Understanding Programs, Processes, and Threads
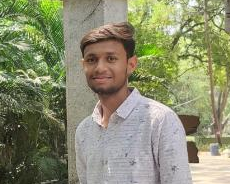
Programs, processes, and threads are fundamental concepts in computer science that often seem intertwined. While they are related, each has its distinct role in how a computer system operates. Let's break down what each means and how they interact.
Programs: The Blueprints
A program is essentially a set of instructions written in a programming language. It's like a recipe for a computer, outlining the steps needed to accomplish a specific task. These instructions are stored in a file on a disk. Programs can be compiled into executable files (like .exe files on Windows) or interpreted directly by the computer.
Programs can range from simple scripts that perform a single function to complex applications with millions of lines of code. They can be written in various programming languages like Python, Java, or C++. Each language has its syntax and rules, but the core idea remains the same: to provide the computer with a clear set of instructions to follow.
When a program runs, it becomes a process, which is an active instance of the program. This process can then create multiple threads to perform tasks concurrently, making the program more efficient. Understanding how programs, processes, and threads work together is crucial for optimizing performance and resource management in software development.
Processes: The Executors
A process is a running instance of a program. When you run a program, the operating system creates a process to execute its instructions. Each process has its own memory space, resources, and a unique identifier. Processes can be in various states: running, waiting, ready, blocked, or terminated.
How Processes Work
When a program starts, the operating system allocates memory and resources to it, creating a process. This process can perform tasks independently and is isolated from other processes. This isolation ensures that one process cannot directly interfere with another, providing stability and security.
Process States
Running: The process is actively executing instructions.
Waiting: The process is waiting for some event to occur (like I/O operations).
Ready: The process is prepared to run but is waiting for CPU time.
Blocked: The process cannot proceed until a specific condition is met.
Terminated: The process has completed its execution or has been stopped.
Inter-Process Communication (IPC)
While processes are isolated, they often need to communicate with each other. This is done through Inter-Process Communication (IPC) mechanisms like pipes, message queues, and shared memory. IPC allows processes to share data and synchronize their actions.
Process Scheduling
The operating system uses a scheduler to manage process execution. The scheduler decides which process runs at any given time, ensuring efficient use of the CPU. Different scheduling algorithms (like round-robin, priority-based) determine the order and duration of process execution.
Understanding processes is crucial for optimizing performance and ensuring smooth operation of computer systems.
Threads: The Multitaskers
A thread is a lightweight unit of execution within a process. A process can have multiple threads, each capable of executing independently. Think of a thread as a subtask within a larger process. Threads share the same memory space as the process they belong to, which makes communication between them more efficient.
Threads are useful for performing multiple tasks simultaneously within a single process. For example, a web browser can use separate threads to load web pages, run scripts, and handle user input at the same time. This parallelism improves the performance and responsiveness of applications.
Threads can be in different states, similar to processes:
Running: The thread is actively executing instructions.
Waiting: The thread is waiting for some event to occur (like I/O operations).
Ready: The thread is prepared to run but is waiting for CPU time.
Blocked: The thread cannot proceed until a specific condition is met.
Terminated: The thread has completed its execution or has been stopped.
Thread management and scheduling are handled by the operating system, which ensures that threads are executed efficiently and fairly. Understanding threads is essential for developing high-performance, responsive applications.
Key Differences
Feature | Process | Thread |
Creation time | Longer | Shorter |
Resource consumption | More | Less |
Isolation | Isolated (each process has its own memory space) | Not fully isolated (share memory with other threads within the same process) |
Communication | Slower (requires inter-process communication) | Faster (share memory) |
Context switching | Slower | Faster |
Blocking | If one thread is blocked, the entire process is blocked | If one thread is blocked, other threads within the process can continue |
Why Use Threads?
Threads offer several advantages:
Improved performance: By breaking down a task into smaller, parallel threads, multiple operations can be executed simultaneously.
Responsiveness: Threads can make applications more responsive by allowing certain tasks to continue while others wait for input or resources.
Efficient resource utilization: Threads can share resources within a process, reducing overhead.
In Conclusion
Programs provide the instructions, processes execute those instructions, and threads allow for parallel execution within a process. Understanding these concepts is crucial for anyone working with computer systems or programming. By effectively utilizing programs, processes, and threads, developers can create more efficient and responsive applications.
Subscribe to my newsletter
Read articles from Darsh Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
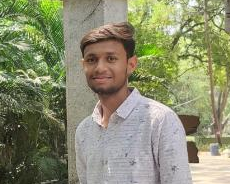
Darsh Patel
Darsh Patel
I'm a final-year student at PES University, specializing in Full-Stack Development and AI/ML. With hands-on experience in building web applications and working on machine learning models, I'm passionate to use it in real world. My academic journey has been a mixture of technical learning and practical application, where I've honed my skills in development, data analysis and artificial intelligence. When I’m not coding, you can find me exploring new trends, tinkering with projects, or sharing some insights here or maybe reading a newspaper.