Top 20 Advanced Angular Interview Questions and Answers
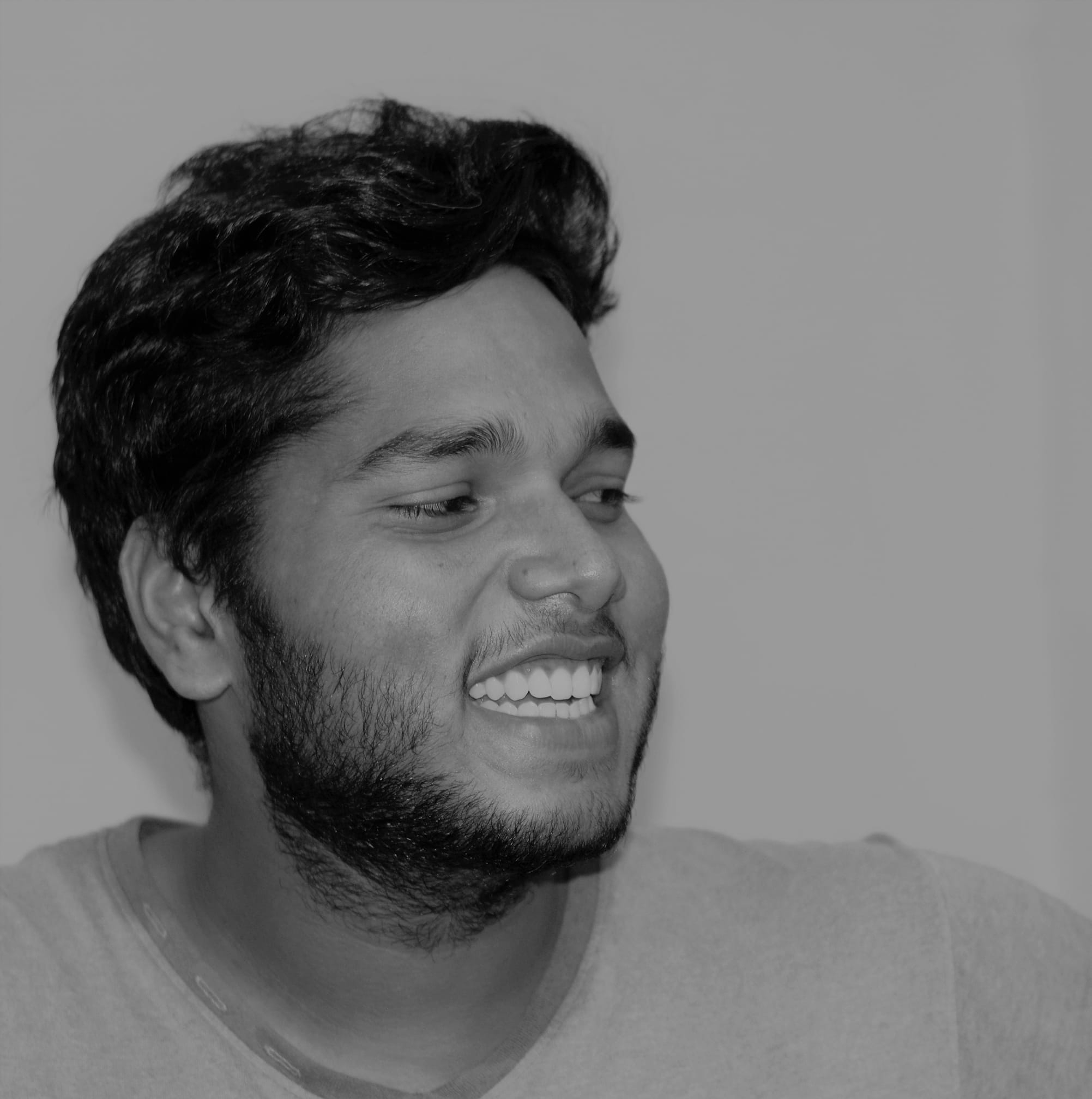
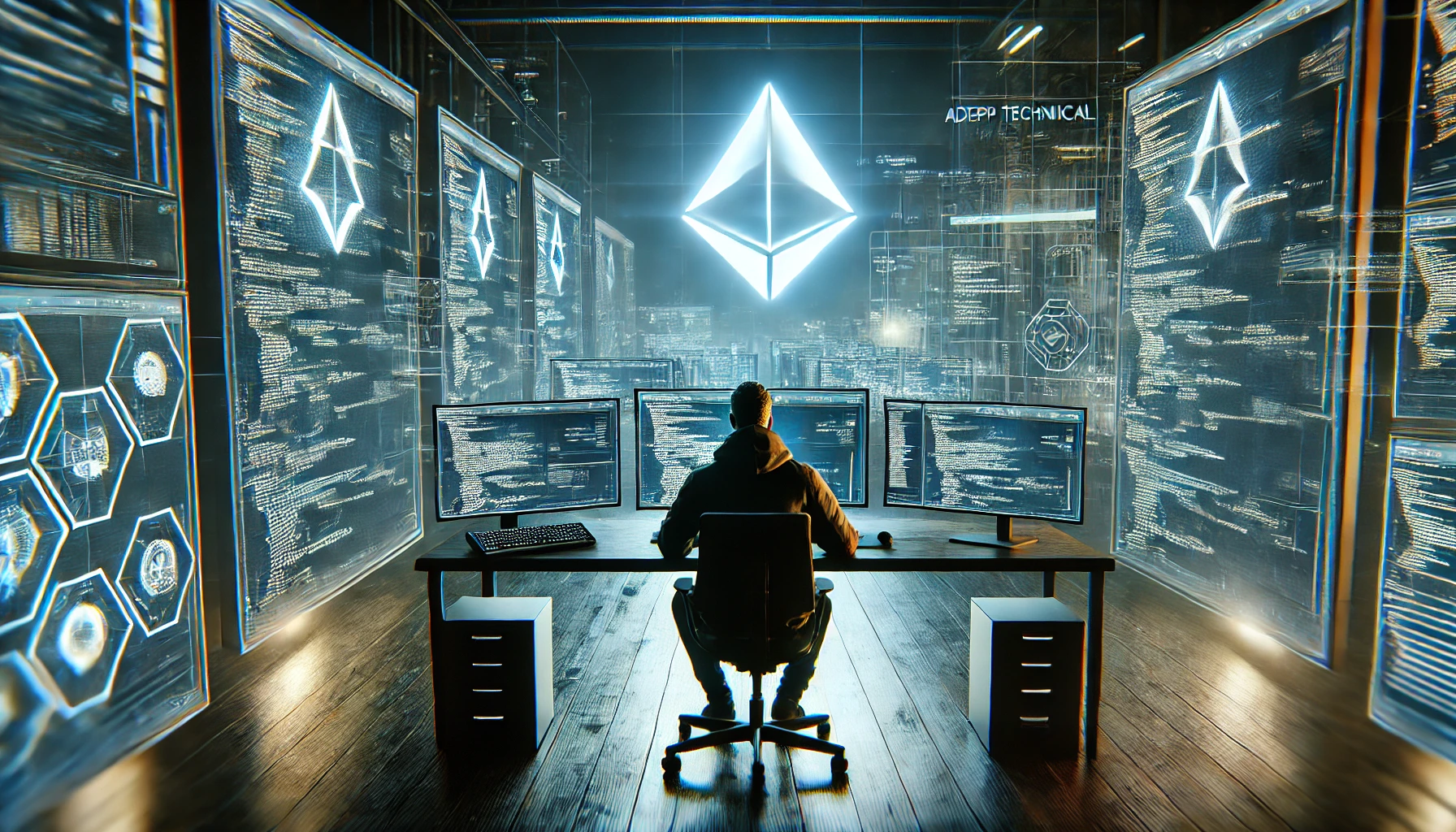
Elevate your Angular expertise with this collection of 20 advanced interview questions and answers. This article delves into complex topics like change detection strategies, Angular Ivy, RxJS observables, and performance optimization techniques. It's designed for experienced developers aiming to demonstrate their in-depth understanding of Angular's inner workings during high-level technical interviews.
What is Change Detection Strategy
OnPush
in Angular, and how does it improve performance?Answer:
The
OnPush
change detection strategy tells Angular to check a component's view only when its inputs change, significantly improving performance by reducing unnecessary checks.Explanation:
By default, Angular uses the
Default
change detection strategy, checking all components every time the model changes. WithOnPush
, Angular skips checking the component unless:An input property reference changes.
An event originated from the component or its children.
An observable linked to the template emits a new value.
This optimization reduces the change detection cycles, making applications more efficient.
Explain the concept of Angular Zones and how they relate to change detection.
Answer:
Angular Zones, powered by Zone.js, intercept asynchronous operations to automatically trigger change detection after they complete.
Explanation:
Zones create execution contexts that capture async operations like
setTimeout
, Promises, or DOM events. Angular relies on Zone.js to know when to run change detection, ensuring the UI stays in sync with the model without manual intervention.What is View Encapsulation in Angular, and what are its modes?
Answer:
View Encapsulation determines how styles defined in a component affect the DOM, with modes:
Emulated
,None
, andShadowDom
.Explanation:
Emulated (Default): Styles are scoped to components using unique attributes, preventing leakage.
None: No encapsulation; styles are global.
ShadowDom: Uses native Shadow DOM encapsulation, providing true style isolation.
Choosing the appropriate mode affects how CSS styles apply across components.
What are Ahead-of-Time (AOT) and Just-in-Time (JIT) compilation, and how do they differ in Angular?
Answer:
AOT compiles Angular code during the build time, while JIT compiles it in the browser at runtime.
Explanation:
AOT Compilation:
Reduces bundle size.
Improves startup performance.
Catches template errors early.
JIT Compilation:
Compiles templates on the fly.
Useful for development due to faster build times.
AOT is preferred for production builds to enhance performance and security.
How do you create a custom structural directive in Angular?
Answer:
A custom structural directive is created using the
@Directive
decorator with an asterisk (*
) prefix, manipulating the DOM by adding or removing elements.Explanation:
Implement the
ngOnInit
method and injectTemplateRef
andViewContainerRef
to control the view.@Directive({ selector: '[appIf]' }) export class AppIfDirective { constructor( private templateRef: TemplateRef<any>, private viewContainer: ViewContainerRef ) {} @Input() set appIf(condition: boolean) { if (condition) { this.viewContainer.createEmbeddedView(this.templateRef); } else { this.viewContainer.clear(); } } }
What is Angular Universal, and what benefits does it provide?
Answer:
Angular Universal enables server-side rendering (SSR) of Angular applications, improving performance and SEO.
Explanation:
SSR renders the initial view on the server, sending the fully rendered HTML to the client. Benefits include:
Faster first meaningful paint.
Better SEO as search engines can index pre-rendered content.
Improved social media link previews.
Explain the difference between
ViewChild
andContentChild
in Angular.Answer:
ViewChild
queries elements within a component's view, whileContentChild
queries projected content from parent components.Explanation:
ViewChild
: Accesses child components, directives, or DOM elements inside the component's template.ContentChild
: Accesses elements projected into the component using<ng-content>
.
They are used to interact with child elements programmatically.
What are dynamic components in Angular, and how do you load them at runtime?
Answer:
Dynamic components are components created and inserted into the DOM at runtime using the
ComponentFactoryResolver
.Explanation:
Use
ViewContainerRef
andComponentFactoryResolver
to create an instance of a component dynamically.@Component({ /* ... */ }) export class DynamicHostComponent { @ViewChild('container', { read: ViewContainerRef }) container: ViewContainerRef; constructor(private resolver: ComponentFactoryResolver) {} loadComponent() { const factory = this.resolver.resolveComponentFactory(DynamicComponent); this.container.createComponent(factory); } }
What is NgRx, and how does it help with state management in Angular applications?
Answer:
NgRx is a library for reactive state management in Angular, implementing the Redux pattern.
Explanation:
NgRx uses a unidirectional data flow and centralized store to manage application state predictably. Benefits include:
Easier state debugging and time-travel debugging.
Simplified state consistency across components.
Improved scalability for complex applications.
How do you optimize Angular applications for performance?
Answer:
Optimize Angular apps by implementing lazy loading, using
OnPush
change detection, minimizing bundle sizes, and avoiding unnecessary computations.Explanation:
Lazy Loading: Load modules on demand.
Change Detection Strategy: Reduce checks with
OnPush
.Tree Shaking: Remove unused code.
AOT Compilation: Enhance startup time.
Pure Pipes and Memoization: Optimize data transformations.
What is the purpose of the
Injector
hierarchy in Angular?Answer:
The
Injector
hierarchy manages dependency injection scopes, allowing different instances of services at various levels.Explanation:
Angular has hierarchical injectors:
Root Injector: Provides singleton services app-wide.
