Code Splitting in Webpack: A Comprehensive Guide
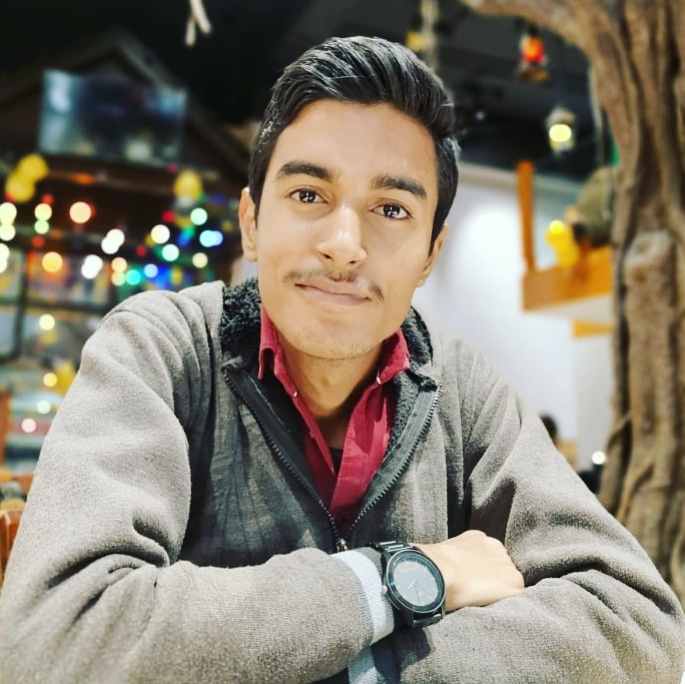
Table of contents
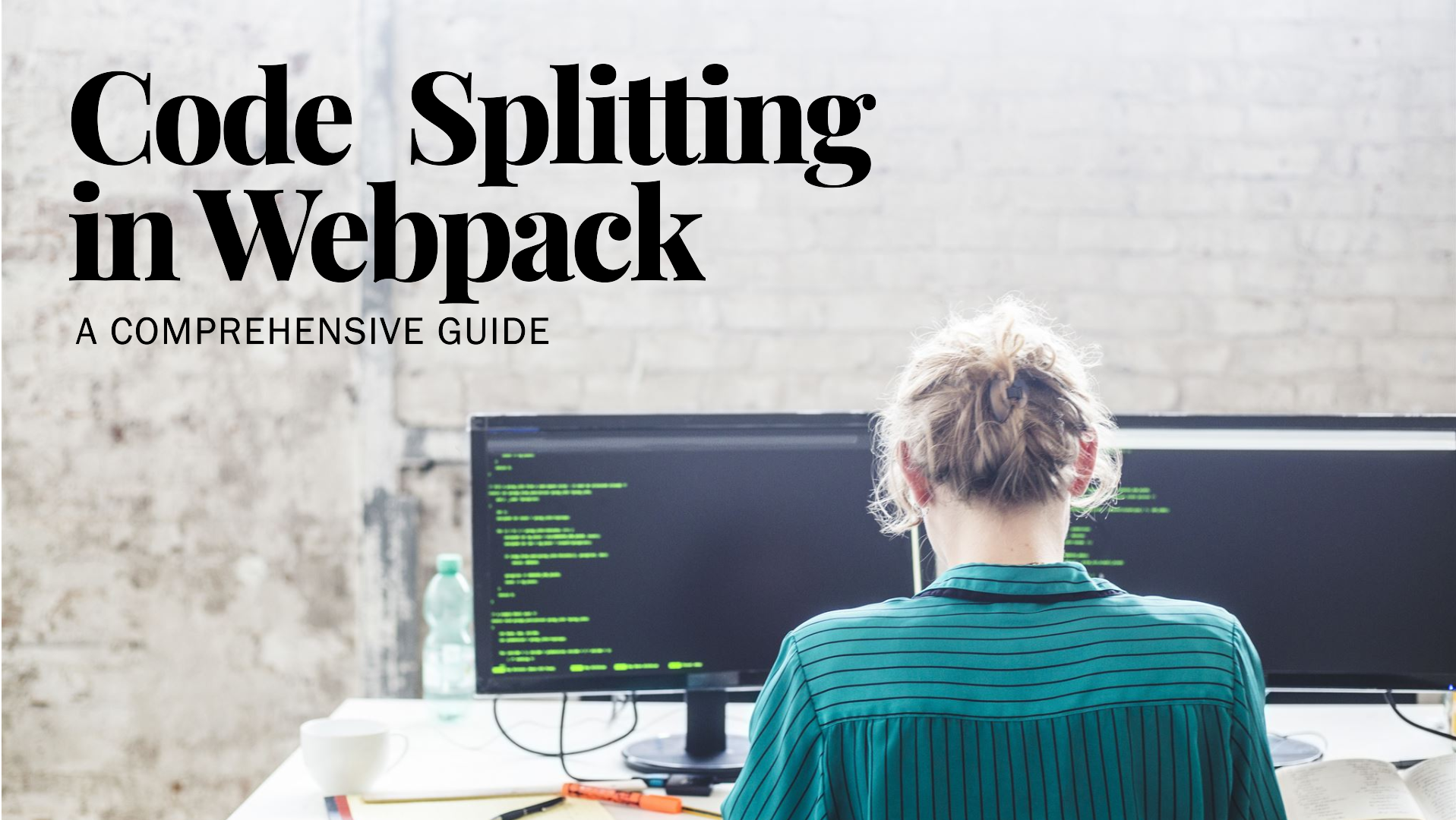
Code splitting is a technique used in web development to divide a large JavaScript bundle into smaller, more manageable chunks. This improves the initial load time of your web application, especially for users with slower internet connections. When code is split, only the necessary chunks are loaded initially, and the rest are loaded on demand as needed.
