Understanding the Difference Between Next.js and React

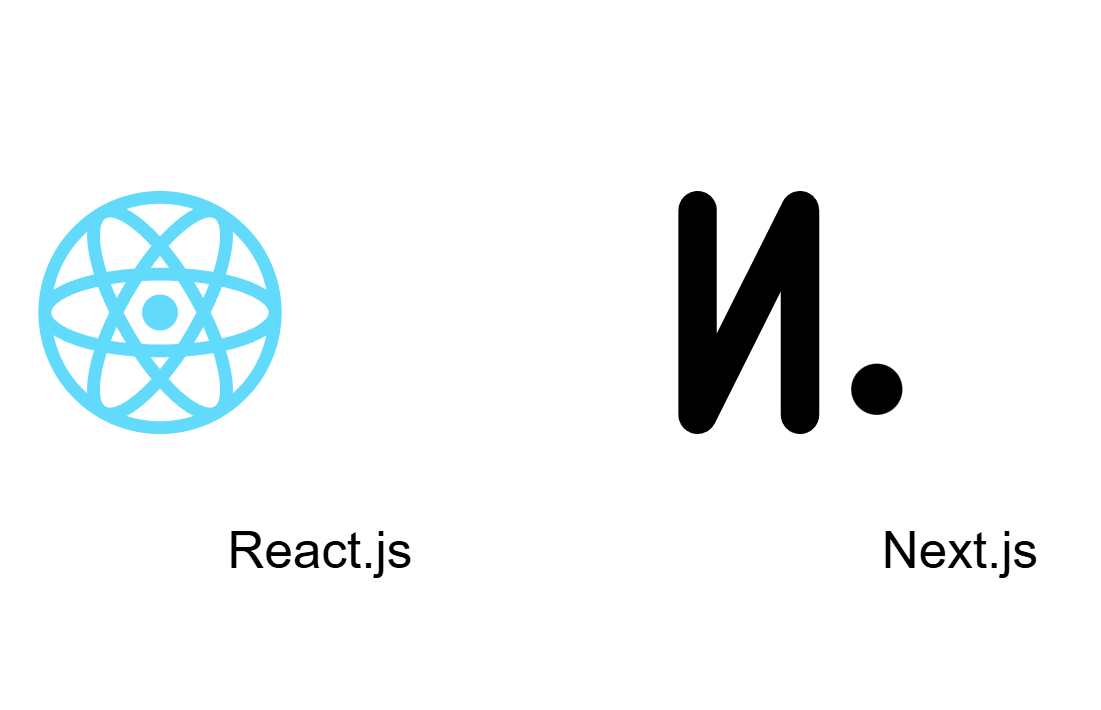
Hey everyone! Today, I want to talk about Next.js and React, two popular JavaScript frameworks that I've worked with on several projects. If you're diving into the world of frontend development, you’ve probably come across these terms. So, let’s break down what makes them different, and why you might want to use one over the other.
What is React?
React is a JavaScript library developed by Facebook for building user interfaces, particularly single-page applications (SPAs). It’s great for creating fast, dynamic user interfaces. React allows me to build components, which are small, reusable pieces of code that can manage their own state. It focuses only on the UI layer and doesn’t concern itself with routing or server-side rendering (SSR).
Here’s a simple React example:
import React from 'react';
function App() {
return (
<div>
<h1>Welcome to my React App!</h1>
<p>This is a simple React component.</p>
</div>
);
}
export default App;
In this example, React renders a simple component to the DOM. It’s super flexible and easy to get started with.
What is Next.js?
Next.js, on the other hand, is a framework built on top of React. While React handles the UI, Next.js provides additional features like server-side rendering (SSR), static site generation (SSG), and routing out of the box. It’s perfect when I need my app to load fast and be SEO-friendly, as it can render pages on the server before sending them to the browser.
Here’s how a similar app looks in Next.js:
import React from 'react';
export default function Home() {
return (
<div>
<h1>Welcome to my Next.js App!</h1>
<p>This is a simple Next.js page component.</p>
</div>
);
}
Notice that I didn't have to set up any routing here! Next.js automatically knows that this component is the homepage because it’s inside the pages
folder. That’s one of the key benefits of using Next.js—automatic routing.
Key Differences
Routing: In React, I have to use libraries like
react-router
to handle routing, while Next.js provides built-in routing by default.Server-Side Rendering (SSR): React is client-side by default, but with Next.js, I can choose to render pages on the server, which makes my apps load faster and helps with SEO.
Static Site Generation (SSG): Next.js can pre-render pages at build time, which is great for static websites.
Deployment: Next.js apps can be easily deployed on platforms like Vercel (which is also developed by the Next.js team), while React apps typically need a hosting service and some extra setup for SSR.
Why Use Next.js Over React?
SEO: If my app needs to be SEO-friendly, Next.js is the way to go, thanks to server-side rendering.
Faster Loading Times: Next.js allows me to pre-render pages, which makes them load faster.
Less Setup: Next.js comes with built-in features like routing and SSR, so I don’t need to install additional libraries.
On the other hand, if I’m building a basic single-page app where SEO isn’t a concern, I’d stick with React. It’s lightweight and gives me full control over the project.
Final Thoughts
In the end, the choice between Next.js and React comes down to the type of project I’m working on. For static sites or apps that require SEO, Next.js is a fantastic choice. If I’m focusing on a client-side application with complex UI, then React might be all I need.
Hope this helps clarify the differences between these two!
Let me know if you have any questions or comments.
Subscribe to my newsletter
Read articles from Rohan Shrivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rohan Shrivastava
Rohan Shrivastava
Hi, I'm Rohan, a B.Tech graduate in Computer Science (Batch 2022) with expertise in web development (HTML, CSS, JavaScript, Bootstrap, PHP, XAMPP). My journey expanded with certifications and intensive training at Infosys, covering DBMS, Java, SQL, Ansible, and networking. I've successfully delivered projects, including a dynamic e-commerce site and an Inventory Management System using Java. My proactive approach is reflected in certifications and contributions to open-source projects on GitHub. Recognized for excellence at Infosys, I bring a blend of technical proficiency and adaptability. Eager to leverage my skills and contribute to innovative projects, I'm excited about exploring new opportunities for hands-on experiences. Let's connect and explore how my skills align with your organization's goals.