Essential Interview Questions
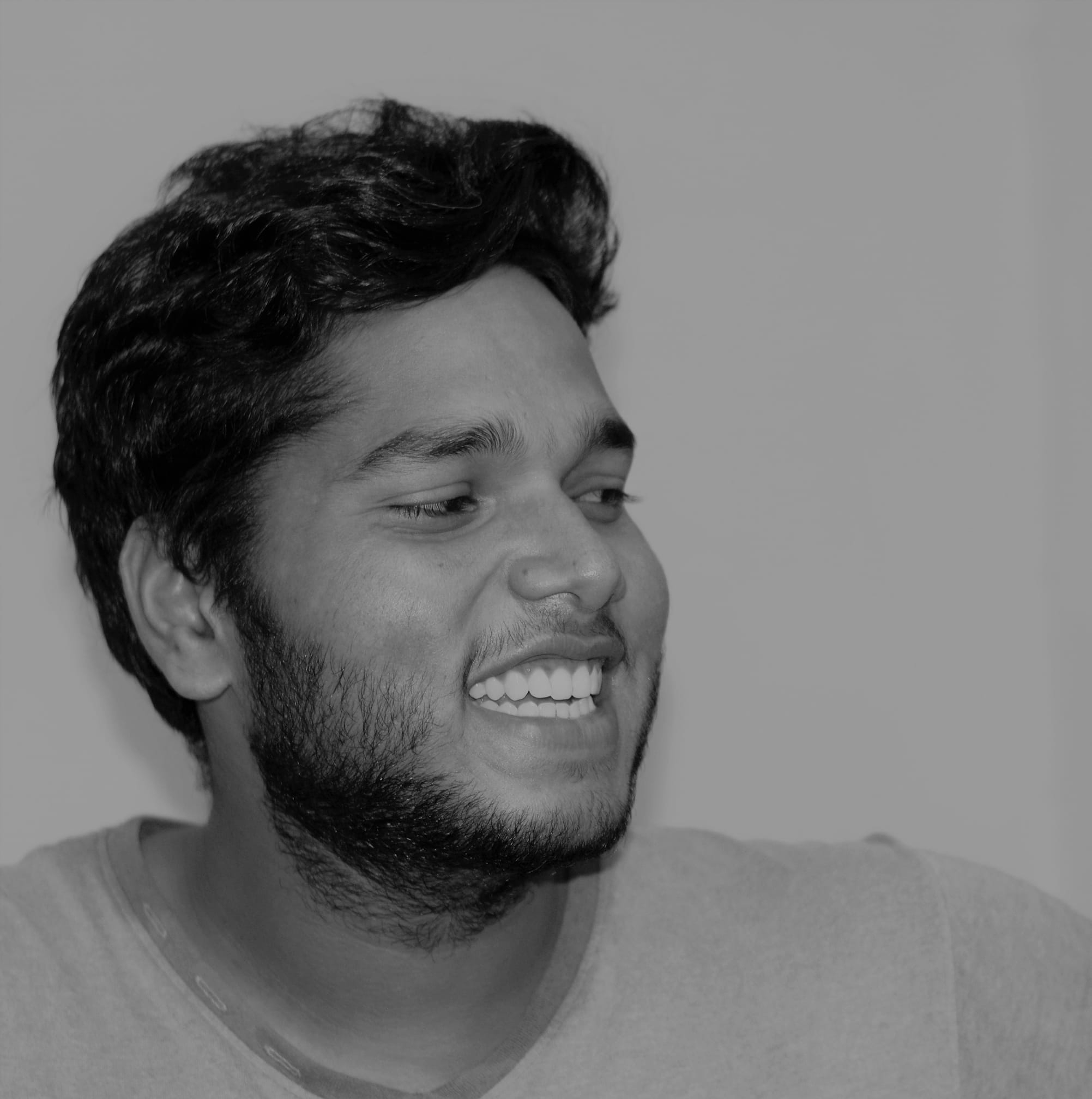
Table of contents
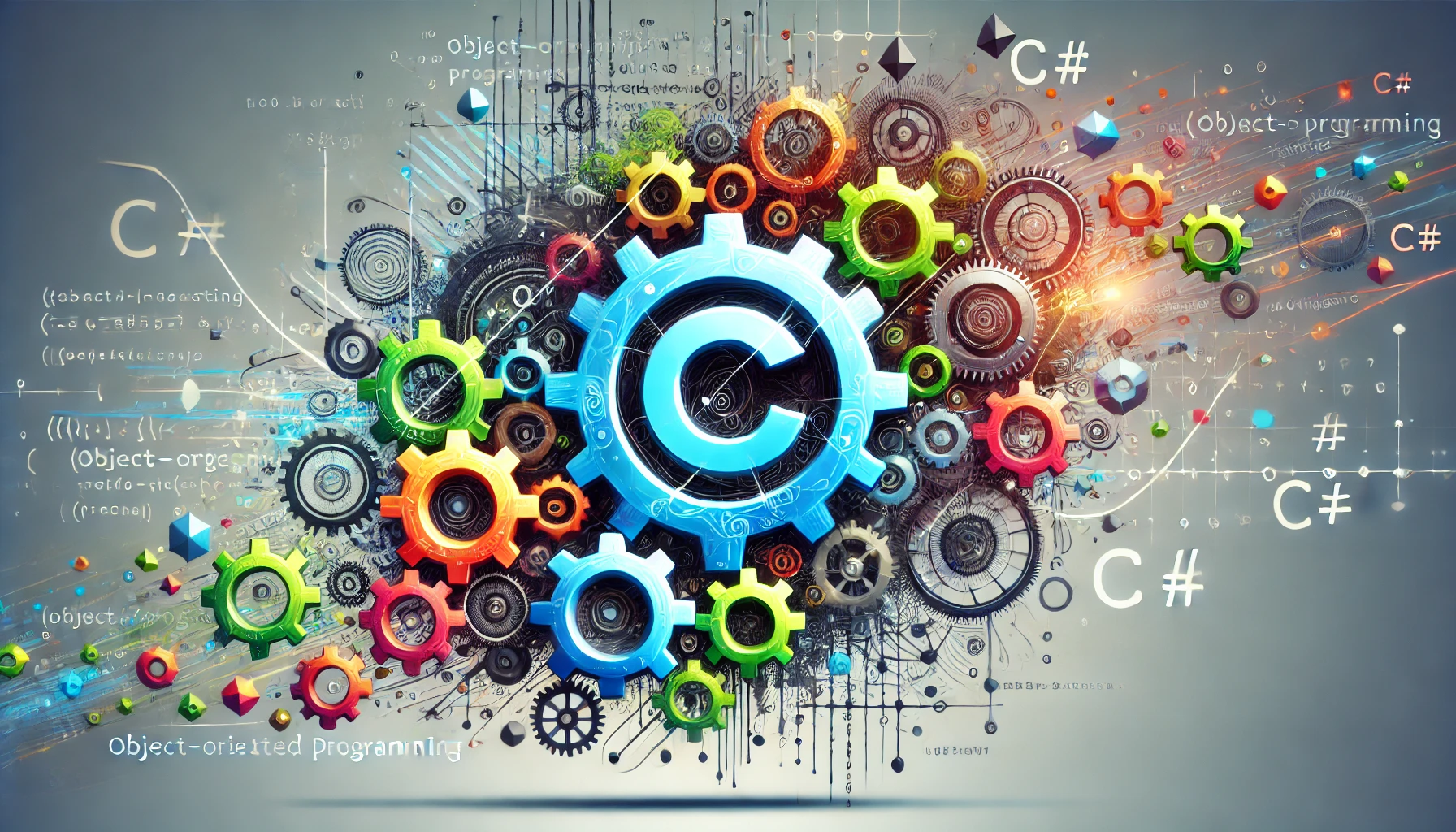
Preparing for a .NET developer interview? This guide compiles essential questions and detailed answers on C# Object-Oriented Programming (OOP), design patterns, SOLID principles, Dependency Injection, Entity Framework, and more. Whether you're brushing up on the basics or delving into intermediate concepts, this resource will help you confidently navigate common interview topics and demonstrate your proficiency in C# and .NET technologies.
What are the four pillars of Object-Oriented Programming (OOP)? Explain each of them.
Answer:
The four pillars of OOP are:
Encapsulation: Bundling data and methods that operate on the data within a class, restricting direct access to some of the object's components. Achieved using access modifiers like
private
,protected
, andpublic
.Abstraction: Hiding complex implementation details and showing only the essential features of an object. Implemented using abstract classes and interfaces.
