C++ Compiler, Linker, and Loader: How C++ Work?
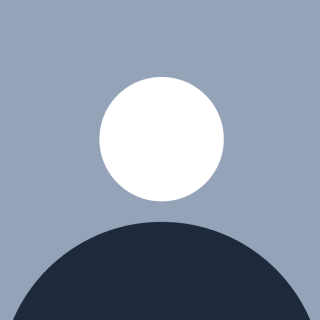
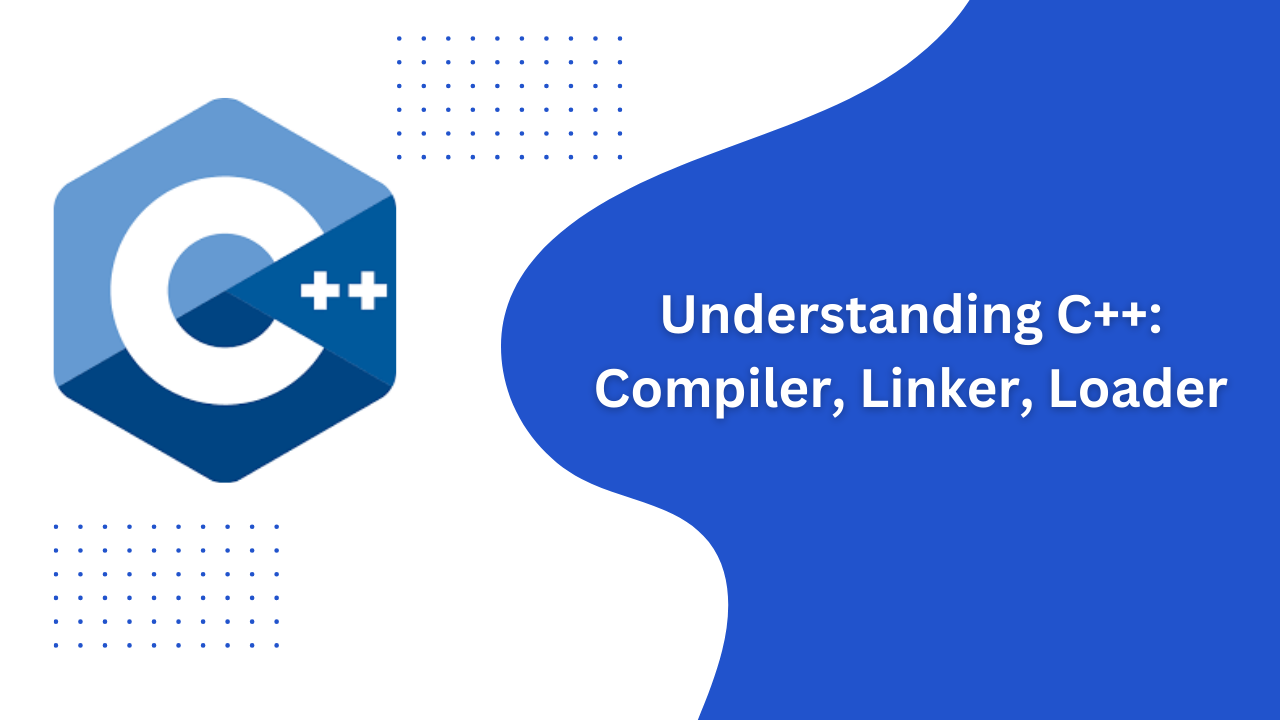
C++ is a powerful programming language that enables developers to create high-performance applications. Understanding how C++ works involves knowing the process from writing source code to generating an executable binary. This transformation includes several stages: preprocessing, compiling, and linking.
Preprocessor Statements
When you write a C++ program, you often start with preprocessor statements. These statements begin with a hash symbol (#) and are processed before the actual compilation. For example, #include <iostream>
tells the preprocessor to include the contents of the iostream file, which is necessary for input and output operations.
