Understanding JavaScript hoisting: Functions and variables with the same name

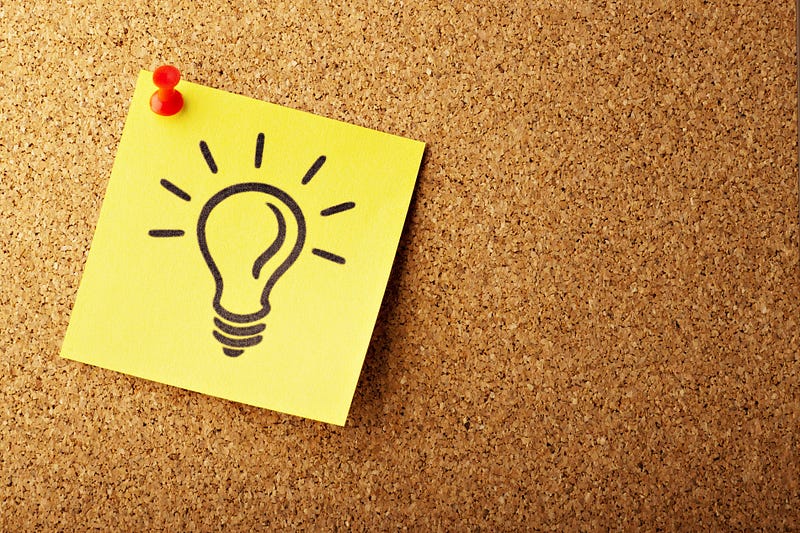
How does hoisting work when you declare function and variable with the same name in JavaScript? Which one takes precedence?
Variable declaration vs function declaration
Test your knowledge: which value will be printed in console — variable, or function?
(function() {
function test() {}
var test;
console.log('test is: ', test);
})();
The answer is:
test is: ƒ test() {}
It occurs because function declaration takes precedence over variable declaration.
Variable assignment vs function declaration
Now, let’s look at another example: what value will be printed in this case?
(function() {
var test = 5;
function test() {}
console.log('test is: ', test);
})();
The answer is:
test is: 5
The reason is that variable assignment takes precedence over function declaration.
Note that the order of assigning test
variable and declaring test
function in the last example doesn’t matter. If you reverse them, the result will still be same.
It happens because JavaScript first declares the variables and functions in the given scope and then initializes them with the provided values. Therefore, regardless of the declaration sequence, in the console we will see the value assigned to the test
variable, i.e. - 5
.
Thanks for reading! If you have any notes, remarks or questions, please share them in the comments.
Stay updated with the latest JavaScript and software development news! Join my Telegram channel for more insights and discussions: TechSavvy: Frontend & Backend.
Subscribe to my newsletter
Read articles from Vardan Hakobyan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vardan Hakobyan
Vardan Hakobyan
Hi, I'm a seasoned software engineer with a strong focus on JavaScript. I'm passionate about sharing my knowledge and experiences to help others learn something new and avoid the same pitfalls I've encountered along the way.