Kubernetes Pods

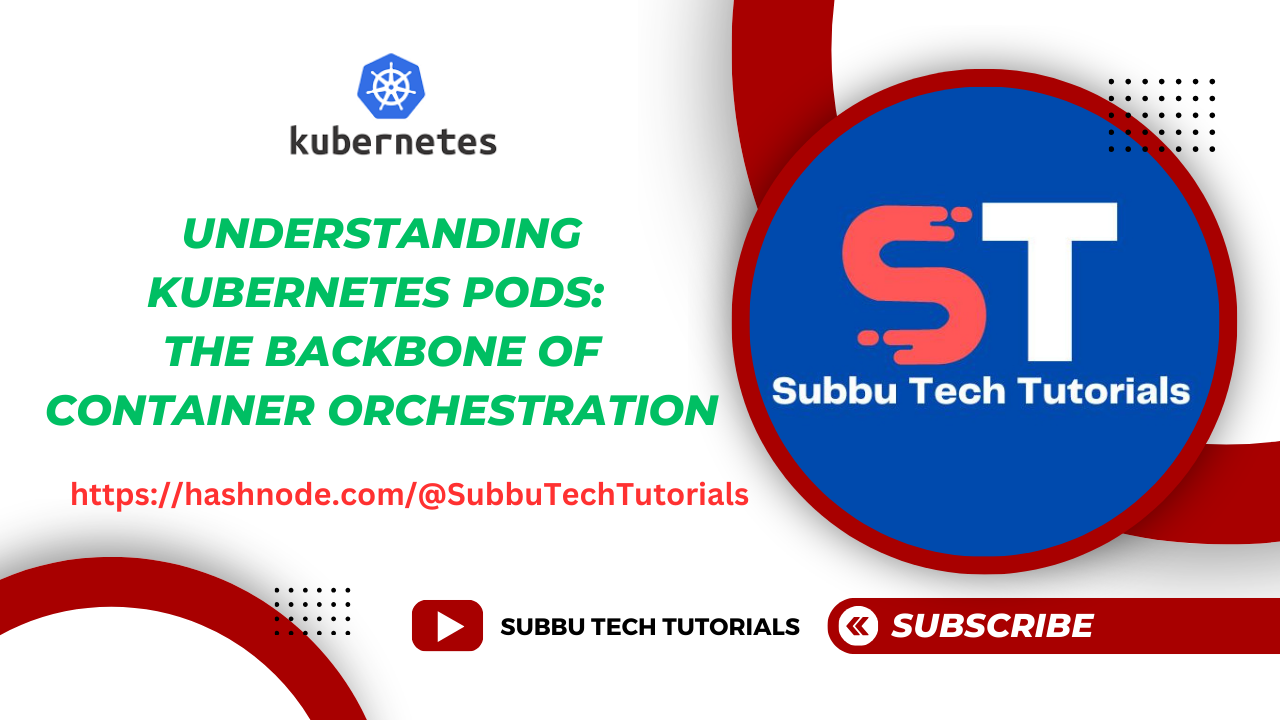
Introduction:
Why Pods?
In Kubernetes, Pods solve the limitations of containers by allowing multiple containers to run together, share resources, and communicate more easily. This makes it simpler for Kubernetes to manage scaling, updates, and load balancing, overcoming the challenges of handling individual containers.
Issues with Containers:
Single Process Focus: Containers are designed to run a single process, which can make managing multi-container applications difficult.
Limited Resource Sharing: Containers have their own isolated storage and network, which can complicate inter-container communication and resource sharing.
Lack of Coordination: Without an abstraction layer, coordinating multiple containers to work as a single unit is challenging.
How Pods Overcome These Issues in Kubernetes:
Multiple Containers in a Pod: Pods can run multiple containers together, allowing them to share resources and work closely.
Shared Networking: Containers in a pod share the same IP address and ports, simplifying communication.
Centralized Management: Kubernetes manages pods as a single entity, allowing features like scaling, fault tolerance, and rolling updates.
What is a Pod in Kubernetes?
A Pod is a Kubernetes abstraction that represents a group of one or more
application containers (such as Docker), and some shared resources for
those containers.
Those resources include:
Shared storage, as Volumes
Networking, as a unique cluster IP address
Information about how to run each container, such as the container image
version or specific ports to use.
Hardware units:
Node:
The smallest computing unit in Kubernetes, typically representing a single
machine (physical or virtual), that runs containerized applications.
Cluster:
A group of machines (nodes) that work together to share resources, balance
workloads, and ensure reliability in a Kubernetes environment.
Software units:
Linux container:
A lightweight software unit that encapsulates one or more processes along with
all the necessary files, making it portable across different machines.
