Comprehensive Guide to Data Preprocessing in Python for Machine Learning

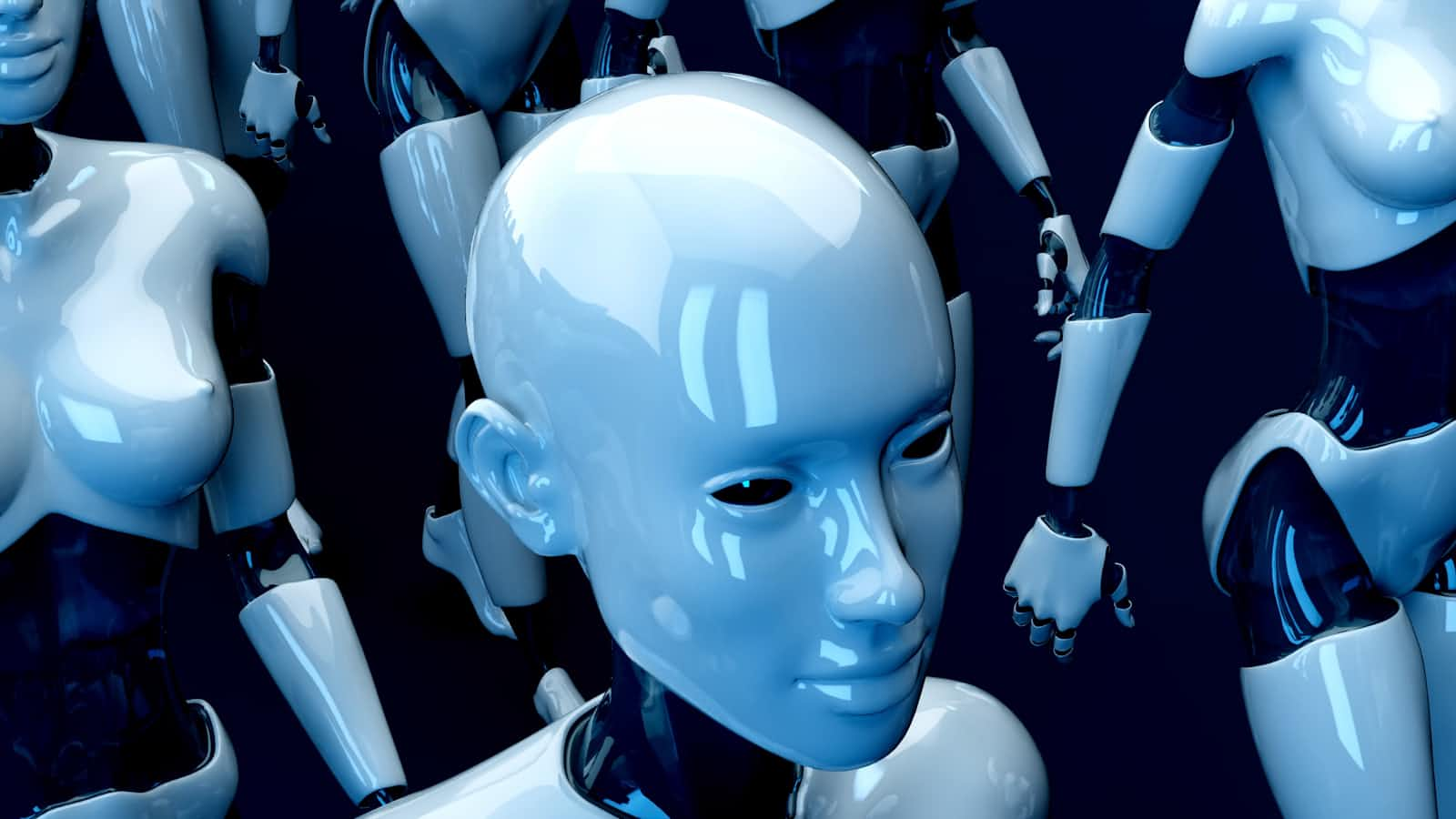
Data preprocessing is a crucial step in the machine learning pipeline to ensure the data is clean, organized, and in a format suitable for training models. Here’s an overview of key topics typically included in data preprocessing:
Topics in Data Preprocessing
Handling Missing Data:
Missing data can occur due to errors in data collection or incomplete datasets.
Common techniques to handle missing data include:
Removing missing data: Delete rows or columns with missing values.
Imputation: Replace missing values using various strategies like mean, median, mode, or interpolation.
Example using
SimpleImputer
fromsklearn
:
pythonCopy codefrom sklearn.impute import SimpleImputer
imputer = SimpleImputer(missing_values=np.nan, strategy='mean')
imputer.fit(x[:, 1:3])
x[:, 1:3] = imputer.transform(x[:, 1:3])
Encoding Categorical Data:
Machine learning models work better with numerical data, so categorical variables must be encoded.
Techniques include:
Label Encoding: Assigns each category a unique integer value.
One-Hot Encoding: Creates binary columns for each category.
Example using
OneHotEncoder
fromsklearn
:
pythonCopy codefrom sklearn.preprocessing import OneHotEncoder
encoder = OneHotEncoder()
x_encoded = encoder.fit_transform(x_categorical).toarray()
Feature Scaling:
Ensures that all features contribute equally to the model by bringing them onto the same scale.
Techniques include:
Standardization: Centers the data around zero with unit variance.
Normalization: Scales values between 0 and 1.
Example using
StandardScaler
fromsklearn
:
pythonCopy codefrom sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
x_scaled = scaler.fit_transform(x)
Splitting the Dataset into Training and Testing Sets:
It’s important to evaluate the model on unseen data. This is done by splitting the dataset into training and testing sets.
Example using
train_test_split
fromsklearn
:pythonCopy codefrom sklearn.model_selection import train_test_split x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.2, random_state=42)
Handling Imbalanced Data:
When classes are not evenly distributed, it can lead to biased models.
Techniques include:
Resampling: Either oversampling the minority class or undersampling the majority class.
Synthetic Data Generation: Using techniques like SMOTE (Synthetic Minority Over-sampling Technique).
Dealing with Outliers:
Outliers can skew model performance.
Techniques include:
Removing Outliers: Using statistical methods like the IQR (Interquartile Range) method.
Transforming Data: Applying logarithmic transformations.
Feature Engineering and Selection:
Create new features or select the most important features for the model.
Techniques include:
PCA (Principal Component Analysis) for dimensionality reduction.
Correlation Analysis to find features with the highest correlation to the target variable.
Example Workflow of Data Preprocessing in Python
pythonCopy codeimport numpy as np
import pandas as pd
from sklearn.impute import SimpleImputer
from sklearn.preprocessing import StandardScaler, OneHotEncoder
from sklearn.model_selection import train_test_split
# 1. Load dataset
dataset = pd.read_csv('Data.csv')
x = dataset.iloc[:, :-1].values
y = dataset.iloc[:, -1].values
# 2. Handle missing data
imputer = SimpleImputer(missing_values=np.nan, strategy='mean')
x[:, 1:3] = imputer.fit_transform(x[:, 1:3])
# 3. Encode categorical data
encoder = OneHotEncoder()
x_categorical = encoder.fit_transform(x[:, 0].reshape(-1, 1)).toarray()
x = np.hstack((x_categorical, x[:, 1:])) # Combine encoded with numerical features
# 4. Feature Scaling
scaler = StandardScaler()
x_scaled = scaler.fit_transform(x)
# 5. Split the data
x_train, x_test, y_train, y_test = train_test_split(x_scaled, y, test_size=0.2, random_state=42)
# Display the preprocessed data
print("Features after preprocessing:\n", x_train)
print("Target variable:\n", y_train)
Conclusion
Data preprocessing transforms raw data into a clean, usable form that can be fed into machine learning models. It includes handling missing data, encoding categorical variables, feature scaling, and more. Proper data preprocessing leads to improved model accuracy and performance.
Subscribe to my newsletter
Read articles from Prasun Dandapat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Prasun Dandapat
Prasun Dandapat
Prasun Dandapat is a Computer Science and Engineering graduate from the Academy of Technology, Hooghly, West Bengal. With a strong interest in AI and Machine Learning, Prasun is also skilled in frontend development and is an aspiring Software Development Engineer (SDE). Passionate about technology and innovation, he constantly seeks opportunities to broaden his expertise and contribute to impactful projects.