Why Use Code Splitting?
Improved Initial Load Time: By loading only the essential code upfront, you can significantly reduce the initial load time of your application.
Better User Experience: Faster load times lead to a better user experience, as users are less likely to abandon your site due to slow performance.
Reduced Bandwidth Usage: Code splitting can help reduce bandwidth usage, as only the required code is transferred over the network.
How Code Splitting Works in Webpack
Webpack provides several mechanisms for code splitting:
Entry Points: Define multiple entry points in your Webpack configuration. Each entry point will generate a separate chunk.
Dynamic Imports: Use JavaScript's
import()
function to dynamically load modules on demand. This allows you to load code only when it's actually needed.SplitChunksPlugin: This plugin automatically extracts commonly used modules into separate chunks, which can be reused by multiple entry points.
Example Using Dynamic Imports
// main.js
import('./componentA').then(module => {
// Use componentA here
});
// componentA.js
// ... component code
In this example, componentA
is loaded dynamically using import()
. It will be loaded as a separate chunk only when needed, improving the initial load time of the main application.
Example Using SplitChunksPlugin
// webpack.config.js
module.exports = {
optimization: {
splitChunks: {
chunks: 'all',
minSize: 30000,
maxSize: 0,
minChunks: 1,
maxAsyncRequests: 5,
maxInitialRequests: 3,
automaticNameDelimiter: '-',
enforceModuleSeparations: true,
cacheGroups: {
default: false,
vendors: {
test: /[\\/]node_modules[\\/]/,
priority: -10
},
commons: {
test: /[\\/]src[\\/]/,
name: 'commons',
chunks: 'initial'
}
}
}
}
};
This configuration extracts common modules from node_modules
into a vendors
chunk and common modules from the src
directory into a commons
chunk.
Best Practices for Code Splitting
Identify Frequently Used Modules: Split frequently used modules into separate chunks to improve load times.
Consider Chunk Size: Balance chunk size with the number of chunks to avoid excessive HTTP requests.
Use Runtime Caching: Enable runtime caching to avoid unnecessary re-downloads of chunks.
Monitor Performance: Use tools like Webpack Bundle Analyzer to analyze your bundle size and identify opportunities for optimization.
By effectively implementing code splitting in Webpack, you can significantly improve the performance and user experience of your web applications.
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
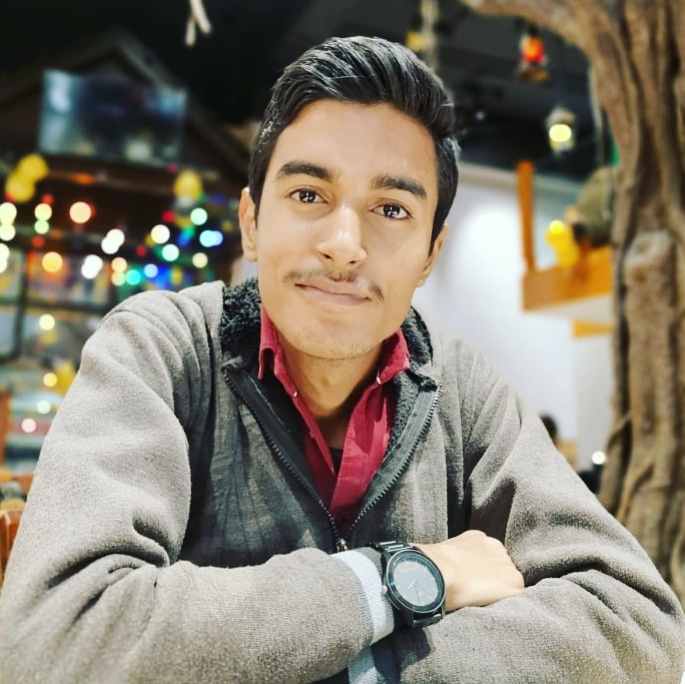
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.