Linked Lists in DSA
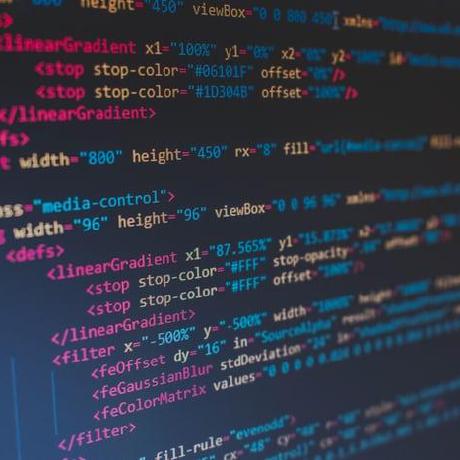
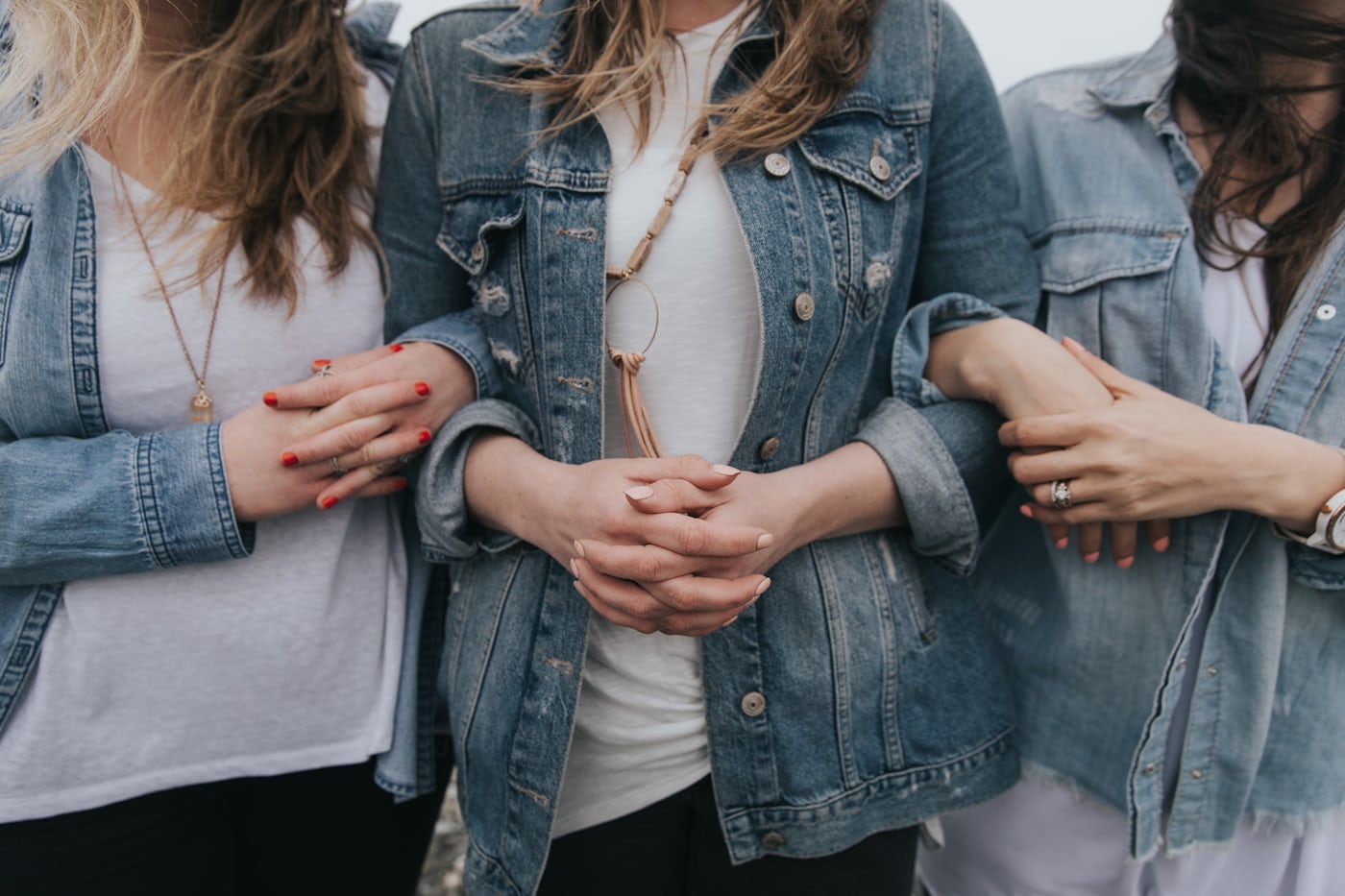
What are Linked Lists?
