifiers
Class Name: The name of a class (e.g.,
Car
,Student
).Method Name: The name of a method (e.g.,
move()
,study()
).Variable Name: The name of a variable (e.g.,
brandName
,id
).
Rules for Identifiers
Must begin with a letter, underscore (
_
), or dollar sign ($
).Can contain letters, digits, underscores, or dollar signs.
Cannot start with a digit.
Case-sensitive (e.g.,
myVar
andmyvar
are different).Cannot use reserved keywords (like
class
,public
,void
).(You will find a detailed explanation below)
Examples of Identifiers in Java
Let’s go through the provided examples and identify the identifiers in each case.
Example 1:
class Test {
public static void main(String[] args) {
int x = 10;
}
}
Identifiers:
Test
: Class name.main
: Method name.String
: Class name (for theargs
parameter).args
: Parameter variable.x
: Local variable.
Total identifiers: 5
Example 2:
class Test {
public static void main(String[] args) {
System.out.println("Rohit");
}
}
Identifiers:
Test
: Class name.main
: Method name.String
: Class name (forargs
).args
: Parameter variable.System
: Class name.out
: Member of theSystem
class.println
: Method fromPrintStream
.
Total identifiers: 7
Example 3:
class Demo {
public static void main(String[] args) {
String name = "Rohit";
String result = name.toUpperCase();
System.out.println(result);
}
}
Identifiers:
Demo
: Class name.main
: Method name.String
: Class name (appears twice).args
: Parameter variable.name
: Local variable.result
: Local variable.toUpperCase
: Method name.System
: Class name.out
: Member of theSystem
class.println
: Method fromPrintStream
.
Total identifiers: 12 (including repeated identifiers)
Rules for Writing an Identifier in Java (With Respect to JVM + Compiler)
Identifiers are fundamental elements in Java that represent names of variables, methods, classes, etc. These identifiers must follow specific rules to be valid in Java. The Java Virtual Machine (JVM) and the compiler enforce these rules strictly, and any violation results in a compilation error.
Rule 1: Allowed Characters in Java Identifiers
Java identifiers can only consist of the following characters:
Lowercase letters:
a
toz
Uppercase letters:
A
toZ
Digits:
0
to9
Underscore (
_
)Dollar sign (
$
)
Other symbols or special characters are not allowed in identifiers. The compiler throws an error if you use them.
Example:
javaCopy codeint ^ = 10; // Invalid (cannot use `^` in an identifier)
int toata;l = 10; // Invalid (semicolon is not allowed in an identifier)
int total# = 10; // Invalid (hash `#` is not allowed)
int rohit10 = 100; // Valid (contains only valid characters)
int ^ = 10;
→ Invalid: The^
symbol is not allowed.int toata;l = 10;
→ Invalid: The semicolon;
is an invalid character.int total# = 10;
→ Invalid: The hash symbol#
is not allowed.int rohit10 = 100;
→ Valid: The identifier contains letters and digits only.
Rule 2: Using Any Other Characters Results in a Compile-Time Error
As explained, using any characters other than letters (a-z
, A-Z
), digits (0-9
), underscores (_
), or dollar signs ($
) in identifiers results in a compile-time error.
Example:
javaCopy codeint my&name = 10; // Invalid (the `&` symbol is not allowed)
int your@name = 10; // Invalid (the `@` symbol is not allowed)
The above lines of code will throw compile-time errors.
Rule 3: Identifiers Cannot Start with a Digit
Identifiers cannot start with a digit. They must begin with either a letter (a-z
, A-Z
), an underscore (_
), or a dollar sign ($
). Starting an identifier with a number will result in a compile-time error.
Example:
javaCopy codeint rohit20 = 100; // Valid (starts with a letter)
int 1rohit = 100; // Invalid (cannot start with a digit)
int rohit20 = 100;
→ Valid: Starts with a letter (r
).int 1rohit = 100;
→ Invalid: The identifier starts with a digit (1
).
Rule 4: Identifiers Are Case-Sensitive
Java is a case-sensitive language, meaning num
, Num
, nUm
, and NUM
are treated as different identifiers. The JVM and compiler treat these as entirely separate variables, even though the only difference is the capitalization of letters.
Example:
class Demo {
int num = 10; // Valid
int Num = 20; // Valid (different from `num`)
int nUm = 30; // Valid (different from `num` and `Num`)
int NUM = 40; // Valid (different from `num`, `Num`, and `nUm`)
}
In this case:
num
,Num
,nUm
, andNUM
are all different variables because Java treats uppercase and lowercase letters as distinct.
Rule 5: No Length Limit for Identifiers
There is no fixed length limit for Java identifiers. Technically, you can make an identifier as long as you want. However, it's a good practice to keep the identifier length below 15 characters to maintain readability and clarity in your code.
Example:
int PriorityofThreadWithMinValue = 1; // Valid (though long)
Even though the identifier PriorityofThreadWithMinValue
is long, it is still valid. However, using long identifiers is not recommended as it makes the code harder to read.
Special Cases: Identifiers Can Start with $
or _
Identifiers can also start with a dollar sign ($
) or an underscore (_
). This is allowed, but it is generally not recommended in practice unless necessary.
Example:
codeint $a = 10; // Valid (starts with a dollar sign)
int _a = 10; // Valid (starts with an underscore)
Both identifiers are perfectly valid according to Java rules, but most developers avoid starting identifiers with $
or _
unless there's a specific need (like for system-generated names).
2.2 Reserved Words in Java
Understanding Reserved Words
Reserved words, also known as keywords, are predefined identifiers in the Java programming language that have specific meanings and functionalities associated with them. The Java compiler and the Java Virtual Machine (JVM) recognize these words and use them to define the structure of the Java language.
Characteristics of Reserved Words:
Predefined Meaning: Each reserved word is tied to a particular action or concept in Java (e.g., control flow, data types, etc.).
Cannot be Used as Identifiers: Since they have special meanings, reserved words cannot be used as names for variables, classes, or methods. Attempting to do so results in a compile-time error.
Case Sensitivity: Java is case-sensitive, which means that identifiers with different cases are treated as different entities.
Examples of Reserved Words
Control Flow Keywords:
if
,else
,while
,for
,switch
: These keywords are used to control the flow of the program based on conditions. For instance,if
checks a condition, andelse
provides an alternative execution path.
Exception Handling Keywords:
try
,catch
,throw
,throws
: These keywords are used to handle exceptions in Java, enabling developers to write robust code that can manage runtime errors gracefully.
Data Type Keywords:
int
,float
,double
,char
: These keywords define the data types of variables. For example,int
is used for integer values, whilefloat
is for floating-point numbers.
Modifiers:
public
,private
,protected
,static
: These keywords modify the accessibility and behavior of classes, methods, and variables. For example,public
allows access from any other class, whileprivate
restricts access to the defining class.
Reserved Literals:
true
,false
,null
: These literals represent boolean values and null references. They are essential for conditional expressions and object initialization.
Rules for Writing Identifiers
Rule 6: Reserved Words Cannot Be Used as Identifiers
Since reserved words have specific meanings, you cannot use them as variable names or identifiers. This rule is critical to avoid conflicts with the Java language syntax.
Example:
int if = 10; // Compile-time error: 'if' is a reserved word.
Rule 7: Predefined Class Names Can Be Used as Identifiers
While it is technically possible to use predefined class names (e.g.,
String
,Runnable
) as identifiers, doing so can lead to confusion and is considered poor practice. It's better to choose descriptive and meaningful names for variables.Examples:
String myString = "Hello"; // 'String' is an inbuilt class name, but valid as an identifier. Runnable myRunnable = new Runnable() { /* implementation */ }; // Using 'Runnable' as an identifier.
Case Sensitivity
Java identifiers are case-sensitive, meaning that
int int = 10;
is valid, but using the same word with different cases, such asint Int = 10;
, would cause a compile-time error.Example:
int intVar = 10; // Valid int IntVar = 20; // Valid (different identifier) int INT = 30; // Valid (different identifier)
Reserved Words Count and Structure
Java has a total of 53 reserved words that can be categorized as follows:
Keywords (50):
Used Keywords (49): These are actively used in programming.