Preprocessor Directives: These are instructions that are executed before the actual compilation of the code begins. They are used for including files, defining constants, and macros, among other things.
Common Directives:
#include
: Used to include the contents of a file. For example,#include <iostream>
includes the standard input-output stream library.#define
: Used to define macros or constants. For example,#define PI 3.14
defines a constant PI with a value of 3.14.#ifdef
,#ifndef
,#endif
: Used for conditional compilation. These directives allow you to compile certain parts of the code only if specific conditions are met.
Entry Point
Every C++ program has a main
function, which serves as the entry point. When you run your application, the execution starts from the main
function. The code inside the main
function is executed line by line, unless control flow statements or function calls alter the order.
Function Signature: The
main
function typically has one of the following signatures:int main()
int main(int argc, char* argv[])
The first version is the simplest form, while the second version allows command-line arguments to be passed to the program.
Return Type: The
main
function returns an integer value. By convention, returning 0 indicates that the program executed successfully, while returning a non-zero value indicates an error.Execution Flow: The statements inside the
main
function are executed sequentially. However, control flow statements like loops (for
,while
), conditionals (if
,switch
), and function calls can alter this sequence.
Compilation Process
The compilation process involves several stages:
Preprocessing: The preprocessor handles all preprocessor directives, such as
#include
and#define
. It essentially prepares the code for the next stage by including header files and expanding macros.Compilation: The compiler translates the preprocessed C++ code into machine code. This machine code is stored in object files with a
.obj
extension.Linking: The linker takes all the object files and combines them into a single executable file. It resolves references between different object files, ensuring that function calls and variable references are correctly linked.
The Compilation Process
Writing Source Code: Start with
.cpp
files.Preprocessing: Handles directives like
#include
.Compilation: Translates code into object code.
Linking: Combines object code with libraries.
Execution: Runs the final executable.
Step 1: Writing Source Code
The process begins with writing the source code in C++. This code is typically stored in files with a .cpp
extension.
Copy
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Step 2: Preprocessing
The preprocessing stage in C++ is the first step in the compilation process. It involves handling preprocessor directives, which are special instructions given to the preprocessor. These directives begin with a hash symbol (#) and are used to include files, define constants, and perform conditional compilation, among other tasks.
File Inclusion (
#include
):The
#include
directive is used to include the contents of another file into the current file. This is commonly used to include header files that contain declarations of functions and variables.For example,
#include <iostream>
includes the standard input-output stream library, which is necessary for usingstd::cout
andstd::cin
.The preprocessor replaces the
#include
directive with the actual contents of the specified file.
Macro Definition (
#define
):The
#define
directive is used to define macros, which are essentially constants or functions that are replaced by their values or code snippets during preprocessing.For example,
#define PI 3.14
defines a constantPI
with a value of 3.14. WheneverPI
is encountered in the code, it is replaced with 3.14.Macros can also take arguments, acting like inline functions. For example,
#define SQUARE(x) ((x) * (x))
defines a macro that calculates the square of a number.
Conditional Compilation (
#ifdef
,#ifndef
,#endif
):These directives allow parts of the code to be compiled only if certain conditions are met. This is useful for including or excluding code based on specific conditions, such as debugging or platform-specific code.
For example:
#ifdef DEBUG std::cout << "Debug mode" << std::endl; #endif
The code inside the
#ifdef
block is compiled only ifDEBUG
is defined.
Other Preprocessor Directives:
#undef
: Used to undefine a macro.#pragma
: Provides additional information to the compiler, such as optimization settings or warnings.#error
: Generates a compilation error with a specified message.#line
: Changes the line number and filename for error reporting.
Comments Removal:
- The preprocessor removes all comments from the source code. This includes both single-line comments (
//
) and multi-line comments (/* ... */
).
- The preprocessor removes all comments from the source code. This includes both single-line comments (
Token Replacement:
- The preprocessor performs token replacement, where it replaces all instances of macros with their defined values or code snippets.
Output:
- The result of preprocessing is an expanded source code file, where all preprocessor directives have been processed and replaced. This expanded file is then passed to the next stage of compilation.
Step 3: Compilation
The compiler translates the preprocessed source code into object code. This involves parsing the code, performing semantic analysis, optimizing the code, and generating the object code.
This stage involves several steps:
Parsing:
Lexical Analysis: The compiler reads the preprocessed source code and breaks it down into tokens. Tokens are the smallest units of meaning, such as keywords, operators, identifiers, and literals.
Syntax Analysis: The compiler checks the sequence of tokens against the grammatical rules of the C++ language. This step ensures that the code follows the correct syntax. The output of this step is a parse tree or abstract syntax tree (AST), which represents the hierarchical structure of the source code.
Semantic Analysis:
Type Checking: The compiler verifies that the operations in the code are semantically correct. For example, it checks that variables are used in a manner consistent with their data types.
Scope Resolution: The compiler ensures that variables and functions are used within their defined scopes. It checks for issues like variable shadowing and ensures that all identifiers are declared before use.
Symbol Table Management: The compiler maintains a symbol table that keeps track of all identifiers (variables, functions, classes, etc.) and their attributes (type, scope, etc.).
Intermediate Code Generation:
- The compiler translates the AST into an intermediate representation (IR). This IR is a lower-level code that is easier to optimize and translate into machine code. It is often platform-independent, allowing for more flexible optimization.
Optimization:
Local Optimization: The compiler performs optimizations within a single basic block or function. Examples include constant folding (evaluating constant expressions at compile time) and dead code elimination (removing code that will never be executed).
Global Optimization: The compiler performs optimizations across multiple functions or the entire program. Examples include inlining (replacing a function call with the function's body) and loop unrolling (expanding loops to reduce the overhead of loop control).
Machine-Dependent Optimization: The compiler tailors the code to take advantage of specific features of the target machine's architecture, such as specialized instructions or registers.
Code Generation:
The compiler translates the optimized IR into machine code specific to the target architecture. This machine code is a set of instructions that the computer's CPU can execute directly.
The output of this step is an object file, which contains the machine code along with additional information needed for linking, such as symbol definitions and references.
Output:
- The final output of the compilation stage is one or more object files, typically with a
.obj
or.o
extension. These object files are then passed to the linking stage to produce the final executable.
- The final output of the compilation stage is one or more object files, typically with a
Step 4: Linking
The linker combines the object code with any necessary libraries to create an executable file. This involves resolving symbols, relocating addresses, and linking libraries.
The linking process:
Combining Object Files:
During the compilation stage, each source file is compiled into an object file. These object files contain machine code but are not yet complete programs.
The linker takes all these object files and combines them into a single executable file.
Resolving Symbols:
Symbols are names for functions, variables, and other entities in your code.
During linking, the linker resolves these symbols by matching function calls and variable references to their definitions.
If a symbol used in one object file is defined in another, the linker ensures that the reference is correctly linked to the definition.
Relocating Addresses:
Object files contain machine code with addresses that are relative to the start of the file.
The linker adjusts these addresses so that they are correct in the context of the final executable.
This process is known as relocation and involves updating the addresses of functions, variables, and other entities to their final locations in memory.
Linking Libraries:
Libraries are collections of precompiled code that can be used by your program.
There are two types of libraries: static and dynamic.
Static Libraries: These are linked at compile time. The code from the static library is copied into the final executable.
Dynamic Libraries: These are linked at runtime. The final executable contains references to the dynamic library, which is loaded into memory when the program runs.
The linker includes the necessary code from static libraries and ensures that references to dynamic libraries are correctly set up.
Handling External Dependencies:
If your program depends on external libraries, the linker ensures that these dependencies are correctly resolved.
This may involve specifying the paths to the libraries and ensuring that the correct versions are used.
Generating the Executable:
After resolving symbols, relocating addresses, and linking libraries, the linker generates the final executable file.
This file is a complete program that can be run on the target machine.
Error Handling:
During the linking process, errors can occur if symbols cannot be resolved or if there are conflicts between different object files or libraries.
The linker provides error messages to help you identify and fix these issues.
Libraries
Static Libraries | Linked at compile time |
Dynamic Libraries | Linked at runtime |
Step 5: Execution
The final executable file can be run on the computer. If dynamic libraries are used, they are loaded into memory at runtime.
The final executable file, which is the result of the compilation and linking stages, can now be run on the computer. This stage involves several key processes:
Loading the Executable:
When you run the executable file, the operating system loads it into memory. This involves reading the file from disk and placing its code and data into the appropriate memory locations.
The operating system also sets up the execution environment, which includes allocating memory for the program's stack and heap, and setting up the initial state of the CPU registers.
Dynamic Libraries:
If the executable depends on dynamic libraries (also known as shared libraries or DLLs in Windows), these libraries are loaded into memory at runtime.
The operating system locates the required dynamic libraries, loads them into memory, and resolves any references to functions or variables in these libraries.
This process is known as dynamic linking, and it allows multiple programs to share the same library code, reducing memory usage and disk space.
Relocation:
During the loading process, the operating system may need to perform additional relocation. This involves adjusting the addresses of functions and variables to reflect their actual locations in memory.
This step is necessary because the executable file and dynamic libraries may not be loaded at the same memory addresses every time the program runs.
Initialization:
Before the
main
function is called, the runtime environment performs several initialization tasks. This includes setting up global variables, initializing static objects, and executing any code in the program's initialization sections.In C++, constructors for global and static objects are called during this phase.
Execution of
main
Function:After initialization, the operating system transfers control to the
main
function of the program. This is the entry point of the program, and the code inside themain
function is executed.The
main
function can take command-line arguments, which are passed to it by the operating system. These arguments can be used to control the behavior of the program.
Runtime Behavior:
During execution, the program may perform various tasks such as reading input, processing data, and producing output. It may also interact with the operating system through system calls to perform tasks like file I/O, network communication, and memory management.
The program's behavior is determined by the code written by the developer, and it can include loops, conditionals, function calls, and other control flow constructs.
Termination:
When the
main
function returns, the program terminates. The return value of themain
function is passed back to the operating system, which can use it to determine the success or failure of the program.Before the program fully terminates, the runtime environment performs cleanup tasks. This includes calling destructors for global and static objects, releasing allocated memory, and closing any open files or network connections.
Exit Status:
The exit status of the program is an integer value returned by the
main
function. By convention, a return value of 0 indicates successful execution, while a non-zero value indicates an error or abnormal termination.The operating system can use the exit status to determine the outcome of the program and take appropriate actions if necessary.
Compiler Settings
In an Integrated Development Environment (IDE) like Visual Studio, you can configure various settings that affect the compilation process. These settings include optimization levels, target platforms (e.g., x86 or x64), and whether to build in debug or release mode.
Multiple Source Files
In larger projects, you often split your code into multiple source files. Each source file is compiled into an object file. The linker then combines these object files into a single executable. To ensure that functions and variables are recognized across different files, you use declarations and definitions. A declaration tells the compiler that a function or variable exists, while a definition provides the actual implementation.
Error Handling
During the compilation and linking stages, you may encounter errors. Syntax errors are caught during compilation, while unresolved references are caught during linking. IDEs provide tools to help you identify and fix these errors.
Conclusion
C++ is a compiled language that transforms source code into machine code through preprocessing, compiling, and linking stages. The process starts with preprocessor directives, followed by the main
function as the entry point. The compilation involves syntax and semantic analysis, intermediate code generation, optimization, and machine code generation. The linker then resolves references and combines object files into an executable. IDE settings and handling multiple source files are crucial for larger projects. Errors are caught during compilation and linking stages.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer