A Beginner's Guide to Type Casting in Python: Day 11

Table of contents
- Introduction:
- Implicit Type-Casting
- Explicit Type-Casting
- Integer Type-Casting
- Example:
- float type casting :
- example:
- complex Type casting :
- Example:
- Example:
- String Type-Casting
- Example:
- Boolean Type-Casting
- Example:
- List Type-Casting
- Example:
- Other Type-Casting
- Example:
- Best Practices
- Example Use Cases
- Resources
- Challenges:
- Goals for Tomorrow:
- Conclusion:
- Connect with me:
- Join the conversation:
- Next Post:
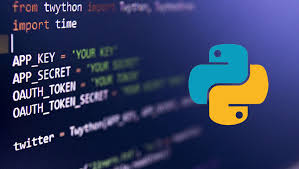
Introduction:
Welcome to Day 11 of my Python journey!
We can convert one type / class value to another type/ class. This conversion is called Typecasting or Type conversion.
The following are various in-built functions for type casting.
1. int()
2. float()
3. complex()
4. bool()
5. str()
Type-casting is the process of converting a value from one data type to another.
Python supports various types of type-casting, which can be explicit or implicit.
Implicit Type-Casting
Python automatically performs implicit type-casting in certain situations:
Numeric operations: int + float becomes float
String concatenation: str + int becomes str (using str() function)
Boolean operations: bool with other types
Explicit Type-Casting
Use the following functions to explicitly cast types:
Integer Type-Casting
We can use this function to convert values from other types to int
Example:
int(123.987)
123
int(10+5j)
TypeError: can't convert complex to int
int(True)
1
int(False)
0
int("10")
10
int("10.5")
ValueError: invalid literal for int() with base 10: '10.5'
int("ten")
ValueError: invalid literal for int() with base 10: 'ten'
int("0B1111")
ValueError: invalid literal for int() with base 10: '0B1111'
int ("101", base =2)
5
Note:
1. We can convert from any type to int except complex type.
2. If we want to convert str type to int type, compulsory str should contain only integral value and should be specified in base-10
float type casting :
We can use float() function to convert other type values to float type.
example:
float(10)
10.0
float(10+5j)
TypeError: can't convert complex to float
float(True)
1.0
float(False)
0.0
float("10")
10.0
float("10.5")
10.5
float("ten")
ValueError: could not convert string to float: 'ten'
float("0B1111")
ValueError: could not convert string to float: '0B1111'
Note:
1. We can convert any type value to float type except complex type.
2. Whenever we are trying to convert str type to float type compulsary str should be either integral or floating point literal and should be specified only in base-10.
complex Type casting :
We can use complex() function to convert other types to complex type.
Format 1: complex(x)
We can use this function to convert x into complex number with real part x and imaginary part 0.
Example:
complex(10) #==>10+0j
complex(10.5) #===>10.5+0j
complex(True) #==>1+0j
complex(False) #==>0j
complex("10") #==>10+0j
complex("10.5") #==>10.5+0j
complex("ten") #==>ValueError: complex() arg is a malformed string
Format-2: complex(x,y)
We can use this method to convert x and y into complex number such that x will be real part and y will be imaginary part.
Example:
complex(10,-2) #==>10-2j
complex(True,False) #==>1+0j
String Type-Casting
We can use this method to convert other type values to str type
Example:
str(10) #==>'10'
str(10.5) #==>'10.5'
str(10+5j) #==>'(10+5j)'
str(True) #==>'True'
repr() : Returns a string representation of the object
format() : Formats a string
Boolean Type-Casting
- We can use this function to convert other type values to bool type.
Example:
bool(0) #==>False
bool(1) #==>True
bool(10) #===>True
bool(10.5) #===>True
bool(0.178) #==>True
bool(0.0) #==>False
bool(10-2j) #==>True
bool(0+1.5j) #==>True
bool(0+0j) #==>False
bool("True") #==>True
bool("False") #==>True
bool("") #==>False
List Type-Casting
list() : Converts to list
tuple() : Converts to tuple
set() : Converts to set
dict() : Converts to dictionary
Example:
x = 'hello' y = list(x) # y becomes ['h', 'e', 'l', 'l', 'o'] z = tuple(x) # z becomes ('h', 'e', 'l', 'l', 'o')
Other Type-Casting
chr() : Converts integer to character
ord() : Converts character to integer
bin() : Converts integer to binary string
hex() : Converts integer to hexadecimal string
oct() : Converts integer to octal string
Example:
x = 65 y = chr(x) # y becomes 'A' z = ord('A') # z becomes 65
Best Practices
Use explicit type-casting for clarity.
Avoid implicit type-casting to prevent unexpected behavior.
Be aware of potential data loss during type-casting (e.g., converting float to int).
Example Use Cases
Data conversion for database interactions
Numeric computations requiring specific data types
String manipulation and formatting
Boolean logic and conditional statements
Feel free to ask me any questions or share your experiences with type-casting in Python!
Resources
official Python Documentation
W3Schools
GeeksforGeeks
Challenges:
Understanding type casting.
Handling coding errors.
Goals for Tomorrow:
Explore something more about type casting.
Learn about exceptions.
Conclusion:
Day 11 was a success!
Mastering type-casting in Python is essential for effective programming. By understanding the different types of type-casting and using them judiciously, you can write more efficient, readable, and maintainable code.
What are your views on type casting ? Share in the comments below.
Connect with me:
LinkedIn: [ https://www.linkedin.com/in/archana-prusty-4aa0b827a/ ]
GitHub: [ https://github.com/p-archana1 ]
Join the conversation:
Share your own learning experiences or ask questions in the comments.
Next Post:
Day 12 : control structure in python.
Happy learning!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.