Node.js Interview Questions and Answers: Part 10

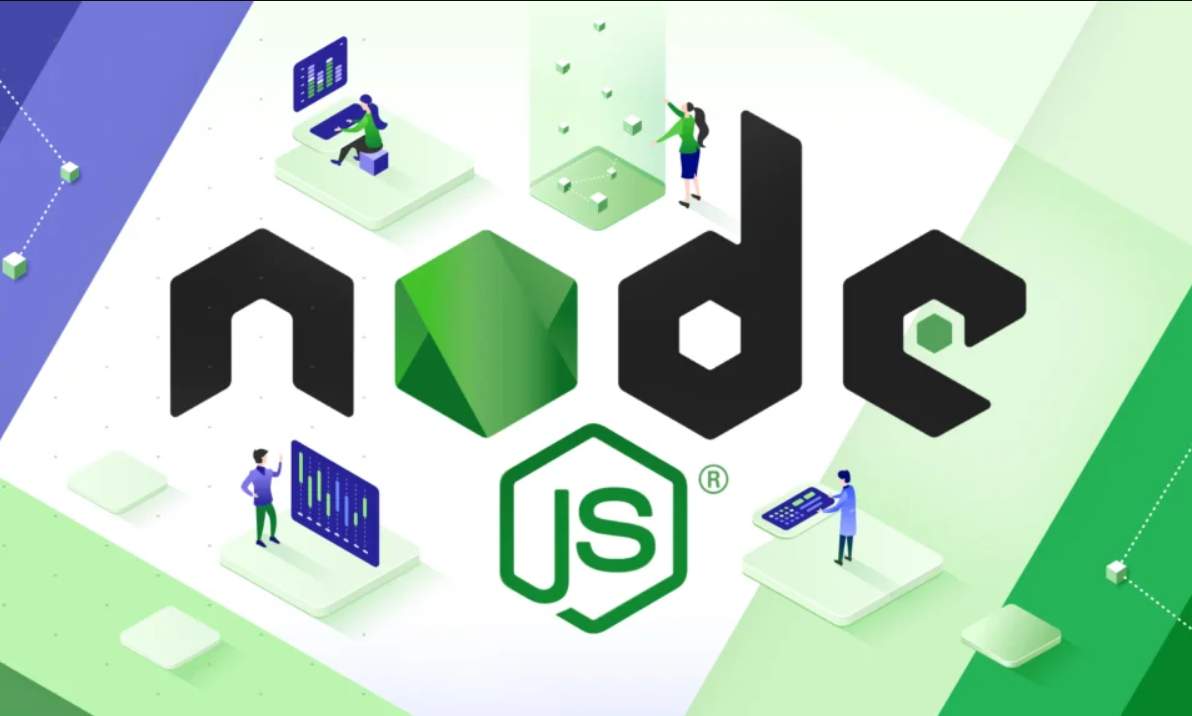
Node.js Basics: An In-depth Interview Guide
In this blog post, we'll explore key concepts of Node.js through an interview-style Q&A session. Each topic will be thoroughly explained with examples, use cases, pros, and cons to help you prepare for your next Node.js interview.
1. Creating a File in Node.js
Interviewer: Can you explain how to create a file in Node.js and why it's important?
Candidate: Certainly! Creating files in Node.js is a fundamental operation that allows us to persist data, generate logs, or create configuration files. Node.js provides the fs
(File System) module to handle file operations.
Here's an example of how to create a file:
const fs = require('fs');
fs.writeFile('example.txt', 'Hello, Node.js!', (err) => {
if (err) throw err;
console.log('File has been created');
});
In this example, we're using the writeFile
method to create a new file named 'example.txt' with the content "Hello, Node.js!".
There are also synchronous versions of these methods, like writeFileSync
:
fs.writeFileSync('example.txt', 'Hello, Node.js!');
console.log('File has been created');
Use cases:
Generating log files
Creating configuration files
Saving user data
Exporting reports
Pros:
Allows data persistence
Enables file-based configurations
Useful for logging and debugging
Cons:
Synchronous operations can block the event loop
File operations can be slower than in-memory operations
Requires proper error handling to manage I/O exceptions
It's important to note that while creating files is powerful, it should be used judiciously, especially in a server environment where file system access might be restricted or have performance implications.
2. Event Modules in Node.js
Interviewer: How do event modules work in Node.js, and why are they important?
