Kotlin Hello World Program
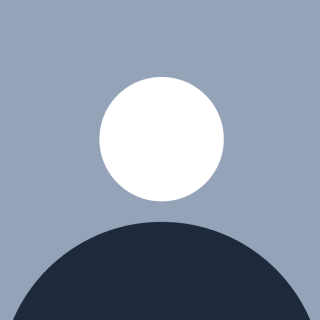
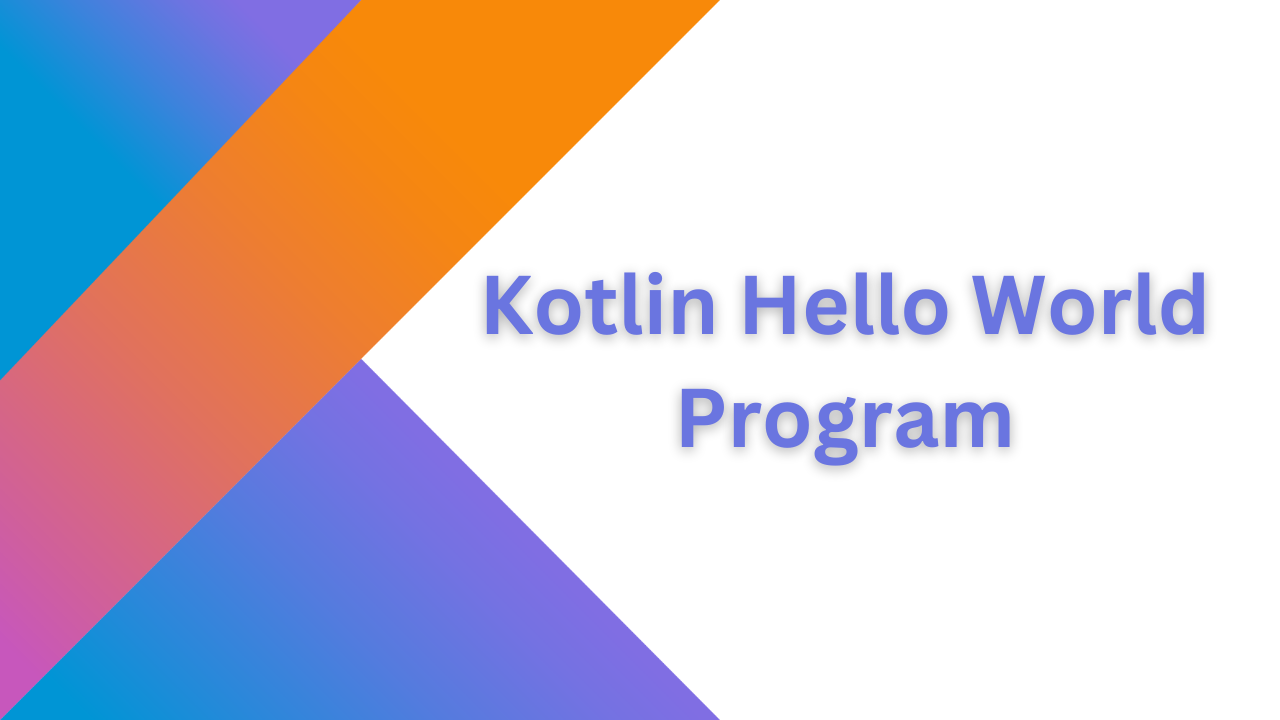
In this Kotlin tutorial, you're going to learn how to write and execute your first Kotlin program. Let's get started with IntelliJ IDEA. When developing Kotlin projects, you have several IDEs to choose from. One popular choice is IntelliJ IDEA, which has been closely associated with the development of the Kotlin programming language. In this tutorial, we’ll walk through the steps to set up a Kotlin/Java project using IntelliJ IDEA.
In addition to setting up a Kotlin project in IntelliJ IDEA, there’s another convenient way to quickly run Kotlin code: Kotlin Playground. It’s an online platform where you can write and execute Kotlin snippets without any local setup.
Prerequisites
Before we begin, ensure that you have IntelliJ IDEA installed on your computer. If you haven’t already, download the latest Community Edition from here and follow the installation instructions.
Start IntelliJ IDEA and Create a New Project
Start IntelliJ IDEA and Create a New Project:
Open IntelliJ IDEA and click on “Create New Project.”
In the left pane, choose “Kotlin.”
Under “Additional Libraries and Frameworks,” select “Kotlin.”
Click “Next.”
Specify Project Name and Location:
Give your project a name (e.g., “KotlinLearning”).
Choose a location for the project.
Click “Create.”
Create a New Kotlin File/Class:
If the Project window is not visible, press Alt+1.
Expand the project structure, and under “src,” right-click on the “src” folder.
Choose “New” > “Kotlin File/Class.”
Name the file (e.g., “Main”) and click “OK.”
Write the Main Method in the Kotlin File/Class:
Inside the newly created Kotlin file, write your main method.
Run the project by clicking “Run” > “Run.”
You’ll see the “Hello, world!” message printed to the output.
Inside the newly created Kotlin file, write your main method:
// Hello World Program
fun main() {
println("Hello, world!")
}
Run the Project
Click on the “Run” button in the toolbar or press Shift+F10 (on Mac, it’s Control+R).
IntelliJ IDEA will build your application, and you will see the “Hello, world!” message printed in the terminal window.
Hello World
All Kotlin programs start at the main
function within a package.
package my.program
fun main(args: Array<String>) {
println("Hello, world!")
}
Place the above code into a file named Main.kt
. When targeting the JVM, the function will be compiled as a static method in a class with a name derived from the filename. In this example, the main class to run would be my.program.MainKt
.
To change the name of the class that contains top-level functions for a particular file, use the @file:JvmName("MyApp")
annotation at the top of the file above the package statement. The main class to run would now be my.program.MyApp
.
Hello World using a Companion Object
You can define the main
function of a Kotlin program using a Companion Object of a class:
package my.program
class App {
companion object {
@JvmStatic fun main(args: Array<String>) {
println("Hello World")
}
}
}
The class name to run is my.program.App
. This method makes the class name more self-evident, and any other functions you add are scoped into the class App
.
A variation that instantiates the class to do the actual "hello":
class App {
companion object {
@JvmStatic fun main(args: Array<String>) {
App().run()
}
}
fun run() {
println("Hello World")
}
}
Hello World using an Object Declaration
Alternatively, use an Object Declaration that contains the main function:
package my.program
object App {
@JvmStatic fun main(args: Array<String>) {
println("Hello World")
}
}
The class name to run is my.program.App
. This method provides a singleton instance of App
to store state and perform other tasks.
Main Methods using Varargs
All main method styles can also be used with varargs:
package my.program
fun main(vararg args: String) {
println("Hello, world!")
}
Compile and Run Kotlin Code in Command Line
Kotlin provides commands similar to Java for compiling and running code:
Use
kotlinc
to compile Kotlin files.Use
kotlin
to run Kotlin files.
Reading Input from Command Line
Arguments passed from the console can be used as input in a Kotlin program. Here's an example:
fun main(args: Array<String>) {
println("Enter Two numbers")
val (a, b) = readLine()!!.split(' ')
println("Max number is: ${maxNum(a.toInt(), b.toInt())}")
}
fun maxNum(a: Int, b: Int): Int {
return if (a > b) {
println("The value of a is $a")
a
} else {
println("The value of b is $b")
b
}
}
Enter two numbers from the command line to find the maximum number. For example:
Enter Two numbers
71 89
The value of b is 89
Max number is: 89
Note: The above example can be compiled and run on IntelliJ IDEA.
Conclusion
Congratulations! You've successfully written and executed your first Kotlin program. This simple "Hello, world!" program is a great starting point for learning Kotlin. As you progress, you'll learn more about Kotlin's syntax, functional programming features, and how to build more complex applications. In the next tutorial, we’ll explore basic Kotlin programming concepts. The Kotlin tutorial page lists down all the important topics you can go through to get a deeper understanding of the language basics and advanced concepts.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer