SetTimeout and SetInterval
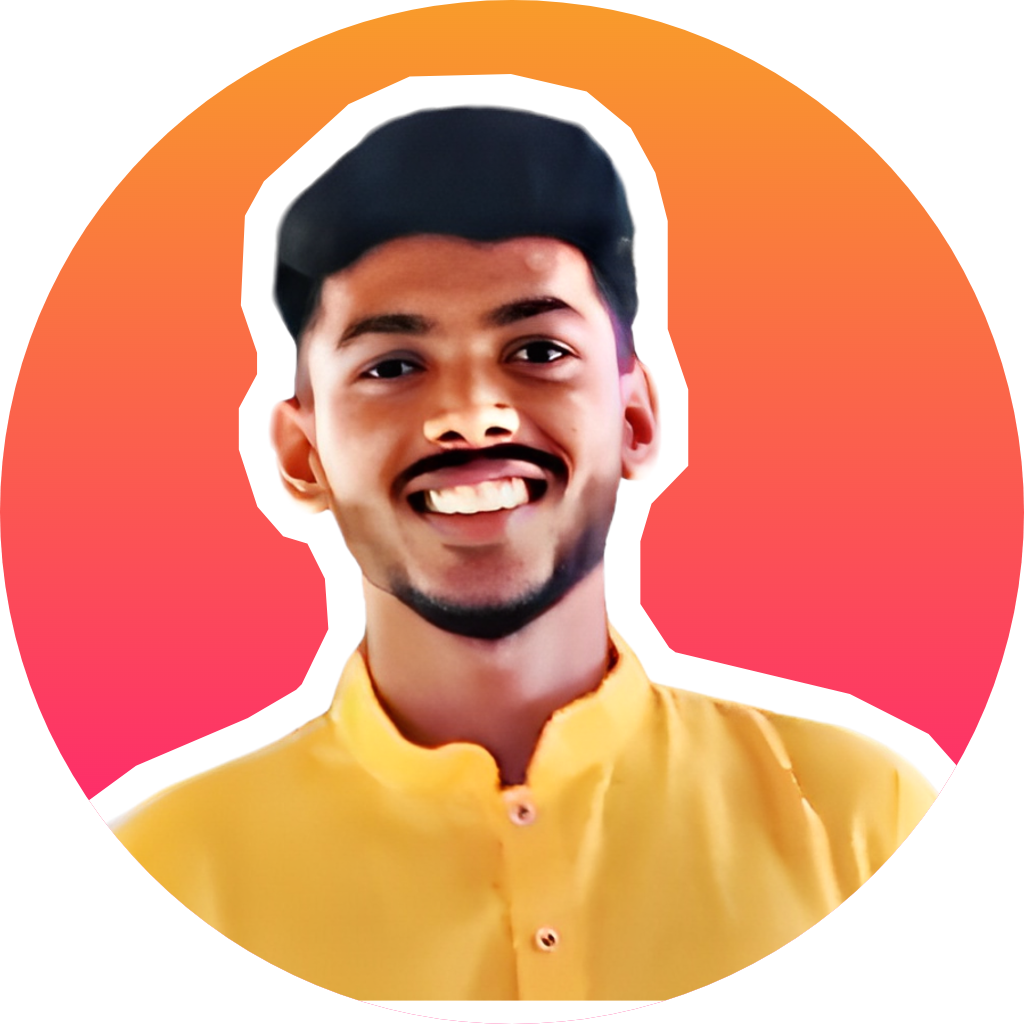
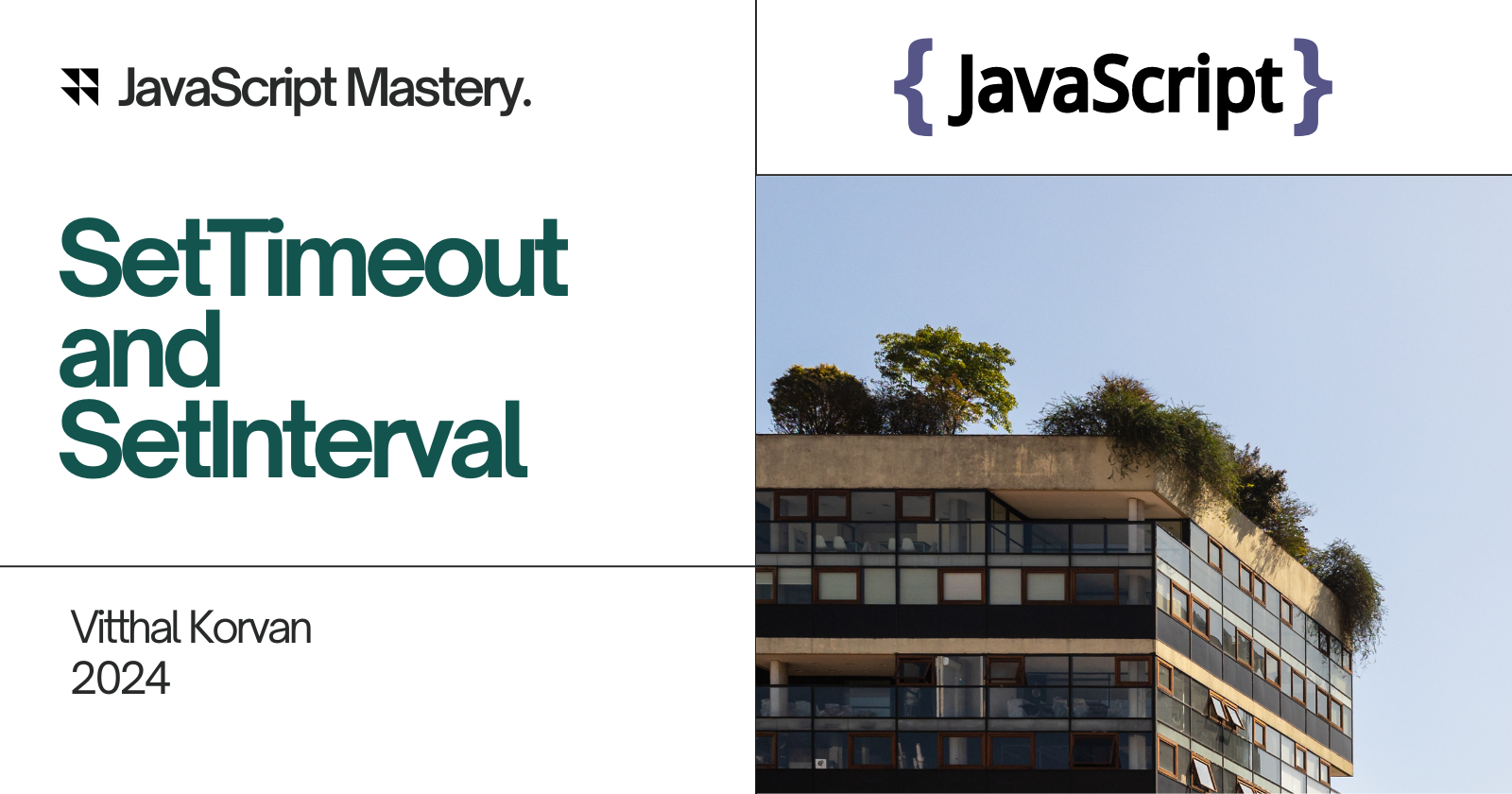
SetTimeout
Definition
setTimeout()
is a built-in function that allows you to execute a piece of code (a callback function) after a specified delay. This delay is measured in milliseconds (1000 milliseconds = 1 second). It schedules the function to be executed once, not repeatedly.
Syntax
//Syntax:
setTimeout(function, delay, arg1, arg2, ...);
function: A function to execute after the delay.
delay: Time (in milliseconds) to wait before executing the function.
arg1, arg2, ...: Optional. Parameters passed to the function when it is executed.
Example 1
const time = ()=>{
console.log('Hello!');
}
setTimeout(time,1000)
Example 2 - Sync vs Async
const id2 = setTimeout(() => {
console.log("Timeout 2");
}, 0);
console.log("Timeout 2", id2);
console.log("Script Starts");
const id = setTimeout(() => {
console.log("Timeout 1");
}, 1000);
console.log("Timeout 1", id);
for (let i = 0; i < 100; i++) {
console.log("inside for");
}
console.log("script end");
//Output:
//Timeout 2 3
//Script Starts
//Timeout 1 4
//100
//inside for
//script end
//Timeout 2
//Timeout 1
clearTimeout
Purpose
clearTimeout
is used to cancel a timeout that was previously set using setTimeout
. If you call clearTimeout
on a valid timeout ID before the timeout period is over, the function scheduled by setTimeout
will not execute.
Syntax
clearTimeout(timeoutID);
- timeoutID: The ID returned by the
setTimeout
function when you created the timer.
Example
let timeoutID = setTimeout(() => {
console.log("This will never happen.");
}, 5000);
// Cancel the timeout before it triggers
clearTimeout(timeoutID);
In this case, the timeout is cleared using clearTimeout
, so the function will never execute.
