Top 20 Essential Angular Interview Questions and Answers
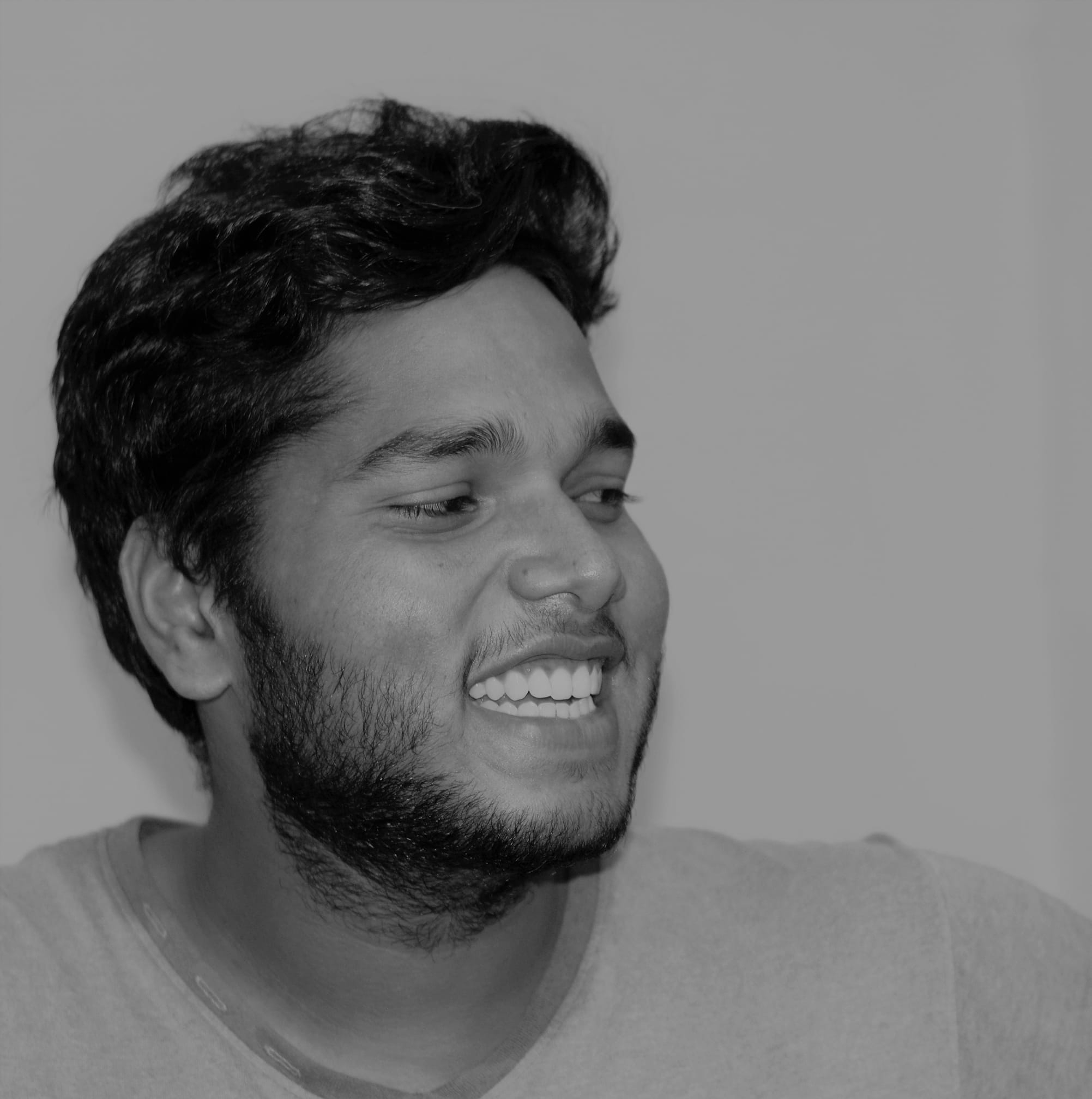
Table of contents
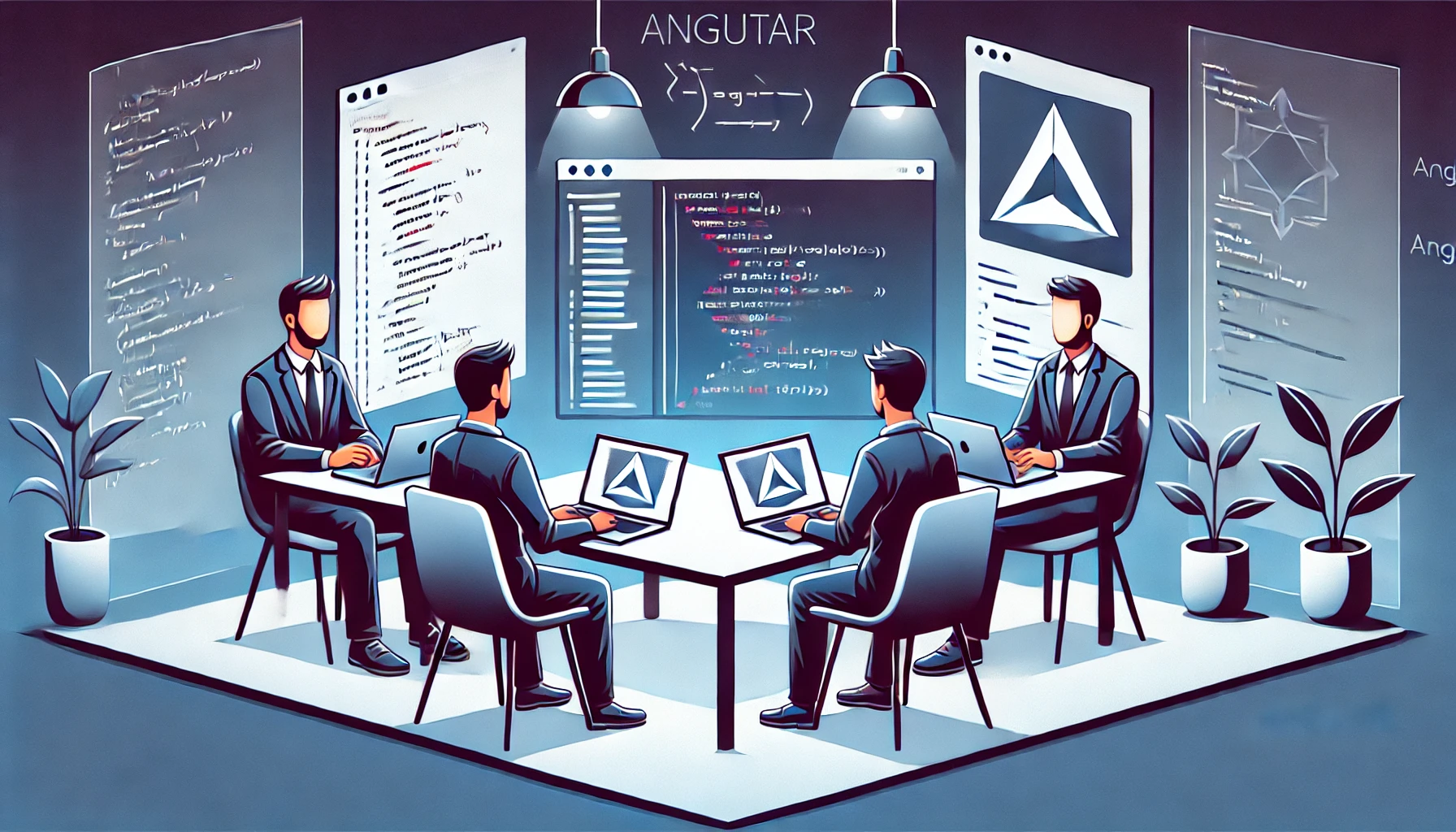
Preparing for an Angular interview can be daunting, especially if you're new to the framework. This article compiles the top 20 essential Angular interview questions and answers to help you understand the core concepts. From components and modules to data binding and dependency injection, this guide will equip you with the knowledge you need to impress your interviewers and secure that developer position.
What is Angular, and why is it used?
Answer:
Angular is a TypeScript-based open-source web application framework developed by Google for building dynamic single-page applications (SPAs).
Explanation:
Angular provides a comprehensive solution for developing rich client applications with features like two-way data binding, dependency injection, and component-based architecture. It simplifies development by handling common tasks and promotes clean code practices.
Explain the concept of Components in Angular.
Answer:
Components are the fundamental building blocks of Angular applications, controlling a part of the user interface through their templates and logic.
Explanation:
Each component in Angular encapsulates its own HTML template, CSS styles, and TypeScript logic. This modularity enhances code reusability and maintainability, allowing developers to build complex UIs by composing components.
What are Modules in Angular, and what is their purpose?
Answer:
Modules are logical containers in Angular that group related components, directives, pipes, and services to organize an application.
Explanation:
An Angular module, defined with
@NgModule
, helps manage the application's structure by bundling functionality. The root module bootstraps the app, while feature modules encapsulate specific areas, improving code separation and scalability.What is Data Binding, and what are its types in Angular?
Answer:
Data binding is the mechanism in Angular that synchronizes data between the model and the view.
Explanation:
Angular supports four types of data binding:
Interpolation (
{{ }}
): Displays component properties in the template.Property Binding (
[ ]
): Binds component properties to element attributes.Event Binding (
( )
): Handles events raised from the view.Two-Way Binding (
[( )]
): Combines property and event binding usingngModel
for forms.
What are Directives in Angular, and how are they classified?
Answer:
Directives are classes that add behavior to elements in Angular applications, classified into components, structural directives, and attribute directives.
Explanation:
Components: Directives with a template.
Structural Directives (
*
): Alter the DOM layout by adding or removing elements (e.g.,*ngIf
,*ngFor
).Attribute Directives: Change the appearance or behavior of an element (e.g.,
ngClass
,ngStyle
).
Explain the concept of Services in Angular.
Answer:
Services are reusable classes that provide specific functionality and can be injected into components or other services.
Explanation:
By using dependency injection, services promote code modularity and testability. They handle tasks like fetching data, logging, or business logic, keeping components focused on the view.
What is Dependency Injection in Angular?
Answer:
Dependency Injection (DI) is a design pattern in Angular that allows a class to receive dependencies from external sources rather than creating them internally.
Explanation:
Angular's DI framework provides components and services with their required dependencies, improving code maintainability and reducing coupling. It uses decorators like
@Injectable
and the injector hierarchy for resolution.What is the purpose of the
ngOnInit
lifecycle hook in Angular?Answer:
ngOnInit
is a lifecycle hook called after Angular initializes all data-bound properties of a component.Explanation:
Implementing the
OnInit
interface,ngOnInit
is used for component initialization logic, such as fetching data or setting up subscriptions, ensuring that inputs are available.Explain the difference between Observables and Promises in Angular.
Answer:
Observables can handle multiple values over time and are cancellable, while Promises deal with a single future value and are not cancellable.
Explanation:
Observables, provided by RxJS, are used extensively in Angular for asynchronous operations like HTTP requests. They offer operators for complex data manipulation and are preferred over Promises for their flexibility.
What is Routing in Angular, and how does it work?
Answer:
Routing enables navigation between different views or components in an Angular application.
Explanation:
The Angular Router interprets URL paths and maps them to components using route configurations. It uses the
RouterModule
,Routes
array, and<router-outlet>
to display components based on the current URL.What is Ahead-of-Time (AOT) compilation in Angular?
Answer:
AOT compilation is the process of compiling Angular templates and components at build time instead of runtime.
Explanation:
AOT improves application performance by reducing the size of the compiled code, catching template errors early, and eliminating the need for the browser to compile the code, resulting in faster rendering.
What are Pipes in Angular, and how are they used?
Answer:
Pipes are simple functions that transform data in templates, allowing for formatting and display adjustments.
Explanation:
Angular provides built-in pipes like
DatePipe
,UpperCasePipe
, and allows custom pipes creation with the@Pipe
decorator. Pipes are used in templates with the|
symbol (e.g.,{{ value | currency }}
).Explain the difference between
constructor
andngOnInit
in Angular components.Answer:
The
constructor
is used for dependency injection, whilengOnInit
is a lifecycle hook for component initialization.Explanation:
The
constructor
should be lightweight, initializing services.ngOnInit
is called after the constructor and is ideal for complex initialization that requires component inputs to be set.What is Change Detection in Angular?
Answer:
Change Detection is the mechanism that updates the view when the model changes.
Explanation:
Angular's change detector monitors component state and updates the DOM accordingly. It can be optimized using change detection strategies like
Default
andOnPush
to improve performance.What are Angular Forms, and how do Template-driven and Reactive Forms differ?
Answer:
Angular Forms handle user input and validation, with two approaches: Template-driven and Reactive Forms.
Explanation:
Template-driven Forms: Use directives in the template, suitable for simple forms.
Reactive Forms: Use explicit form models in the component class, offering more control and better scalability for complex forms.
What is the purpose of
ng-content
in Angular?Answer:
ng-content
allows content projection, enabling components to display external content within their templates.Explanation:
By acting as a placeholder,
ng-content
enables developers to create reusable components that can render dynamic content provided by other components or templates.What are Promises, and how are they used in Angular?
Answer:
Promises represent a single asynchronous operation that completes in the future, returning a value or error.
Explanation:
While Angular primarily uses Observables, Promises can be used for simpler async tasks. They provide
then
andcatch
methods for handling success and errors.What is the purpose of
async
andawait
in Angular?Answer:
async
andawait
are used in TypeScript to write asynchronous code in a synchronous style.Explanation:
Marking a function as
async
allows the use ofawait
to pause execution until a Promise resolves, simplifying the handling of asynchronous operations without nested callbacks.Explain what Angular CLI is and its benefits.
Answer:
Angular CLI is a command-line tool that assists in creating, building, and managing Angular applications.
Explanation:
It streamlines development by automating tasks like project setup, code scaffolding, testing, and optimization, ensuring adherence to best practices and reducing configuration overhead.
What is Lazy Loading in Angular?
Answer:
Lazy Loading is a technique that delays the loading of feature modules until they are needed.
Explanation:
By configuring routes to load modules on demand, Lazy Loading reduces the initial bundle size, improving application load times and performance, especially beneficial in large applications.
Subscribe to my newsletter
Read articles from Arpit Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
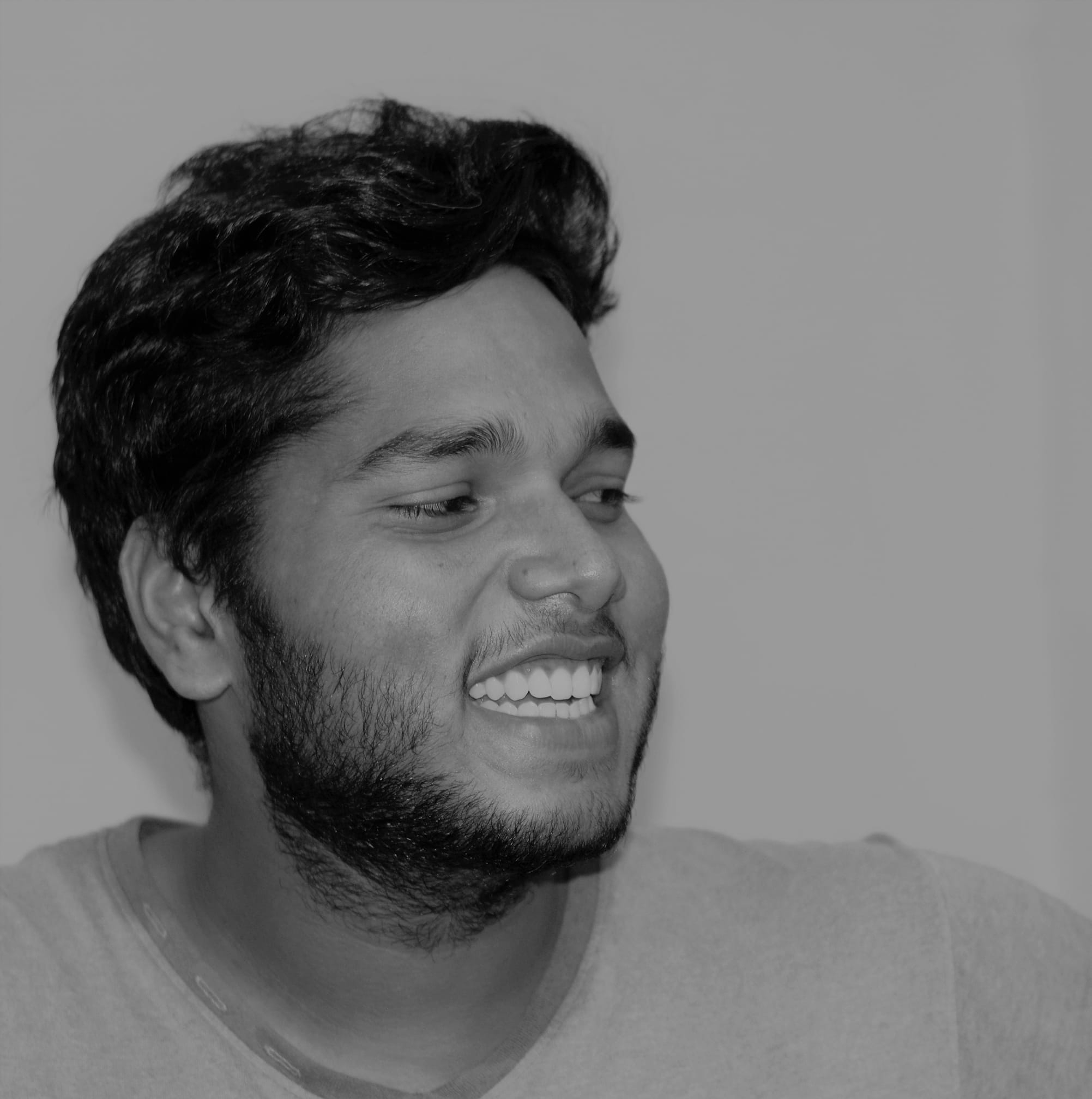
Arpit Dwivedi
Arpit Dwivedi
Crafting code with a finesse that bridges the digital divide! As a Full Stack Developer, I’ve conjured innovations at industry front-runners like Infosys and Kline & Company. With a suite boasting C#, ASP.NET CORE, Angular, and the complex dance of microservices, I’m not just programming – I’m telling stories. Beyond raw code, I'm a tech translator, dishing out insights as an avid writer, leading the charge in the open-source world, and floating on the Azure cloud. From corporates to intense tech programs, my journey’s been anything but ordinary. Curiosity? It's not just a sidekick; it's my co-pilot. Eagerly scanning the horizon for the next tech marvel. 🚀