Top 20 .NET Core Interview Questions and Answers
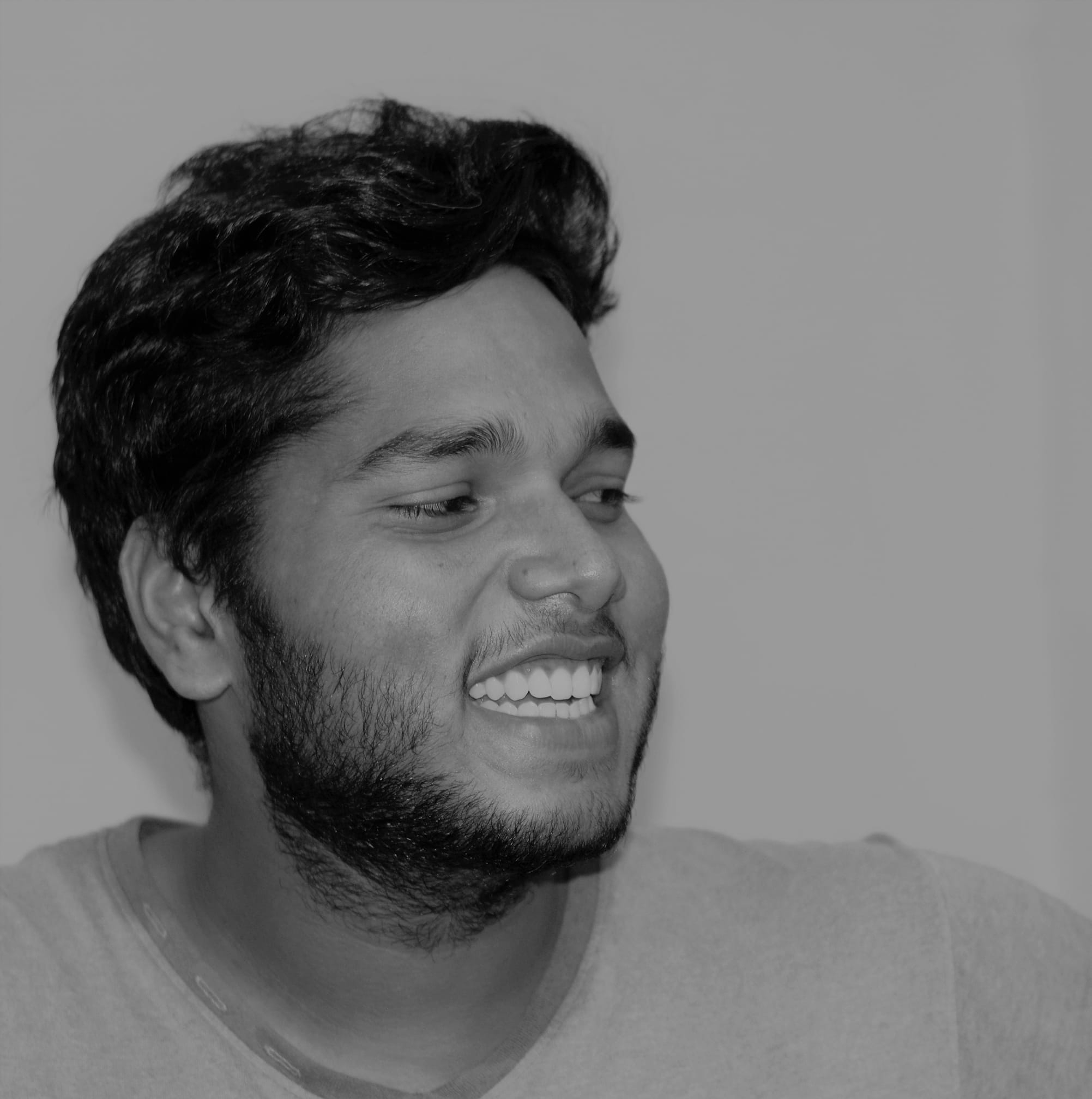
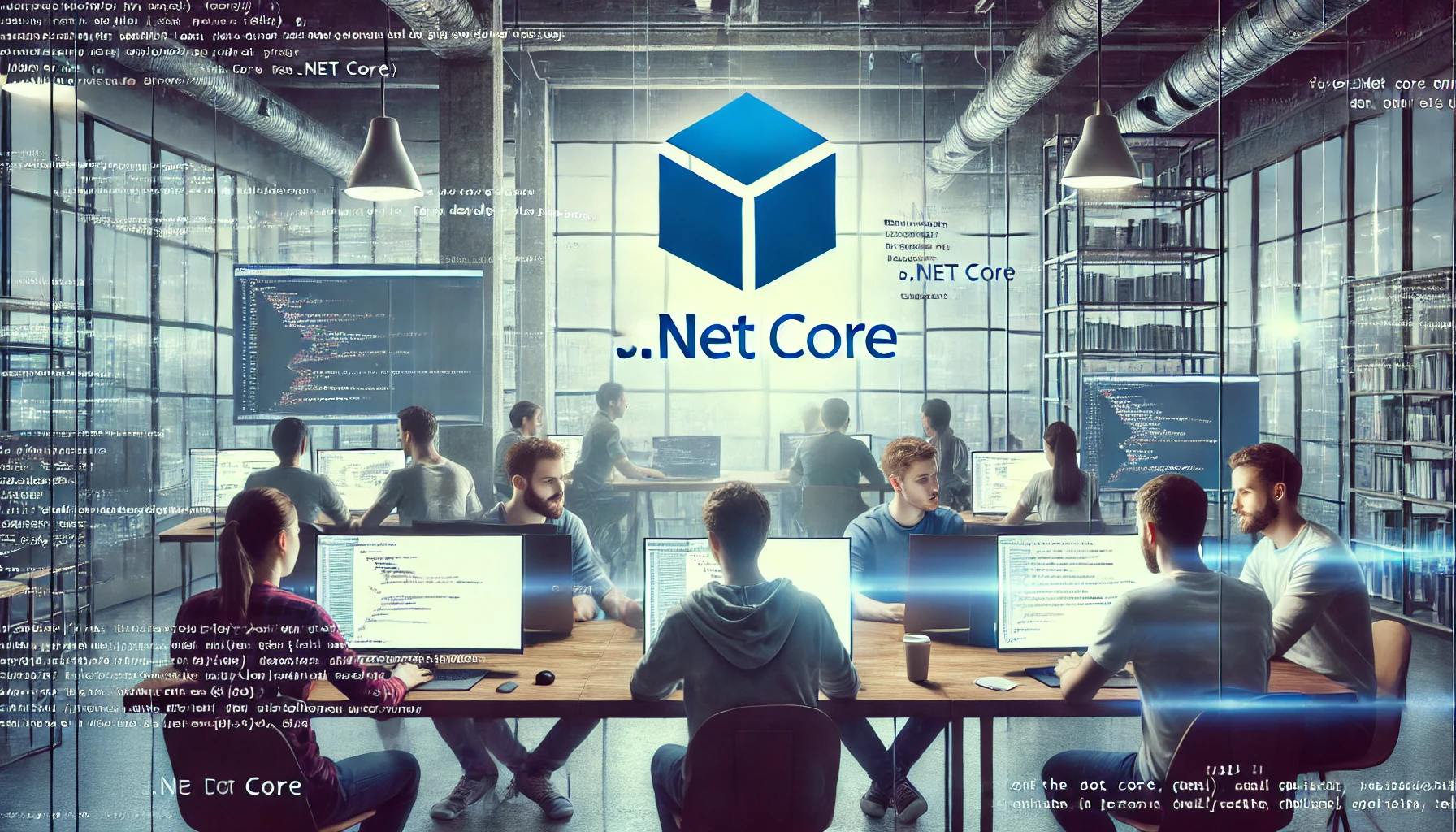
Are you gearing up for a .NET Core interview? This article presents the top 20 fundamental questions and answers that cover key aspects of .NET Core and ASP.NET Core. From understanding the differences between .NET Framework and .NET Core to exploring middleware and dependency injection, this guide will help you build a strong foundation and boost your confidence for the interview.
