3 Frameworks, 1 Simple Idea: Declarative UI

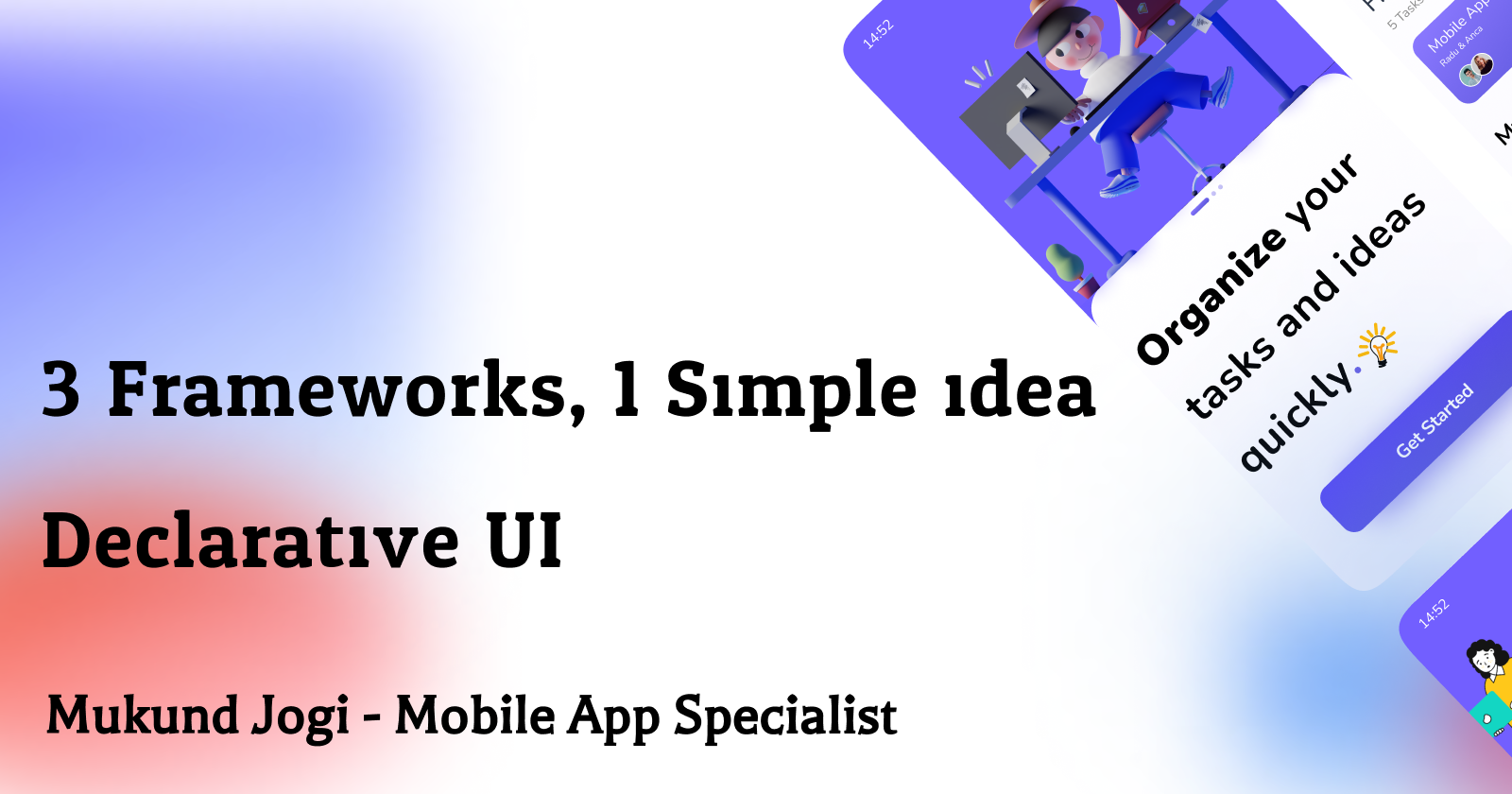
Imagine you're building a house. You could tell a construction crew exactly how to lay each brick, where to place every window, and how to connect the plumbing โ a very detailed and potentially messy approach!
This is how building mobile apps used to be. Developers had to meticulously instruct their apps on how to create every button, position each image, and update the screen whenever something changed. This "imperative" approach was tedious and prone to errors. It was like trying to build a house by giving instructions for every single nail and piece of wood - exhausting and complex!
