C++ Naming Conventions
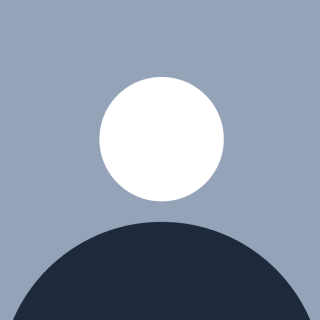
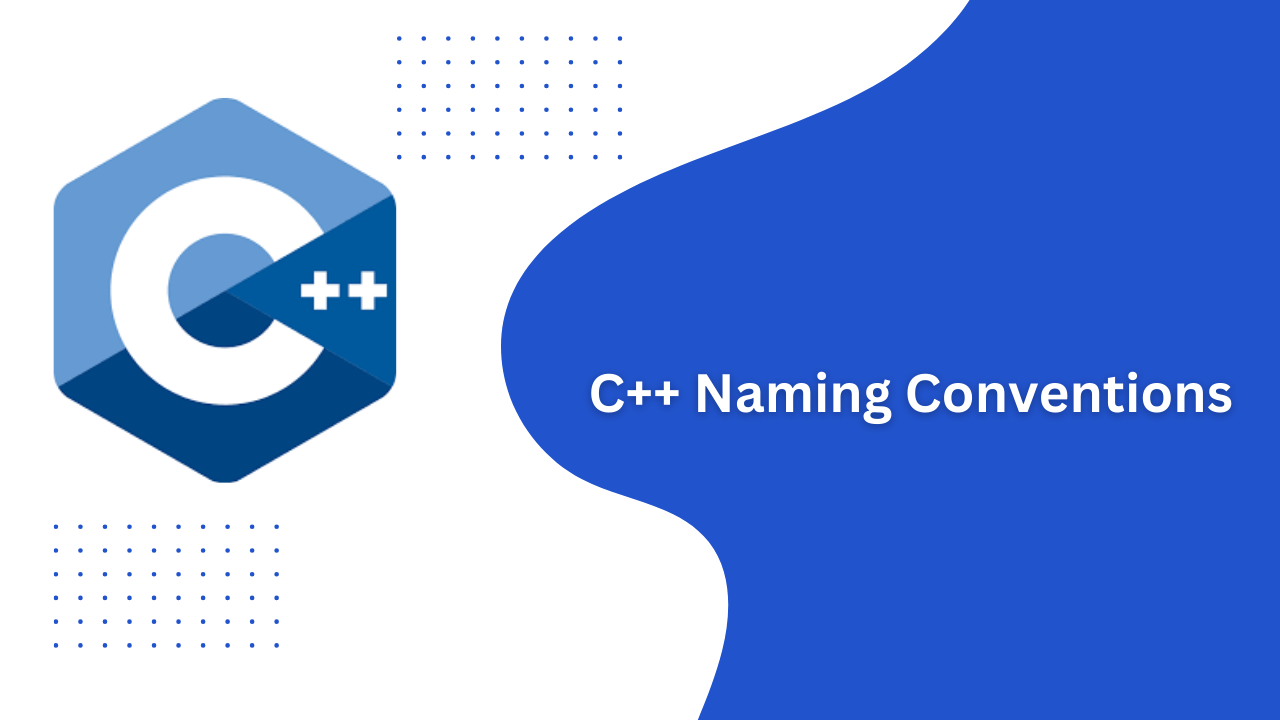
Naming conventions are essential guidelines for writing consistent and readable code. When working with C++, following these conventions ensures that your code remains clear and maintainable.
Namespace Names
Namespace names should be in all lowercase letters. If the name consists of multiple words, separate them with underscores.
Example:
namespace my_project {
namespace utilities {
}
}
Class Names
Class names should be nouns in PascalCase (first letter of each word capitalized).
Example:
class ArrayList {
};
class Employee {
};
class Record {
};
class Identity {
};
Interface Names
Interface names should be nouns or adjectives in PascalCase. Prefix interface names with an "I" to distinguish them from classes.
Example:
class ISerializable {
};
class ICloneable {
};
class IIterable {
};
class IList {
};
Method Names
Method names should be verbs or verb phrases in camelCase (first letter lowercase, subsequent words capitalized).
Example:
class Employee {
public:
void getId();
void remove(Object o);
void update(Object o);
Report getReportById(long id);
Report getReportByName(const std::string& name);
};
Variable Names
Variable names (instance, static, and method parameters) should be in camelCase. Choose descriptive names that convey their purpose.
Example:
class Employee {
private:
long id;
EmployeeDao employeeDao;
Properties properties;
};
Constant Naming Conventions
Constants should be in all uppercase with words separated by underscores. Use the const
or constexpr
keyword for constant variables.
Example:
const std::string SECURITY_TOKEN = "...";
const int INITIAL_SIZE = 16;
const int MAX_SIZE = INT_MAX;
Naming Generic Types
Generic type parameter names should consist of uppercase single letters. The letter ‘T’ (for type) is commonly recommended.
Example:
template <typename T>
class MyGenericClass {
};
template <typename K, typename V>
class Map {
};
template <typename E>
class List : public Collection<E> {
};
Naming Enums
Enumeration names should be in PascalCase, while enumeration values should be in all uppercase letters with words separated by underscores.
Example:
enum Direction {
NORTH,
EAST,
SOUTH,
WEST
};
Naming Annotations
Annotation names follow PascalCase notation. They can be adjectives, verbs, or nouns based on their purpose.
Example:
[[deprecated]]
void oldFunction();
[[nodiscard]]
int computeValue();
Summary
Class Names: Should be nouns in PascalCase (e.g.,
PerimeterRectangle
). No underscores are allowed. Private attributes should be prefixed with 'm' (e.g.,mLength
).Function Names: Should start with a verb and use camelCase (e.g.,
getValue()
). Function arguments should start with a lowercase letter and use camelCase (e.g.,lengthRectangle
).Variable Names: Should start with an alphabet, can include digits but no special symbols except underscores. Pointer variables should be prefixed with 'p' (e.g.,
*pName
), reference variables with 'r', and static variables with 's'.Constants: Should be in all uppercase letters with underscores separating words (e.g.,
TWO_PI
).File Names: Should end with
.c
or.cpp
and can include underscores or dashes but no other special characters. Avoid using names that conflict with standard library headers.
Conclusion
In this article, we’ve discussed essential naming conventions in C++. Consistently applying these conventions ensures that your code remains readable and maintainable. Following these guidelines will help you write clean and professional C++ code.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer