How to Connect Angular with Spring Boot for RESTful APIs

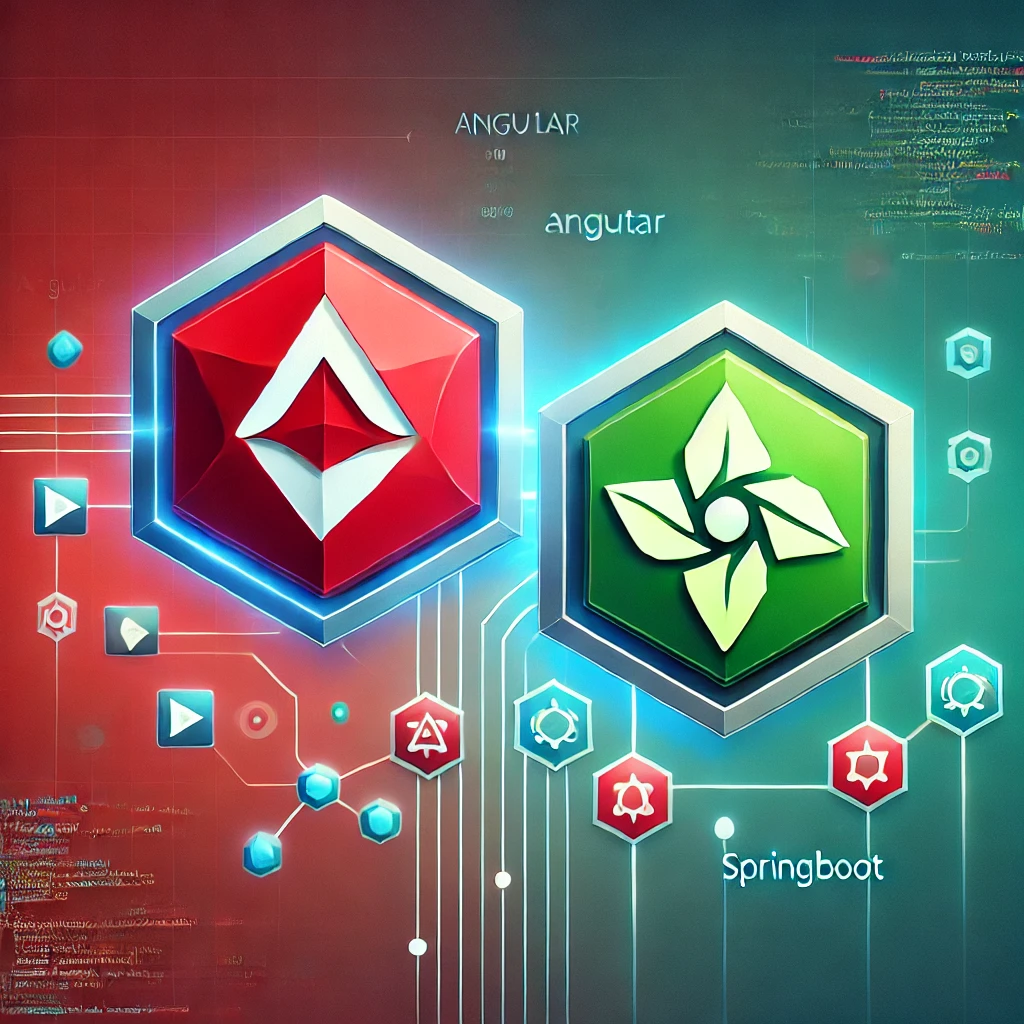
When building modern web applications, a common approach is to use Angular as the front-end framework and Spring Boot for the backend. Angular helps you create a dynamic user interface, while Spring Boot simplifies building robust and scalable RESTful APIs. In this tutorial, I’ll walk you through the steps to configure Angular and Spring Boot to work together seamlessly via REST API.
Prerequisites
Before we begin, make sure you have the following installed on your machine:
Java (JDK 11 or higher)
Node.js (for Angular)
Angular CLI
Spring Boot (via Spring Initializr or a Maven/Gradle project)
Step 1: Setting up the Spring Boot Backend
Spring Boot makes building REST APIs straightforward. Let’s start by creating a simple Spring Boot application that exposes a REST endpoint.
1.1. Create a Spring Boot Project
You can create a new Spring Boot project using Spring Initializr.
Open Spring Initializr.
Select the following:
Project: Maven Project
Language: Java
Spring Boot Version: 2.7.0+
Dependencies: Spring Web
Generate the project and unzip it.
1.2. Define the REST Controller
In your Spring Boot project, create a simple REST controller that will expose an API.
package com.example.demo;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GreetingController {
@GetMapping("/api/greeting")
public String greeting() {
return "Hello from Spring Boot!";
}
}
This REST endpoint returns a simple message when accessed. It will be called by the Angular application later.