Kubernetes pod:
The smallest deployable unit in Kubernetes, consisting of one or more Linux
containers that share resources, designed for efficient cluster management
and resource utilization.
Note:
You need to install a container runtime into each node in the cluster so that
Pods can run there.
Pods overview:
How pods are working in Kubernetes?
Containers Sharing Resources: Containers within a pod share networking (a single IP address) and storage (volumes).
Deployment: Pods are scheduled on nodes where they are managed and orchestrated by Kubernetes.
Lifecycle Management: Kubernetes monitors pods, restarting them if they fail, scaling them as needed, and managing updates.
Communication: Pods can communicate with each other through services and can expose applications to the external world using Kubernetes services like Load Balancer or Ingress.
Key Points:
Runs Containers: A pod usually runs one or more containers (like Docker containers), which are the actual applications.
One Application per Pod: Typically, one pod runs one application or a specific part of an application.
Short-Lived: Pods aren't permanent. If a pod crashes or finishes its job, Kubernetes can automatically create a new one.
Assigned to a Node: Pods run on nodes (which are like servers), and Kubernetes ensures they run efficiently.
Networking: Each pod gets its own internal IP address, allowing it to communicate with other pods or the outside world.
The Pod Manifest:
Pods are described in a Pod manifest. The Pod manifest is just a text-file representation of the Kubernetes API object. Kubernetes strongly believes in declarative configuration.
Imperative Configuration: In contrast, imperative configuration involves manually executing commands to change the system. For example, running apt-get install foo
to install software manually.
Ex: To create a pod imperatively, you directly run a command:
kubectl run my-pod --image=nginx
Declarative Configuration: In Kubernetes, declarative configuration means you define the desired state of the system in a configuration file (e.g., a Pod manifest), and Kubernetes ensures that this state is achieved. For example, you write a YAML file specifying that a pod should run with a certain container image.
To create a pod declaratively, you write a YAML file (pod-manifest.yaml) like this:
apiVersion: v1
kind: Pod
metadata:
name: nginx
spec:
containers:
- name: nginx
image: nginx:1.14.2
ports:
- containerPort: 80
Then, you apply it using:
kubectl apply -f pod-manifest.yaml
Pod Lifecycle:
Pending: The pod is accepted by the cluster, but one or more containers are not yet running. This could be because resources are being assigned or the pod is waiting for scheduling.
Running: The pod is successfully scheduled, and all containers are running or being started.
Succeeded: All containers in the pod have successfully completed and will not restart.
Failed: One or more containers have terminated, and at least one container failed.
Unknown: It occurs when the Kubelet (the node agent) cannot communicate with the API server, meaning Kubernetes doesn’t know the actual state of the pod
CrashLoopBackOff: A pod is continuously crashing and restarting due to container errors.
Basic Commands:
kubectl config get-contexts
To determine which namespace is associated with your current Kubernetes context.
#: kubectl config get-contexts CURRENT NAME CLUSTER AUTHINFO NAMESPACE * kubernetes-admin@kubernetes kubernetes kubernetes-admin my-namespace
kubectl get pods
Lists all the pods in the current namespace. If no namespace is specified, it defaults to the 'default' namespace.
#: kubectl get pods NAME READY STATUS RESTARTS AGE my-pod 1/1 Running 2 (4h6m ago) 2d19h
kubectl get pod -o wide
It provides detailed information about the pods in your current namespace, including which node they are running on and other extended details.
#: kubectl get pod -o wide NAME READY STATUS RESTARTS AGE IP NODE NOMINATED NODE READINESS GATES my-pod 1/1 Running 2 (4h59m ago) 2d20h 10.44.0.2 ip-172-31-26-203 <none> <none>
kubectl get pods --namespace=<namespace>
Lists all the pods in a specific namespace.
Example: kubectl get pods --namespace=my-namespace
#: kubectl get pods --namespace=my-namespace NAME READY STATUS RESTARTS AGE my-pod 1/1 Running 2 (4h28m ago) 2d19h
kubectl get pods --all-namespaces
To list all pods across all namespaces
#: kubectl get pods --all-namespaces NAMESPACE NAME READY STATUS RESTARTS AGE default nginx-demo 1/1 Running 5 (4h47m ago) 12d kube-system coredns-6f6b679f8f-fzqqx 1/1 Running 5 (4h47m ago) 12d kube-system coredns-6f6b679f8f-kznll 1/1 Running 5 (4h47m ago) 12d kube-system etcd-ip-172-31-25-43 1/1 Running 5 (4h47m ago) 12d kube-system kube-apiserver-ip-172-31-25-43 1/1 Running 5 (4h47m ago) 12d kube-system kube-controller-manager-ip-172-31-25-43 1/1 Running 5 (4h47m ago) 12d kube-system kube-proxy-29v2d 1/1 Running 5 (4h47m ago) 12d kube-system kube-proxy-9pszf 1/1 Running 5 (4h47m ago) 12d kube-system kube-proxy-lrqpv 1/1 Running 5 (4h47m ago) 12d kube-system kube-scheduler-ip-172-31-25-43 1/1 Running 5 (4h47m ago) 12d kube-system metrics-server-587b667b55-774k9 1/1 Running 0 135m kube-system weave-net-9gpqz 2/2 Running 11 (4h47m ago) 12d kube-system weave-net-dxvcz 2/2 Running 11 (4h47m ago) 12d kube-system weave-net-pjjtt 2/2 Running 11 (4h47m ago) 12d my-namespace my-pod 1/1 Running 2 (4h47m ago) 2d20h
kubectl get pods --all-namespaces -o wide
To list all pods across all namespaces and see which node they are running on.
#: kubectl get pods --all-namespaces -o wide NAMESPACE NAME READY STATUS RESTARTS AGE IP NODE NOMINATED NODE READINESS GATES default nginx-demo 1/1 Running 5 (4h39m ago) 12d 10.44.0.1 ip-172-31-26-203 <none> <none> kube-system coredns-6f6b679f8f-fzqqx 1/1 Running 5 (4h39m ago) 12d 10.32.0.2 ip-172-31-25-43 <none> <none> kube-system coredns-6f6b679f8f-kznll 1/1 Running 5 (4h39m ago) 12d 10.32.0.3 ip-172-31-25-43 <none> <none> kube-system etcd-ip-172-31-25-43 1/1 Running 5 (4h39m ago) 12d 172.31.25.43 ip-172-31-25-43 <none> <none> kube-system kube-apiserver-ip-172-31-25-43 1/1 Running 5 (4h39m ago) 12d 172.31.25.43 ip-172-31-25-43 <none> <none> kube-system kube-controller-manager-ip-172-31-25-43 1/1 Running 5 (4h39m ago) 12d 172.31.25.43 ip-172-31-25-43 <none> <none> kube-system kube-proxy-29v2d 1/1 Running 5 (4h39m ago) 12d 172.31.21.7 ip-172-31-21-7 <none> <none> kube-system kube-proxy-9pszf 1/1 Running 5 (4h39m ago) 12d 172.31.25.43 ip-172-31-25-43 <none> <none> kube-system kube-proxy-lrqpv 1/1 Running 5 (4h39m ago) 12d 172.31.26.203 ip-172-31-26-203 <none> <none> kube-system kube-scheduler-ip-172-31-25-43 1/1 Running 5 (4h39m ago) 12d 172.31.25.43 ip-172-31-25-43 <none> <none> kube-system metrics-server-587b667b55-774k9 1/1 Running 0 128m 10.44.0.3 ip-172-31-26-203 <none> <none> kube-system weave-net-9gpqz 2/2 Running 11 (4h39m ago) 12d 172.31.25.43 ip-172-31-25-43 <none> <none> kube-system weave-net-dxvcz 2/2 Running 11 (4h39m ago) 12d 172.31.21.7 ip-172-31-21-7 <none> <none> kube-system weave-net-pjjtt 2/2 Running 11 (4h39m ago) 12d 172.31.26.203 ip-172-31-26-203 <none> <none> my-namespace my-pod 1/1 Running 2 (4h39m ago) 2d20h 10.44.0.2 ip-172-31-26-203 <none> <none>
kubectl describe pod <pod-name>
Provides detailed information about a specific pod, including its events, status, and configurations.
Example: kubectl describe pod my-pod
#: kubectl describe pod my-pod Name: my-pod Namespace: my-namespace Priority: 0 Service Account: default Node: ip-172-31-26-203/172.31.26.203 Start Time: Mon, 16 Sep 2024 14:47:40 +0000 Labels: app=my-app Annotations: <none> Status: Running IP: 10.44.0.2 IPs: IP: 10.44.0.2
kubectl logs <pod-name>
Fetches logs from a specific pod, useful for debugging issues.
Example: kubectl logs my-pod
#: kubectl logs my-pod /docker-entrypoint.sh: /docker-entrypoint.d/ is not empty, will attempt to perform configuration /docker-entrypoint.sh: Looking for shell scripts in /docker-entrypoint.d/ /docker-entrypoint.sh: Launching /docker-entrypoint.d/10-listen-on-ipv6-by-default.sh 10-listen-on-ipv6-by-default.sh: info: Getting the checksum of /etc/nginx/conf.d/default.conf 10-listen-on-ipv6-by-default.sh: info: Enabled listen on IPv6 in /etc/nginx/conf.d/default.conf /docker-entrypoint.sh: Sourcing /docker-entrypoint.d/15-local-resolvers.envsh /docker-entrypoint.sh: Launching /docker-entrypoint.d/20-envsubst-on-templates.sh /docker-entrypoint.sh: Launching /docker-entrypoint.d/30-tune-worker-processes.sh /docker-entrypoint.sh: Configuration complete; ready for start up 2024/09/19 06:17:58 [notice] 1#1: using the "epoll" event method 2024/09/19 06:17:58 [notice] 1#1: nginx/1.27.1 2024/09/19 06:17:58 [notice] 1#1: built by gcc 12.2.0 (Debian 12.2.0-14) 2024/09/19 06:17:58 [notice] 1#1: OS: Linux 6.8.0-1015-aws 2024/09/19 06:17:58 [notice] 1#1: getrlimit(RLIMIT_NOFILE): 1048576:1048576 2024/09/19 06:17:58 [notice] 1#1: start worker processes 2024/09/19 06:17:58 [notice] 1#1: start worker process 29
kubectl exec <pod-name> -- <command>
Runs a command inside a running pod, similar to SSHing into a server.
Example: kubectl exec my-pod -- ls
#: kubectl exec my-pod -- ls bin boot dev docker-entrypoint.d docker-entrypoint.sh etc home lib lib64 media mnt opt proc root run sbin srv sys tmp usr var
Execute a command inside a running pod interactively:
kubectl exec <pod-name> -it -- <command>
Example: kubectl exec my-pod -it -- /bin/bash
#: kubectl exec my-pod -it -- /bin/bash #: ls -lrth total 64K drwxr-xr-x 2 root root 4.0K Aug 14 16:10 home drwxr-xr-x 2 root root 4.0K Aug 14 16:10 boot drwxr-xr-x 1 root root 4.0K Sep 4 09:00 var drwxr-xr-x 1 root root 4.0K Sep 4 09:00 usr drwxrwxrwt 2 root root 4.0K Sep 4 09:00 tmp drwxr-xr-x 2 root root 4.0K Sep 4 09:00 srv lrwxrwxrwx 1 root root 8 Sep 4 09:00 sbin -> usr/sbin drwxr-xr-x 2 root root 4.0K Sep 4 09:00 opt drwxr-xr-x 2 root root 4.0K Sep 4 09:00 mnt drwxr-xr-x 2 root root 4.0K Sep 4 09:00 media lrwxrwxrwx 1 root root 9 Sep 4 09:00 lib64 -> usr/lib64 lrwxrwxrwx 1 root root 7 Sep 4 09:00 lib -> usr/lib lrwxrwxrwx 1 root root 7 Sep 4 09:00 bin -> usr/bin -rwxr-xr-x 1 root root 1.6K Sep 4 23:10 docker-entrypoint.sh drwxr-xr-x 1 root root 4.0K Sep 4 23:11 docker-entrypoint.d dr-xr-xr-x 13 root root 0 Sep 19 06:17 sys dr-xr-xr-x 191 root root 0 Sep 19 06:17 proc drwxr-xr-x 1 root root 4.0K Sep 19 06:17 etc drwxr-xr-x 5 root root 360 Sep 19 06:17 dev drwxr-xr-x 1 root root 4.0K Sep 19 06:17 run drwx------ 1 root root 4.0K Sep 19 11:25 root
kubectl top pod <pod-name>
Shows CPU and memory usage of a pod.
Example: kubectl top pod my-pod
#: kubectl top pod my-pod NAME CPU(cores) MEMORY(bytes) my-pod 0m 4Mi
kubectl get pods -w
Watch Pod Status in Real-Time
Continuously monitor pod status changes, such as pods starting, terminating, or moving between states.
#: kubectl get pods -w NAME READY STATUS RESTARTS AGE my-pod 1/1 Running 2 (4h33m ago) 2d20h
kubectl delete pod <pod-name>
Deletes a pod from the cluster. The pod will be restarted if it’s part of a deployment or replica set.
Example: kubectl delete pod my-pod
#: kubectl get pods NAME READY STATUS RESTARTS AGE my-pod 1/1 Running 2 (5h9m ago) 2d20h #: kubectl delete pod my-pod pod "my-pod" deleted #: kubectl get pods No resources found in my-namespace namespace.
"Learning never exhausts the mind." — Leonardo da Vinci
Thank you, Happy Learning!
Subscribe to my newsletter
Read articles from Subbu Tech Tutorials directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
