[2024] How to Add a Watermark in Word or Remove It with Python

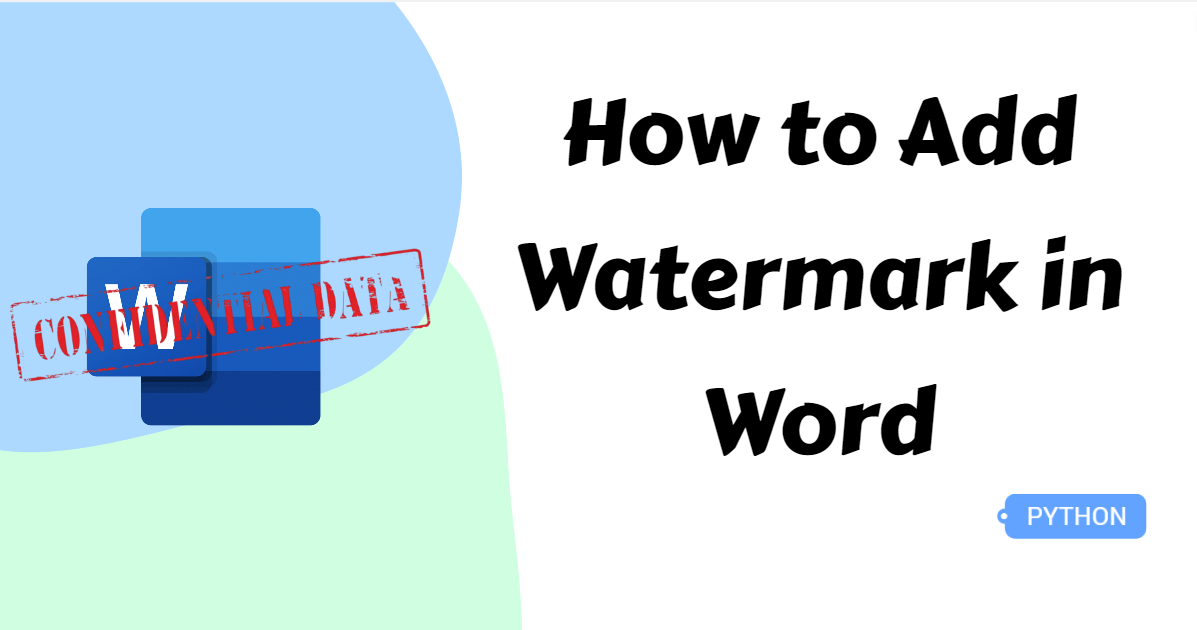
Whether you need to label internal documents with your company's logo or mark a Word document as confidential, adding watermarks is an effective solution. Watermarks are semi-transparent text or images that sit behind the main content, allowing you to identify ownership without compromising readability. While MS Word offers a built-in watermark feature, dealing with multiple files can be troublesome since you need to open each document individually. Instead, this article will show you how to add a watermark in Word using Python, providing a more efficient method for handling multiple documents, along with a way to remove the watermark if needed.
Prepare for the Task
Speaking of adding a watermark to a Word document in Python, a Python library is needed. This blog recommends Spire.Doc for Python, a powerful Word control that enables developers to create, modify, and convert Word documents easily, including adding confidential watermarks, of course.
You can install Spire.Doc for Python from PyPI using the following pip command:
pip install Spire.Doc
How to Add a Watermark in Word: Text Watermark
Suppose you are fully prepared right now, let’s dive into the key point. Normally speaking, there are two types of watermarks in Word, that is text watermark and image watermark. Both types of watermarks help protect Word documents to a certain degree. People are free to choose the one that suits the content of the document.
In this section, we will first go through how to add a text watermark in Word documents.
Steps to add a text watermark in Word documents:
Import essential modules.
Create an instance of the Document class and use the Document.LoadFromFile() method to load a file from the disk.
Create a TextWatermark object.
Customize the text watermark’s format, including the text, font size, color, and layout with the TextWatermark.Text, TextWatermark.FontSize, TextWatermark.Color, TextWatermark.Layout properties.
Add the watermark to the Word document using the Document.Watermark property.
Save the resulting Word document to the file with the Document.SaveToFile() method and release the resources.
Here is an example of adding “DO NOT COPY” as a text watermark in Word documents:
from spire.doc import *
from spire.doc.common import *
# Create an instance of Document class
doc = Document()
# Load a Word document from the disk
doc.LoadFromFile("/sample.docx")
# Create a TextWatermark object
txtWatermark = TextWatermark()
# Set the format of the text watermark
txtWatermark.Text = "DO NOT COPY"
txtWatermark.FontSize = 65
txtWatermark.Color = Color.get_Red()
txtWatermark.Layout = WatermarkLayout.Diagonal
# Add the text watermark to the document
doc.Watermark = txtWatermark
# Save the resulting document to the disk
doc.SaveToFile("/TextWatermark.docx", FileFormat.Docx)
# Release resources
doc.Close()
How to Add a Watermark in Word: Image Watermark
As we talked about above, Microsoft Word allows users to apply both text and picture watermarks. Seamly, Spire.Doc for Python supports adding image watermarks through properties under the PictureWatermark class. This part will illustrate how to add an image watermark in Word documents with Python, along with steps and a code example.
Guide to add picture watermark on Word documents:
Instantiate a Document class.
Open the Word document to be modified from the file with the Document.LoadFromFile() method.
Create an object of the PictureWatermark class.
Specify the file path of the image watermark with the PictureWatermark.SetPicture() method.
Configure the format of the image watermark.
Add a watermark in Word documents using the Document.Watermark property.
Save the modified document as a new file by calling the Document.SaveToFile() method and release the resources.
Below is the code example of adding a confidential watermark in Word documents:
from spire.doc import *
from spire.doc.common import *
# Create an instance of the Document class
doc = Document()
# Load a Word document from the disk
doc.LoadFromFile("/sample.docx")
# Create a PictureWatermark object
picture = PictureWatermark()
# Configure the format of the picture watermark
picture.SetPicture("/confidential.png")
picture.Scaling = 100
picture.IsWashout = False
# Add the image watermark to the document
doc.Watermark = picture
# Save the modified document as a new Word file
doc.SaveToFile("/ImageWatermark.docx", FileFormat.Docx)
# Close the document
doc.Close()
How to Delete Watermark in Word Quickly
After going through adding text and image watermarks, you might find yourself needing to remove watermarks in some cases. For example, the data is no longer confidential, or you want to replace the current watermark with a new one.
We will guide you through how to delete watermarks in Word quickly with Python, saving you time and effort.
Steps to remove watermarks from Word documents:
Import needed modules.
Create an instance of the Document class.
Read a Word document with watermarks from the disk with the Document.LoadFromFile() method.
Delete watermarks from the Word document by setting the Document.Watermark property to None.
Write the Word document to files by calling the Document.SaveToFile() method.
Here is an example of deleting the image watermark from a Word document:
from spire.doc import *
from spire.doc.common import *
# Create an instance of the Document class
doc = Document()
# Load a Word document with a watermark
doc.LoadFromFile("/ImageWatermark.docx")
# Remove the watermark from the Word document
doc.Watermark = None
# Save the updated Word document
doc.SaveToFile("/RemoveWatermark.docx", FileFormat.Auto)
# Release the resource
doc.Close()
The Conclusion
This page mainly covers how to add a watermark in Word documents using Python. We explain adding text watermarks and image watermarks with step-by-step instructions and code examples. Moreover, the blog offers a solution for deleting watermarks in Word documents just in case. We hope you find it useful!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