1.3. Run the Spring Boot Application
Navigate to your project’s root folder and run the following command to start the Spring Boot server.
./mvnw spring-boot:run
The application will start on port 8080
by default. You can visit http://localhost:8080/api/greeting
to see the output from the API.
Step 2: Setting up the Angular Frontend
Now that our backend is up and running, let’s create an Angular project to connect with it.
2.1. Create a New Angular Project
Use the Angular CLI to create a new Angular project.
ng new angular-springboot-client
Navigate into the project directory:
cd angular-springboot-client
2.2. Install HttpClientModule
To interact with the backend API, Angular provides HttpClientModule
. Import it in your app.module.ts
.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http'; // Import this
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, HttpClientModule], // Add it here
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
2.3. Create a Service to Fetch Data
Now, create an Angular service that will call the Spring Boot API.
Generate a service using the Angular CLI:
ng generate service greeting
Then, update the service (greeting.service.ts
) to make an HTTP GET request to the Spring Boot API.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root',
})
export class GreetingService {
private apiUrl = 'http://localhost:8080/api/greeting';
constructor(private http: HttpClient) {}
getGreeting(): Observable<string> {
return this.http.get(this.apiUrl, { responseType: 'text' });
}
}
2.4. Display Data in the Angular Component
To display the data from the API, update the app.component.ts
and app.component.html
.
In app.component.ts
, inject the service and call the API.
import { Component, OnInit } from '@angular/core';
import { GreetingService } from './greeting.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent implements OnInit {
title = 'angular-springboot-client';
greeting: string;
constructor(private greetingService: GreetingService) {}
ngOnInit(): void {
this.greetingService.getGreeting().subscribe((response) => {
this.greeting = response;
});
}
}
In app.component.html
, display the greeting message.
<h1>{{ greeting }}</h1>
2.5. Run the Angular Application
To run your Angular app, use the following command:
ng serve
This will start the Angular app on http://localhost:4200
. Now, Angular will make a request to the Spring Boot API and display the message.
Step 3: Enabling Cross-Origin Requests
If you try to connect Angular and Spring Boot, you might face a CORS (Cross-Origin Resource Sharing) issue since they run on different ports. To solve this, you need to configure CORS in your Spring Boot backend.
Create a new class WebConfig.java
to handle the CORS configuration globally for your entire Spring Boot application.
3.1 Create the WebConfig
class
In the src/main/java/com/example/demo/config
folder (or an appropriate package), create a new Java class named WebConfig.java
:
package com.example.demo.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**") // Specify the API paths for CORS
.allowedOrigins("http://localhost:4200") // Allow the Angular app's URL
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS") // Allowed HTTP methods
.allowedHeaders("*") // Allow any headers
.allowCredentials(true) // Allow credentials (cookies, authorization headers, etc.)
.maxAge(3600); // Cache preflight response for 1 hour
}
}
3.2 Explanation
@Configuration
: This annotation makesWebConfig
a Spring configuration class.WebMvcConfigurer
: This interface allows you to customize the Spring MVC configuration.addCorsMappings
: This method configures CORS mappings.addMapping("/api/**")
: Defines the paths (in this case, any endpoint under/api/
) for which the CORS policy will apply.allowedOrigins("
http://localhost:4200
")
: Specifies the allowed origin, which is the Angular app running onlocalhost:4200
.allowedMethods(...)
: Specifies the HTTP methods that are allowed (e.g., GET, POST, etc.).allowedHeaders("*")
: Allows any headers in the request.allowCredentials(true)
: Allows cookies, authorization headers, etc.maxAge(3600)
: Specifies how long the browser should cache the CORS preflight response (3600 seconds = 1 hour).
Your Angular application should now be able to make API requests to the Spring Boot backend without running into CORS issues.
That’s it! You've successfully configured Angular and Spring Boot to communicate via REST API. This setup forms the foundation for building full-stack applications. You can now extend both your backend and frontend with more complex features such as authentication, database interactions, and more.
Happy coding!
Subscribe to my newsletter
Read articles from Uttam Mahata directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Uttam Mahata
Uttam Mahata
As an undergraduate student pursuing a Bachelor's degree in Computer Science and Technology at the Indian Institute of Engineering Science and Technology, Shibpur, I have developed a deep interest in data science, machine learning, and web development. I am actively seeking internship opportunities to gain hands-on experience and apply my skills in a formal, professional setting. Programming Languages: C/C++, Java, Python Web Development: HTML, CSS, Angular, JavaScript, TypeScript, PrimeNG, Bootstrap Technical Skills: Data Structures, Algorithms, Object-Oriented Programming, Data Science, MySQL, SpringBoot Version Control : Git Technical Interests: Data Science, Machine Learning, Web Development