String Slicing in Python ✂️

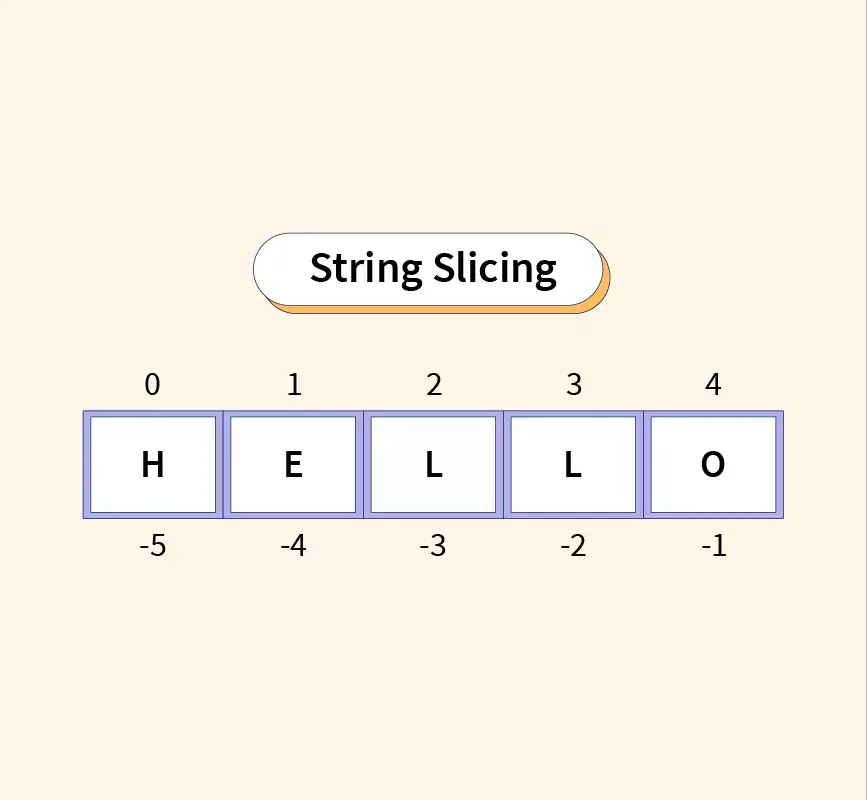
Introduction
String slicing is a technique used to extract specific portions of a string. By using slicing, you can retrieve substrings, reverse strings, or even skip characters. In this blog, we’ll explore how to slice strings efficiently.
Understanding String Indexing 🔢
Each character in a string has an index. Python allows you to access individual characters using these indexes. Indexes start from 0
for the first character and -1
for the last character.
text = 'Python'
print(text[0]) # Output: P
print(text[-1]) # Output: n
Basic Slicing Syntax 🧵
The basic syntax for slicing is:
string[start:stop:step]
start
: The starting index (inclusive).stop
: The ending index (exclusive).step
: The interval between characters.
Slicing from start to end:
text = 'Python' print(text[0:4]) # Output: Pyth
Omitting start or end:
pythonCopy codeprint(text[:4]) # Output: Pyth (start from 0) print(text[3:]) # Output: hon (end at last)
Using step:
pythonCopy codeprint(text[0:6:2]) # Output: Pto (every second character)
Negative Indexing 🛑
You can also use negative numbers to slice from the end of the string.
pythonCopy codeprint(text[-4:]) # Output: thon
print(text[-6:-2]) # Output: Pyth
Reversing a String 🔄
By using a negative step, you can easily reverse a string.
pythonCopy codeprint(text[::-1]) # Output: nohtyP
In this blog, we covered the basics of string slicing. Understanding slicing is crucial when working with strings as it allows you to manipulate text efficiently. In the next blog, we’ll cover advanced slicing techniques, where we’ll dive deeper into slicing patterns and tricks.
Subscribe to my newsletter
Read articles from Shrey Dikshant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrey Dikshant
Shrey Dikshant
Aspiring data scientist with a strong foundation in adaptive quality techniques. Gained valuable experience through internships at YT Views, focusing on operation handling. Proficient in Python and passionate about data visualization, aiming to turn complex data into actionable insights.