Maximizing Performance in MongoDB: Best Practices for High-Performance Data Transformation and Analysis Using Aggregation Pipelines
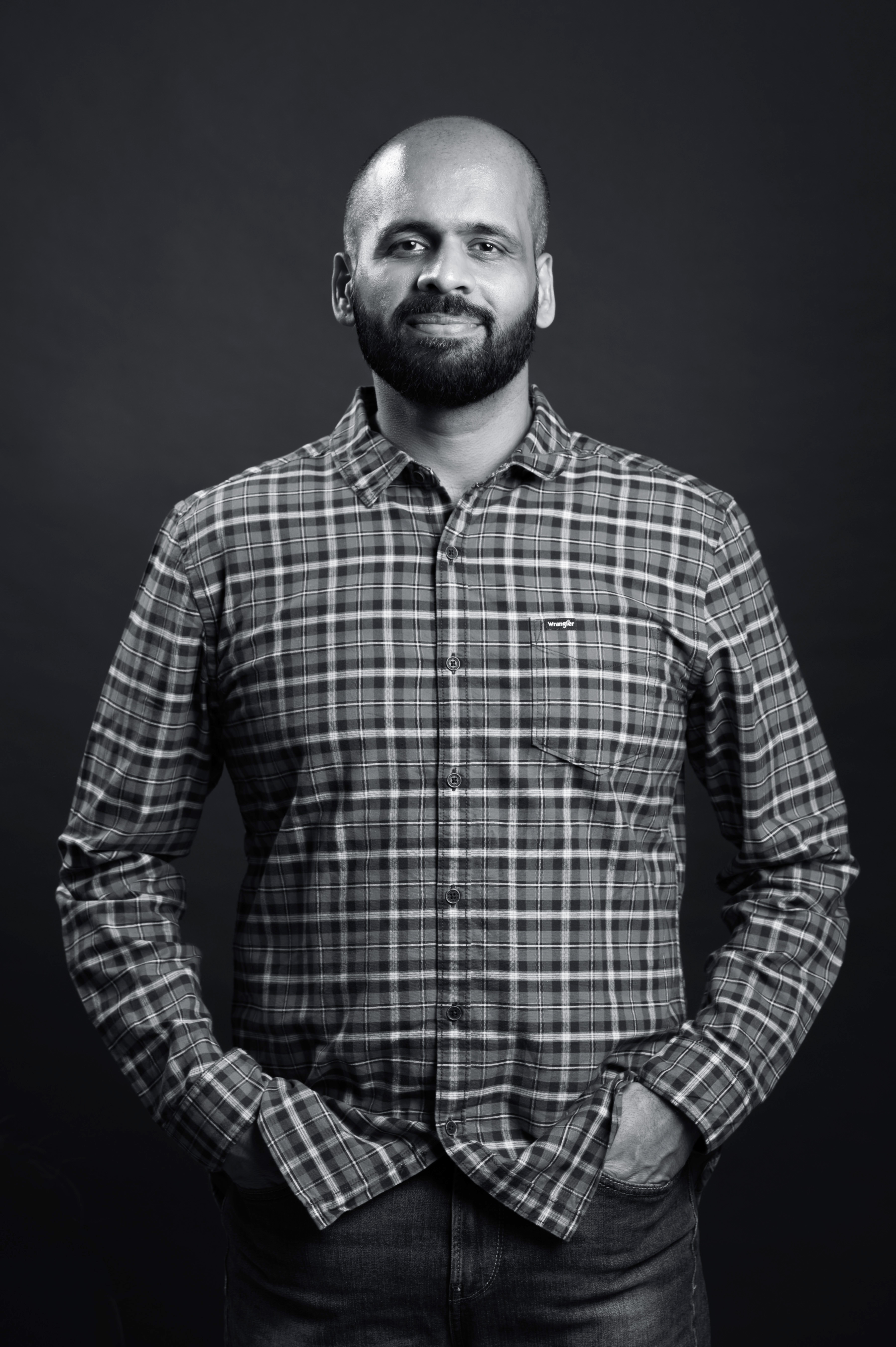
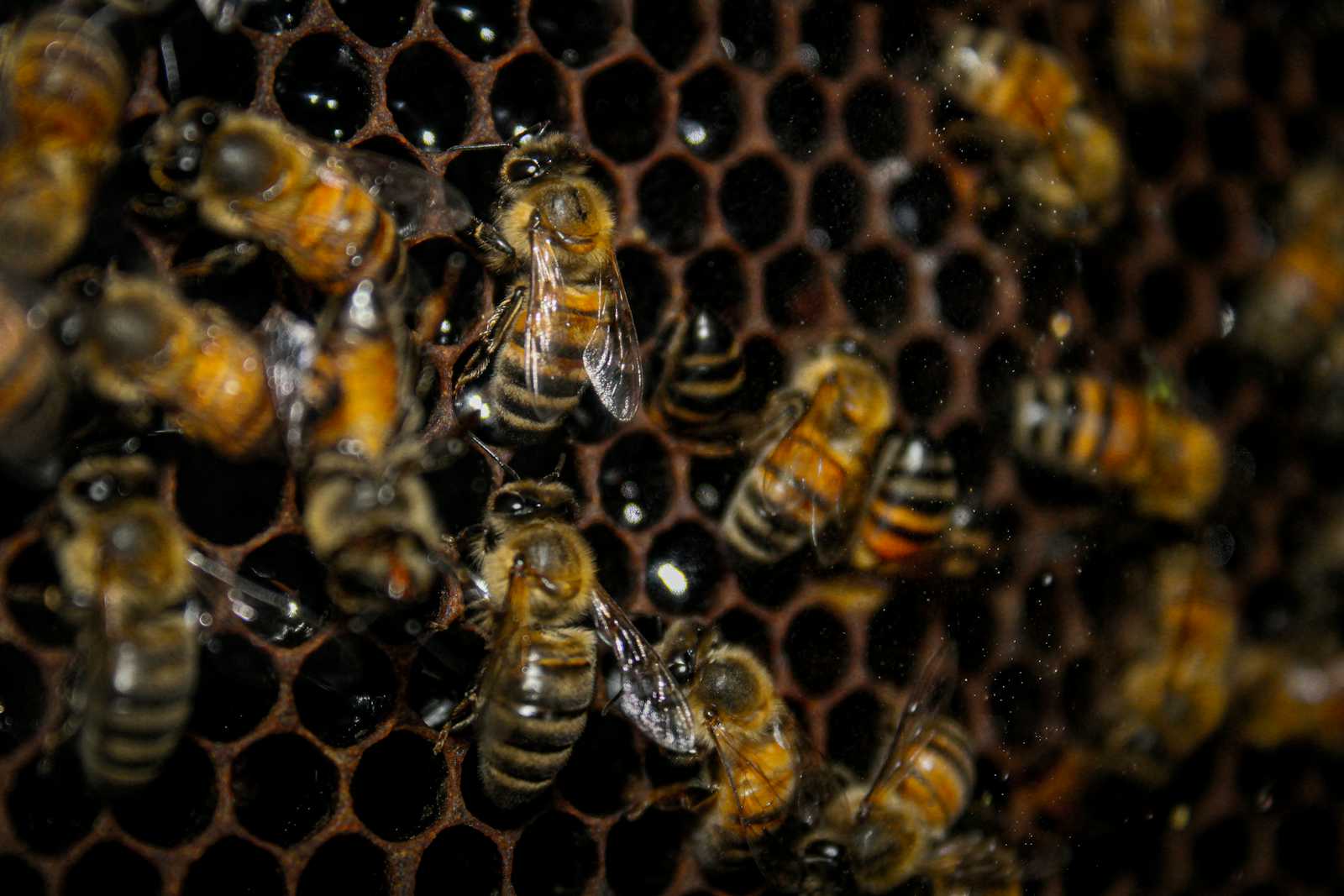
Using aggregation pipelines in MongoDB is an efficient way to perform data transformation and analysis directly within the database, which can result in significant performance improvements by leveraging MongoDB's optimized data processing capabilities. Aggregation pipelines allow for complex operations such as filtering, grouping, transforming, and reshaping documents in collections with minimal overhead.
