Exploring the 'this' Keyword in JavaScript: My Learning Journey

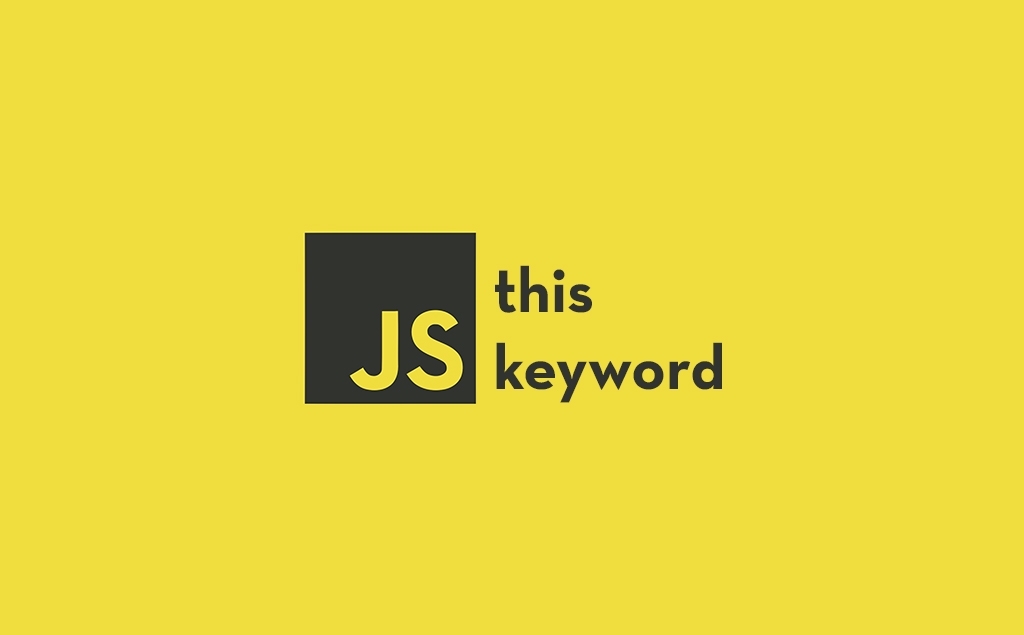
Hello friends, welcome to my weekly "Learn in Public" blog where I share updates on my journey learning the MERN stack. In this blog, I will be covering the this
keyword and how it is used..
Basically, the this
keyword refers to different objects depending on how it is being used.
1)when used within a method of an object
Firstly, I learned that a method is basically a function present inside an object.
this
when used within an method of an object it points to that object
const person={
firstName:"Aniket",
age:24,
about(){
console.log(this);
}
}
person.about(); // this prints the person object
//output
Here you can see the output which prints the person
object
2)When this
is used by itself, it will point to the global object:
It depends on where i am using this
a)On its own
Here the below code willl print the global object that will be window object
b)In “use strict”
mode
Here this
works differently
"use strict";
function about() {
console.log(this);
}
about();
Here i will get output as undefined
3)when we use this
in an function
Here this
will points to the global object
function about() {
console.log(this);
}
about();// output will be global object
4)methods like call()
, apply()
and bind()
can ressign this
to any desired object
a)call() method:
This method calls the function directly and sets this
as an first argument to the call method
const user1={
firstName:"Aniket",
age:"24",
about(){
console.log(this.firstName,this.age);
}
}
const user2={
firstName:"Shubham",
age:26,
}
user1.about.call(user2);
b)apply() method
It is basically the same as the call method, but the only difference is that it takes the preceding arguments as an array.
const user1={
firstName:"Aniket",
age:"24",
about(state){
console.log(this.firstName,this.age,state[0],state[1]);
}
}
const user2={
firstName:"Shubham",
age:26,
}
user1.about.call(user2,["maharashtra","Mumbai"]);
Here, I am getting the array as a parameter, and I can perform operations on it as an array. Refer to the output for the same.
Shubham 26 maharashtra Mumbai //this is the output fir the above code snippet
c) blind() method
This method is almost similar to pervious methods but only difference is that is returns a new object
const user1={
firstName:"Aniket",
age:"24",
about(){
console.log(this.firstName,this.age);
}
}
const user2={
firstName:"Shubham",
age:26,
}
const myfunc=user1.about.bind(user2);
myfunc();
The output will be Shubham 26
Conclusion:
So, that's all for this blog. I hope you liked it. As I mentioned in my previous blogs, I know that there is a lot of content out there about this same topic, but as I am in the early phase of my "learn in public" journey and learning JavaScript, this is all I can share for now. But I promise once I have completed my basics, I am planning to work on some projects to build the logic. Once that begins, I will be sharing the logic, problems that I encountered, and how I solved them. So, that's it for the week. See you next week. Do subscribe to my newsletter.
Subscribe to my newsletter
Read articles from Aniket Mogare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
