Understanding Python Syntax and Semantics: A Comprehensive Guide for Beginners

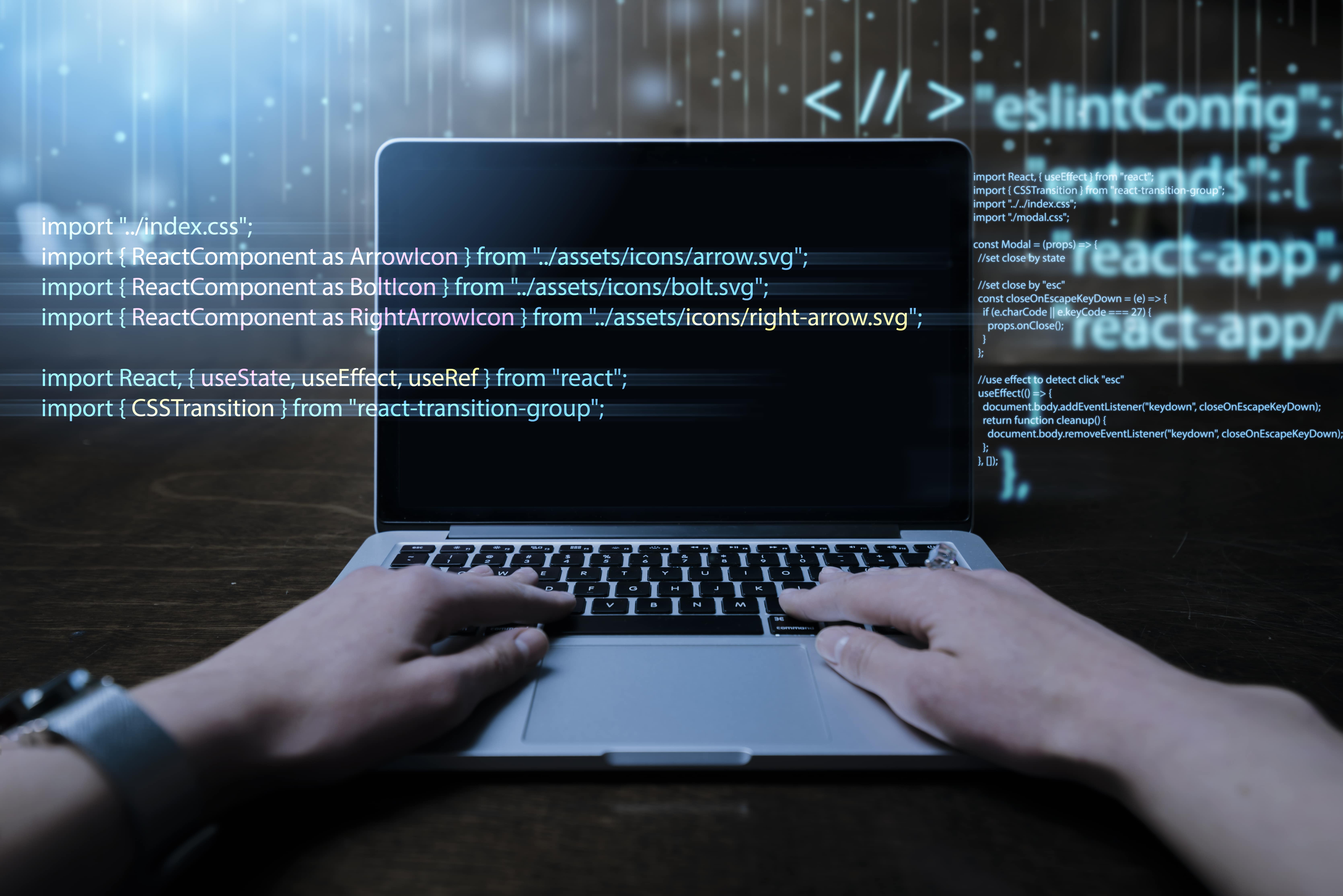
Python has become one of the most popular programming languages in the world, thanks to its simplicity, readability, and versatility. Whether you're diving into programming for the first time or transitioning from another language, understanding Python's syntax and semantics is crucial. This blog post aims to demystify these core concepts, providing a foundation for anyone looking to excel in Python.
What Is Python Syntax?
Python syntax refers to the set of rules that define the structure of a Python program. It's essentially the grammar that governs how you write Python code. Python is known for its clean and readable syntax, which makes it an excellent choice for beginners and experienced developers
alike.
Key Features of Python Syntax
1. Indentation:
- Unlike many other programming languages
,
Python uses indentation to define code blocks. This feature eliminates the need for braces {}
or other delimiters, making the code more readable.
- For example:
```python
if True:
print("This is indented")
```
Here, the indentation indicates that the print
statement belongs to the if
block.
2. Case Sensitivity:
- Python is case-sensitive, meaning Variable
and variable
would be recognized as two distinct entities.
3. Comments:
- Python supports single-line comments using the #
symbol. Comments are crucial for explaining code, especially in collaborative environments.
```python
# This is a comment
print("Hello, World!")
```
4. Statements and Expressions:
- In Python, a statement is an instruction that the Python interpreter can execute, such as print("Hello, World!")
. An expression, on the other hand, is a combination of values and operators that can be evaluated to a value.
5. Line Continuation:
- Python allows for the continuation of long statements across multiple lines using a backslash \
.
```python
result = 1 + 2 + 3 + \
4 + 5 + 6
```
6. Multiple Statements on a Single Line:
- Although not recommended for readability, Python allows multiple statements on a single line separated by a semicolon ;
.
```python
x = 1; y = 2; print(x + y)
```
What Is Python Semantics?
While syntax deals with the structure of code, semantics concerns the meaning of that code. Understanding Python semantics involves knowing what the code does when it's executed. This includes understanding variable types, scopes, control flows, and error handling.
Key Concepts in Python Semantics
1. Variables and Data Types:
- Python is dynamically typed, meaning you don't need to declare a variable's type explicitly. The interpreter determines the type based on the value assigned.
```python
x = 10 # Integer
y = 3.14 # Float
name = "John" # String
```
2. Type Conversion:
- Sometimes, you may need to convert a variable from one type to another. Python provides built-in functions like int()
, float()
, and str()
for this purpose.
```python
x = 10
y = str(x) # Converts integer to string
```
3. Mutable vs. Immutable Types:
- Understanding the difference between mutable and immutable types is crucial in Python. Mutable types (e.g., lists, dictionaries) can be changed after creation, while immutable types (e.g., strings, tuples) cannot.
```python
my_list = [1, 2, 3]
my_list[0] = 10 # This works because lists are mutable
my_tuple = (1, 2, 3)
my_tuple[0] = 10 # This raises an error because tuples are immutable
```
4. Variable Scope:
- The scope of a variable determines where that variable can be accessed within the code. Python has local, global, and nonlocal scopes.
```python
def my_function():
x = 10 # Local scope
print(x)
x = 20 # Global scope
my_function()
print(x)
```
5. Control Flow:
- Control flow refers to the order in which individual statements, instructions, or function calls are executed in a program. Python provides several control flow tools, including if
, for
, while
, and try-except
blocks.
```python
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is 5 or less")
```
6. Functions and Arguments:
- Functions are blocks of reusable code that perform a specific task. Understanding how to define functions, pass arguments, and return values is key to mastering Python semantics.
```python
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
```
7. Error Handling:
- Python uses try-except
blocks to handle errors gracefully, ensuring your program doesn’t crash unexpectedly.
```python
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
```
8. Loops and Iterators:
- Python's for
and while
loops are powerful tools for iteration, allowing you to execute a block of code repeatedly.
```python
for i in range(5):
print(i)
```
9. Modules and Packages:
- Python allows you to organize your code into modules and packages, making it easier to manage larger projects. Understanding how to import and use modules is essential for writing modular code.
```python
import math
print(math.sqrt(16))
```
10. Object-Oriented Concepts:
- Python is an object-oriented language, meaning it allows for the creation and manipulation of objects. Key concepts include classes, objects, inheritance, and polymorphism.
```python
class Dog:
def init(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return f"{self.name} says woof!"
my_dog = Dog("Rex", "German Shepherd")
print(my_dog.bark())
```
Best Practices for Writing Python Code
1. Follow PEP 8 Guidelines:
- PEP 8 is Python's style guide that outlines best practices for writing clean and readable code. Adhering to these guidelines will make your code more consistent and easier to maintain.
2. Use Meaningful Variable Names:
- Always use descriptive names for your variables and functions. This practice improves code readability and helps others understand your code quickly.
3. Keep Code DRY (Don’t Repeat Yourself):
- Avoid writing redundant code. If you find yourself repeating code blocks, consider encapsulating them in a function or loop.
4. Write Comments and Docstrings:
- Use comments and docstrings to explain your code. This is especially important when working on collaborative projects or writing code that will be maintained in the future.
5. Test Your Code:
- Regularly test your code to catch errors early. Python provides built-in testing tools like unittest
to help you ensure your code works as expected.
Conclusion
Understanding Python syntax and semantics is the first step toward becoming proficient in this versatile language. By mastering these foundational concepts, you'll be well-equipped to tackle more complex programming challenges. Whether you're aiming to build web applications, automate tasks, or delve into data science, Python's clean syntax and powerful semantics will be your allies.
Remember, practice is key. The more you code, the more comfortable you'll become with Python's syntax and semantics. So, start coding today and take your first step toward Python mastery!
Subscribe to my newsletter
Read articles from Steve directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