Unused Keywords (2):
goto
andconst
are reserved but not used in the Java language.
Reserved Literals (3):
true
,false
,null
: These literals are integral to the language's boolean data type.
Structure of Reserved Words
Here's a visual representation of the structure of reserved words in Java:
+-------------------------------------+
| Reserved Words |
+-------------------------------------+
|
+------------------+------------------+
| |
+-------------+ +--------------+
| Keywords | | Reserved |
| (50) | | Literals (3) |
+-------------+ +--------------+
| |
| |
+--------------------+ +-----------+
| Used Keywords | | true |
| (49) | | false |
+--------------------+ | null |
| |
| |
| |
+---------------------+ +---------------------+
| Control Flow | | Data Types |
| (if, else, for, | | (int, float, |
| while, switch) | | double, char) |
+---------------------+ +---------------------+
| Exception Handling | | Modifiers |
| (try, catch, throw, | | (public, private, |
| throws) | | protected, static) |
+---------------------+ +---------------------+
| Others | | Others |
| (class, interface, | | (import, package) |
| extends, implements) | | |
+---------------------+ +---------------------+
Literals in Java
A literal is a constant value that can be directly assigned to a variable. It represents a fixed value that does not change during the execution of a program.
Types of Literals:
Integer Literals: Represent whole numbers, e.g.,
int data = 10;
Floating-Point Literals: Represent decimal numbers, e.g.,
float price = 19.99;
Character Literals: Represent single characters enclosed in single quotes, e.g.,
char initial = 'A';
String Literals: Represent sequences of characters enclosed in double quotes, e.g.,
String name = "John";
Boolean Literals: Represent truth values, either
true
orfalse
.
Reserved Literals
Java has three reserved literals:
true
: Represents the logical value true.false
: Represents the logical value false.null
: Represents a null reference, indicating that a variable does not point to any object.
Examples of Reserved Literals
Boolean Example:
boolean result = 2; // Compile-time error, as 2 is not a valid boolean value. boolean flag = false; // Valid assignment. boolean examResult = True; // Compile-time error; 'True' is not recognized. boolean examResult = true; // Valid assignment.
Comparison with C/C++:
In C and C++,
0
representsfalse
and1
representstrue
.In Java,
0
means zero, and1
means one. Only the boolean literalstrue
andfalse
are valid for boolean variables.
Note: For the boolean data type, the only allowed values for a variable are true
or false
. Any attempt to assign other values will result in a compile-time error.
Analysis of Reserved Words/Keywords/Built-in Words
1. Reserved Words/Keywords Lists
i) final, finalize, finally
final
: This is a reserved keyword in Java used to declare constants, prevent method overriding, and prevent inheritance of classes.finalize
: This is not a reserved keyword. It is a method in theObject
class that can be overridden to perform cleanup operations before an object is garbage collected.finally
: This is a reserved keyword used in exception handling to define a block of code that will execute after atry
block, regardless of whether an exception was thrown.
Result: This list contains both reserved words and a method name (finalize
).
ii) break, continue, return, exit
break
: A reserved keyword used to exit a loop or switch statement.continue
: A reserved keyword that skips the current iteration of a loop and proceeds to the next iteration.return
: A reserved keyword used to exit from a method and optionally return a value.exit
: This is not a reserved keyword in Java. It is often associated with theSystem.exit()
method, which terminates the JVM but is not a keyword.
Result: This list contains mostly reserved words, with exit
being a method rather than a keyword.
iii) byte, short, integer, long
byte
: A reserved keyword in Java representing a primitive data type that can hold an 8-bit signed integer.short
: A reserved keyword representing a primitive data type that can hold a 16-bit signed integer.integer
: This is not a reserved keyword. The correct keyword for a 32-bit signed integer isint
.long
: A reserved keyword representing a primitive data type that can hold a 64-bit signed integer.
Result: This list contains some reserved words, but also includes integer
, which is not a keyword.
iv) throw, throws, thrown
throw
: A reserved keyword used to explicitly throw an exception.throws
: A reserved keyword used in method declarations to specify that a method can throw one or more exceptions.thrown
: This is not a reserved keyword in Java; rather, it is often used in the context of exceptions but is not an official keyword.
Result: This list contains mostly reserved words, with thrown
not being a keyword.
Data Types in Java
Data Types Overview
Every variable and expression in Java has a type. Java is a strictly typed and statically typed language, meaning that all types must be explicitly declared at compile time. This helps the compiler check whether the value assigned to a variable is compatible with its data type.
Compiler Role
The Java compiler performs type checking to ensure that the values stored in variables are compatible with their declared types. For example:
int data = 10; // Valid
Boolean result = true; // Valid
In this case, the compiler checks whether the integer 10
can be stored in the variable data
and whether the boolean value true
is valid for the result
variable.
Primitive Data Types in Java
Primitive data types are the most basic data types supported by the language, allowing the storage of simple values. There are eight primitive data types in Java:
Numeric Types:
Integral Types (whole numbers):
byte
: 8 bitsshort
: 16 bitsint
: 32 bitslong
: 64 bits
Floating Point Types (real numbers):
float
: 32 bitsdouble
: 64 bits
Character Type:
char
: 16 bits (stores a single 16-bit Unicode character)
Boolean Type:
boolean
: Represents one of two values:true
orfalse
.
Tree Diagram for Primitive Data Types
Here’s a representation of the primitive data types in Java:
Primitive Data Types
|
+----------------+----------------+
| |
Numeric Types Non-Numeric Types
| |
+-----+-----+ +---+-----+
| | | |
Integral Types Floating Point Types Character Boolean
| | | |
+--+---+ +-------+-------+ +-----+ +-----+
| | | | | | | |
byte short float double
Data Type Info
Size of Data Types: Specifies how much memory is allocated in RAM for each data type by the Java Virtual Machine (JVM).
Range of Data Types: Indicates the minimum and maximum values that can be stored in each data type.
Example: Byte Data Type
Size: 8 bits
Range:
Minimum value: -128
Maximum value: 127
Java Command: You can check the information about the byte
data type using the command:
javap java.lang.Byte
Examples:
byte marks = 35; // Valid
byte mark = 135; // Compile-time error: possible loss of precision (found int type)
The compiler will check whether the value being assigned to the variable is within the allowable range for that data type. This strict type checking ensures that Java maintains type safety, preventing many types of runtime errors.
Reserved Words for Data Types (8):
byte
short
int
long
float
double
char
boolean
These reserved words are predefined in Java and represent primitive data types. Here's an explanation and some examples for each:
1. byte
Data Type:
Size: 1 byte (8 bits)
Min Value: -128
Max Value: 127
Use Case: Commonly used to handle data from network streams or when you need to save memory in large arrays.
Examples:
byte a = true;
→ Invalid (Compile Time Error: Incompatible types)byte a = 10;
→ Validbyte a = "Rohit";
→ Invalid (Compile Time Error: Incompatible types)
When to use: Use the byte
data type when dealing with stream data or if you want to minimize memory usage.
Example code to know size, min, and max value:
System.out.println("Size of Byte: " + Byte.SIZE); // Output: Size of Byte: 8
System.out.println("Min Value of Byte: " + Byte.MIN_VALUE); // Output: Min Value of Byte: -128
System.out.println("Max Value of Byte: " + Byte.MAX_VALUE); // Output: Max Value of Byte: 127
2. short
Data Type:
Size: 2 bytes (16 bits)
Min Value: -32,768
Max Value: 32,767
Examples:
Short data = 137;
→ ValidShort data = true;
→ Invalid (Compile Time Error: Incompatible types)Short data = "Rohit";
→ Invalid (Compile Time Error: Incompatible types)
System.out.println("Size of Short: " + Short.SIZE); // Output: Size of Short: 16
System.out.println("Min Value of Short: " + Short.MIN_VALUE); // Output: Min Value of Short: -32768
System.out.println("Max Value of Short: " + Short.MAX_VALUE); // Output: Max Value of Short: 32767
- Note: The
short
data type is rarely used in modern Java applications and is typically more useful for memory-constrained environments (e.g., legacy systems).
3. int
Data Type:
Size: 4 bytes (32 bits)
Min Value: -2,147,483,648
Max Value: 2,147,483,647
Use Case: The most commonly used data type for storing whole numbers.
Examples:
int data = 234567;
→ Validint result = true;
→ Invalid (Compile Time Error: Incompatible types)int result = "pass";
→ Invalid (Compile Time Error: Incompatible types)int data = 234567; // Valid // int result = true; // Invalid (Compile Time Error: Incompatible types) // int result = "pass"; // Invalid (Compile Time Error: Incompatible types) System.out.println("Size of Int: " + Integer.SIZE); // Output: Size of Int: 32 System.out.println("Min Value of Int: " + Integer.MIN_VALUE); // Output: Min Value of Int: -2147483648 System.out.println("Max Value of Int: " + Integer.MAX_VALUE); // Output: Max Value of Int: 2147483647
Note: By default, any whole number literal in Java is considered of int
type unless specified otherwise.
4. long
Data Type:
Size: 8 bytes (64 bits)
Min Value: -9,223,372,036,854,775,808
Max Value: 9,223,372,036,854,775,807
Use Case: Useful for storing large numeric values, especially when working with large files or data sets that exceed the range of
int
.Examples:
long data = 35587948683085L;
→ Validlong data = 23456789876543l;
→ Validlong data = 155;
→ Valid (Still treated as anint
unless suffixed withL
)
System.out.println("Size of Long: " + Long.SIZE); // Output: Size of Long: 64
System.out.println("Min Value of Long: " + Long.MIN_VALUE); // Output: Min Value of Long: -9223372036854775808
System.out.println("Max Value of Long: " + Long.MAX_VALUE); // Output: Max Value of Long: 9223372036854775807
- Note: If the number exceeds the range of
int
, you must suffix the number withL
orl
to indicate it aslong
.
Example Scenario: Byte Arithmetic and Type Conversion
Case 1: Arithmetic with Byte Data Type
byte a = 10;
byte b = 5;
byte result = a * b; // Compile Time Error: Possible lossy conversion from int to byte
System.out.println(result);
Explanation: In Java, arithmetic operations between byte
values result in an int
by default, which may cause a loss of precision. Hence, casting is required.
Case 2: Byte Addition Without Result Assignment
byte a = 10;
byte b = 5;
System.out.println(a + b); // No compile-time error, prints the result of a + b as an int
Explanation: Directly printing the result of a + b
does not cause an error because the result is treated as int
and no explicit cast is needed.
Conclusion
In this chapter, we've explored the foundational concepts that are essential for anyone starting their journey in Java programming. We began with Object-Oriented Principles, understanding how real-world objects can be represented in code through classes. By recognizing that every object has attributes (what it has) and behaviors (what it does), we set the stage for building our own Java classes.
We then delved into Reserved Keywords, identifying their crucial role in the Java programming language. Understanding that these keywords cannot be used as identifiers helps avoid common pitfalls for beginners. It’s vital to grasp these terms, as they form the backbone of the syntax and structure you will encounter in Java coding.
Finally, we looked at Data Types, distinguishing between primitive and reference types. Knowing how to choose the correct data type is essential for effective memory management and optimal program performance. As you continue your learning, remember that the right data type can greatly influence how your programs behave and interact with the system.
As you progress further into Java, keep these fundamental concepts in mind. They will serve as the building blocks for more complex programming techniques and will help you write clean, efficient code. Always remember, understanding the basics is key to mastering any programming language!
Further Reading and Resources
If you found this post insightful, you might be interested in exploring more detailed topics in my ongoing series:
Related Posts in My Series:
Full Stack Java Development Series:
Writing My First Java Program – Learn how to get started with Java, from setting up your environment to writing and executing your first Java program.
Exploring Association in Java – Dive deep into the concepts of Association in Java, including One-to-One, One-to-Many, and Many-to-One relationships with practical examples.
Other Series:
DSA in Java – Master Data Structures and Algorithms in Java with a step-by-step approach, including practice problems and explanations.
Full Stack JavaScript Development – Become proficient in JavaScript with this series covering everything from front-end to back-end development, including frameworks like React and Node.js.
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repositories and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