Combining setTimeout and clearTimeout
A common use case is to schedule a timeout but provide the user or program with the ability to cancel it before it triggers.
Example
function delayedMessage() {
console.log("This message is delayed.");
}
// Schedule the message to be shown after 3 seconds
let timeoutID = setTimeout(delayedMessage, 3000);
// Simulate a cancellation of the timeout after 1 second
setTimeout(() => {
clearTimeout(timeoutID);
console.log("Timeout was cleared before it triggered.");
}, 1000);
In this example, the first setTimeout
schedules the delayedMessage
to run after 3 seconds. However, another setTimeout
cancels it after 1 second, so the message is never logged.
Anonymous Functions
You can also use anonymous functions in setTimeout
:
setTimeout(function() {
console.log("This is an anonymous function executed after 2 seconds.");
}, 2000);
Timing Precision
The minimum delay for setTimeout
is not guaranteed to be exactly as specified. The delay is influenced by the event loop and browser environment. In most browsers, the minimum delay is around 4ms, even if 0
or a very low value is passed.
Recursive setTimeout
A common technique to create a recurring timer (like setInterval
) is to call setTimeout
recursively:
function repeatMessage() {
console.log("Repeating every 2 seconds");
setTimeout(repeatMessage, 2000);
}
setTimeout(repeatMessage, 2000);
Here, instead of using setInterval
, we create a loop where setTimeout
calls itself after each execution.
setInterval in JavaScript
The setInterval
method is used to repeatedly execute a function or piece of code at a fixed time interval (in milliseconds) until it is explicitly stopped using clearInterval
.
Syntax
let intervalID = setInterval(function, delay, arg1, arg2, ...);
function: The function to execute every time the interval occurs.
delay: The time (in milliseconds) between successive function calls. If the delay is omitted, it defaults to
0
, but this is almost never used since a 0ms interval results in high CPU usage.arg1, arg2, ...: Optional. Any additional parameters that should be passed to the function when it's executed.
Return Value
- The
setInterval
method returns a unique interval ID (a number), which is used to clear (stop) the interval later usingclearInterval(intervalID)
.
Example
function greet() {
console.log("Hello, world!");
}
// Executes greet() every 2 seconds (2000 milliseconds)
let intervalID = setInterval(greet, 2000);
In this example, the greet
function will execute every 2 seconds. This will continue until the interval is stopped using clearInterval
.
Using Parameters in setInterval
You can pass arguments to the function being executed at each interval.
function greet(name) {
console.log(`Hello, ${name}!`);
}
// Executes greet("Vitthal") every 3 seconds
let intervalID = setInterval(greet, 3000, "Vitthal");
Here, "Vitthal"
is passed to the greet
function every 3 seconds, resulting in Hello, Vitthal!
being logged repeatedly.
Stopping the Interval with clearInterval
Purpose
To stop an interval, use clearInterval(intervalID)
where intervalID
is the value returned by setInterval
.
Example
let count = 0
const interval = setInterval(()=>{
count += 2
console.log(count);
//Stop the interval if count is 10
if (count === 10) clearInterval(interval);
},1000)
In this case, the current time is printed every second, but the interval is cleared after 5 seconds, stopping the repeated execution.
Common Use Cases of setInterval
Real-time clocks: Displaying the current time on the web page by updating it every second.
Periodic Data Fetching: Polling a server every few seconds or minutes to fetch new data (e.g., live data updates).
Animations: Creating simple animations that update at regular intervals.
Game Loops: Running game logic at regular intervals.
Recursion vs. setInterval
You can achieve a similar effect to setInterval
using setTimeout
with recursion (repeatedly calling setTimeout
from within itself). The advantage of using setTimeout
for periodic tasks is better control over the timing of each iteration, particularly if the function takes variable time to execute.
Example Using Recursive setTimeout
function repeatTask() {
console.log("This message repeats every 2 seconds");
setTimeout(repeatTask, 2000); // Recursive call
}
setTimeout(repeatTask, 2000);
This approach ensures that the next call only happens after the current execution completes, which is more precise if the task being executed takes an unpredictable amount of time.
Important Notes
Minimum Delay Limitations: Modern browsers have a minimum delay limit of 4ms for
setInterval
andsetTimeout
. Even if you specify a delay of0ms
, the actual interval will be around 4ms or more. This is to avoid performance issues.Inaccuracy of
setInterval
:setInterval
schedules function calls at fixed intervals, but the actual execution might be delayed due to other tasks running on the event loop or system resource availability. This can cause the intervals to "drift."If you need accurate timing, it's often better to use
setTimeout
recursively, since it can account for the time taken by the previous function execution.