Candidate: Event modules are a core part of Node.js's architecture, embodying its event-driven, non-blocking I/O model. The main class for working with events is the EventEmitter
class, which is part of the events
module.
Here's a basic example of how to use EventEmitter
:
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on('event', () => {
console.log('An event occurred!');
});
myEmitter.emit('event');
In this example, we create a custom emitter, attach a listener to the 'event' event, and then emit that event.
Use cases:
Building real-time applications (e.g., chat systems)
Implementing publish-subscribe patterns
Handling asynchronous operations
Creating custom streams
Pros:
Allows for loose coupling between components
Enables asynchronous, event-driven programming
Highly scalable for I/O-bound applications
Cons:
Can lead to "callback hell" if not managed properly
Potential memory leaks if listeners aren't removed
Debugging can be challenging due to asynchronous nature
Event modules are crucial in Node.js because they allow for efficient handling of I/O operations without blocking the main thread, making Node.js particularly well-suited for applications with high concurrency needs.
3. Creating a Port in Node.js
Interviewer: How do you create and use a port in Node.js, and why is it important?
Candidate: In Node.js, we don't exactly "create" a port, but rather we bind our application to a specific port to listen for incoming connections. This is typically done when creating a server. Here's an example using the http
module:
const http = require('http');
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World');
});
server.listen(port, () => {
console.log(`Server running at http://localhost:${port}/`);
});
In this example, we're creating an HTTP server and binding it to port 3000.
Use cases:
Web servers
API endpoints
WebSocket servers
Microservices
Pros:
Allows multiple services to run on the same machine
Enables network communication
Facilitates load balancing and service discovery
Cons:
Port conflicts can occur if not managed properly
Some ports require elevated privileges
Security considerations for exposed ports
Ports are crucial in networking as they allow multiple network services to coexist on a single IP address. In Node.js applications, properly managing ports is essential for creating scalable, networked applications.
4. Creating an HTTP Server in Node.js
Interviewer: Can you explain how to create an HTTP server in Node.js and why it's important?
Candidate: Certainly! Creating an HTTP server is one of the most common tasks in Node.js, especially for web applications and APIs. Node.js provides the http
module to create HTTP servers easily.
Here's a basic example:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World');
});
server.listen(3000, 'localhost', () => {
console.log('Server running at http://localhost:3000/');
});
In this example, we create a server that responds with "Hello World" to all requests. The server listens on port 3000 on the localhost.
Use cases:
Web applications
RESTful APIs
Microservices
Proxy servers
Pros:
Built-in module, no external dependencies required
Lightweight and fast
Highly customizable
Supports HTTPS out of the box
Cons:
Low-level API, might require additional frameworks for complex applications
Requires manual handling of routes and HTTP methods
No built-in middleware support
Creating HTTP servers in Node.js is fundamental because it allows you to build web applications and services. It's the foundation upon which many higher-level frameworks like Express.js are built.
5. HTTP Methods in Node.js
Interviewer: How do you handle different HTTP methods in a Node.js server?
Candidate: Handling different HTTP methods in Node.js involves examining the req.method
property of the incoming request and responding accordingly. Here's an example that demonstrates handling GET and POST methods:
const http = require('http');
const server = http.createServer((req, res) => {
if (req.method === 'GET') {
if (req.url === '/') {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello World');
} else if (req.url === '/api') {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'This is the API endpoint' }));
}
} else if (req.method === 'POST') {
if (req.url === '/api') {
let body = '';
req.on('data', chunk => {
body += chunk.toString();
});
req.on('end', () => {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'Data received', data: body }));
});
}
} else {
res.writeHead(405, { 'Content-Type': 'text/plain' });
res.end('Method Not Allowed');
}
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
Use cases:
RESTful API design
CRUD operations
File uploads (POST/PUT)
Authentication (POST)
Pros:
Allows for creating fully-fledged web applications and APIs
Enables RESTful design principles
Provides flexibility in handling different types of requests
Cons:
Can become complex for large applications
Requires manual routing and method checking
Error-prone without proper structure
Handling different HTTP methods is crucial for building robust web applications and APIs. It allows your server to perform different actions based on the type of request, adhering to RESTful principles and providing a clear interface for clients to interact with your server.
6. Dynamic Routing in Node.js
Interviewer: Can you explain how dynamic routing works in Node.js and provide an example?
Candidate: Certainly! Dynamic routing in Node.js allows you to handle routes with variable parameters. Instead of defining a separate route for each possible value, you can create a route pattern that matches multiple URLs.
Here's an example using the url
module to implement basic dynamic routing:
const http = require('http');
const url = require('url');
const server = http.createServer((req, res) => {
const parsedUrl = url.parse(req.url, true);
const path = parsedUrl.pathname;
const trimmedPath = path.replace(/^\/+|\/+$/g, '');
if (trimmedPath.startsWith('users/')) {
const userId = trimmedPath.split('/')[1];
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: `User details for ID: ${userId}` }));
} else if (trimmedPath === 'products') {
const query = parsedUrl.query;
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'Product list', filter: query }));
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('404 Not Found');
}
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, /users/:userId
is a dynamic route that can handle requests like /users/123
, /users/abc
, etc. The /products
route can handle query parameters for filtering.
Use cases:
RESTful API design (e.g.,
/users/:id
,/posts/:postId/comments
)Handling pagination (e.g.,
/products?page=2&limit=10
)Multilingual routes (e.g.,
/:lang/about
)Version control in APIs (e.g.,
/api/v1/users
)
Pros:
Allows for more flexible and scalable route definitions
Reduces code duplication
Enables creation of cleaner, more intuitive URLs
Cons:
Can become complex with deeply nested routes
Requires careful handling to prevent security issues (e.g., path traversal attacks)
May require additional validation of route parameters
Dynamic routing is essential for building flexible and scalable web applications. It allows you to create more intuitive URLs and handle a wide range of requests with less code. However, it's often easier to implement and manage dynamic routing using a framework like Express.js, which provides a more robust routing system out of the box.
7. Channel Route in Node.js
Interviewer: Can you explain what a channel route is in Node.js and how it might be implemented?
Candidate: I apologize, but there seems to be a misunderstanding here. The term "channel route" is not a standard or commonly used concept in Node.js. It's possible you might be referring to one of these concepts:
WebSocket channels: These are used for real-time, bidirectional communication between clients and servers. While not strictly a "route", they can be thought of as communication channels.
Event channels: In event-driven programming, you might have different channels for different types of events.
Routing in message queues: Some message queue systems use the concept of channels for routing messages.
Let me provide an example of implementing WebSocket channels, which is closest to the concept of a "channel route":
const WebSocket = require('ws');
const http = require('http');
const server = http.createServer();
const wss = new WebSocket.Server({ server });
const channels = new Map();
wss.on('connection', (ws) => {
ws.on('message', (message) => {
const data = JSON.parse(message);
if (data.type === 'join') {
if (!channels.has(data.channel)) {
channels.set(data.channel, new Set());
}
channels.get(data.channel).add(ws);
ws.channel = data.channel;
} else if (data.type === 'message') {
if (channels.has(ws.channel)) {
channels.get(ws.channel).forEach((client) => {
if (client !== ws && client.readyState === WebSocket.OPEN) {
client.send(JSON.stringify({ type: 'message', text: data.text }));
}
});
}
}
});
ws.on('close', () => {
if (ws.channel && channels.has(ws.channel)) {
channels.get(ws.channel).delete(ws);
if (channels.get(ws.channel).size === 0) {
channels.delete(ws.channel);
}
}
});
});
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
This example creates a WebSocket server that allows clients to join different channels and send messages to all other clients in the same channel.
Use cases:
Real-time chat applications
Live updates in collaborative tools
Gaming server communication
IoT device communication
Pros:
Enables real-time, bidirectional communication
Allows for efficient broadcasting to specific groups of clients
Reduces server load by only sending updates to relevant clients
Cons:
More complex to implement and maintain than traditional HTTP routes
Requires careful management of connections and channels
May require additional infrastructure for scaling
While this isn't a standard "channel route" in Node.js, it demonstrates how you might implement channel-based communication in a Node.js application. This approach is particularly useful for applications requiring real-time updates or bidirectional communication.
8. CORS in Node.js
Interviewer: Can you explain what CORS is and how to implement it in a Node.js server?
Candidate: Certainly! CORS stands for Cross-Origin Resource Sharing. It's a security feature implemented by web browsers to restrict web pages from making requests to a different domain than the one serving the web page. CORS is crucial for protecting users from potentially malicious cross-origin requests.
In a Node.js server, you can implement CORS by setting appropriate headers in your HTTP responses. Here's a basic example:
const http = require('http');
const server = http.createServer((req, res) => {
// Set CORS headers
res.setHeader('Access-Control-Allow-Origin', '*');
res.setHeader('Access-Control-Allow-Methods', 'GET, POST, OPTIONS, PUT, DELETE');
res.setHeader('Access-Control-Allow-Headers', 'X-Requested-With,content-type');
// Handle preflight requests
if (req.method === 'OPTIONS') {
res.writeHead(204);
res.end();
return;
}
// Your regular route handling goes here
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello World');
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, we're setting CORS headers to allow requests from any origin (*
). In a production environment,
you'd typically want to be more specific about which origins are allowed.
Use cases:
Building APIs consumed by web applications on different domains
Allowing resource sharing between microservices
Implementing third-party integrations
Developing frontend and backend separately
Pros:
Enhances security by controlling which origins can access your resources
Allows for flexible resource sharing policies
Enables separation of frontend and backend concerns
Cons:
Can be complex to configure correctly
Overly permissive CORS policies can introduce security vulnerabilities
Debugging CORS issues can be challenging
Implementing CORS correctly is crucial for building secure web applications that interact with APIs or resources from different origins. It's important to carefully consider your CORS policy to balance security and functionality.
9. Cluster Module in Node.js
Interviewer: Can you explain what the Cluster module is in Node.js and how it's used?
Candidate: Certainly! The Cluster module in Node.js allows you to create child processes (workers) that run simultaneously and share the same server port. It's designed to help you take advantage of multi-core systems, improving the performance and reliability of your Node.js applications.
Here's an example of how to use the Cluster module:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
// Fork workers.
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`worker ${worker.process.pid} died`);
});
} else {
// Workers can share any TCP connection
// In this case it is an HTTP server
http.createServer((req, res) => {
res.writeHead(200);
res.end('hello world\n');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
This script creates a worker for each CPU core. Each worker runs an HTTP server on the same port (8000).
Use cases:
Improving performance of CPU-intensive applications
Increasing reliability by restarting crashed workers
Utilizing multi-core systems effectively
Handling high concurrent loads in web servers
Pros:
Improves application performance on multi-core systems
Enhances reliability through worker redundancy
Allows for zero-downtime restarts and updates
Cons:
Increases complexity of the application
Can lead to increased memory usage
Not suitable for all types of applications (e.g., those with shared state)
The Cluster module is particularly useful for improving the performance and reliability of Node.js web servers and other applications that can benefit from parallel processing.
10. Non-blocking and Blocking Operations in Node.js
Interviewer: Can you explain the difference between non-blocking and blocking operations in Node.js, and provide examples of each?
Candidate: Certainly! Understanding the difference between non-blocking and blocking operations is crucial in Node.js, as it directly impacts the performance and scalability of applications.
Blocking operations execute synchronously and block the execution of any additional JavaScript until the operation completes. They tie up the Node.js event loop and prevent it from handling other requests.
Non-blocking operations execute asynchronously, allowing the execution of additional JavaScript while the operation is running. They don't block the event loop, enabling Node.js to handle multiple operations concurrently.
Here's an example to illustrate both:
const fs = require('fs');
// Blocking (synchronous) operation
console.log('Start reading file synchronously...');
const dataSync = fs.readFileSync('example.txt', 'utf8');
console.log(dataSync);
console.log('Finished reading file synchronously');
// Non-blocking (asynchronous) operation
console.log('Start reading file asynchronously...');
fs.readFile('example.txt', 'utf8', (err, dataAsync) => {
if (err) throw err;
console.log(dataAsync);
});
console.log('Finished starting async read');
In the blocking example, readFileSync
will complete before moving to the next line. In the non-blocking example, readFile
will start the operation and immediately move to the next line, with the callback function executing when the operation completes.
Use cases for non-blocking operations:
I/O operations (file system, database queries)
Network requests
Cryptographic operations
Any potentially long-running operation
Pros of non-blocking operations:
Improved performance and scalability
Better utilization of system resources
Ability to handle many concurrent operations
Cons of non-blocking operations:
Can lead to callback hell if not managed properly
More complex error handling
Debugging can be more challenging
Non-blocking operations are a cornerstone of Node.js's efficiency, allowing it to handle many concurrent connections with a single thread. However, it's important to use them appropriately and manage asynchronous flow to avoid issues like callback hell.
11. Use of libuv in Node.js
Interviewer: Can you explain what libuv is and its role in Node.js?
Candidate: Certainly! libuv is a multi-platform support library that provides asynchronous I/O operations, including file system operations, networking, and concurrency. It's a critical component of Node.js, serving as the abstraction layer between Node.js and the operating system.
Key features and roles of libuv in Node.js include:
Event Loop: libuv implements the event loop, which is central to Node.js's non-blocking I/O model.
Thread Pool: It provides a thread pool for offloading work for some types of asynchronous I/O operations.
File System Operations: libuv handles file I/O operations asynchronously.
Networking: It provides asynchronous TCP and UDP sockets.
Child Processes: libuv allows for the creation and management of child processes.
Cross-platform Abstraction: It provides a consistent interface across different operating systems.
Here's a simplified example of how Node.js might use libuv for file I/O:
const fs = require('fs');
console.log('Starting file read...');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log('File contents:', data);
});
console.log('File read operation initiated');
In this example, when fs.readFile
is called:
Node.js delegates the file read operation to libuv.
libuv adds the operation to its thread pool.
Node.js continues executing the next line of JavaScript.
When the file read is complete, libuv triggers the callback, which Node.js then executes.
Use cases:
Handling file system operations
Network programming
Inter-process communication
Timers and event scheduling
Pros:
Enables efficient, non-blocking I/O operations
Provides cross-platform compatibility
Allows Node.js to handle many concurrent operations with a single thread
Cons:
Complexity in understanding and debugging the event loop
Potential for race conditions if not used carefully
Learning curve for developers new to asynchronous programming
Understanding libuv is crucial for Node.js developers, especially when dealing with performance optimization and understanding the internals of how Node.js handles asynchronous operations.
12. Fork in Node.js
Interviewer: Can you explain what forking is in Node.js and provide an example of how it's used?
Candidate: Certainly! In Node.js, forking refers to the process of creating a new Node.js process (child process) that runs concurrently with the parent process. This is achieved using the child_process
module, specifically the fork()
method.
Forking is different from creating a new thread because it creates a separate V8 instance with its own memory and event loop. This allows for true parallelism, as opposed to the concurrency provided by the event loop within a single process.
Here's an example of how forking works in Node.js:
First, let's create a file called child.js
:
// child.js
process.on('message', (msg) => {
console.log('Message from parent:', msg);
let sum = 0;
for(let i = 0; i < 1e9; i++) {
sum += i;
}
process.send({ result: sum });
});
Now, let's create our main file that will fork this child process:
// parent.js
const { fork } = require('child_process');
console.log('Parent process started');
const child = fork('./child.js');
child.on('message', (msg) => {
console.log('Result from child:', msg.result);
child.kill();
});
child.send({ hello: 'world' });
console.log('Forked child process');
In this example, the parent process forks a child process, sends it a message, and then waits for a response. The child process performs a CPU-intensive calculation and sends the result back to the parent.
Use cases:
Distributing CPU-intensive tasks across multiple cores
Isolating unstable or experimental code
Running multiple instances of an application for redundancy
Implementing worker pools for task distribution
Pros:
Allows for true parallelism, utilizing multiple CPU cores
Provides isolation between processes, improving stability
Enables more efficient handling of CPU-bound tasks
Cons:
Higher memory overhead compared to a single process
Inter-process communication can be more complex
Not suitable for fine-grained concurrency (threads might be better in some cases)
Forking is a powerful feature in Node.js that allows developers to take full advantage of multi-core systems and implement more complex, parallel processing architectures. However, it should be used judiciously, as it comes with its own set of challenges and overheads.
This concludes our deep dive into Node.js basics. These topics cover a wide range of fundamental concepts that are crucial for any Node.js developer to understand. Remember, while knowing these concepts is important, practical experience in applying them is equally valuable. Happy coding!
Subscribe to my newsletter
Read articles from Bodheesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bodheesh
Bodheesh
🚀 Backend Developer | Tech Enthusiast | Tech Blogger I’m a passionate backend developer with 3+ years of experience building scalable systems and efficient APIs using the MERN stack. I advocate for clean code, maintainable architectures, and lifelong learning. Through blogs and videos, I simplify tech concepts, share insights on Node.js, system design, and interview prep, and inspire others to excel in software development. Let’s connect and build something impactful together!