Linked lists are a fundamental data structure in computer science, characterized by a series of nodes, where each node contains two main components: the data and a reference (or pointer) to the next node in the sequence. This structure allows for dynamic memory allocation, meaning that linked lists can grow and shrink in size as needed, unlike static data structures such as arrays.
To illustrate this concept in a relatable manner, consider a train. Each coach (node) of the train is connected to the next one by a coupling (the pointer). If you want to add or remove a coach, you simply uncouple it from the train without needing to rearrange all the other coaches. This flexibility is one of the key advantages of linked lists.
Types of Linked Lists
There are several types of linked lists, each serving different purposes:
Singly Linked List: In this simplest form, each node contains data and a reference to the next node. The final node points to nothing (or NULL).
It allows traversal in one direction—from the head (first node) to the tail (last node).
Doubly Linked List: Here, each node has two references—one pointing to the next node and another pointing to the previous node. This bidirectional traversal allows for more flexible operations but requires more memory.
Circular Linked List: In this variation, the last node points back to the first node instead of having a null reference. This creates a circular structure that can be useful for applications requiring continuous looping through data.
Operations and Time Complexities
Linked lists support various operations, each with different time complexities:
Insertion:
At the head:
O(1)
– Adding a new node at the beginning is straightforward and efficient. This is because, you can just create a new node and then set the pointer to the head.At the tail or specific position:
O(n)
– Requires traversal to find the correct insertion point.
Deletion:
From the head:
O(1)
– Removing the first node is quick.From other positions:
O(n)
– Similar to insertion, it requires traversal to locate the node.
Traversal:
O(n)
– Accessing elements sequentially involves visiting each node until reaching the desired one.
Uses of Linked Lists
Linked lists are versatile and find applications in various domains:
Implementing Stacks and Queues: Their dynamic nature makes them ideal for these abstract data types, allowing for efficient push/pop or enqueue/dequeue operations.
Maintaining Playlists in Music Applications: Users can easily add or remove songs without disrupting the entire list.
Managing Browser History: Browsers often use linked lists to keep track of visited pages, enabling easy back-and-forth navigation.
Advantages Over Arrays
Linked lists offer several advantages compared to arrays:
Dynamic Size: They can easily grow or shrink as elements are added or removed, making them more adaptable than arrays with fixed sizes.
Efficient Insertions/Deletions: Unlike arrays that require shifting elements when modifying their contents, linked lists allow for quick insertions and deletions without such overhead.
No Memory Waste: Since they allocate memory as needed, linked lists can be more memory-efficient in certain scenarios.
Disadvantages
Despite their advantages, linked lists come with some drawbacks:
Memory Overhead: Each node requires additional memory for storing pointers, which can lead to increased memory usage compared to arrays that only store data. However, remember that in Arrays, we could have a lot of unused allocated memory.
Sequential Access: Unlike arrays that allow direct access to any element via indexing, linked lists require traversal from the head to reach specific nodes. This can result in slower access times for large datasets.
Conclusion
In summary, linked lists are a flexible and efficient way to manage dynamic data, making them great for adding and removing items quickly. Even though they have some downsides, like using more memory (although can be more optimized when used properly) and being slower to access, they are really important for anyone learning to code.
Subscribe to my newsletter
Read articles from Kingsley Ihemelandu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
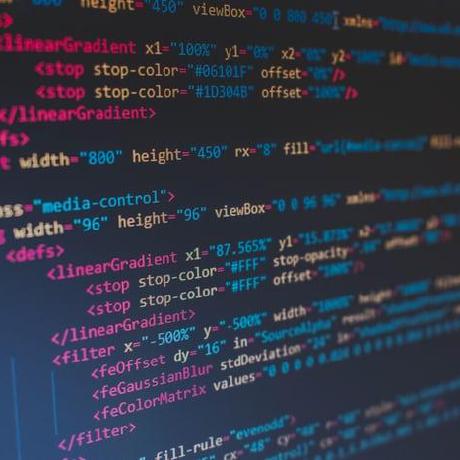