Strings in JavaScript
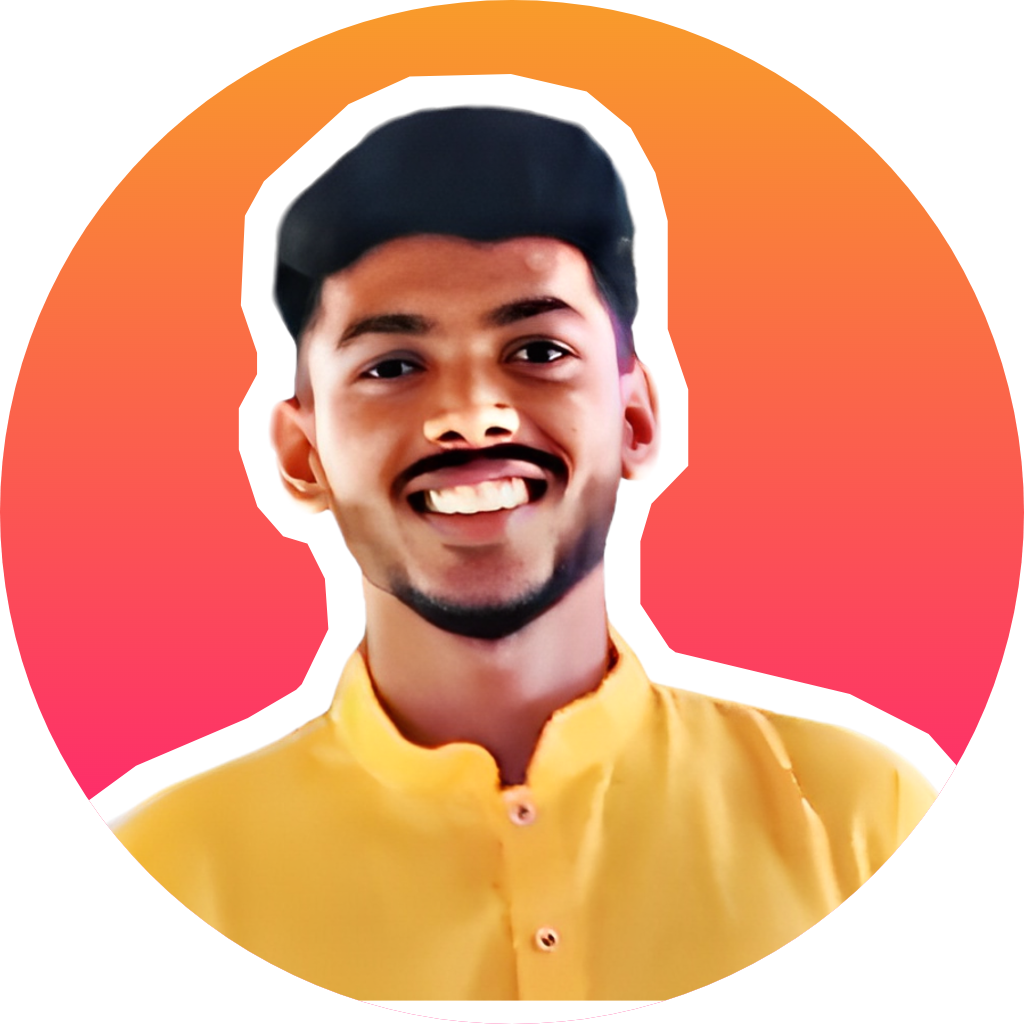
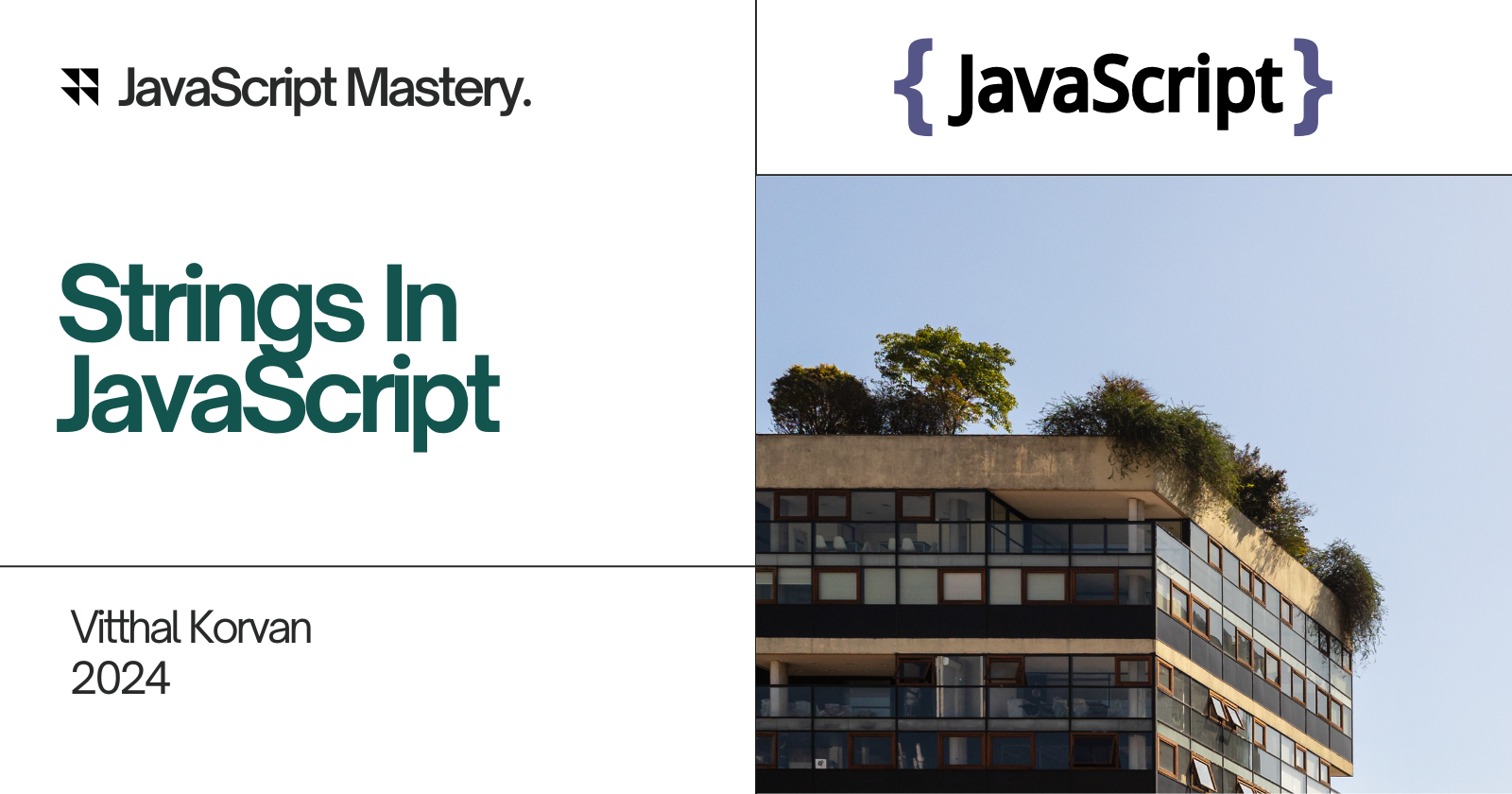
What is String?
A string in programming is a sequence of characters (letters, numbers, symbols, and spaces) typically used to represent text. Strings are often enclosed in quotation marks (" " or ' ').
String have some in build methods which can used in manipulation of string.
String Methods:-
Length Property
It is used to check the length of the string.
let fname = 'Priya'
console.log(fname.length); //5
CharAt()
The charAt() method in JavaScript returns the character at a specified index in a string.
let str = "Hello, World!";
console.log(str.charAt(0)); // H
concat()
The concat() method in JavaScript is used to join two or more strings together. It does not change the original strings but returns a new concatenated string.
let str1 = "Hello";
let str2 = "World";
let str3 = "!";
console.log(str1.concat(", ", str2, str3)); // "Hello, World!"
Inculdes()
Checks if the string contains a substring (returns true or false).
//string.includes('str-elem', start-index)
let sentence = "The quick brown fox jumps over the lazy dog.";
onsole.log(sentence.includes("quick")); // true
console.log(sentence.includes("Quick")); // false
console.log(sentence.includes("lazy", 30)); // true
IndexOf()
Returns the index of the first occurrence of a substring.
let sentence = "The quick brown fox jumps over the lazy dog.";
console.log(sentence.indexOf("quick")); // 4
Slice(startIndex, endIndex)
Similar to substring() but supports negative indices to count from the end.
let sentence = "The quick brown fox jumps over the lazy dog.";
console.log(sentence.slice(4, 9)); // "quick"
Split(separator, liimit)
Splits the string into an array at the specified separator.
let sentence = "The quick brown fox jumps over the lazy dog.";
console.log(sentence.split(" "));
ToLowerCase()
Converts the string to lowercase.
let sentence = "The Quick Brown Fox.";
console.log(sentence.toLowerCase()); // "the quick brown fox."
ToUpperCase()
Converts the string to uppercase.
let sentence = "The Quick Brown Fox.";
console.log(sentence.toUpperCase()); // "THE QUICK BROWN FOX."
Trim()
Removes leading and trailing whitespace.
let greeting = " Hello, World! ";
console.log(greeting.trim()); // "Hello, World!"
Replace(searchValue, newValue)
Replaces occurrences of a substring with another substring.
let sentence = "The quick brown fox jumps over the lazy dog.";
console.log(sentence.replace("fox", "cat"));
//The quick brown cat jumps over the lazy dog.
padStart() and padEnd()
It will give padding at start or at the end.
const originalString = "hello";
const paddedString = originalString.padStart(10, "Hey! "); // // "-----hello"
const paddedString2 = originalString.padEnd(12, " Shweta"); // "hello-----"
Repeat()
Repeats the string count times.
const originalString = "hello";
const repeatedString = originalString.repeat(3); // "hellohellohello"
console.log(repeatedString);
StartsWith()
Checks if the string starts with the specified prefix (returns true or false).
const originalString = "hello world";
const startsWithHello = originalString.startsWith("hello"); // true
EndsWith()
Checks if the string ends with the specified suffix (returns true or false).
const originalString = "hello world";
const endsWithWorld = originalString.endsWith("world"); // true
CharCodeAt()
Returns the Unicode value of the character at the specified index.
let str = "Hello";
console.log(str.charCodeAt(1)); // 101 (Unicode for 'e')
Subscribe to my newsletter
Read articles from Vitthal Korvan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
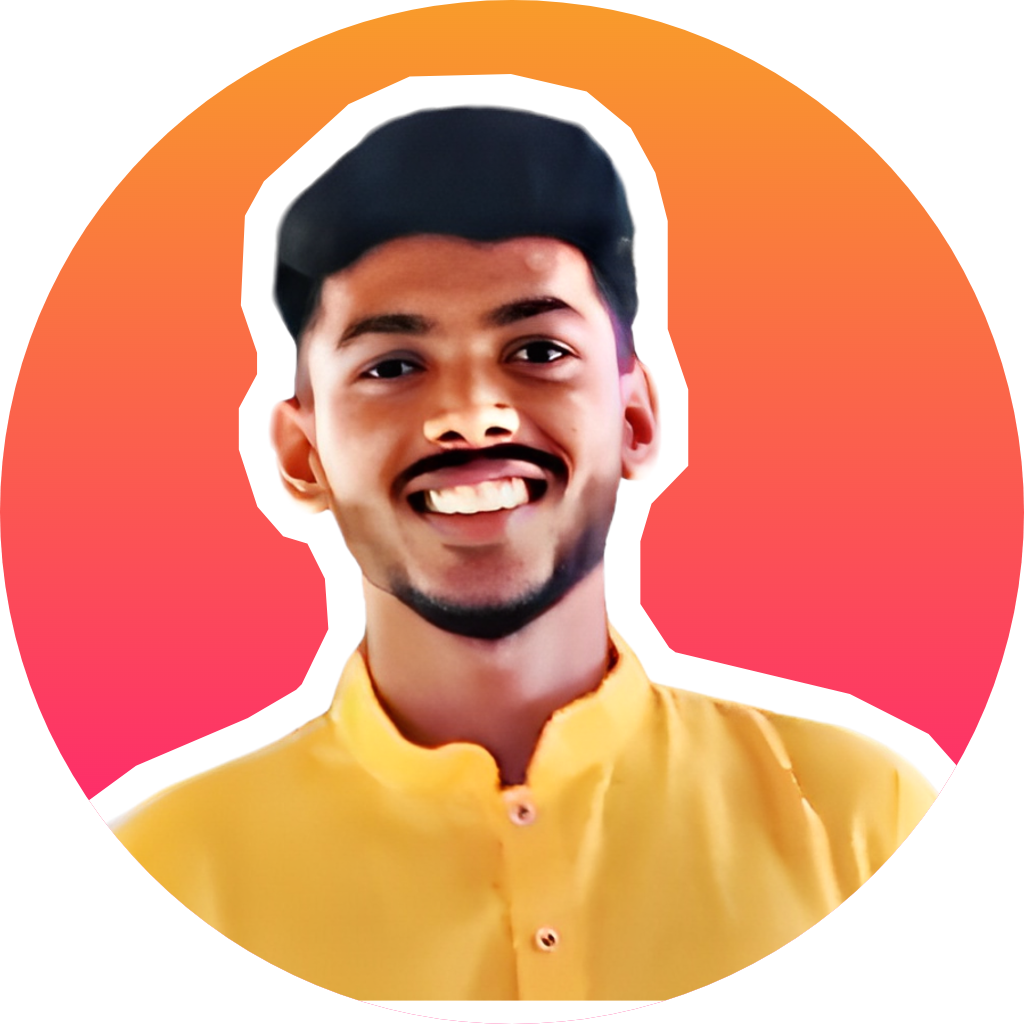
Vitthal Korvan
Vitthal Korvan
๐ Hello, World! I'm Vitthal Korvan ๐ As a passionate front-end web developer, I transform digital landscapes into captivating experiences. you'll find me exploring the intersection of technology and art, sipping on a cup of coffee, or contributing to the open-source community. Life is an adventure, and I bring that spirit to everything I do.