Best Practices for Writing Clean and Efficient Swift Code
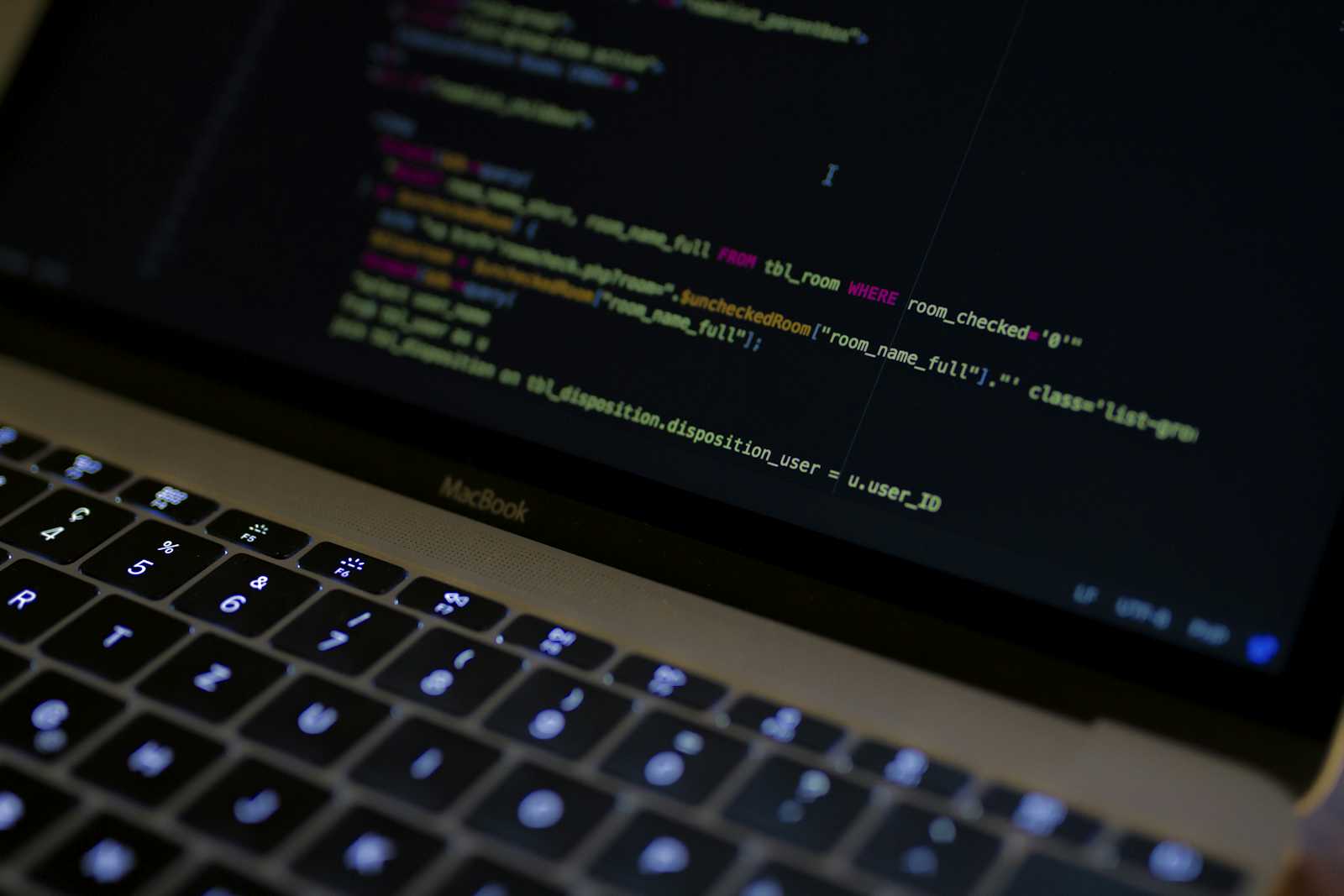
Swift, Apple's powerful and intuitive programming language, offers developers a wide range of tools and features to create robust applications. However, with great power comes great responsibility. Writing clean, efficient, and maintainable code is crucial for long-term project success. In this blog post, we'll explore some best practices that will help you elevate your Swift coding skills.
Use Clear and Descriptive Naming
Good naming conventions make your code self-documenting and easier to understand:
· Use camelCase for variable and function names
· Use PascalCase for type names (classes, structs, enums)
· Be descriptive but concise
· Avoid abbreviations unless they're widely understood
// Good
let userAge = 25
func calculateTotalPrice() { ... }
// Avoid
let a = 25
func calcTP() { ... }
Leverage Swift's Type Inference
Swift's type inference can make your code cleaner and more readable. Use it when the type is obvious:
// Good
let name = "John Doe"
let numbers = [1, 2, 3, 4, 5]
// Unnecessary
let age: Int = 30
let isActive: Bool = true
Use Optionals Safely
Swift's optionals are powerful, but they need to be handled carefully:
· Use optional binding or nil coalescing when unwrapping
· Avoid force unwrapping (!
) unless you're absolutely sure the value isn't nil
// Good
if let unwrappedName = optionalName {
print("Hello, \(unwrappedName)")
}
let displayName = optionalName ?? "Guest"
// Avoid
print("Hello, \(optionalName!)")
Embrace Functional Programming Concepts
Swift supports functional programming paradigms, which can lead to more concise and readable code:
// Using map, filter, reduce
let squares = numbers.map { $0 * $0 }
let evenNumbers = numbers.filter { $0 % 2 == 0 }
let sum = numbers.reduce(0, +)
Use Access Control
Properly scope your properties and methods using access control keywords:
·private
: accessible only within the defining type
· fileprivate
: accessible within the current file
· internal
: (default) accessible within the module
· public
: accessible from any module that imports yours
· open
: like public, but allows subclassing and overriding outside the module
public class MyClass {
private var internalState: Int
public func publicMethod() { ... }
}
Follow the DRY Principle
DRY (Don't Repeat Yourself) is crucial for maintaining clean code:
· Extract repeated code into functions or extensions
· Use protocol extensions for shared behavior
extension Collection where Element: Numeric {
func sum() -> Element {
return reduce(0, +)
}
}
Use Enums for Grouped Constants
Enums are great for grouping related constants:
enum Config {
static let baseURL = "https://api.example.com"
static let maxRetries = 3
static let timeout = 30.0
}
// Usage
let url = Config.baseURL
Prefer Structs Over Classes When Possible
Structs are value types and generally more efficient:
· Use structs for simple data models
· Use classes when you need inheritance or reference semantics
struct User {
let id: Int
var name: String
}
Write Self-Documenting Code
While comments are sometimes necessary, aim to write code that's self-explanatory:
// Less ideal
// Check if user is adult
if user.age >= 18 { ... }
// Better
let isAdult = user.age >= 18
if isAdult { ... }
Use Swift's Latest Features
Stay updated with Swift's latest features and use them when appropriate:
· Utilize async/await
for asynchronous code
· Use property wrappers like @Published
in SwiftUI
· Leverage result builders for creating DSLs
Conclusion
Writing clean and efficient Swift code is an ongoing process that requires attention to detail and a commitment to best practices. By following these guidelines, you'll create more maintainable, readable, and performant Swift applications. Remember, the goal is not just to write code that works, but code that's easy to understand, modify, and scale as your project grows.
Keep practicing, stay updated with the latest Swift developments, and always look for ways to improve your code quality. Happy coding!
Subscribe to my newsletter
Read articles from Alperen Sarışan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by