Debouncing in JavaScript: Optimize Performance by Limiting Function Calls
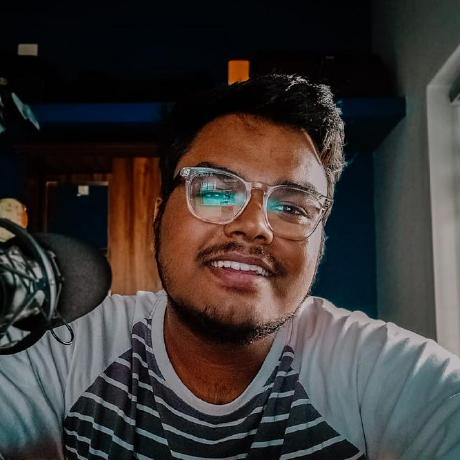
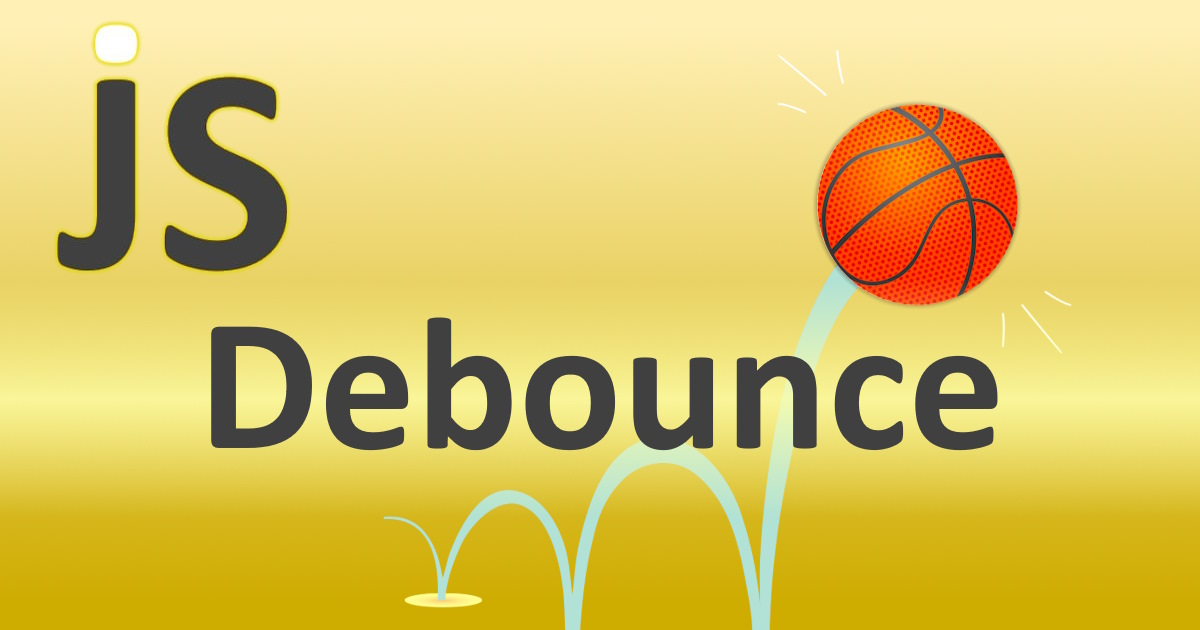
In modern web applications, performance is key to ensuring a seamless user experience. One common issue that can degrade performance is the frequent and unnecessary execution of functions, especially during high-frequency events like typing, scrolling, or resizing the browser window. This is where debouncing comes into play.
Debouncing is a programming technique used to limit the number of times a function is executed, ensuring that it only fires after a specified delay. It’s particularly useful in situations where a function might otherwise be called too frequently, which can lead to performance bottlenecks.
When to Use Debouncing
Debouncing is helpful in the following scenarios:
Search Input Fields: To limit API calls when a user types in a search box. Instead of sending a request with each keystroke, debounce can wait until the user has stopped typing for a specified time.
Window Resize Events: Frequently triggered events like window resizing can degrade performance if a function is fired with every slight change in size.
Button Clicks: Avoid multiple unintended clicks by debouncing the button’s click handler.
How Debouncing Works
The core idea behind debouncing is to delay the function execution until a specified amount of time has passed since the last invocation. If the function is called again during the wait period, the timer resets, and the function is delayed again.
This mechanism ensures that the function will only execute after the user has completed an action, improving the app's responsiveness and performance.
Debounce Implementation in JavaScript
Let’s break down a simple debounce function in JavaScript
function debounce(func, delay) { let timeoutId; return function (...args) { if (timeoutId) clearTimeout(timeoutId); timeoutId = setTimeout(() => { func(...args); }, delay); }; }
Explanation:
debounce(func, delay)
returns a new function.Inside this new function, we use
setTimeout
to ensure the original function (func
) is executed only after the specified delay.The
clearTimeout(timeoutId)
ensures that if the function is called again before the delay finishes, the previous timeout is canceled, and a new one is set.This simple debounce implementation limits how frequently the provided function (
func
) is executed.Debouncing in Action
Here’s an example of debouncing for a search input field
const searchInput = document.querySelector('#search'); searchInput.addEventListener('input', debounce((event) => { // This function will only execute after the user has stopped typing for 300ms. console.log(`Searching for: ${event.target.value}`); }, 300));
In this example, the
debounce
function ensures that the search functionality only fires after the user has stopped typing for 300 milliseconds. This minimizes the number of requests sent to the server, improving both performance and user experience.Debouncing vs Throttling
While debouncing limits how often a function can be called by delaying its execution until a specific time has passed, throttling is another technique that limits the number of times a function can execute over time. Throttling ensures that the function is executed at regular intervals, regardless of how many times the event is triggered.
Key Differences:
Debounce: Function fires after the specified delay from the last event.
Throttle: Function fires at regular intervals during the event.
Benefits of Using Debouncing
Improved Performance: Debouncing prevents excessive function calls, reducing CPU and memory usage, especially in scenarios involving rapid events like scrolling or resizing.
Enhanced User Experience: By reducing the number of function calls, especially in search bars or forms, debouncing ensures smoother and more responsive applications.
Efficient API Calls: Debouncing minimizes the number of API requests made during user input, reducing server load and improving response times.
Conclusion
Debouncing is an essential technique in modern JavaScript development that helps prevent performance degradation by limiting the frequency of function execution. By delaying function calls until a specified delay has passed, debouncing can dramatically improve the efficiency of your applications, particularly when dealing with high-frequency events.
Subscribe to my newsletter
Read articles from NiKHIL NAIR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
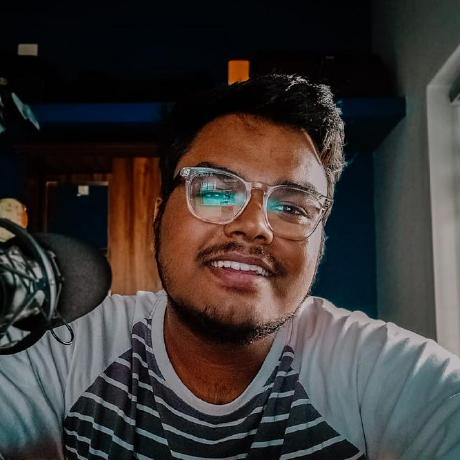