Component Injector: Allows overriding or providing services at the component level.
This hierarchy enables flexible and efficient dependency management.
Explain how to create a custom Angular Pipe and the difference between pure and impure pipes.
Answer:
A custom pipe transforms input values in templates, defined with the
@Pipe
decorator.Explanation:
Pure Pipes (Default): Executed only when input references change; ideal for performance.
Impure Pipes: Run on every change detection cycle; use
pure: false
for pipes that depend on mutable data.
@Pipe({
name: 'exponential'
})
export class ExponentialPipe implements PipeTransform {
transform(value: number, exponent: number = 1): number {
return Math.pow(value, exponent);
}
}
What are Angular Elements, and how do they integrate with non-Angular applications?
Answer:
Angular Elements allow Angular components to be packaged as custom elements (Web Components) for use in non-Angular environments.
Explanation:
By using
@angular/elements
, you can convert Angular components into standards-based custom elements, enabling their use in any HTML page or framework.How does Angular handle security, particularly XSS protection?
Answer:
Angular provides built-in security features like automatic sanitization to prevent Cross-Site Scripting (XSS) attacks.
Explanation:
Angular sanitizes untrusted values in templates. For cases requiring trusted values, developers can use the
DomSanitizer
to bypass sanitization carefully.typescriptCopy codeconstructor(private sanitizer: DomSanitizer) {} this.safeUrl = this.sanitizer.bypassSecurityTrustUrl(untrustedUrl);
What are route guards in Angular, and what types are available?
Answer:
Route guards control navigation by allowing or denying access to routes.
Explanation:
Angular provides several guard interfaces:
CanActivate
: Checks if a route can be activated.CanDeactivate
: Checks if a route can be exited.Resolve
: Pre-fetches data before route activation.CanLoad
: Determines if a module can be loaded.
Guards enhance application security and user experience.
Explain the concept of Content Projection using
ngTemplateOutlet
.Answer:
ngTemplateOutlet
allows embedding aTemplateRef
into a component's view dynamically.Explanation:
It provides advanced content projection by rendering templates based on runtime conditions.
<ng-container *ngTemplateOutlet="templateRef; context: contextObject"></ng-container>
What is the Ivy Renderer in Angular, and what benefits does it offer?
Answer:
Ivy is Angular's next-generation compilation and rendering pipeline, offering faster builds and smaller bundle sizes.
Explanation:
Ivy introduces:
Faster Compilation: Improved build times.
Better Tree Shaking: Removes unused code more effectively.
Improved Debugging: More readable generated code.
It's the default renderer from Angular version 9 onwards.
How do you implement internationalization (i18n) in Angular applications?
Answer:
Angular provides built-in support for internationalization using the
@angular/localize
package.Explanation:
Use Angular's i18n tools to mark translatable text with
i18n
attributes, extract messages, and build locale-specific versions.<p i18n="@@welcomeMessage">Welcome to our application!</p>
What are Preloading Strategies in Angular Routing, and how do they improve application performance?
Answer:
Preloading Strategies load lazy-loaded modules in the background after the app has stabilized.
Explanation:
Angular offers strategies like
PreloadAllModules
or custom strategies to preload modules, reducing wait times when users navigate to those routes.RouterModule.forRoot(routes, { preloadingStrategy: PreloadAllModules })
Explain how to handle forms with custom validation in Angular Reactive Forms.
Answer:
Custom validators in Reactive Forms are functions that take a control and return a validation result.
Explanation:
Implement custom validation logic:
function forbiddenNameValidator(nameRe: RegExp): ValidatorFn { return (control: AbstractControl): ValidationErrors | null => { const forbidden = nameRe.test(control.value); return forbidden ? { forbiddenName: { value: control.value } } : null; }; } this.form = this.fb.group({ username: ['', [forbiddenNameValidator(/admin/)]] });
Custom validators enhance form validation beyond built-in validators.
These advanced Angular interview questions and answers are designed to provide concise, high-value information, helping you quickly understand and review key concepts necessary for an in-depth understanding of Angular.
Subscribe to my newsletter
Read articles from Arpit Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
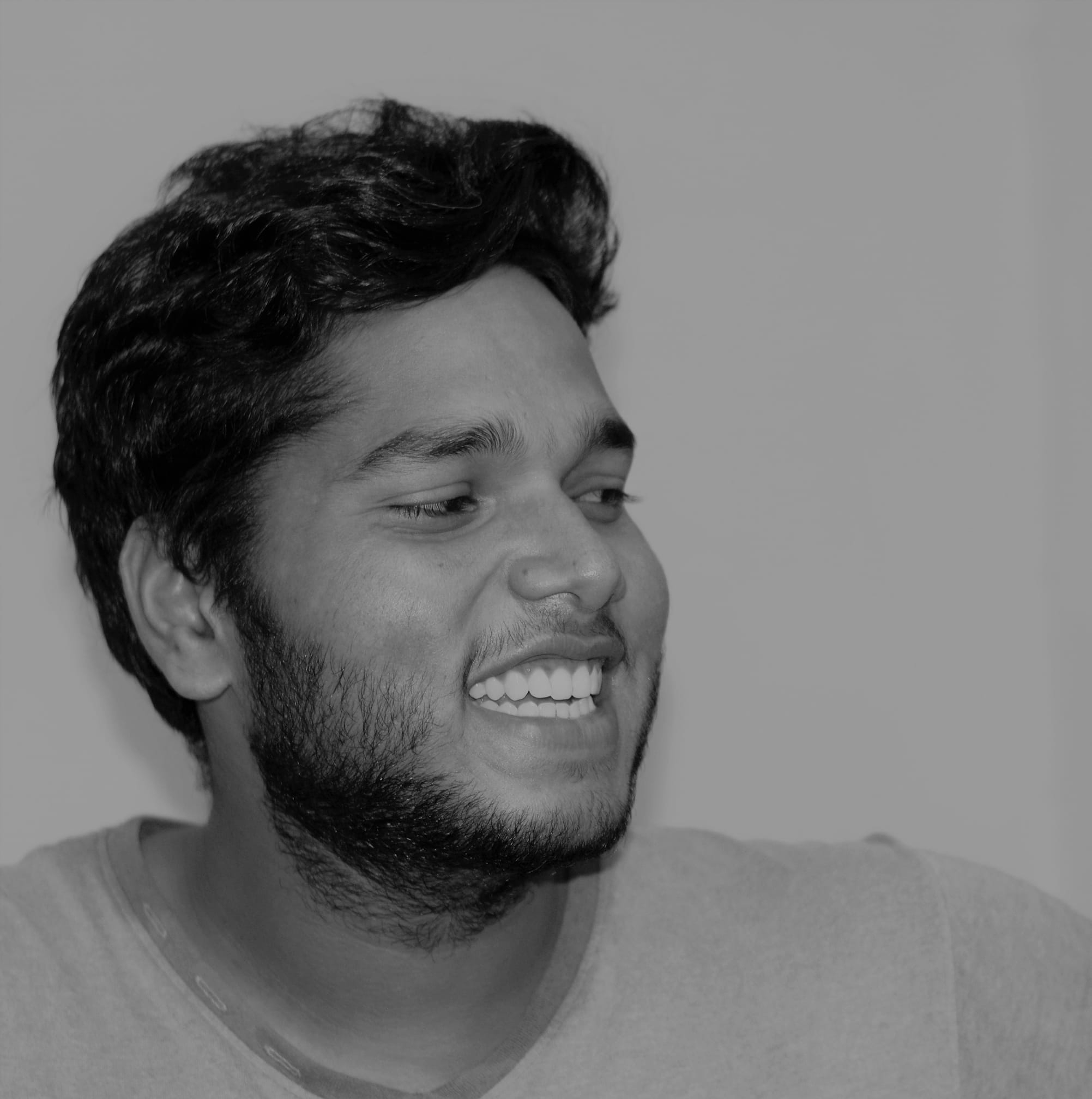
Arpit Dwivedi
Arpit Dwivedi
Crafting code with a finesse that bridges the digital divide! As a Full Stack Developer, I’ve conjured innovations at industry front-runners like Infosys and Kline & Company. With a suite boasting C#, ASP.NET CORE, Angular, and the complex dance of microservices, I’m not just programming – I’m telling stories. Beyond raw code, I'm a tech translator, dishing out insights as an avid writer, leading the charge in the open-source world, and floating on the Azure cloud. From corporates to intense tech programs, my journey’s been anything but ordinary. Curiosity? It's not just a sidekick; it's my co-pilot. Eagerly scanning the horizon for the next tech marvel. 🚀