How to Use PHP Callbacks: Examples and Guide
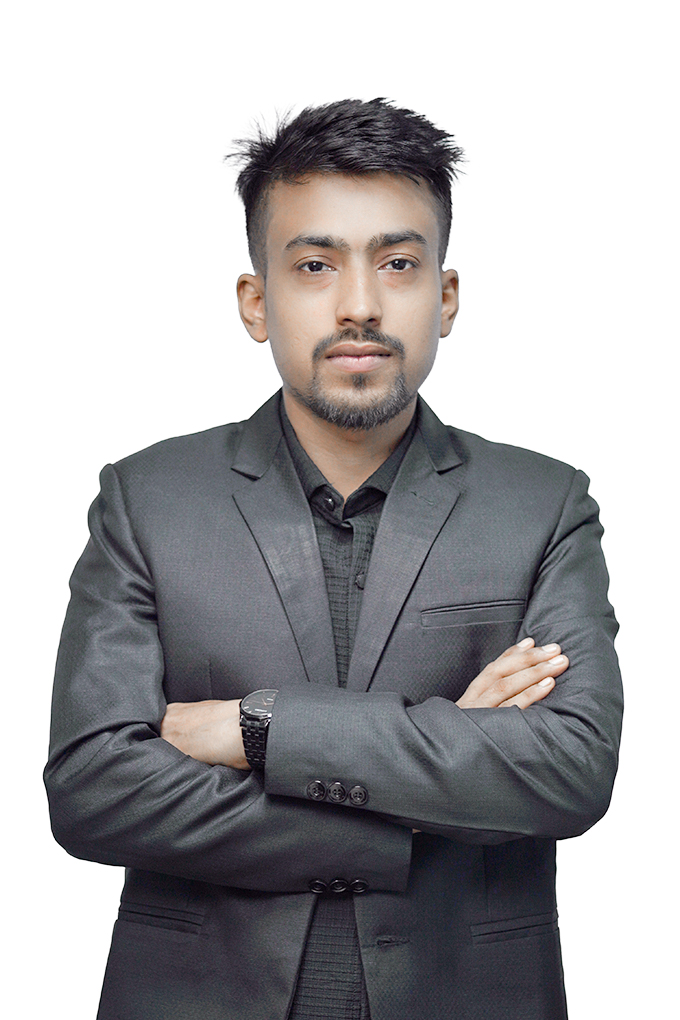
Introductions:
In PHP, callbacks are functions that are passed as arguments to other functions, allowing them to be called later, either immediately or at a specific point during execution. They are useful when you want to allow the behavior of a function to be customized without modifying its core logic.
In this blog, we’ll explore how callbacks work in PHP with practical examples, and discuss why and when you might use them in your applications.
What is a Callback in PHP?
A callback in PHP is essentially a way to pass a function (or method) to another function as an argument, allowing it to be invoked at a later time. Callbacks can be:
Functions
Static methods
Object methods
Anonymous functions (closures)
PHP provides several ways to handle callbacks, making them highly flexible.
Example 1: Passing a Function as a Callback
In PHP, you can pass a function as a callback using the function name as a string.
function sayHello($name) {
echo "Hello, $name!";
}
function greet($callback, $name) {
// Call the callback function
call_user_func($callback, $name);
}
greet('sayHello', 'John'); // Outputs: Hello, John!
In this example:
The
sayHello
function is passed as a string to thegreet
function.Inside
greet
,call_user_func
is used to invoke the callback, passing$name
as an argument.
Example 2: Using Anonymous Functions (Closures) as Callbacks
PHP also supports anonymous functions (also called closures), which are useful for one-time callback definitions.
function performOperation($a, $b, $callback) {
return $callback($a, $b);
}
$result = performOperation(5, 3, function($a, $b) {
return $a + $b;
});
echo $result; // Outputs: 8
Here, we pass an anonymous function as the callback that performs the addition of $a
and $b
.
Using Static and Object Methods as Callbacks
In PHP, both static methods and object methods can be used as callbacks.
Example 3: Static Method Callback
class Calculator {
public static function add($a, $b) {
return $a + $b;
}
}
function executeOperation($a, $b, $callback) {
return call_user_func($callback, $a, $b);
}
echo executeOperation(5, 3, ['Calculator', 'add']); // Outputs: 8
In this example, the static method Calculator::add
is passed as a callback, and call_user_func
invokes the method.
Example 4: Object Method Callback
class Greeter {
public function sayHello($name) {
echo "Hello, $name!";
}
}
$greeter = new Greeter();
function greetUser($callback, $name) {
call_user_func($callback, $name);
}
greetUser([$greeter, 'sayHello'], 'Alice'); // Outputs: Hello, Alice!
Here, we pass the instance method sayHello
of the Greeter
class as a callback.
Using Callbacks with Built-in PHP Functions
Several PHP built-in functions accept callbacks, making them very powerful for flexible programming. Common examples include array_map
, array_filter
, and usort
.
Example 5: array_map
The array_map
function allows you to apply a callback to each element in an array.
$numbers = [1, 2, 3, 4, 5];
$doubled = array_map(function($number) {
return $number * 2;
}, $numbers);
print_r($doubled); // Outputs: Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 )
In this example, an anonymous callback is used to double each value in the array.