Inheritance: Allowing a class to inherit fields and methods from another class. Promotes code reusability and establishes a hierarchical relationship. In C#, a class can inherit from one base class and implement multiple interfaces.
Polymorphism: Enabling objects to be treated as instances of their parent class rather than their actual class. Achieved through method overloading (compile-time polymorphism) and method overriding (runtime polymorphism).
What is the difference between method overloading and method overriding in C#?
Answer:
Method Overloading: Defining multiple methods with the same name but different parameters within the same class. It is a form of compile-time polymorphism.
public void Print(int number) { /* ... */ } public void Print(string text) { /* ... */ }
Method Overriding: Allowing a derived class to provide a specific implementation of a method already defined in its base class. The base method must be marked with
virtual
, and the overriding method usesoverride
. It is a form of runtime polymorphism.public class BaseClass { public virtual void Display() { /* ... */ } } public class DerivedClass : BaseClass { public override void Display() { /* ... */ } }
Explain what an abstract class is in C# and when you would use it.
Answer:
An abstract class cannot be instantiated and may contain abstract methods with no implementation. It's used when you want to provide a common base class with default behavior while forcing derived classes to implement specific methods.
public abstract class Animal { public abstract void MakeSound(); } public class Dog : Animal { public override void MakeSound() { /* Bark */ } }
What is an interface in C#, and how does it differ from an abstract class?
Answer:
An interface defines a contract with methods and properties but no implementation. Classes or structs that implement the interface must provide the implementation.
Differences:
Interfaces cannot contain implementation (prior to C# 8.0), while abstract classes can.
A class can implement multiple interfaces but inherit only from one class.
Interfaces cannot have fields, whereas abstract classes can.
public interface IMovable
{
void Move();
}
public class Vehicle : IMovable
{
public void Move() { /* Implementation */ }
}
Does C# support multiple inheritance? If not, how can you achieve similar functionality?
Answer:
C# does not support multiple inheritance of classes to prevent complexity and ambiguity. However, similar functionality can be achieved through interfaces.
public interface IA { /* ... */ } public interface IB { /* ... */ } public class C : IA, IB { /* ... */ }
What are the SOLID principles in C#? Briefly explain each.
Answer:
Single Responsibility Principle (SRP): A class should have only one reason to change.
Open/Closed Principle (OCP): Classes should be open for extension but closed for modification.
Liskov Substitution Principle (LSP): Subclasses should be substitutable for their base classes.
Interface Segregation Principle (ISP): No client should be forced to depend on methods it does not use.
Dependency Inversion Principle (DIP): Depend on abstractions, not on concrete implementations.
What is Dependency Injection (DI), and how is it implemented in C#?
Answer:
DI is a design pattern that allows removing hard-coded dependencies, making it possible to change them at runtime or compile time. It's implemented by injecting dependencies through constructors, properties, or methods.
public class Service { private readonly IRepository _repository; public Service(IRepository repository) { _repository = repository; } }
What is an ORM, and what are the benefits of using one like Entity Framework?
Answer:
An Object-Relational Mapper (ORM) maps objects in code to database tables. Benefits include:
Reduces boilerplate SQL code.
Enables LINQ queries.
Handles data mapping and change tracking.
Simplifies database operations.
Compare Entity Framework and Dapper.
Answer:
Entity Framework: Full-featured ORM, handles complex scenarios, includes change tracking, and is easier for rapid development.
Dapper: Micro-ORM, focuses on performance, requires manual SQL queries, and is lightweight.
Explain the Repository Pattern and its benefits.
Answer:
The Repository Pattern abstracts data access, providing a central place for data interactions. Benefits include:
Encapsulates data access logic.
Improves testability.
Promotes loose coupling.
public interface ICustomerRepository
{
void Add(Customer customer);
Customer GetById(int id);
}
What is CQRS, and how would you implement it in a C# application?
Answer:
Command Query Responsibility Segregation (CQRS) separates read and write operations. Implementation involves:
Commands: For write operations.
Queries: For read operations.
Handlers: Processing commands and queries.
public class CreateOrderCommand { /* ... */ }
public class GetOrderQuery { /* ... */ }
What is a Design Pattern, and how does it differ from an Architecture Pattern and a Style?
Answer:
Design Pattern: Reusable solution to common software design problems (e.g., Singleton).
Architecture Pattern: High-level structural organization of software systems (e.g., MVC).
Style: Coding conventions and guidelines (e.g., naming conventions).
