Creating an Options Page for Your Chrome Extension

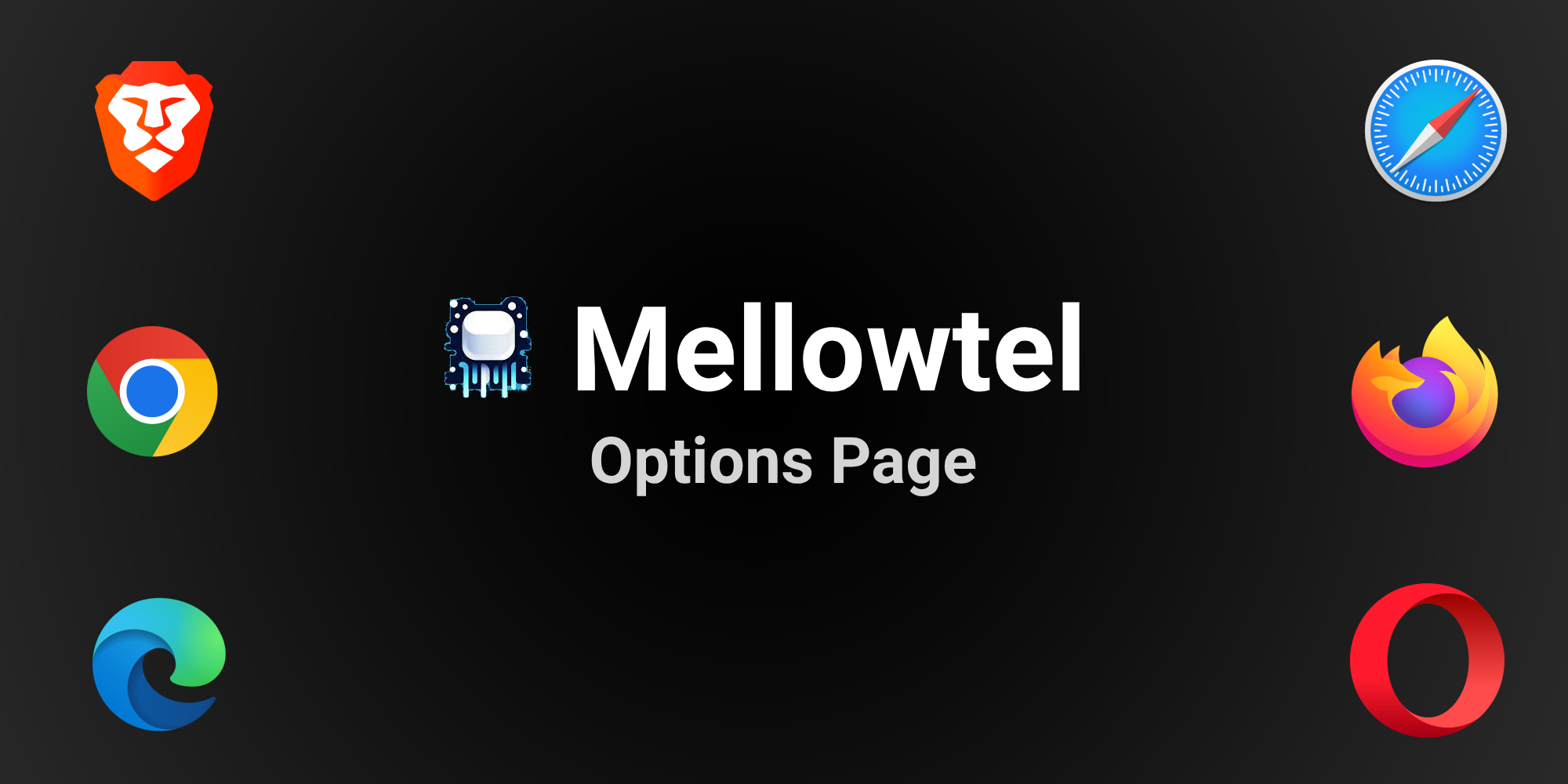
An options page allows users to customize your Chrome extension's behavior. This guide will walk you through creating and implementing an options page in your extension.
What is an Options Page?
An options page is a dedicated page that allows users to configure settings for your extension. It's a great way to make your extension more flexible and user-friendly.
If you're new to Chrome extension development, start with our guide on How to Create Your First Chrome Extension.
Steps to Create an Options Page
1. Create the Options Page HTML
First, create a file named options.html
in your extension's directory:
<!DOCTYPE html>
<html>
<head><title>My Extension Options</title></head>
<body>
<label>
Favorite color:
<select id="color">
<option value="red">red</option>
<option value="green">green</option>
<option value="blue">blue</option>
<option value="yellow">yellow</option>
</select>
</label>
<button id="save">Save</button>
<script src="options.js"></script>
</body>
</html>
2. Create the Options Page JavaScript
Create a file named options.js
:
// Saves options to chrome.storage
function save_options() {
var color = document.getElementById('color').value;
chrome.storage.sync.set({
favoriteColor: color
}, function() {
// Update status to let user know options were saved.
var status = document.getElementById('status');
status.textContent = 'Options saved.';
setTimeout(function() {
status.textContent = '';
}, 750);
});
}
// Restores select box and checkbox state using the preferences
// stored in chrome.storage.
function restore_options() {
chrome.storage.sync.get({
favoriteColor: 'red' // default value
}, function(items) {
document.getElementById('color').value = items.favoriteColor;
});
}
document.addEventListener('DOMContentLoaded', restore_options);
document.getElementById('save').addEventListener('click', save_options);
This script uses the Chrome Extension Storage API to save and retrieve user preferences.
3. Register the Options Page in manifest.json
Add the options page to your manifest.json
:
{
"options_page": "options.html"
}
Using Options in Your Extension
You can access the saved options in other parts of your extension, such as background scripts or content scripts:
chrome.storage.sync.get({
favoriteColor: 'red' // default value
}, function(items) {
console.log('The user's favorite color is ' + items.favoriteColor);
});
Best Practices
Keep the options simple and easy to understand
Provide default values for all options
Use appropriate input types (checkboxes, radio buttons, select dropdowns) for different types of options
Provide immediate feedback when options are saved
Consider using the options page to allow users to customize API endpoints or provide API keys for Making API Calls in Chrome Extensions
Conclusion
An options page is a powerful tool for making your Chrome extension more flexible and user-friendly. By following this guide, you've learned how to create an options page, save user preferences, and use those preferences in your extension.
As you develop more complex extensions, consider how you can use options pages in conjunction with other extension features to create a customizable and powerful user experience.
Subscribe to my newsletter
Read articles from Mellowtel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mellowtel
Mellowtel
Easily monetize your browser extensions. Mellowtel is an open-source, ethical monetization engine that respects user privacy and maximizes your revenue.