A Leaner Approach To Building Projects
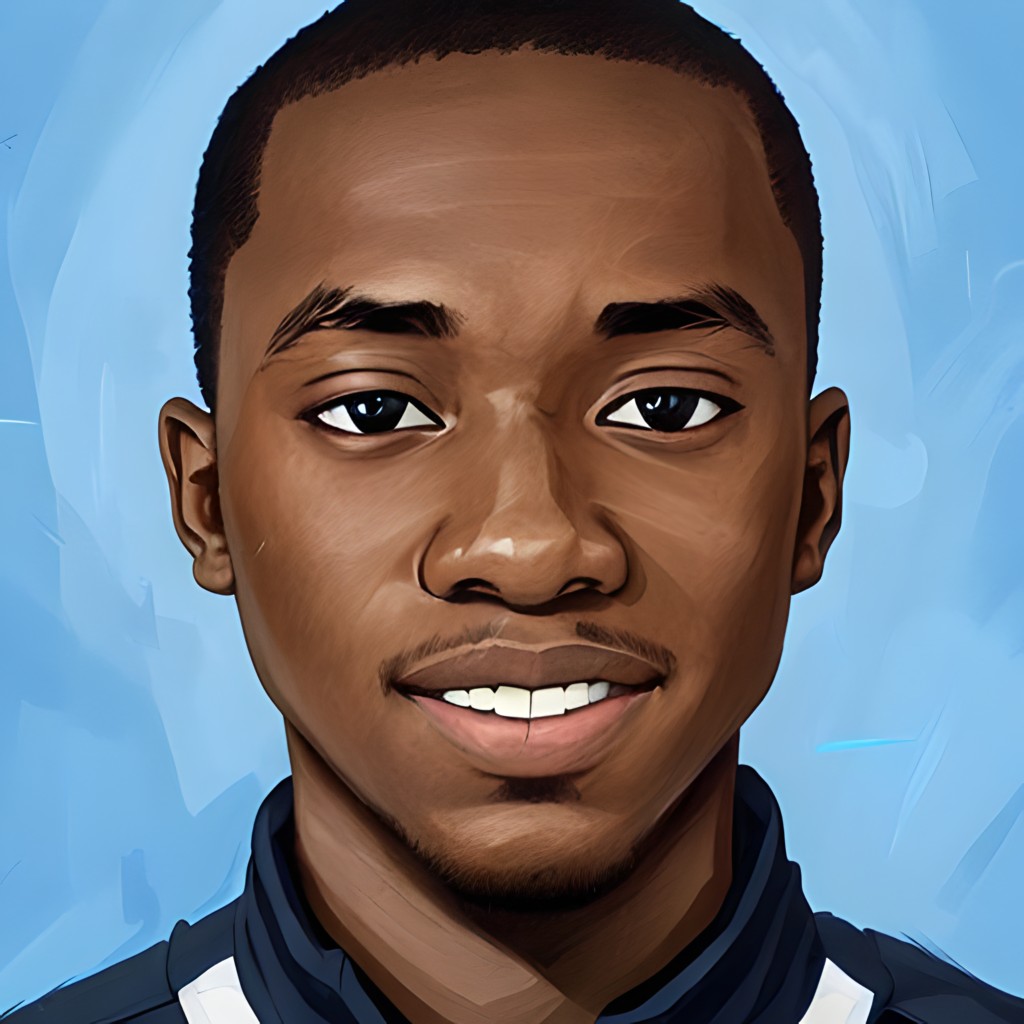
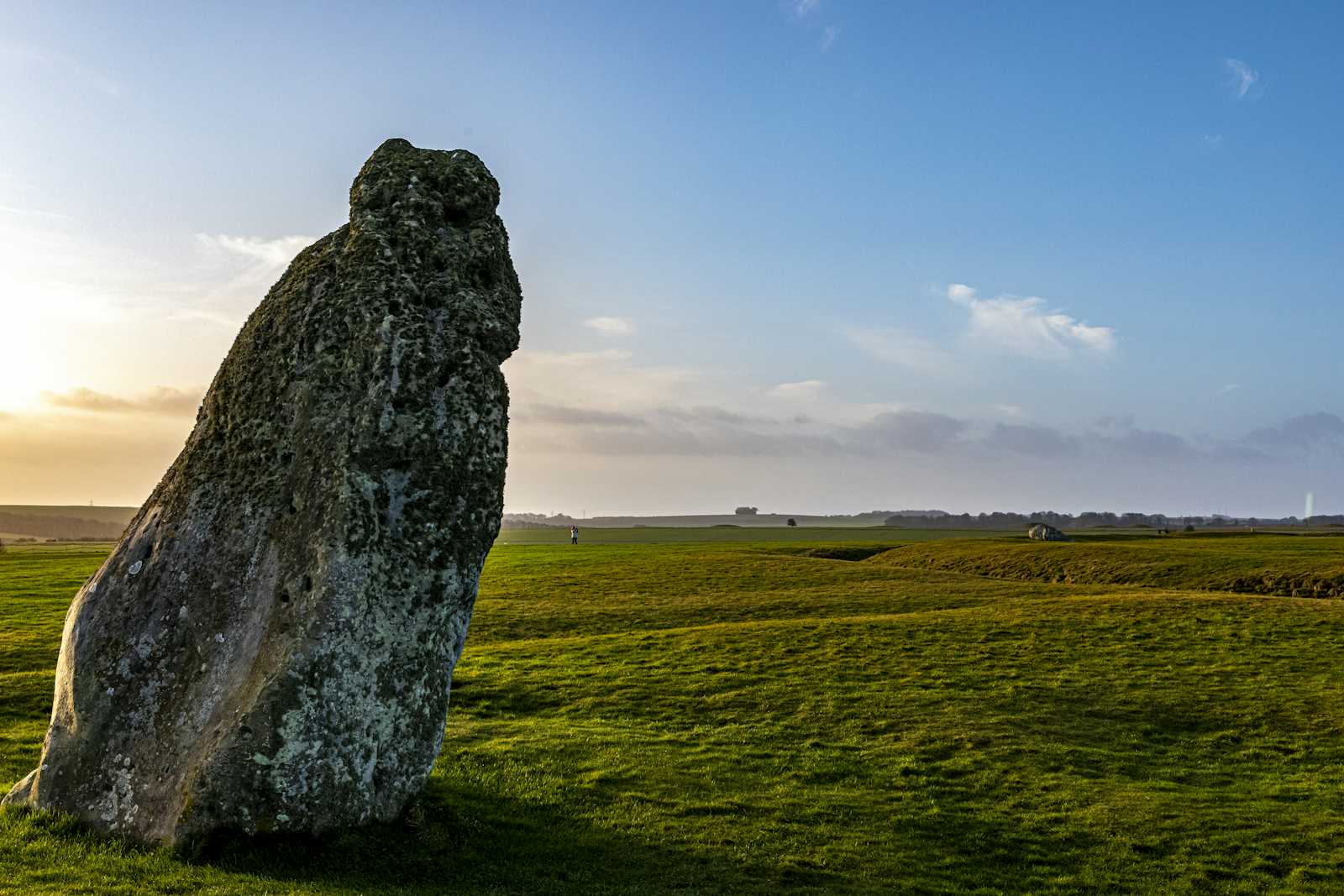
Prerequisites
To get the most out of this guide, you should have some basic knowledge of:
Git
GitHub
Basic terminal commands
Setting Up the Folder with Git
Step One
Create a main project folder:
mkdir mainProjectFolder
cd mainProjectFolder
Step Two
Initialize a GitHub repository locally:
git init
Step Three
Create an online repository for your folder (link provided).
Step Four
Connect the local and online repositories:
git remote add origin "link"
Step Five
Add a README file within the folder:
touch readMe.md
A README
file is used to describe the project and provide basic information. You should include the following in your README:
A title
An explanation
An installation guide
Step Six
Make your first push by running these commands sequentially:
git add .
git commit -m "commit message"
git push --set-upstream origin main
Folder Structure
Step One
Create a .gitignore
file:
touch .gitignore
This file specifies which folders and files should not be uploaded to the repository.
Step Two
Specify the files and folders to ignore. You can find .gitignore
templates tailored to various languages and frameworks.
Step Three
Create two or more folders based on how you want to organize your code. For example, if you primarily work with Webpack, you might create three folders: one for source code files, one for bundled files, and one for tests (when you start learning about testing). Inside the mainProjectFolder
, create these folders:
mkdir src
mkdir dist
mkdir tests
Step Four
Create subfolders as needed. For example, if using the MVC (Model-View-Controller) pattern, you can create subfolders for model, view, and controller:
mkdir src/model
mkdir src/view
mkdir src/controller
Step Five
Make a commit to save your changes:
git add .
git commit -m "added directories"
Feature Boards
Before I established a coding system, I wasn't aware of GitHub's project boards. GitHub boards allow you to organize your projects into "To Do," "Doing," and "Done" categories.
Step One
Log in to your GitHub profile and navigate to the Projects section.
Step Two
Create a new project.
Step Three
Name the project and choose a view. For simplicity, I recommend using boards, though you can explore other views such as roadmaps and tables.
Step Four
After creating the project, you can add a new task, which will be in the To Do
section. This will also appear as an issue. Don't forget to specify the repository by typing an #
.
Step Five
With your project view ready, move tasks to Doing
and Done
as you work on them. This helps you stay focused. Pro tip: Avoid having more than three tasks in the Doing
section at once.
With your project board set up, you can organize and prioritize your tasks more effectively rather than juggling multiple features and potentially creating buggy code.
Branches
To work effectively with teams, you need to understand how to use Git branches and merge features. For basic workflows, you should have a feature
branch alongside your main branch. In Git, a branch is a copy of the main branch (usually master
or main
) where you can work on different features without affecting the main branch.
Step One
Create a new branch:
git branch release
git branch feature
Step Two
Switch to the new branch:
git checkout feature
Now you are in the feature
branch, a copy of the main branch.
Step Three
Check the status of the current branch and add changes to the staging area:
git status
git add --all
- This adds all files in the branch.
git commit -m "add a new feature in a branch"
Step Four
Switch back to the main branch and merge the changes from the feature
branch:
git checkout main
git merge feature
Refactoring
As you work on multiple features and technologies, consider refactoring and improving existing code. You can create a new branch specifically for refactoring. To improve your code base, focus on readability, use descriptive names for variables and functions, add comments, remove duplication (following the DRY - Don't Repeat Yourself principle), optimize time and space complexity with appropriate data structures and algorithms, and enhance error handling.
Conclusion
In this guide, we’ve walked through the essential steps for setting up a Git-based project, organizing your folder structure, using feature boards, managing branches, and maintaining code quality through refactoring. By following these practices, you will establish a solid foundation for managing your project efficiently, whether you're working solo or as part of a team.
Effective use of Git and GitHub enhances your workflow, making it easier to track changes, collaborate with others, and ensure that your code remains clean and well-organized. Embracing these practices will not only streamline your development process but also improve your ability to handle complex projects and adapt to evolving requirements.
Remember, the key to successful project management and development is consistency. Regularly update your repository, keep your branches organized, and continually refine your code. With these strategies in place, you'll be well-equipped to tackle your projects with confidence and efficiency.
Subscribe to my newsletter
Read articles from Kelvin Okuroemi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
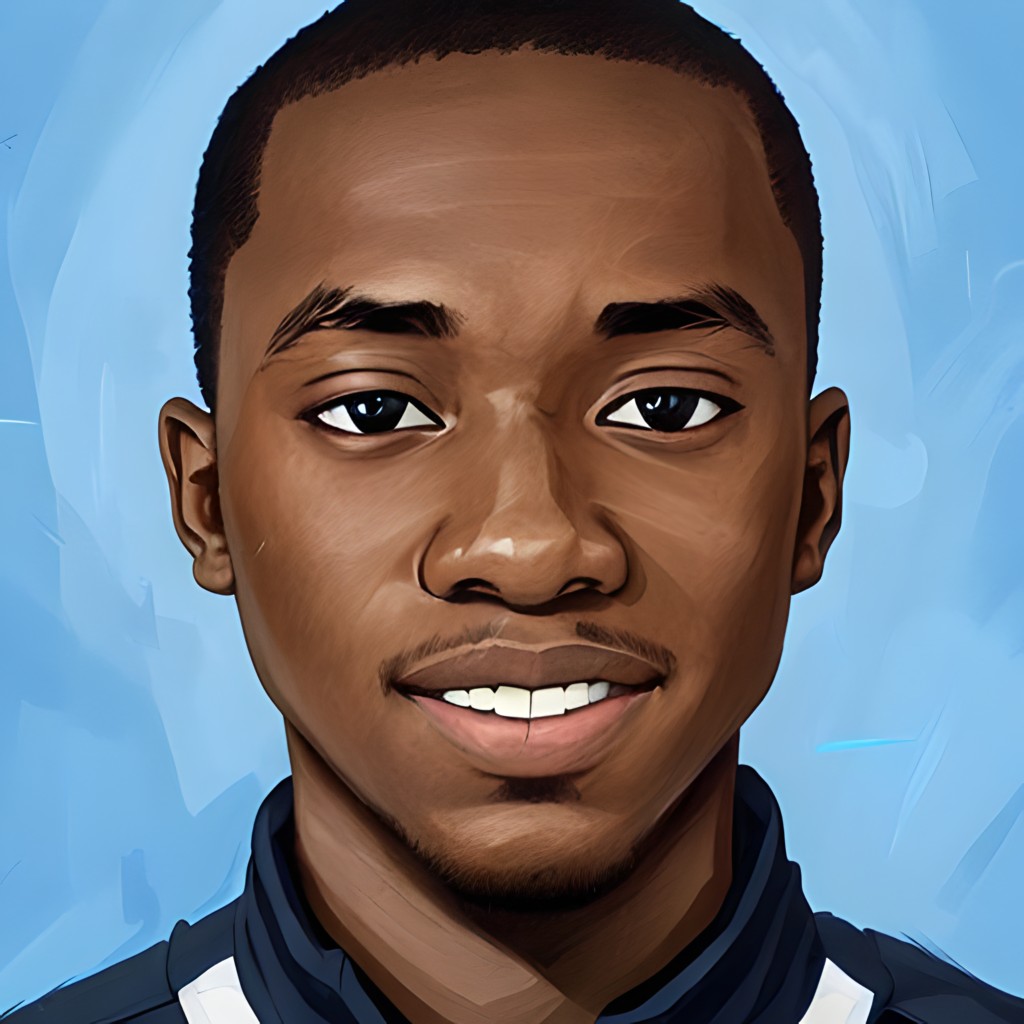
Kelvin Okuroemi
Kelvin Okuroemi
Life is becoming more interesting every day... Currently, I am invested in being a better backend engineer, and also a medical doctor in training. I have a keen interest in cloud engineering, biotechnology and molecular biology, and a fascination with VR. In my free time, I enjoy reading, playing strategy games, experiencing music, and exploring philosophy.