Declarative UI takes a simpler approach. It's like showing a blueprint to the construction crew. You don't need to tell them how to build every part, you just show them the final design. The blueprint describes what you want the house to look like, and the crew handles all the details of building it.
Declarative UI for Apps
The same concept applies to building apps. Instead of telling the computer how to do everything, you describe what you want the app to look like and how it should behave. Frameworks, take this description and handle the complex details of creating and updating the UI.
Why It's Important:
This shift to declarative UI is revolutionizing mobile development. It offers several benefits:
Simplicity: Code becomes much easier to read and understand, as it directly reflects the app's visual design. It's like having a clear blueprint, making it easy to see how things are supposed to work.
Efficiency: Developers can focus on the core functionality of their apps, rather than getting bogged down in UI details. It's like letting the construction crew do their job, allowing the architect to focus on design and functionality.
Maintainability: Changes to the app's design or logic are easier to implement without introducing bugs. It's like being able to easily adjust the blueprint and see how those changes affect the final house.
The Future is Declarative:
Declarative UI isn't just a trend; it's a paradigm shift that's making mobile development more efficient, enjoyable, and leading to better user experiences.
Now, let's explore use of this declarative approach. We'll see how they make building apps simpler and more efficient, while also showcasing the unique strengths of each framework.
Three popular mobile frameworks โ Compose UI, SwiftUI, and Flutter โ use this declarative approach to make building apps easier and more efficient.
Let's break down how each framework works with a simple example:
Imagine we want to display a list of our favorite fruits:
Compose UI:
@Composable
fun FruitList() {
LazyColumn { // Like a scrollable list
items(fruits) { fruit -> // For each fruit in the list
Text(fruit) // Display the fruit name
}
}
}
SwiftUI:
struct FruitList: View {
let fruits = ["Apple", "Banana", "Orange"] // Our fruit list
var body: some View {
List(fruits) { fruit in // Similar to Compose's LazyColumn
Text(fruit) // Display the fruit name
}
}
}
Flutter:
class FruitList extends StatelessWidget {
final List<String> fruits = ["Apple", "Banana", "Orange"];
@override
Widget build(BuildContext context) {
return ListView.builder( // Like Compose's LazyColumn and SwiftUI's List
itemCount: fruits.length,
itemBuilder: (context, index) {
return Text(fruits[index]); // Display the fruit name
}
);
}
}
What's happening here?
Simple Code: We're simply defining the data (the fruits) and how to display them (as text).
Framework Does the Work: The frameworks handle all the details of creating the list, making it scrollable, and updating it when the list changes.
So, what makes each framework different?
Compose UI: It's designed specifically for Android, making it super-efficient and integrated with Android's tools.
SwiftUI: Made for Apple devices (iPhone, iPad, Mac, etc.), it offers a seamless experience within Apple's ecosystem.
Flutter: A cross-platform powerhouse, Flutter can build apps for Android, iOS, web, and even desktops, making it versatile for a wide range of projects.
Which one to choose?
Compose UI: If you're building for Android, Compose UI is a great choice.
SwiftUI: Stick with SwiftUI if you're focusing on Apple devices.
Flutter: For reaching the broadest audience, Flutter's cross-platform capabilities are unmatched.
At the end:
Declarative UI is revolutionizing mobile app development. By focusing on what you want, instead of how to achieve it, these frameworks make building beautiful and efficient apps much easier, even for beginners!
๐๐ If I am wrong or need to update any part of this explanation, please let me know. I would love to make the necessary changes. ๐๐
Keep clapping until your claps add up to 10.
Check out my previous series on Flutter interview questions for all levels. Take a look and share your thoughts.
https://mukundjogi.hashnode.dev/series/flutter-interview
Keep sharing ๐ซฐ๐ป, keep learning ๐๐ป. Practice these examples ๐ค๐ป.
Subscribe to my newsletter
Read articles from Mukund Jogi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mukund Jogi
Mukund Jogi
๐ Iโm from: Ahmedabad, Gujarat, India ๐ Designation: Project Manager (at 7Span) ๐ป Tech Stack: Flutter, Android , iOS ๐ง Let's connect: โฃ LinkedIn: https://linkedin.com/in/mukund-a-jogi/ โฃ Twitter: https://twitter.com/mukundjogi โฃ Medium: https://medium.com/@mukundjogi