Example 6: usort
The usort
function sorts an array using a user-defined comparison function.
$fruits = ['apple', 'banana', 'pear', 'orange'];
usort($fruits, function($a, $b) {
return strlen($a) - strlen($b);
});
print_r($fruits); // Outputs: Array ( [0] => pear [1] => apple [2] => banana [3] => orange )
Here, the anonymous function is used to sort the fruits by the length of their names.
Callbacks with Parameters
PHP supports passing additional parameters to the callback function through call_user_func_array
. This is useful when the callback requires more than just the arguments of the calling function.
Example 7: Callback with Parameters
function multiply($a, $b) {
return $a * $b;
}
function applyOperation($callback, ...$args) {
return call_user_func_array($callback, $args);
}
echo applyOperation('multiply', 4, 3); // Outputs: 12
In this example, we use call_user_func_array
to pass multiple arguments (4
and 3
) to the multiply
callback.
Practical Use Case: Hook System with Callbacks
Callbacks are often used in hook systems, where you want certain actions to be executed dynamically during an event. Here’s a simplified hook system using PHP callbacks.
Example 8: Implementing a Simple Hook System
class HookSystem {
private $hooks = [];
public function addHook($hookName, $callback) {
$this->hooks[$hookName][] = $callback;
}
public function runHook($hookName, ...$params) {
if (isset($this->hooks[$hookName])) {
foreach ($this->hooks[$hookName] as $callback) {
call_user_func_array($callback, $params);
}
}
}
}
$hooks = new HookSystem();
// Adding callbacks to a hook
$hooks->addHook('beforeSave', function($data) {
echo "Before saving: " . json_encode($data) . "\n";
});
// Running the hook
$data = ['name' => 'Alice', 'email' => 'alice@example.com'];
$hooks->runHook('beforeSave', $data);
In this example, the HookSystem
class allows you to add hooks and execute callbacks tied to those hooks. The beforeSave
hook is triggered before data is saved, and the callback can handle any necessary logic.
Conclusion
Callbacks in PHP provide a powerful way to write flexible, reusable, and dynamic code. By passing functions or methods as arguments, you can customize the behavior of your functions without changing their core logic. This technique is particularly useful when working with event-driven code, asynchronous operations, and higher-order functions like array_map
or usort
.
Understanding how to use callbacks effectively will enable you to write cleaner and more maintainable PHP code. Whether you’re passing anonymous functions, methods, or static functions, callbacks help you build more dynamic and reusable applications.
Subscribe to my newsletter
Read articles from Borhan Uddin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
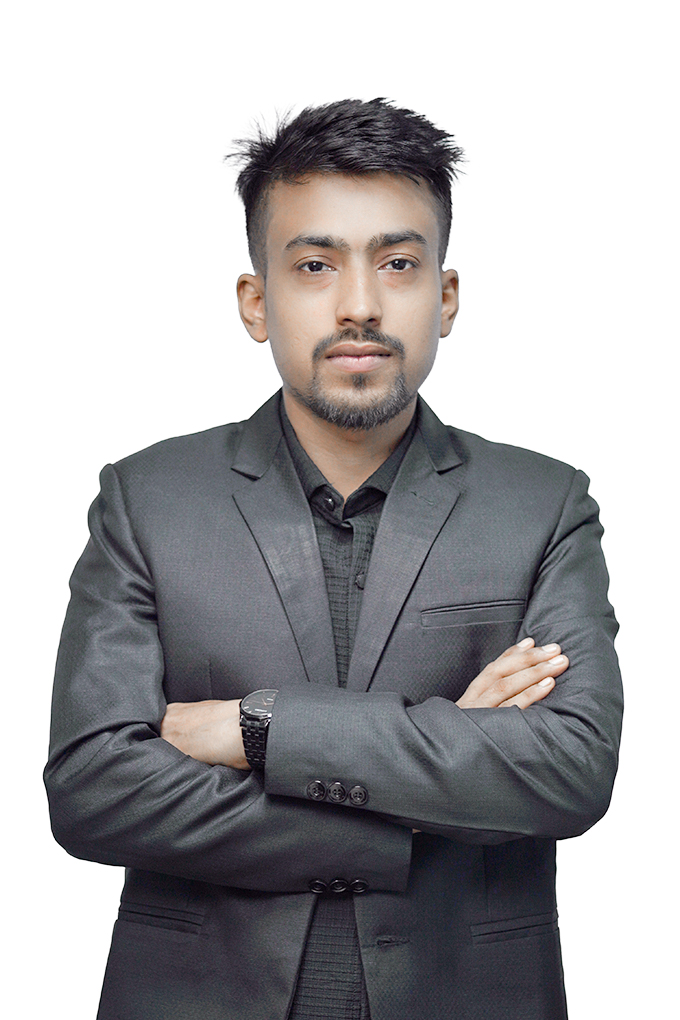
Borhan Uddin
Borhan Uddin
Experienced Full Stack Developer with 3 years of experience. Adept at creating efficient and user-friendly web applications. Strong problem-solving and teamwork skills. Committed to delivering high-quality results and continuously improving development practices.