Explain the Singleton Design Pattern and how to implement it in C#.
Answer:
Ensures a class has only one instance and provides a global point of access.
public sealed class Singleton { private static readonly Singleton _instance = new Singleton(); private Singleton() { } public static Singleton Instance => _instance; }
What is the Factory Design Pattern, and when would you use it?
Answer:
The Factory Pattern creates objects without specifying the exact class. Use it when:
The creation process is complex.
You need to encapsulate object creation.
public interface IProduct { /* ... */ }
public class ConcreteProduct : IProduct { /* ... */ }
public class ProductFactory
{
public static IProduct CreateProduct() => new ConcreteProduct();
}
What is the Dependency Inversion Principle, and how does it relate to Dependency Injection?
Answer:
Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules; both should depend on abstractions.
Relation to DI: DI is a technique to implement DIP by injecting dependencies rather than creating them within the class.
Explain the difference between Aggregation, Composition, and Association.
Answer:
Association: General relationship between classes.
Aggregation: Weak relationship; the child can exist independently of the parent.
Composition: Strong relationship; the child cannot exist without the parent.
// Aggregation
class Engine { /* ... */ }
class Car { public Engine Engine { get; set; } }
// Composition
class House
{
private Room _room = new Room();
}
What is Domain-Driven Design (DDD), and what are its main components?
Answer:
DDD focuses on modeling software based on the domain. Main components:
Entities
Value Objects
Aggregates and Aggregate Roots
Repositories
Services
Explain what an Aggregate Root is in DDD.
Answer:
An Aggregate Root is the main entity that ensures the integrity of the aggregate by controlling access to its components. Other objects within the aggregate are only accessible via the root.
What is the purpose of the Open/Closed Principle, and how can it be implemented in C#?
Answer:
Purpose: To allow software entities to be extensible without modifying existing code.
Implementation: Use interfaces and abstract classes to extend behavior.
public interface IShape
{
double Area();
}
public class Circle : IShape { /* ... */ }
public class Rectangle : IShape { /* ... */ }
Describe the Decorator Pattern and provide a C# example.
Answer:
The Decorator Pattern adds behavior to objects dynamically.
public interface INotifier { void Send(string message); } public class Notifier : INotifier { /* ... */ } public abstract class NotifierDecorator : INotifier { protected INotifier _notifier; public NotifierDecorator(INotifier notifier) { _notifier = notifier; } public abstract void Send(string message); } public class SMSNotifier : NotifierDecorator { public SMSNotifier(INotifier notifier) : base(notifier) { } public override void Send(string message) { _notifier.Send(message); // Send SMS } }
What is a Data Transfer Object (DTO), and why is it used?
Answer:
A DTO is an object that carries data between processes. It's used to:
Simplify data transfer.
Decouple layers.
Improve performance by transferring only necessary data.
What is the difference between an Abstract Class and an Interface in terms of use cases?
Answer:
Abstract Class: Use when classes share common behavior and state. Allows method implementations and fields.
Interface: Use to define a contract for classes without implementation. Supports multiple inheritance.
Explain the concept of Inversion of Control (IoC) and its benefits.
Answer:
IoC is a principle where the control of objects or portions of a program is transferred to a container or framework. Benefits include:
Promotes loose coupling.
Enhances testability.
Improves maintainability.
What are wrong classification and wrong abstraction, and how can they impact a system?
Answer:
Wrong Classification: Misplacing responsibilities in classes, leading to violations of SRP.
Wrong Abstraction: Incorrectly generalizing behavior, causing rigidity or fragility.
Impact:
Makes the system hard to understand and maintain.
Increases the likelihood of bugs.
What is the Liskov Substitution Principle, and can you provide an example in C#?
Answer:
Definition: Objects of a superclass should be replaceable with objects of a subclass without affecting the correctness.
Example:
public class Bird { public virtual void Fly() { /* ... */ } } public class Penguin : Bird { public override void Fly() { throw new NotImplementedException(); } }
Substituting
Penguin
forBird
violates LSP sincePenguin
cannot fly.
Subscribe to my newsletter
Read articles from Arpit Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
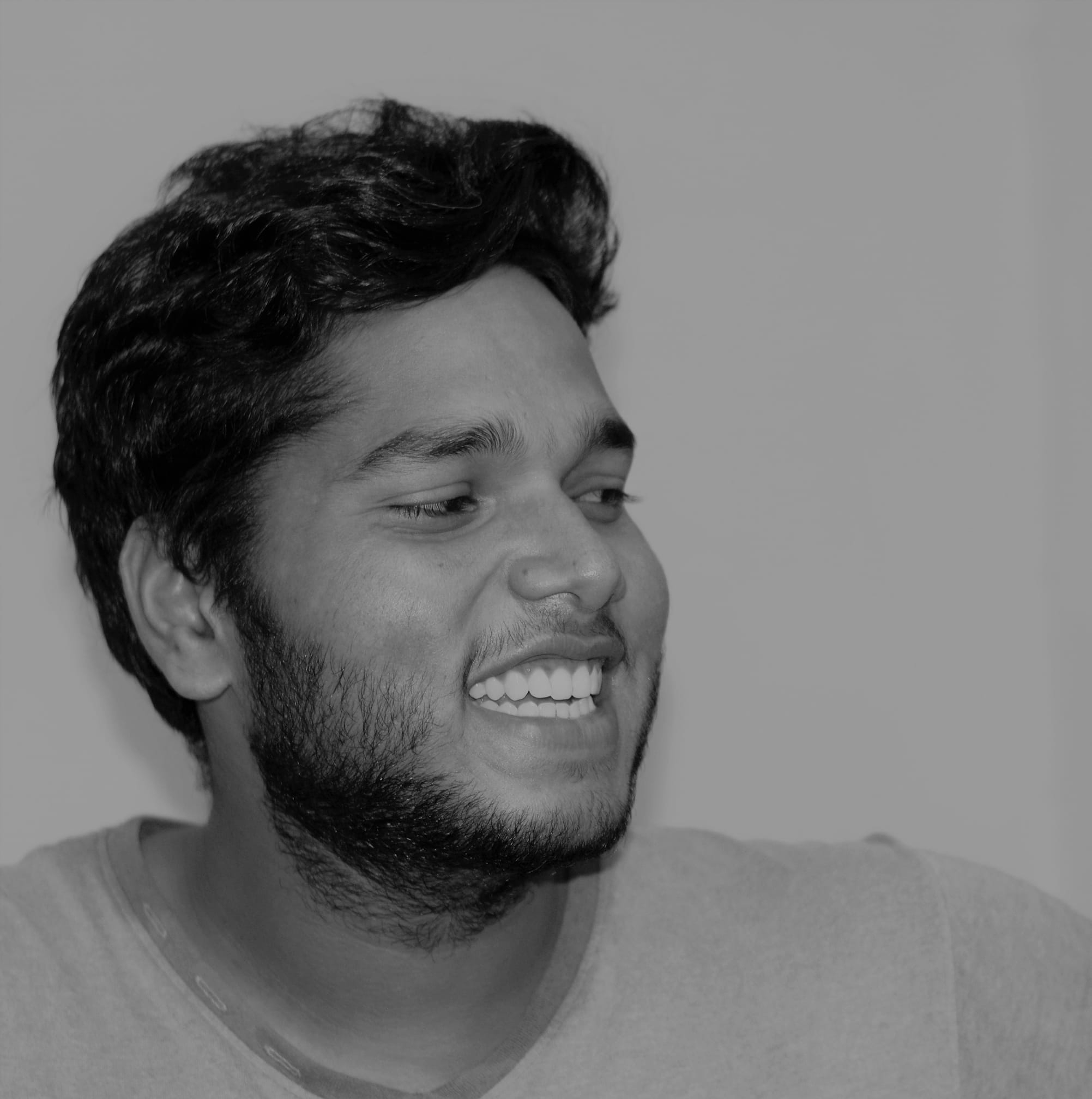
Arpit Dwivedi
Arpit Dwivedi
Crafting code with a finesse that bridges the digital divide! As a Full Stack Developer, I’ve conjured innovations at industry front-runners like Infosys and Kline & Company. With a suite boasting C#, ASP.NET CORE, Angular, and the complex dance of microservices, I’m not just programming – I’m telling stories. Beyond raw code, I'm a tech translator, dishing out insights as an avid writer, leading the charge in the open-source world, and floating on the Azure cloud. From corporates to intense tech programs, my journey’s been anything but ordinary. Curiosity? It's not just a sidekick; it's my co-pilot. Eagerly scanning the horizon for the next tech marvel. 🚀