Create and Deploy NFTs on Aptos
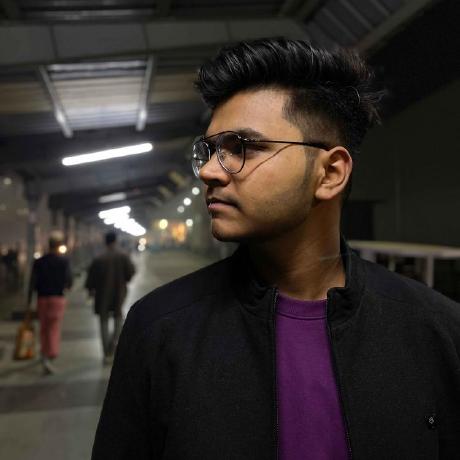

Introduction
Growing in popularity, the Aptos blockchain is a potent Layer-1 network that provides programmers with a distinct setting in which to create decentralized apps with the Move programming language. We'll demonstrate in this blog how to use the Aptos CLI to deploy your first Move module, generate NFTs, and manage tokens. Developers that are new to the Aptos ecosystem and wish to start creating tokens, NFTs, and smart contracts will find this book to be very helpful.
You will have a firm grasp of how to use Move contracts and build on the Aptos blockchain by the time you finish reading this blog.
Getting Started with Aptos and Move Programming
Before diving into deploying contracts and creating NFTs, setting up your development environment is essential. Follow these steps to ensure you’re ready to build on Aptos:
Install Rust: Aptos development requires Rust. Install it using the following command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Install Aptos CLI: Download and set up the Aptos CLI from the official documentation.
Initialize Aptos Account: Create an Aptos account using the CLI:
aptos init
Deploying Your First Move Module Using Aptos CLI
Now that you have the necessary tools, let's deploy a basic Move module on Aptos.
Create Your Move Module
Create a file named
HelloWorld.move
and add the following code:module HelloWorld { public fun greet(): vector<u8> { b"Hello, Aptos!" } }
This module defines a simple function
greet()
that returns a greeting message.Compile the Module
Use the Aptos CLI to compile your module:
aptos move compile --package-dir path/to/your/module
Deploy the Module to the Aptos Network
Deploy your compiled module using the following command:
aptos move publish --package-dir path/to/your/module
Your module is now live on the Aptos network! You can interact with it using the CLI or build a frontend application to call the greet()
function.
Creating Soulbound and Dynamic NFTs on Aptos
Non-fungible tokens (NFTs) are a popular use case on blockchain platforms. In this section, we'll create both SoulBound and dynamic NFTs on the Aptos blockchain using Move.
Soulbound NFTs are unique because they cannot be transferred once assigned to an account.
Define the Soulbound NFT Struct
module SoulboundNFT { resource struct Soulbound { id: u64, owner: address, metadata: vector<u8>, } public fun create_soulbound(account: &signer, id: u64, metadata: vector<u8>) { let nft = Soulbound { id, owner: signer::address_of(account), metadata }; move_to(account, nft); } }
Deploy and Test
Compile and deploy the module using the CLI commands from earlier.
Call the
create_soulbound
function to create your first soulbound NFT.
Creating a Dynamic NFT
Dynamic NFTs have properties that can change over time.
Define the Dynamic NFT Struct
module DynamicNFT { resource struct Dynamic { id: u64, metadata: vector<u8>, mutable_properties: vector<u8>, } public fun create_dynamic(account: &signer, id: u64, metadata: vector<u8>, mutable_properties: vector<u8>) { let nft = Dynamic { id, metadata, mutable_properties }; move_to(account, nft); } public fun update_properties(account: &signer, new_properties: vector<u8>) { let nft = borrow_global_mut<Dynamic>(signer::address_of(account)); nft.mutable_properties = new_properties; } }
Deploy and Test
Compile and deploy the module using the same CLI process.
Create and update your dynamic NFT using the provided functions.
Mutating NFT Properties on Aptos
The ability to mutate NFT properties can be highly useful for various applications, such as games or collectible platforms.
Call the
update_properties
function from theDynamicNFT
module to change the mutable properties of your NFT.This action allows the NFT to evolve, making it ideal for use cases where characteristics need to change over time.
Managing Tokens on Aptos: Creating, Freezing, and Using Multisig Accounts
The Aptos blockchain allows you to create and manage tokens with advanced functionality, such as freezing accounts or using multi-sig wallets.
Freezing Accounts to Prevent Token Transfer
To prevent certain accounts from transferring a token, you can implement a freezing mechanism using Move:
module TokenModule {
resource struct Token {
id: u64,
frozen: bool,
}
public fun freeze_account(account: &signer) {
let token = borrow_global_mut<Token>(signer::address_of(account));
token.frozen = true;
}
public fun is_frozen(account: &address): bool {
borrow_global<Token>(account).frozen
}
}
Use the
freeze_account
function to prevent transfers from the account.The
is_frozen
function can check if an account is frozen.
Using a Multisig Account with Managed Fungible Assets
A multi-sig account requires multiple approvals for certain actions, adding an extra layer of security.
Refer to the official guide on multisig-managed fungible assets for a detailed explanation and implementation example.
Issuing a Stable Coin on Aptos
Aptos allows you to issue stablecoins, which are tokens pegged to a stable asset like the US dollar.
- Refer to the detailed guide on issuing stablecoins provided in the Aptos documentation for step-by-step instructions
Conclusion
Congratulations! You've learned how to deploy your first Move module on Aptos, create both soulbound and dynamic NFTs, and manage tokens with features like freezing and multi-sig accounts. The Aptos blockchain, combined with the Move programming language, offers an exciting and secure environment for building a wide variety of decentralized applications and assets.
As you continue your journey, explore more advanced features and experiment with different modules to become proficient in Move programming. Welcome to the future of Web3 development!
Subscribe to my newsletter
Read articles from Rudransh Singh Tomar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
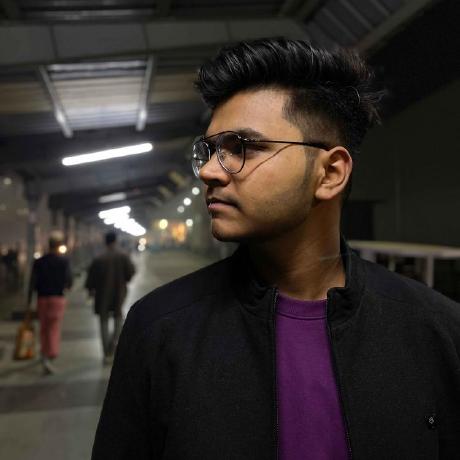