When Calling Functions Goes Wrong: Unpacking the 'z is not defined' Error in JavaScript

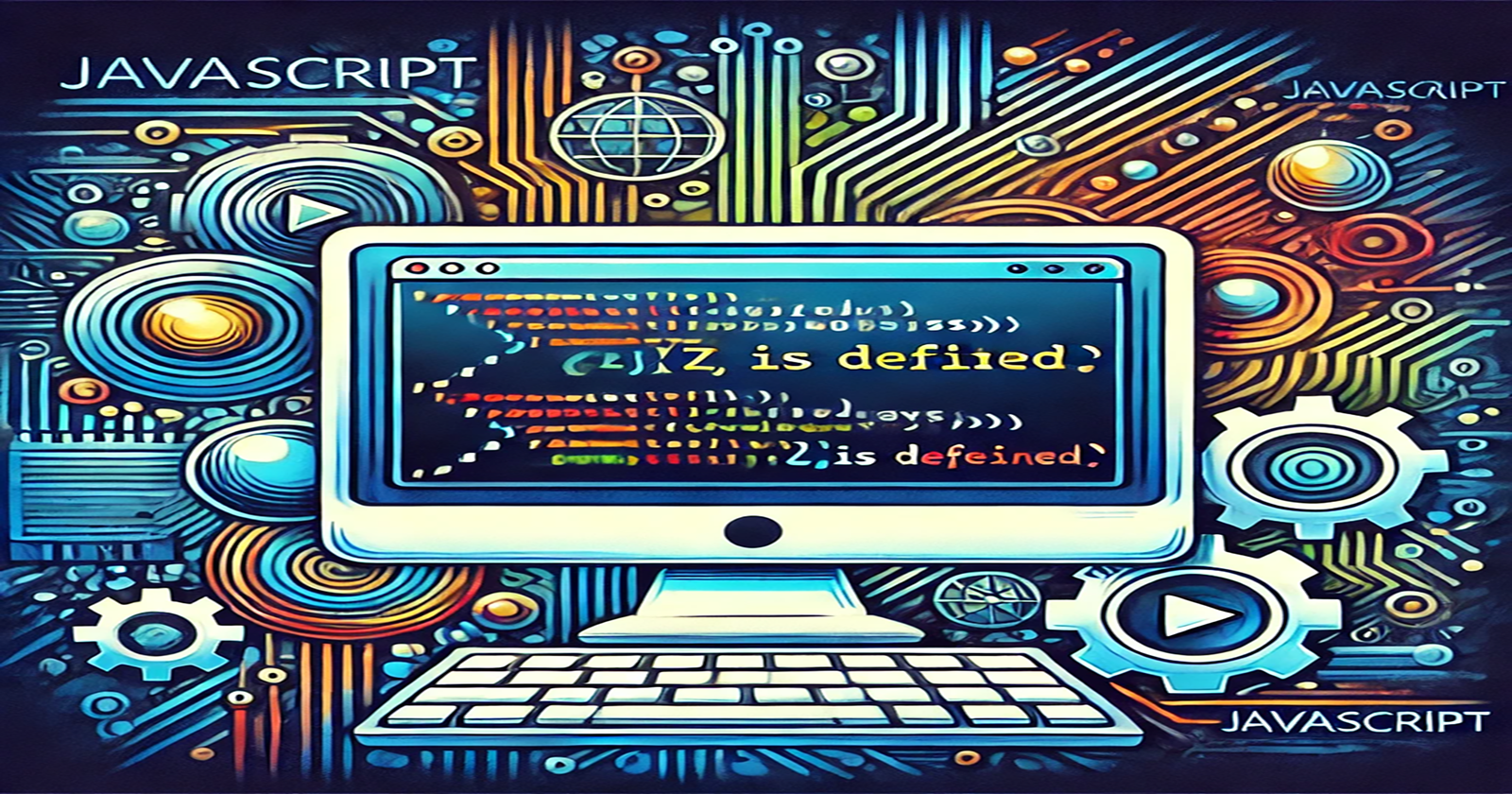
Here this led me to an error🤔🤔🤔
function x(l) {
console.log("X");
z();
}
x(function z() {
console.log("Y");
});
Output:
X index.js:3
Uncaught ReferenceError: z is not defined
at x (index.js:3:3)
at index.js:6:1
I encountered an error while passing functions in JavaScript, which prompted me to revisit the concepts of parameters and arguments. This experience led me to better understand how arguments are stored in parameters and how they can be invoked within functions. By dissecting the error, I gained clarity on the relationship between functions and their parameters, enhancing my grasp of this fundamental concept in JavaScript.
I will show you the correct code and how this was rectified meanwhile lets start from scratch.
Introduction to Parameters and Arguments
Definition:
Parameters are variables listed as part of a function's definition. They act as placeholders for the values (or arguments) that will be passed to the function when it is called.
Arguments are the actual values that you pass to the function when invoking it.
function add(a, b) { // here the `a` and `b` are parameters
}
add(5, 3); // invoking add(); here 5, and 3 are arguments
- Example: In the function declaration
function add(a, b)
,a
andb
are parameters. When you calladd(5, 3)
, the numbers5
and3
are the arguments.
As we all know, In JavaScript, functions are considered first-class citizens, which means they can be treated like any other value. This allows us to assign functions to variables, pass them as arguments to other functions, return them from functions, and even create them dynamically.
Now getting back to the code I’ve added in the top which led me to an error, I have passed a function z()
as an argument to function x()
while invoking (calling) it(function x()
).
Where do you think the potential threat/mistake was that lead to
'z is not defined'
You are right!
So that you don’t have to scroll up and down to see the code let me paste the code here too
function x(l) {
console.log("X");
z();
}
x(function z() {
console.log("Y");
});
Here, the parameter in function x(l
) which is l
that act as a placeholder to the argument passed while involking funciton x()
which is
function z() { console.log("Y"); }
We understand that the function z()
is stored in parameter l
so it was supposed to be called l()
instead of z()
.
I hope this was simple and clear. Now let paste the rectified code:
function x(l) {
console.log("X");
l(); // instead of z()! you know why?
}
x(function z() {
console.log("Y");
});
Output:
X
Y
Explanation:
Here, the function z()
is defined inline and passed as an argument to x()
. When x()
is called, it logs "X" and then executes l()
, which calls the function z()
and it logs “Y“.
Thank you for reading!
I welcome any feedback or critiques for improvisation. For more insights and resources, feel free to visit my GitHub.
For a deeper dive ⬇️
Reference: https://en.wikipedia.org/wiki/Parameter_(computer_programming)#Parameters_and_arguments
Subscribe to my newsletter
Read articles from Pradeep Thapa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pradeep Thapa
Pradeep Thapa
Hi, I'm Pradeep Thapa, a web developer with a passion for frontend design and digital solutions. With a background in teaching and a love for continuous learning, I specialize in React, JavaScript, HTML, CSS, and Tailwind CSS. Always eager to grow, I enjoy turning ideas into functional, user-friendly web experiences.