To maximize performance when using MongoDB's aggregation framework, follow these best practices and strategies:
1. Structure the Aggregation Pipeline Efficiently
MongoDB aggregation pipelines are composed of stages that process the data in sequence. Each stage performs an operation on the documents and passes the results to the next stage.
Key stages for high-performance data transformations include:
$match
: Filters documents. Always place this stage early in the pipeline to minimize the number of documents that need to be processed by subsequent stages.$project
: Reshapes each document by specifying or adding fields. Use it to eliminate unnecessary fields to reduce data load as early as possible.$group
: Groups documents by a specified key and applies accumulators (likesum
,avg
,count
).$sort
: Sorts documents by a field. This can be expensive if not properly indexed.$limit
: Limits the number of documents passed to the next stage, which can improve performance if large datasets are involved.
Example:
db.orders.aggregate([
{ $match: { status: "complete" } }, // Filter first to reduce document set
{ $group: { _id: "$customerId", totalSpent: { $sum: "$amount" } } },
{ $sort: { totalSpent: -1 } }, // Sort based on total spent
{ $limit: 5 } // Limit to top 5 customers
])
Best Practice: Use match
, project
, and limit
as early as possible in the pipeline to reduce the number of documents processed.
2. Leverage Indexes for Performance
MongoDB aggregation can take advantage of indexes to speed up pipeline stages. To improve performance, ensure that:
Fields used in
$match
and$sort
stages are indexed. MongoDB will use indexes to quickly retrieve the filtered data or perform fast sorting.Compound indexes (multi-field indexes) can be used if multiple fields are involved in filtering and sorting.
Example:
If you frequently query by status
and sort by date
, you can create a compound index:
db.orders.createIndex({ status: 1, date: -1 });
Best Practice: Use indexes that align with the fields in $match
and $sort
stages to improve query performance.
3. Use $lookup
Carefully
The $lookup
stage is used for performing joins between collections in MongoDB. While it is powerful, it can be resource-intensive if not used carefully, especially with large collections.
Strategies for Optimizing $lookup
:
Ensure that the foreign field in the collection being joined has an index to speed up lookups.
Consider reducing the dataset with
$match
before using$lookup
.If possible, denormalize your data to avoid excessive use of
$lookup
.
Example:
db.orders.aggregate([
{ $match: { status: "complete" } }, // Filter first to reduce dataset
{ $lookup: { // Join with customers collection
from: "customers",
localField: "customerId",
foreignField: "_id",
as: "customerDetails"
}},
{ $unwind: "$customerDetails" } // Unwind the joined array to get individual customer details
])
Best Practice: Use $lookup
for critical joins, but if performance issues arise, consider restructuring the data to reduce the need for frequent lookups.
4. Minimize Data Transferred with $project
The $project
stage allows you to reshape documents and limit the number of fields returned in the output. By excluding unused fields, you can significantly reduce the amount of data that is transferred or processed in later stages.
Example:
db.orders.aggregate([
{ $match: { status: "complete" } },
{ $project: { customerId: 1, amount: 1, _id: 0 } } // Only include necessary fields
])
Best Practice: Use $project
early in the pipeline to exclude unnecessary fields and reduce the data footprint, improving performance.
5. Use $facet
for Multiple Aggregations
The $facet
stage allows you to run multiple aggregations in parallel and return the results in a single document. This can be more efficient than running multiple separate queries, especially if the initial stages (like $match
) are the same.
Example:
db.orders.aggregate([
{ $match: { status: "complete" } }, // Filter once for all facets
{ $facet: {
totalSales: [{ $group: { _id: null, total: { $sum: "$amount" } } }],
avgSale: [{ $group: { _id: null, avg: { $avg: "$amount" } } }],
orderCount: [{ $count: "totalOrders" }]
}}
])
Best Practice: Use $facet
to consolidate multiple calculations that can be performed from the same dataset, reducing the need for multiple passes over the data.
6. Use the $merge
Stage for Long-Running Aggregations
For complex or long-running aggregation pipelines, consider using the $merge
stage to store the results in another collection. This can be helpful for storing pre-computed results that are used frequently, reducing the need to re-run the aggregation pipeline each time.