What is the difference between .NET Framework and .NET Core?
Answer:
.NET Framework is a Windows-only, mature framework for building desktop and web applications, while .NET Core is a cross-platform, open-source framework designed for building modern applications on Windows, Linux, and macOS.
Explanation:
.NET Framework:
Released in 2002.
Supports Windows platforms.
Includes Windows Forms, WPF for desktop applications.
Web applications built using ASP.NET.
.NET Core:
Introduced in 2016.
Cross-platform support.
Modular, lightweight, and high performance.
Web applications built using ASP.NET Core.
Suitable for microservices and cloud-based applications.
What is ASP.NET Core, and why is it important?
Answer:
ASP.NET Core is a cross-platform, high-performance framework for building modern web applications and APIs.
Explanation:
Unified framework for MVC and Web API.
Improved performance and scalability.
Supports dependency injection natively.
Built-in support for modern web development practices.
Can run on .NET Core or .NET Framework.
Explain the project structure of a typical ASP.NET Core application.
Answer:
An ASP.NET Core project typically includes the
Program.cs
andStartup.cs
files, along with configuration files likeappsettings.json
.Explanation:
Program.cs: Contains the
Main
method and sets up the web host.Startup.cs: Configures services and middleware in the
ConfigureServices
andConfigure
methods.appsettings.json: Stores configuration settings.
Controllers, Models, Views: Organized per MVC pattern.
What are Middleware components in ASP.NET Core?
Answer:
Middleware are software components that form a pipeline to handle HTTP requests and responses in ASP.NET Core applications.
Explanation:
Each middleware component can process requests and pass them to the next component.
Examples include authentication, routing, error handling.
Configured in the
Configure
method ofStartup.cs
usingapp.Use...
methods.
How does Dependency Injection work in ASP.NET Core?
Answer:
Dependency Injection (DI) in ASP.NET Core allows services to be injected into components, promoting loose coupling and easier testing.
Explanation:
Built-in DI container registers services in
ConfigureServices
.Services can have lifetimes: Singleton, Scoped, or Transient.
Components like controllers request services via constructor parameters.
What are the different service lifetimes in ASP.NET Core Dependency Injection?
Answer:
The service lifetimes are Singleton, Scoped, and Transient.
Explanation:
Singleton: One instance throughout the application's lifetime.
Scoped: One instance per request.
Transient: A new instance each time it's requested.
What is Kestrel in ASP.NET Core?
Answer:
Kestrel is the default cross-platform web server for ASP.NET Core applications.
Explanation:
Lightweight and high-performance.
Can be used as an edge server or behind a reverse proxy like IIS or Nginx.
Supports HTTPS, WebSockets, and other modern web protocols.
Explain the use of
appsettings.json
in ASP.NET Core.Answer:
appsettings.json
is a configuration file that stores application settings in a JSON format.Explanation:
Replaces
web.config
from previous ASP.NET versions.Supports hierarchical data and environment-specific configurations.
Accessed using the Configuration API.
What is the purpose of the
IHostingEnvironment
interface?Answer:
IHostingEnvironment
provides information about the web hosting environment.Explanation:
Determines the current environment (Development, Staging, Production).
Used to configure environment-specific services or middleware.
Accessed via dependency injection.
How do you implement Logging in ASP.NET Core?
Answer:
Logging in ASP.NET Core is implemented using the built-in Logging API, which allows logging to various providers.
Explanation:
Configure logging providers in
ConfigureServices
.Use the
ILogger
interface in controllers or services.Supports filtering logs by levels like Information, Warning, Error.
What is Routing in ASP.NET Core, and how does it work?
Answer:
Routing in ASP.NET Core maps incoming HTTP requests to controller actions.
Explanation:
Configured in
Startup.cs
usingUseRouting
andUseEndpoints
.Supports attribute routing and conventional routing.
Enables creating RESTful APIs and clean URLs.
Explain Model Binding and Validation in ASP.NET Core.
Answer:
Model Binding automatically maps HTTP request data to action method parameters, while Validation ensures the data meets specified rules.
Explanation:
Uses data annotations like
[Required]
,[Range]
for validation.Model state is checked using
ModelState.IsValid
.Validation errors can be returned to the client.
What are Tag Helpers in ASP.NET Core MVC?
Answer:
Tag Helpers enable server-side code to participate in creating and rendering HTML elements in Razor views.
Explanation:
Provide an HTML-friendly way to work with Razor.
Built-in Tag Helpers include forms, links, and input controls.
Custom Tag Helpers can be created for reusable components.
What is the difference between Razor Pages and MVC in ASP.NET Core?
Answer:
Razor Pages is a page-based programming model, while MVC is a controller-based model.
Explanation:
Razor Pages:
Focuses on individual pages.
Combines page model and view.
Simpler for page-centric scenarios.
MVC:
Uses Controllers, Models, and Views.
Suitable for complex applications.
How do you implement authentication and authorization in ASP.NET Core?
Answer:
Authentication and authorization are implemented using middleware and attributes like
[Authorize]
.Explanation:
Use Identity for membership system.
Configure authentication schemes like Cookies, JWT Bearer.
Apply policies and roles for authorization.
What is Entity Framework Core, and how is it used in ASP.NET Core?
Answer:
Entity Framework Core (EF Core) is an ORM that allows developers to work with databases using .NET objects.
Explanation:
Supports LINQ queries, change tracking, and migrations.
Configured in
ConfigureServices
usingAddDbContext
.Works with multiple database providers like SQL Server, SQLite.
Explain the concept of Middleware Pipeline in ASP.NET Core.
Answer:
The Middleware Pipeline is the sequence of middleware components through which an HTTP request passes.
Explanation:
Configured in
Startup.Configure
.Order matters; middleware is executed in the order added.
Each middleware can handle requests and responses.
What are Filters in ASP.NET Core MVC?
Answer:
Filters are attributes that run code before or after specific stages in the request processing pipeline.
Explanation:
Types include Authorization, Action, Result, and Exception filters.
Can be applied globally, to controllers, or to actions.
Used for cross-cutting concerns like logging, caching.
How do you perform Configuration in ASP.NET Core applications?
Answer:
Configuration is performed using the Configuration API, which reads settings from various sources.
Explanation:
Supports JSON files, environment variables, command-line arguments.
Accessed via
IConfiguration
.Supports options pattern with strongly typed settings classes.
What is the role of
UseEndpoints
in ASP.NET Core?Answer:
UseEndpoints
configures the endpoints for routing in the middleware pipeline.Explanation:
Placed after
UseRouting
inStartup.Configure
.Maps endpoints for controllers, Razor Pages, SignalR hubs.
Allows for centralized route configuration.
These questions cover essential concepts in .NET Core and ASP.NET Core, providing concise answers and explanations suitable for quick review and interview preparation.
Subscribe to my newsletter
Read articles from Arpit Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
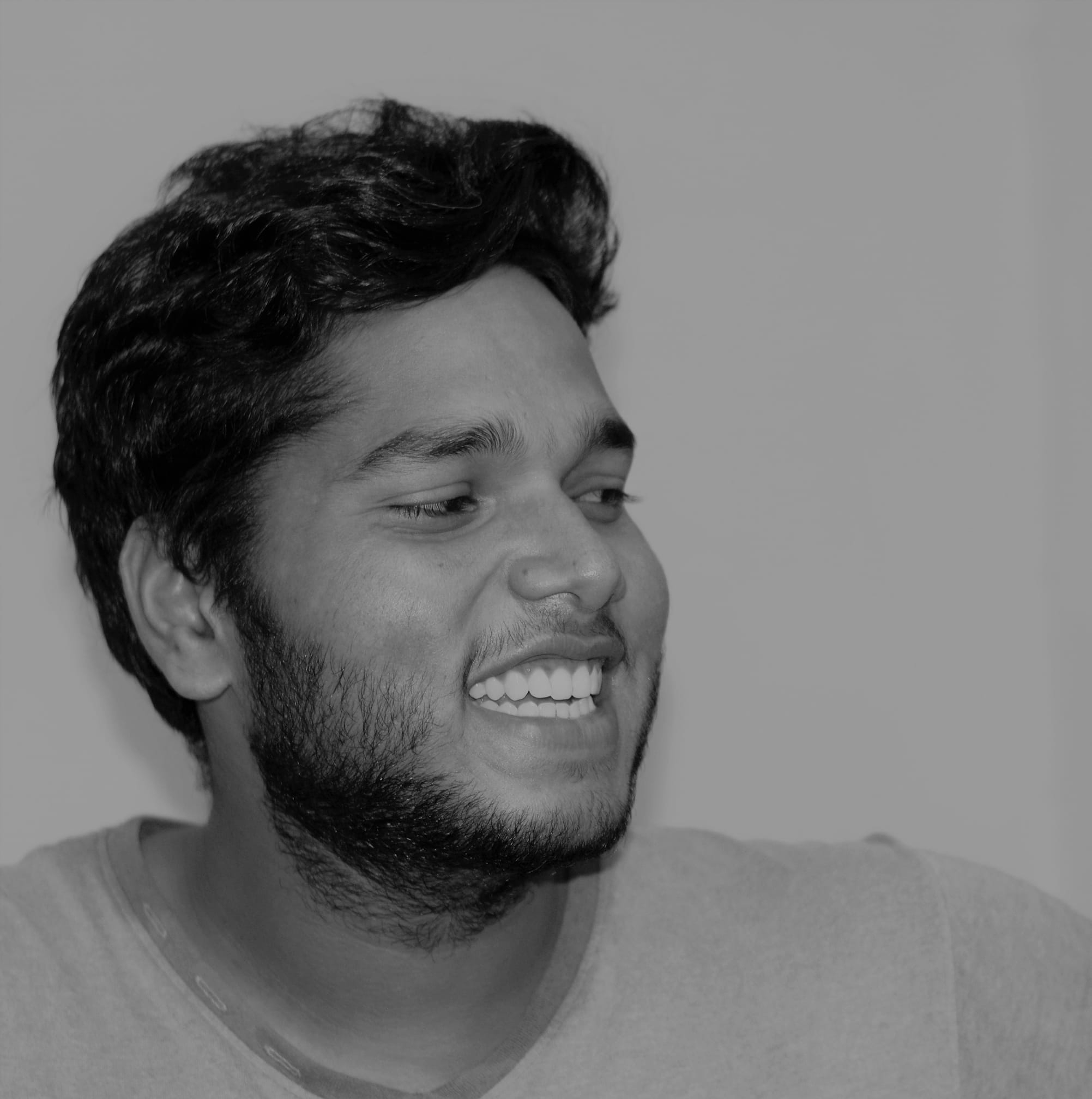
Arpit Dwivedi
Arpit Dwivedi
Crafting code with a finesse that bridges the digital divide! As a Full Stack Developer, I’ve conjured innovations at industry front-runners like Infosys and Kline & Company. With a suite boasting C#, ASP.NET CORE, Angular, and the complex dance of microservices, I’m not just programming – I’m telling stories. Beyond raw code, I'm a tech translator, dishing out insights as an avid writer, leading the charge in the open-source world, and floating on the Azure cloud. From corporates to intense tech programs, my journey’s been anything but ordinary. Curiosity? It's not just a sidekick; it's my co-pilot. Eagerly scanning the horizon for the next tech marvel. 🚀