Problem Statement: Creating a Car Class in Java to Understand Object-Oriented Programming (OOP)

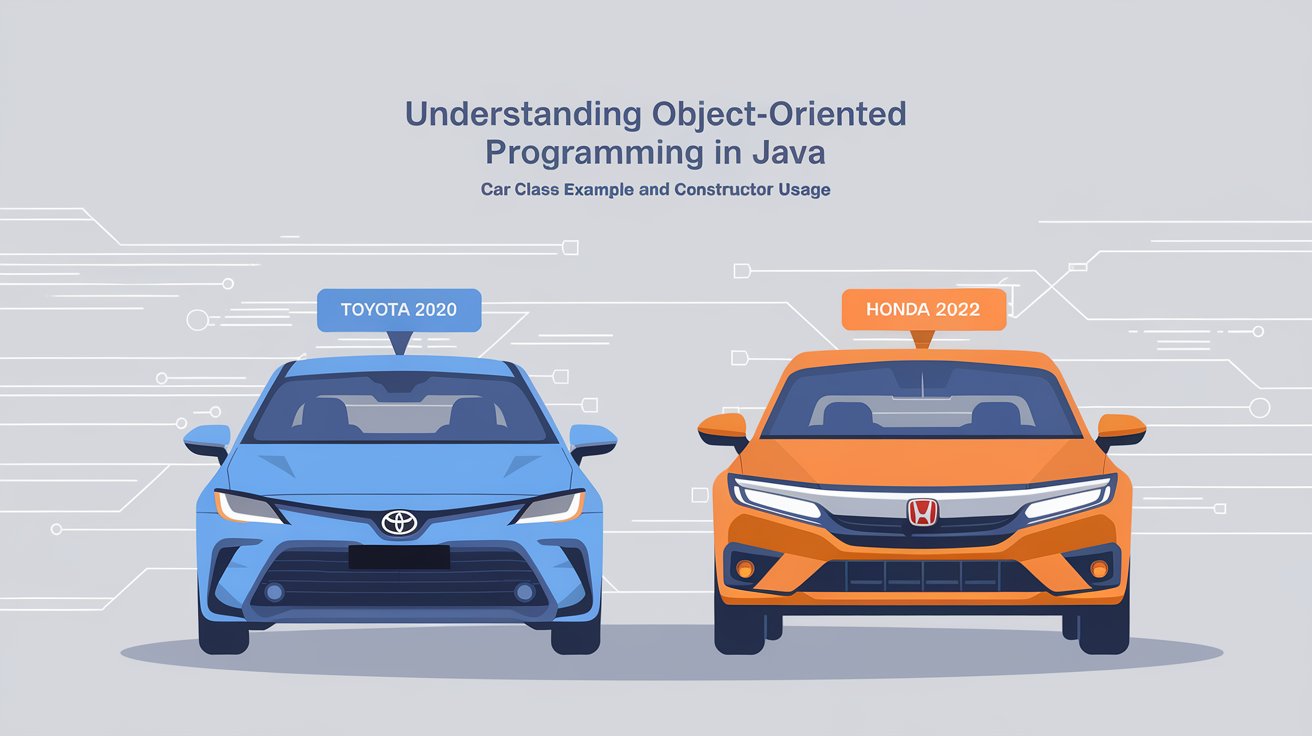
New Problem Statement
Question:
Create a class named
Car
with two attributes:brand
andyear
.Write a constructor that initializes these attributes using the
this
keyword.Create a method called
showDetails()
to print the car's brand and year of manufacturing.Create two objects of the
Car
class with different brands and years.Call the
showDetails()
method on both objects.
Breaking Down the Problem
We will walk through each requirement step by step:
Creating a Class: The
Car
class will have two attributes:brand
(for the car's brand name) andyear
(for the year the car was manufactured).Using a Constructor: A constructor is a special method in Java used to initialize objects. It will use the
this
keyword to differentiate between class attributes and constructor parameters.Method to Show Details: The
showDetails()
method will be responsible for printing the car’s brand and year.Creating Objects: We will create two objects of the
Car
class, each representing a different car with its unique brand and year.Calling Methods: Finally, we will call the
showDetails()
method to display information about both cars.
Java Code Example
public class Car {
// Step 1: Declare the attributes
String brand;
int year;
// Step 2: Constructor to initialize the attributes
public Car(String brand, int year) {
this.brand = brand; // "this" refers to the class attribute
this.year = year;
}
// Step 3: Method to display the car details
public void showDetails() {
System.out.println("Car Brand: " + brand + ", Year: " + year);
}
// Step 4 and 5: Main method to create objects and display their details
public static void main(String[] args) {
// Creating two car objects
Car car1 = new Car("Toyota", 2020);
Car car2 = new Car("Honda", 2022);
// Calling showDetails() method for each car
car1.showDetails();
car2.showDetails();
}
}
Explanation of the Code
Class Declaration:
We declare a class named
Car
that will represent a car's details.Inside the class, we define two attributes:
brand
(a string for the car's name) andyear
(an integer for the year it was manufactured).
Constructor:
A constructor is a special method that is called when an object of the class is created.
In the
Car
class, we have a constructor that takes two parameters,brand
andyear
, and assigns them to the class attributes using thethis
keyword.
Method:
- The
showDetails()
method is used to print the car's information in a readable format. It accesses thebrand
andyear
attributes and prints them.
- The
Creating Objects:
In the
main
method, we create two objects,car1
andcar2
, each initialized with a different brand and year using the constructor.We pass
"Toyota", 2020
for the first car and"Honda", 2022
for the second car.
Calling the Method:
- Finally, we call the
showDetails()
method on bothcar1
andcar2
objects to print their details.
- Finally, we call the
Output
Car Brand: Toyota, Year: 2020
Car Brand: Honda, Year: 2022
Key Concepts Covered
Classes and Objects:
- A class in Java is like a blueprint, and objects are instances created from that blueprint. The
Car
class is a blueprint, andcar1
andcar2
are objects.
- A class in Java is like a blueprint, and objects are instances created from that blueprint. The
Attributes:
- These are the properties or characteristics of a class. In our example,
brand
andyear
are the attributes of theCar
class.
- These are the properties or characteristics of a class. In our example,
Constructor:
- A constructor initializes an object with values when it is created. We used a constructor to set the brand and year of the car when creating the objects
car1
andcar2
.
- A constructor initializes an object with values when it is created. We used a constructor to set the brand and year of the car when creating the objects
Methods:
- A method in Java is a block of code that performs an action. The
showDetails()
method in this case prints the car’s brand and year.
- A method in Java is a block of code that performs an action. The
The
this
Keyword:- The
this
keyword is used inside the constructor to refer to the current object's attributes, helping to distinguish between the attributes and the parameters with the same name.
- The
Conclusion
In this blog, we learned how to create a simple Car
class in Java, define attributes, use a constructor to initialize objects, and write a method to display the object's data. This example demonstrates key Object-Oriented Programming concepts like classes, objects, constructors, and methods.
Once you understand these basic concepts, you can easily apply them to create more complex classes and objects to model real-world scenarios. Whether it's for managing car details or representing other entities, Object-Oriented Programming is a powerful tool to organize your code efficiently!
Further Learning
Practice Exercises: Try creating another class like
Mobile
with attributesbrand
,model
, and a methoddisplayDetails()
. Create objects for different mobile brands and display their details.Advanced Topics: After mastering basic classes and objects, move on to more advanced OOP concepts like inheritance, polymorphism, and abstraction!
Subscribe to my newsletter
Read articles from Sabat Ali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
