Java Naming conventions
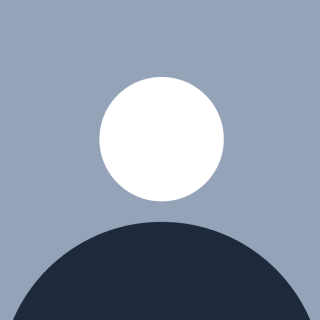
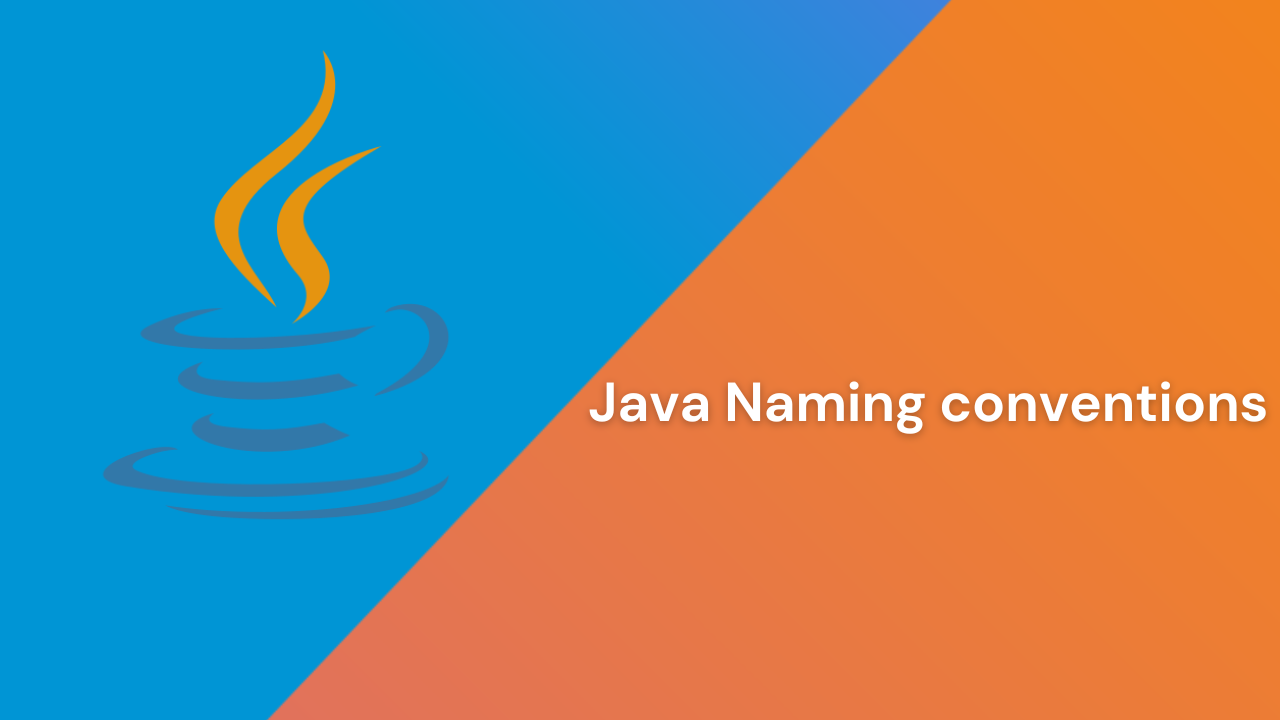
Naming conventions are essential guidelines for writing consistent and readable code. When working with Java, following these conventions ensures that your code remains clear and maintainable. Let’s explore some commonly used naming conventions in Java:
Package Names
Package names should start with lowercase domain names (e.g., com, org, net).
Subsequent parts of the package name can vary based on your organization’s internal conventions.
Example:
package com.dilip.webapp.controller;
package com.company.myapplication.web.controller;
package com.google.search.common;
Class Names
Class names should be nouns in title case (first letter of each separate word capitalized).
Example:
class ArrayList;
class Employee;
class Record;
class Identity;
Interface Names
Interface names should be adjectives or, in some cases, nouns representing a family of classes.
Use title case for interface names.
Example:
interface Serializable;
interface Clonable;
interface Iterable;
interface List;
Method Names
Method names should be verbs, clearly indicating the action they perform.
Use camel case notation for method names.
Example:
public Long getId();
public void remove(Object o);
public void update(Object o);
public Report getReportById(Long id);
public Report getReportByName(String name);
Variable Names
Variable names (instance, static, and method parameters) should be in camel case.
Choose descriptive names that convey their purpose.
Example:
private Long id;
private EmployeeDao employeeDao;
private Properties properties;
Constant Naming Conventions
Constants should be in all uppercase with words separated by underscores.
Use the final modifier for constant variables.
Example:
public static final String SECURITY_TOKEN = "...";
public static final int INITIAL_SIZE = 16;
public static final int MAX_SIZE = Integer.MAX_VALUE;
Naming Generic Types
Generic type parameter names should consist of uppercase single letters.
The letter ‘T’ (for type) is commonly recommended.
In JDK classes:
E is used for collection elements.
S is used for service loaders.
K and V are used for map keys and values.
Example:
class MyGenericClass<T> {}
interface Map<K, V> {}
interface List<E> extends Collection<E> {}
<E> Iterator<E> iterator();
Naming Enums
Similar to class constants, enumeration names should be in all uppercase letters.
Example:
public enum Direction { NORTH, EAST, SOUTH, WEST }
Naming Annotations
Annotation names follow title case notation.
They can be adjectives, verbs, or nouns based on their purpose.
Example:
@interface FunctionalInterface {}
@interface Deprecated {}
@interface Documented {}
@Async
@interface MyAsyncAnnotation {}
@Test
@interface MyTestAnnotation {}
Conclusion
In this article, we’ve discussed essential naming conventions in Java. Consistently applying these conventions ensures that your code remains readable and maintainable. The Java documentation provides a comprehensive guide to all the important topics you can explore to get a deeper understanding of the language basics and advanced concepts.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer