Understanding Python Data Types: Day 9 of Your Learning Journey

Table of contents
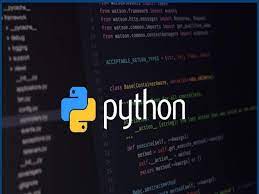
Introduction:
Welcome to Day 9 of my Python journey!
Today, I ventured into the realm of Data Type .
These concepts are essential for efficient data storage.
Data Type :
.Data Type represent the type of data present inside a variable.
. In Python we are not required to specify the type explicitly. Based on value provided,the type will be assigned automatically.Hence Python is Dynamically Typed Language.
Python contains the following inbuilt data types
1.Number
i. int
a. decimal
b. binary
c. octal
d. hex decimal
ii. float
iii.complex
2.bool
3.None
4.str
5.bytes
6.bytearray
7.range
8.list
9.tuple
10.set
11.frozenset
12.dict
Note :
Syntax to create object in C++
class_name obj_name ;
Syntax to create object in Python:
obj_name = class_name
How to declare data type in general way :
Ex:
x= 10
print (type (x), x ) #int
x= 10.6
print (type (x), x ) #float
x= 5+7j
print (type (x), x ) #complex
x= None
print (type (x), x ) #None
x= True
print (type (x), x ) #bool
x= []
print (type (x), x ) #list
x= ()
print (type (x), x ) #tuple
x= 10,
print (type (x), x ) #tuple
x= {}
print (type (x), x ) #dict
x= {()}
print (type (x), x ) #set
how to declare using class
obj = int ()
print (type (obj), obj)
obj =float ()
print (type (obj), obj)
obj =complex ()
print (type (obj), obj)
obj =bool ()
print (type (obj), obj)
obj =None
print (type (obj), obj)
obj = str ()
print (type (obj), obj)
obj = bytes ()
print (type (obj), obj)
obj = bytearray ()
print (type (obj), obj)
obj = range (10)
print (type (obj), obj)
obj = list ()
print (type (obj), obj)
obj = tuple ()
print (type (obj), obj)
obj = dict ()
print (type (obj), obj)
obj = set ()
print (type (obj), obj)
obj = frozenset ()
print (type (obj), obj)
Int class
We can represent int values in the following ways
1. Decimal form
2. Binary form
3. Octal form
4. Hexa decimal form
1. Decimal form(base-10):
It is the default number system in Python
The allowed digits are: 0 to 9
Example:
a =10
type (a)
<class 'int'>
a
10
2. Binary form(Base-2):
The allowed digits are : 0 & 1
Literal value should be prefixed with 0b or 0B
Eg:
a = 0B1111
type (a)
<class 'int'>
a
15
a =0B123 #Error
a=b111 #Error
3. Octal Form(Base-8):
The allowed digits are : 0 to 7
Literal value should be prefixed with 0o or 0O.
Example:
a=0o123
type (a)
<class 'int'>
a
83
a=0o786 #Error
4. Hexa Decimal Form(Base-16):
The allowed digits are : 0 to 9, a-f (both lower and upper cases are allowed)
Literal value should be prefixed with 0x or 0X
Example:
a =0XFACE
type (a)
<class 'int'>
a
64206
a=0XBeef
type (a)
<class 'int'>
a
48879
a =0XBeer #Error
x = 10
print (type (x), x )
x= 0B10
print (type (x), x )
#x= 0b1012 Error
x= 0o10
print (type (x), x )
#x= 0o1018 Error
x = 0xf
print (type (x), x )
#x= 0x12AG #Error
print (type (x), x )
Challenges:
Understanding data types.
Handling coding errors.
Resources:
Official Python Documentation: Data types.
W3Schools' Python Tutorial: Data types
Scaler's Python Course: Data Types
Goals for Tomorrow:
Explore something more about data types.
Learn about exceptions.
Conclusion:
Day 9 was a success!
Data types are now under my belt.
What are your views on data types ? Share in the comments below.
Connect with me:
LinkedIn: [ https://www.linkedin.com/in/archana-prusty-4aa0b827a/ ]
GitHub: [ https://github.com/p-archana1 ]
Join the conversation:
Share your own learning experiences or ask questions in the comments.
Next Post:
Day 10: basic data types
Happy learning!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.