Combining setInterval with DOM Manipulation
A very common use of setInterval
is to update the DOM at regular intervals, for example, creating a countdown timer or updating a progress bar.
Example: Countdown Timer
let countdown = 10;
let countdownInterval = setInterval(() => {
if (countdown > 0) {
console.log(`Time left: ${countdown} seconds`);
countdown--;
} else {
clearInterval(countdownInterval);
console.log("Countdown finished!");
}
}, 1000);
In this example, the setInterval
function decrements the countdown every second and stops when it reaches 0
.
Using setInterval
with Anonymous Functions
You can directly pass an anonymous function to setInterval
if the task is simple and doesn't need to be reused elsewhere.
setInterval(() => {
console.log("This will run every 2 seconds");
}, 2000);
Subscribe to my newsletter
Read articles from Vitthal Korvan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
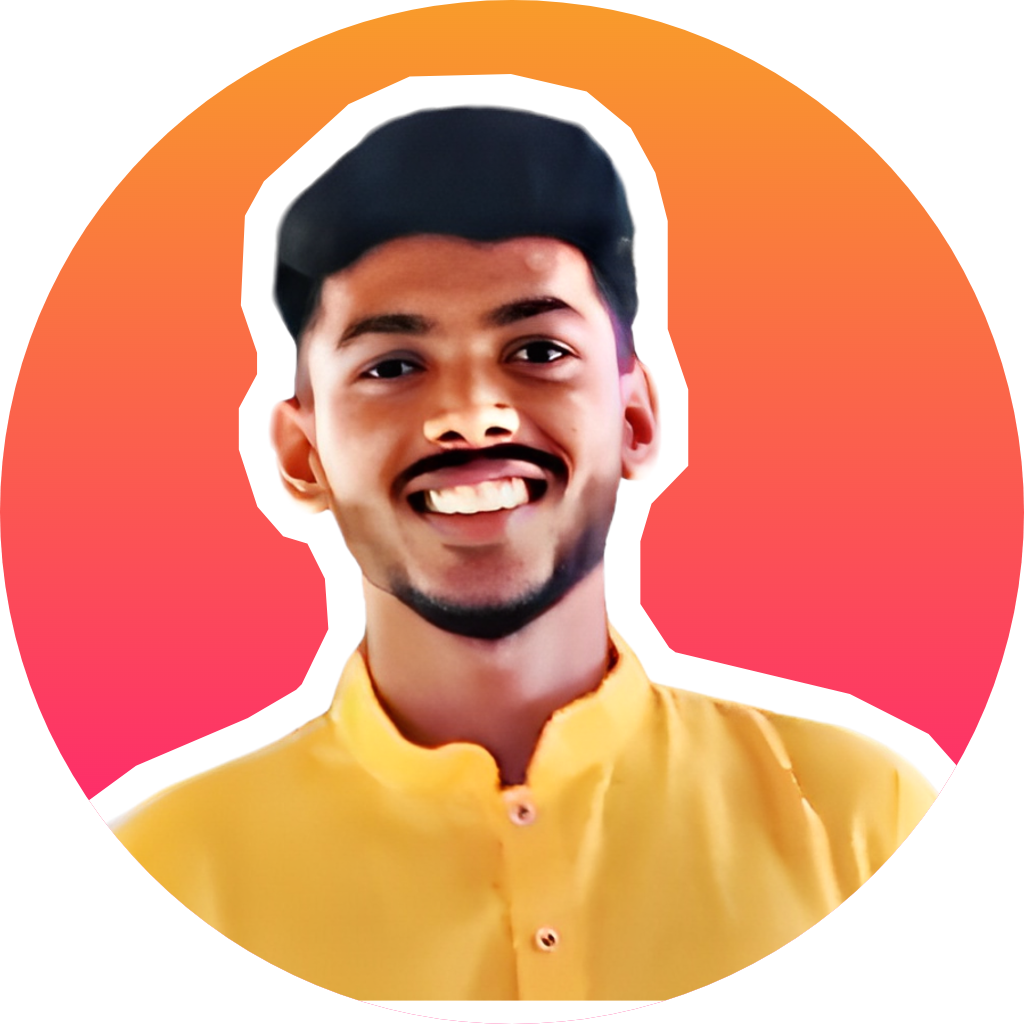
Vitthal Korvan
Vitthal Korvan
๐ Hello, World! I'm Vitthal Korvan ๐ As a passionate front-end web developer, I transform digital landscapes into captivating experiences. you'll find me exploring the intersection of technology and art, sipping on a cup of coffee, or contributing to the open-source community. Life is an adventure, and I bring that spirit to everything I do.