Understanding Large Language Models: Tokenization and TF-IDF Vectorization

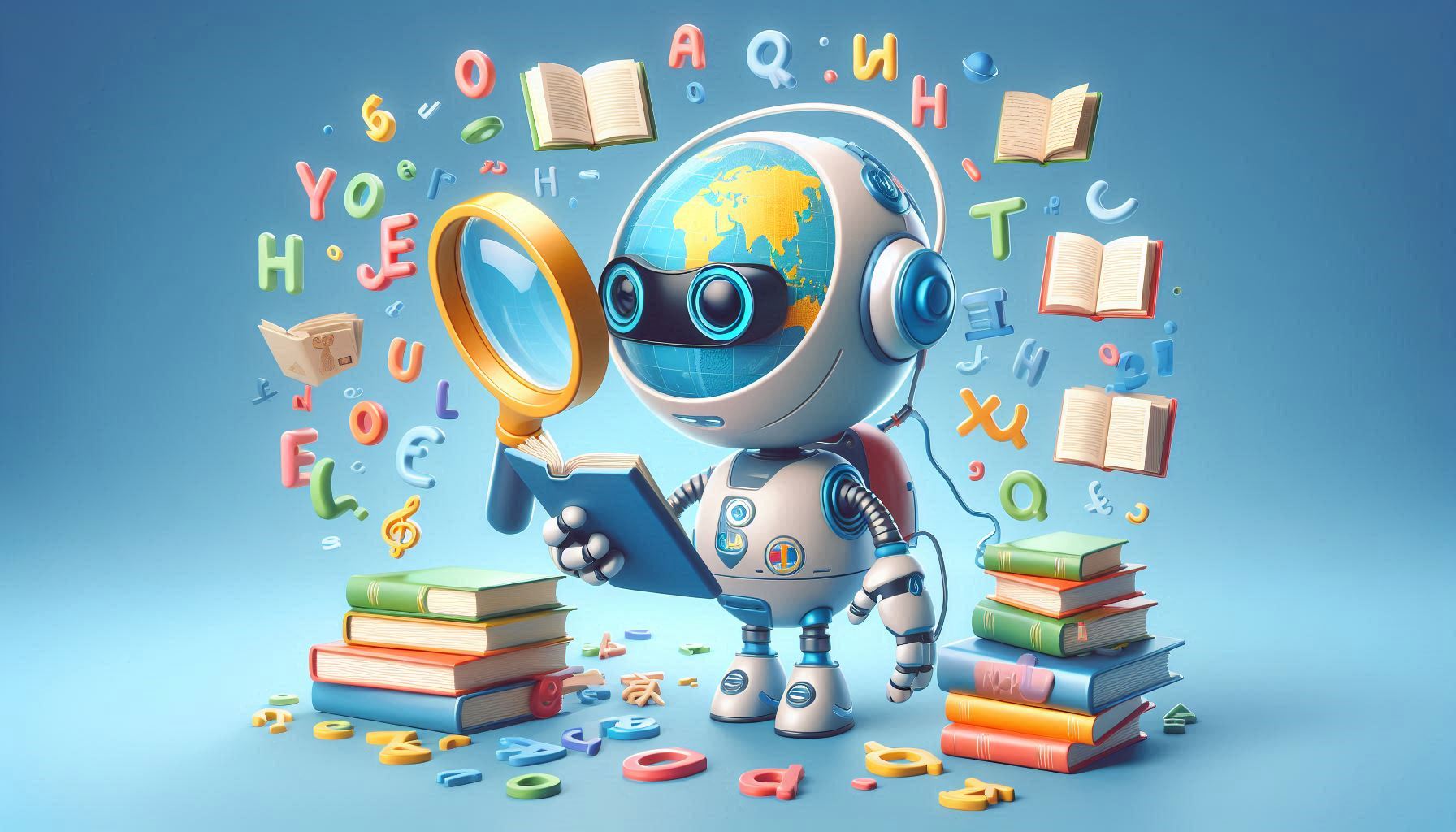
Large Language Models (LLMs) have become a cornerstone in natural language processing (NLP), enabling machines to understand, analyze, and generate human language. The power behind these models stems from the way they process and transform text data. In this blog post, we will delve into two key techniques used in preparing text for LLMs: Tokenization and TF-IDF Vectorization.
Tokenization: Breaking Text into Components
Tokenization is the process of converting text into smaller, more manageable units called tokens. These tokens are typically words or subwords, and this transformation is critical because machine learning models cannot process raw text. Instead, they require numerical representations of the text.
In Python, we can achieve tokenization using tools such as TensorFlow's Tokenizer
. Here's an overview of how it works:
Example Code Using TensorFlow's Tokenizer
:
from tensorflow.keras.preprocessing.text import Tokenizer
# Initialize the Tokenizer
tokenizer = Tokenizer()
# Fit the tokenizer on a list of words (training it on the text)
tokenizer.fit_on_texts(['a list with string of words'])
# Convert text to sequences (list of strings to list of integers)
sequence = tokenizer.texts_to_sequences(['a list with string of words'])
# View the index-word mapping
index_mapping = tokenizer.index_word
Key Points:
Tokenizer.fit
_on_texts()
: This function learns the word index from a list of words. It assigns a unique integer to each word in the vocabulary.texts_to_sequences()
: Once the tokenizer is trained, this function converts a list of words into sequences of integers.index_word
: This is a dictionary where each word from the vocabulary is mapped to an integer index.
By tokenizing text, we enable LLMs to process language more efficiently, paving the way for tasks like sentiment analysis, translation, and question answering.
TF-IDF Vectorization: Converting Text to Features
While tokenization breaks text into individual components, TF-IDF (Term Frequency-Inverse Document Frequency) Vectorization is a statistical method used to quantify the importance of words in a document relative to a collection of documents. This technique transforms text into a matrix of numerical features, making it ideal for machine learning algorithms.
Example Code Using TfidfVectorizer
from scikit-learn:
from sklearn.feature_extraction.text import TfidfVectorizer
# Initialize the TfidfVectorizer
tfidfvec = TfidfVectorizer(min_df=2, max_df=0.95, stop_words='english')
# Fit and transform the text data (here, 'df[colName]' is a column of text)
vectorized_data = tfidfvec.fit_transform(df[colName])
# Get the feature names (the unique words used as features)
features = tfidfvec.get_feature_names()
# Convert the transformed data into an array
data_array = vectorized_data.toarray()
# Create a DataFrame from the TF-IDF matrix
tfidf_df = pd.DataFrame(data_array, columns=features)
# Set the index of the DataFrame to the original text data
tfidf_df.index = df[colName]
Key Points:
TfidfVectorizer()
: This function converts a collection of text documents into a matrix of TF-IDF features. You can control how common or rare a word should be to include it as a feature usingmin_df
(minimum document frequency) andmax_df
(maximum document frequency).min_df=2
: Ignores terms that appear in fewer than two documents.max_df=0.95
: Removes words that appear too frequently (in more than 95% of documents), as they might not provide useful information.stop_words='english'
: Removes common English words (e.g., "the", "is") that do not add much meaning to the document.
fit_transform()
: This function learns the TF-IDF model and transforms the text data into the TF-IDF matrix.get_feature_names()
: After transforming the text, this function lists the unique words (features) that represent the documents.toarray()
: Converts the sparse matrix (output from the TF-IDF transformation) into a dense array for further analysis.pd.DataFrame()
: The transformed array can be easily visualized by converting it into a Pandas DataFrame, making it easier to understand which words correspond to which numerical features.
Why TF-IDF?
TF-IDF allows us to focus on the most important words in a document. It down-weights the words that appear frequently across many documents (because they are likely less informative) while giving higher importance to words that are unique to a particular document. This technique is commonly used for:
Text classification (e.g., spam detection)
Clustering (e.g., grouping similar documents together)
Information retrieval (e.g., search engines)
Conclusion
Both tokenization and TF-IDF vectorization are essential steps in preparing text data for use in large language models. Tokenization breaks down text into manageable units, while TF-IDF quantifies the importance of words across documents, helping machine learning models to understand the underlying patterns in language.
Together, these techniques enable LLMs to process text data more effectively, powering applications like chatbots, search engines, content generation, and beyond.
By combining these techniques with the powerful capabilities of LLMs, we unlock the potential for deeper, more nuanced text analysis and generation, making AI a valuable tool for understanding and interacting with human language.
With these foundational steps, data scientists and AI practitioners can continue to explore and optimize LLMs to create even more sophisticated and capable language-driven applications.
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.