Java Console Input and Output Explained: Scanner, println, print, and printf

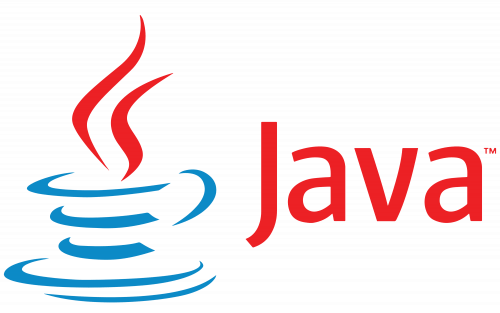
Hey everyone,
Welcome to the third post in our Java series! Whether you're new to programming or looking to refresh your skills, this series will guide you through Java from the basics to advanced topics. Let's get started!
Prologue
We’ve been using println
for a while now, but today we’ll dive deeper into how to use it properly, understand how it works, and learn different ways to print output. Additionally, we’ll explore how to take input from the user, making our applications more interactive.
Scanner
In Java, one of the most common ways to take input from the user is by using the Scanner class, which is part of the java.util
package. The Scanner class provides methods to read various types of primitive data (such as int
, float
, and String
) from the keyboard and store these values in variables.
To use the Scanner, you typically create an object of the class once in a given block of code. This object can then be used multiple times to gather input from the user without needing to create new instances.
It's considered a good practice to close the Scanner object after you finish using it. This helps free up system resources that the Scanner was utilizing.
Syntax of close(): scannerObject.close();
IllegalStateException
at runtime.import java.util.*; // Has Scanner class in it and is compulsory to import it
// Syntax for creating an object of Scanner
Scanner reference_variable_name = new Scanner(System.in);
// Reads input from the keyboard
Some methods provided by the Scanner class
Method Name | Description |
nextInt() | Accepts int value |
nextByte() | Accepts byte value |
nextLong() | Accepts long value |
nextFloat() | Accepts float value |
nextLine() | Accepts string value |
nextDouble() | Accepts double value |
nextShort() | Accepts short value |
nextBoolean() | Accepts Boolean value |
next() | Accept string till whitespace |
We will call a method from Scanner class to read input, then store the returned value in the variable
// The method from the Scanner class will read the input
datatype variable_name = scannerObject.method_Of_Scanner_Class();
// The result will be stored in the variable_name
// The datatype of the variable must match the return type of the method you are calling
Handling Console Output
Java package: java.io
provides a predefined class name PrintStream for displaying data on standard output device.
PrintStream
class provides following methods for displaying data.
Formatted Output
printf()
Unformatted Output
print()
println()
We are provided with an object name System.out
which is of type PrintStream. It is used for outputting the text to console (standard output). When you use methods like System.out.print()
, System.out.println()
, or System.out.printf()
, you're calling those methods on the PrintStream instance represented by System.out
.
You can also create your own object of PrintStream class with
PrintStream variable_name = new PrintStream(System.out);
and then use the variable name to call the method to print the output
printf
The printf()
method is a powerful tool for formatting output in java, making it easier to present the data in a structured way like you want, it has c language like syntax.
Basic syntax of printf()
is System.out.printf(String format, args list);
Here string format is the content which is displayed into the console, and args are the values that are to be printed, corresponding to the format specifiers (acts as a placeholder) specified in the format string . Each specifier in the format string corresponds to an argument in the args
list (which is comma separated list of variable name).
Some of the format specifiers are:
format specifier | description |
%d | integer in decimal number system |
%x | integer in hexadecimal number system |
%o | integer in octal number system |
%f | fixed decimal notation (floating point number) |
%e | computerized scientific notation |
%c | UNICODE character |
%s | string |
%t | date and time |
println
The primary purpose of println()
is to print text followed by a newline character, ensuring that any subsequent output starts on a new line.
Basic Syntax of println is System.out.println(x + "actual string");
Here x is a variable or literal of any data type (including primitive types, objects, or strings). The println()
method automatically converts it to a string representation if it's not already a string (by calling toString()
method).
You can concatenate multiple values using + operator into a single println()
.
There are a lot of similarity between print()
and println()
but Unlike println()
, the print()
method does not append a newline character at the end of the output, meaning that subsequent output will continue on the same line, Allows multiple output on the same line. That is the only difference between print()
and println()
.
Program
import java.io.*; // using for making our own object of PrintStream
import java.util.*; // using for taking input using Scanner
class Output{
public static void main(String[] args) {
// Creating a Scanner to read input from System.in (keyboard)
Scanner sc = new Scanner(System.in);
System.out.print("Enter your name: "); // Using print(), the cusor does not move to new/next line
String name = sc.nextLine(); // Reads the name input from the user and save it in "name"
PrintStream ps = new PrintStream(System.out); // Creating our own PrintStream object instead of System.out, need to import java.io
ps.println("Hello, " + name + "!"); // cursor moves to next line autometically
System.out.printf("Bye, %s! \n", name); // Using formatted way of printing the output
// Closing the Scanner to free resources
sc.close();
}
}
OUTPUT:
Program (closing Scanner object)
import java.util.*; // For taking input from user
class Input {
public static void main(String[] args) {
// Creating a Scanner to read input from System.in (keyboard)
Scanner sc = new Scanner(System.in);
System.out.println("Enter your name: "); // Will place the cursor to the new line
String name = sc.nextLine(); // Reads the name input from the user
// Closing the Scanner to free resources
sc.close();
System.out.println("Enter your age: ");
// After closing, you can't use the object of Scanner, using it will throw an error at runtime
// Uncommenting the below line will cause a runtime error
// int age = sc.nextInt(); // This line will throw an IllegalStateException, as shown below
}
}
OUTPUT:
What’s next?
So, today we learned about Handling console Input and Output and there is much more to come. In the next part, we’ll learn to work on Data Types, which are the foundation of creating data members.
So, Stay tuned!!
PS: I am also learning Java while teaching it. I believe in continuous growth and am committed to learning as much as I am teaching. If you have any questions, suggestions, or just want to chat about Java, please leave a comment! I'm also new to blogging, so any feedback is appreciated as I strive to improve. Thank you for joining me on this journey! Let's learn and grow together.
Subscribe to my newsletter
Read articles from Sarthak Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sarthak Sharma
Sarthak Sharma
Hi, I’m Sarthak, a passionate tech enthusiast, a student and blogger. I’m on a mission to share the things that i learn or find interesting.