Example:
db.orders.aggregate([
{ $match: { status: "complete" } },
{ $group: { _id: "$customerId", totalSpent: { $sum: "$amount" } } },
{ $merge: { into: "customerSpending", whenMatched: "merge" } } // Merge results into another collection
])
Best Practice: Use $merge
for storing the output of frequent or computationally expensive aggregations, enabling you to retrieve results quickly without reprocessing.
7. Optimize Memory Usage with $bucket
and $bucketAuto
The $bucket
and $bucketAuto
stages allow you to group data into ranges or buckets, which can significantly reduce memory usage, especially with large datasets.
Example:
db.orders.aggregate([
{ $bucket: {
groupBy: "$amount", // Group by amount ranges
boundaries: [0, 50, 100, 200], // Define the ranges
default: "Other",
output: {
count: { $sum: 1 },
totalAmount: { $sum: "$amount" }
}
}}
])
Best Practice: Use $bucket
or $bucketAuto
for efficient data bucketing when dealing with large ranges or continuous data.
8. Monitor and Optimize with explain()
Always use the explain()
method to analyze how MongoDB is executing your aggregation pipeline. This provides insights into index usage, document scanning, and the overall execution plan. If you notice that MongoDB is performing collection scans or using inefficient indexes, adjust your pipeline or indexes accordingly.
Example:
db.orders.aggregate([
{ $match: { status: "complete" } },
{ $group: { _id: "$customerId", totalSpent: { $sum: "$amount" } } }
]).explain("executionStats")
Best Practice: Use explain()
to ensure that your aggregation pipeline is optimized for performance by identifying bottlenecks and opportunities for improvement.
9. Use the Aggregation Pipeline Builder (MongoDB Compass)
If you're unsure of how to structure or optimize your aggregation pipeline, you can use MongoDB Compass, which provides a visual aggregation pipeline builder. This tool helps you create and test pipelines interactively, allowing you to see the output at each stage and fine-tune the performance.
Summary of Best Practices for High-Performance Aggregation Pipelines
Best Practice | Action |
Place $match and $limit early | Filter data as early as possible to minimize the number of documents processed downstream. |
Use indexes for $match and $sort | Ensure fields in $match and $sort stages are indexed for optimal query performance. |
Limit fields with $project early | Reduce data size by projecting only required fields to minimize memory usage and processing. |
Be cautious with $lookup | Ensure foreign fields are indexed and join only when necessary to avoid performance hits. |
Use $facet for multiple aggregations | Consolidate multiple related aggregations in a single pipeline with $facet . |
Leverage $merge for large datasets | Store results in another collection to avoid re-running complex aggregations repeatedly. |
Test with explain() | Analyze the execution plan to |
Conclusion
Optimizing MongoDB aggregation pipelines is crucial for achieving high-performance data transformation and analysis. By following these best practices, you can significantly improve the efficiency and speed of your database operations. Remember to:
Structure your pipeline efficiently, placing filtering operations early
Utilize appropriate indexes to support your queries
Minimize data transfer with strategic use of $project
Use $facet for parallel aggregations and $merge for storing results of complex operations
Monitor and optimize your pipelines using the explain() method
By implementing these strategies, you can harness the full power of MongoDB's aggregation framework, enabling faster data processing and more responsive applications. Always test your aggregations with representative datasets to ensure optimal performance in production environments.
© 2024 MinervaDB Inc. All rights reserved.
The content in this document, including but not limited to text, graphics, and code examples, is the intellectual property of MinervaDB Inc. and is protected by copyright laws. No part of this document may be reproduced, distributed, or transmitted in any form or by any means, including photocopying, recording, or other electronic or mechanical methods, without the prior written permission of MinervaDB Inc., except in the case of brief quotations embodied in critical reviews and certain other noncommercial uses permitted by copyright law.
For permissions requests, please contact MinervaDB Inc. at contact@minervadb.com.
Subscribe to my newsletter
Read articles from Shiv Iyer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
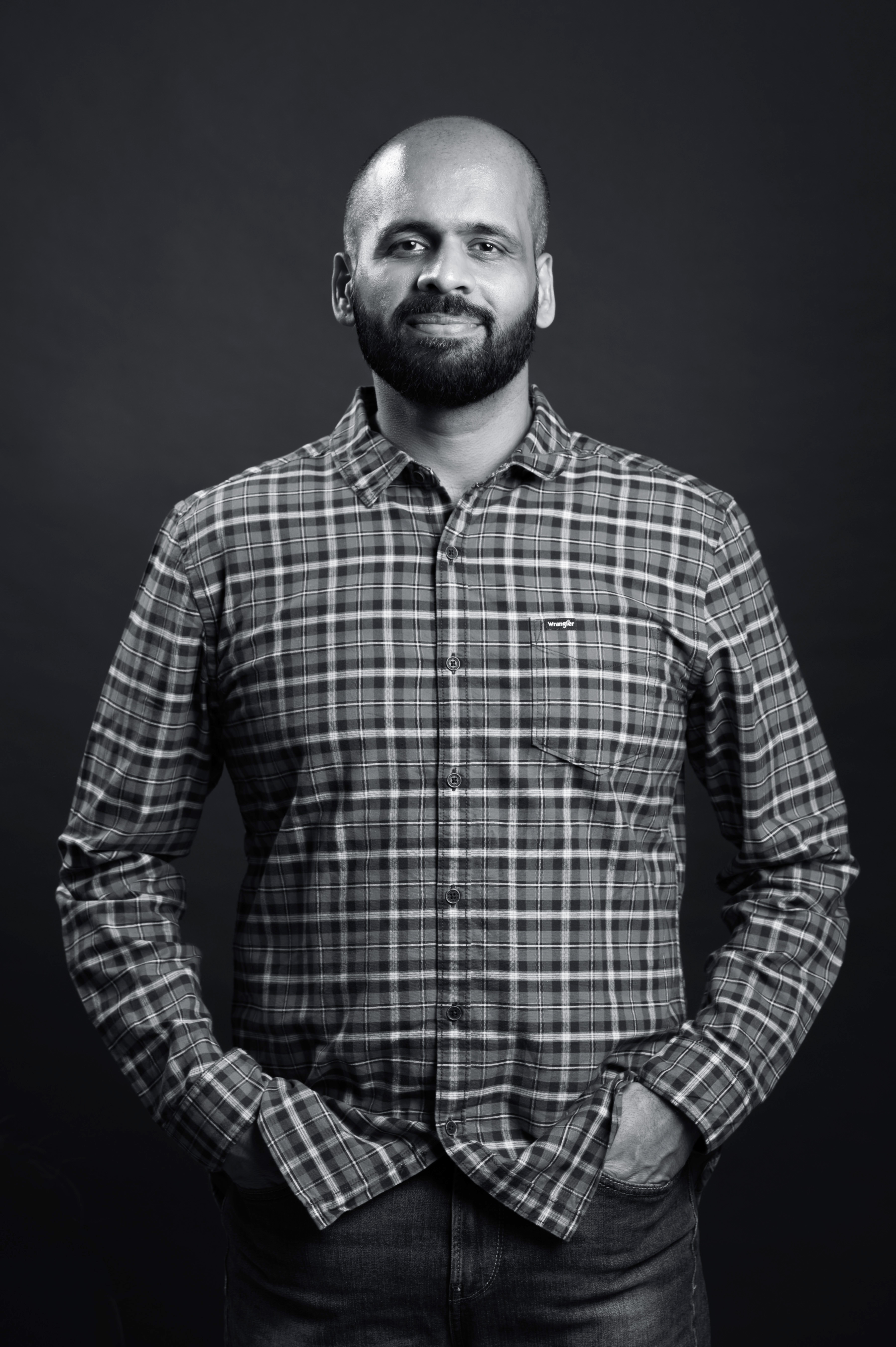
Shiv Iyer
Shiv Iyer
Over two decades of experience as a Database Architect and Database Engineer with core expertize in Database Systems Architecture/Internals, Performance Engineering, Scalability, Distributed Database Systems, SQL Tuning, Index Optimization, Cloud Database Infrastructure Optimization, Disk I/O Optimization, Data Migration and Database Security. I am the founder CEO of MinervaDB Inc. and ChistaDATA Inc.