Back to Basics: Mastering K-Means Clustering with NumPy
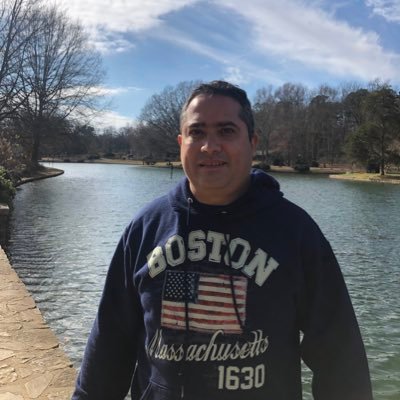
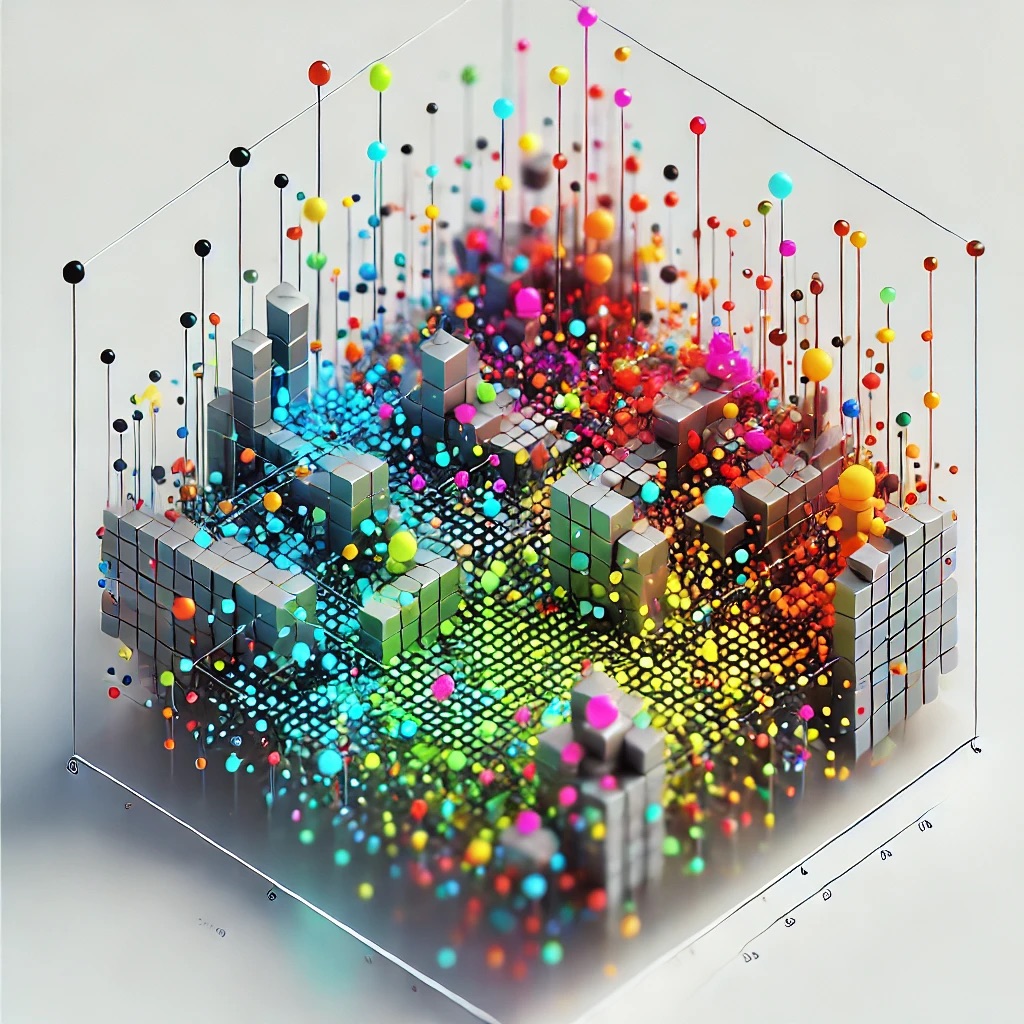
Imagine you're standing in front of a massive, chaotic pile of colorful marbles.
Your task? Organize them into distinct groups based on their similarities.
Sounds daunting, right?
Now, picture an intelligent algorithm that can do this for you, not just with marbles, but with complex, multi-dimensional data points.
Welcome to the world of K-Means Clustering, a powerful unsupervised machine learning technique that's revolutionizing data analysis across industries.
In this article, we'll delve deep into the mechanics of K-Means Clustering, explore its implementation using NumPy, and provide you with the knowledge to apply it effectively in real-world scenarios.
What is K-Means Clustering?
K-Means Clustering is an unsupervised machine learning algorithm used to partition a dataset into K distinct, non-overlapping clusters.
It's like having a smart assistant that can look at your data and say, "I see K different groups here."
The algorithm's goal is simple yet powerful: minimize the variance within each cluster.
It achieves this by iteratively assigning data points to clusters based on the nearest mean (centroid) and updating these centroids based on the current cluster memberships.
The Core Concept
At its heart, K-Means operates on a straightforward principle:
Choose K initial centroids (cluster centers) randomly.
Assign each data point to the nearest centroid.
Recalculate centroids based on the assigned points.
Repeat steps 2 and 3 until convergence.
The Mathematics Behind K-Means
Let's dive into the key mathematical components that make this algorithm tick.
Euclidean Distance
The cornerstone of K-Means is the distance metric used to determine similarity between points.
Typically, Euclidean distance is employed.
For two points in n-dimensional space, p = (p1, p2, ..., pn) and q = (q1, q2, ..., qn), the Euclidean distance is given by:
d(p,q) = √((p1 - q1)² + (p2 - q2)² + ... + (pn - qn)²)
This formula quantifies how "far apart" two points are in the feature space.
Implementing K-Means in Python
Now that we understand the theory, let's roll up our sleeves and implement K-Means using NumPy.
This implementation will give you a deep, hands-on understanding of how the algorithm works.
import numpy as np
class KMeans:
def __init__(self, n_clusters=3, max_iters=100, random_state=None):
self.n_clusters = n_clusters
self.max_iters = max_iters
self.random_state = random_state
self.centroids = None
def fit(self, X):
np.random.seed(self.random_state)
# Initialize centroids randomly
idx = np.random.choice(len(X), self.n_clusters, replace=False)
self.centroids = X[idx]
for _ in range(self.max_iters):
# Assign points to nearest centroid
distances = self._calculate_distances(X)
labels = np.argmin(distances, axis=0)
# Update centroids
new_centroids = np.array([
X[labels == k].mean(axis=0)
for k in range(self.n_clusters)
])
# Check for convergence
if np.all(self.centroids == new_centroids):
break
self.centroids = new_centroids
return labels
def predict(self, X):
distances = self._calculate_distances(X)
return np.argmin(distances, axis=0)
def _calculate_distances(self, X):
"""
Calculate distances between each point in X and all centroids.
"""
n_samples = X.shape[0]
distances = np.zeros((self.n_clusters, n_samples))
for i in range(n_samples):
diff = self.centroids - X[i]
distances[:, i] = np.linalg.norm(diff, axis=1)
return distances
This implementation encapsulates the core K-Means algorithm in a class.
Let's break down the key components:
Initialization
The __init__
method sets up the basic parameters:
n_clusters
: The number of clusters (K)max_iters
: Maximum number of iterationsrandom_state
: Seed for random number generation, ensuring reproducibility
Fitting the Model
The fit
method is where the magic happens:
It randomly initializes centroids.
It iteratively assigns points to clusters and updates centroids.
It checks for convergence or stops after
max_iters
.
Distance Calculation
The _calculate_distances
method computes the Euclidean distances between points and centroids.
This is a critical part of the algorithm, determining which cluster each point belongs to.
Prediction
The predict
method allows us to assign new data points to clusters based on the trained model.
Testing the Implementation
def test_kmeans():
# Test case 1: Simple 2D dataset
X1 = np.array([[1, 2], [1.5, 1.8], [5, 8], [8, 8], [1, 0.6], [9, 11]])
kmeans = KMeans(n_clusters=2, random_state=42)
labels1 = kmeans.fit(X1)
assert len(np.unique(labels1)) == 2, "Test case 1 failed: Incorrect number of clusters"
# Test case 2: Larger random dataset
np.random.seed(42)
X2 = np.vstack((np.random.randn(100, 2) * 0.5 + np.array([2, 2]),
np.random.randn(100, 2) * 0.5 + np.array([-2, -2]),
np.random.randn(100, 2) * 0.5 + np.array([2, -2])))
kmeans = KMeans(n_clusters=3, random_state=42)
labels2 = kmeans.fit(X2)
assert len(np.unique(labels2)) == 3, "Test case 2 failed: Incorrect number of clusters"
# Test case 3: Predict on new data
X_new = np.array([[0, 0], [5, 5]])
predicted_labels = kmeans.predict(X_new)
assert len(predicted_labels) == 2, "Test case 3 failed: Incorrect number of predictions"
print("All test cases passed!")
Optimizing K-Means: The Efficiency Frontier
While our initial implementation is functional, there's always room for improvement.
Let's explore some optimizations that can significantly boost the performance of our K-Means algorithm.
Vectorized Distance Calculation
One of the most computationally intensive parts of K-Means is calculating distances.
We can leverage NumPy's broadcasting capabilities to perform this calculation more efficiently:
def _compute_distances(self, X):
"""
Compute the Euclidean distance between each data point in X and each centroid.
Parameters:
- X (np.ndarray): Data points.
- centroids (np.ndarray): Centroid points.
Returns:
- distances (np.ndarray): Distance matrix of shape (n_samples, n_clusters).
"""
# Using broadcasting to compute distances efficiently
return np.sqrt(((X[:, np.newaxis, :] - self.centroids[np.newaxis, :, :]) ** 2).sum(axis=2))
This single line replaces our entire _calculate_distances
method.
X[:, np.newaxis, :] reshapes the data points to have an extra dimension, resulting in shape (n_samples, 1, n_features).
centroids[np.newaxis, :, :] reshapes the centroids similarly to (1, n_clusters, n_features).
The subtraction X[:, np.newaxis, :] - centroids[np.newaxis, :, :] leverages broadcasting to compute differences between each data point and each centroid, resulting in a shape (n_samples, n_clusters, n_features).
Squaring the differences ** 2 prepares the data for Euclidean distance calculation. And .sum(axis=2) aggregates the squared differences across features, yielding squared distances with shape (n_samples, n_clusters).
And finally, np.sqrt(...) computes the final Euclidean distances.
Benefits of Broadcasting:
Vectorization: Eliminates the need for explicit Python loops, enhancing speed.
Memory Efficiency: Utilizes NumPy's optimized operations for handling large arrays.
Readability: Provides a concise and clear implementation of distance calculations.
In order to integrate the Optimized Distance Function into our KMeans class, we can modify the code as follows:
class KMeans:
def __init__(self, n_clusters=3, max_iters=100, random_state=None):
self.n_clusters = n_clusters
self.max_iters = max_iters
self.random_state = random_state
self.centroids = None
def fit(self, X):
np.random.seed(self.random_state)
# Initialize centroids randomly
idx = np.random.choice(len(X), self.n_clusters, replace=False)
self.centroids = X[idx]
for _ in range(self.max_iters):
# Assign points to nearest centroid using the optimized distance function
distances = self._compute_distances(X)
labels = np.argmin(distances, axis=1)
# Update centroids
new_centroids = np.array([X[labels == k].mean(axis=0) for k in range(self.n_clusters)])
# Check for convergence
if np.all(self.centroids == new_centroids):
break
self.centroids = new_centroids
return labels
def predict(self, X):
distances = self._compute_distances(X)
return np.argmin(distances, axis=1)
def _compute_distances(self, X):
# Using broadcasting to compute distances efficiently
return np.sqrt(((X[:, np.newaxis, :] - self.centroids[np.newaxis, :, :]) ** 2).sum(axis=2))
Real-World Applications: K-Means in Action
K-Means Clustering's versatility makes it applicable across numerous domains.
Customer Segmentation in Marketing
Marketers use K-Means to group customers based on purchasing behavior, demographics, and other attributes.
This segmentation allows for targeted marketing strategies and personalized customer experiences.
Example scenario:
Collect data on customer age, income, and purchase frequency.
Apply K-Means to identify distinct customer segments.
Tailor marketing campaigns for each segment.
Image Compression
K-Means can be used for lossy image compression by reducing the number of colors in an image:
Treat each pixel as a point in 3D space (RGB values).
Apply K-Means to cluster these points.
Replace each pixel's color with its cluster centroid.
This technique can significantly reduce file size while maintaining visual quality.
Anomaly Detection in Cybersecurity
K-Means can help identify unusual patterns in network traffic:
Collect data on packet sizes, frequencies, and destinations.
Use K-Means to cluster "normal" traffic patterns.
Flag data points that are far from all cluster centroids as potential anomalies.
This approach can detect novel cyber threats that signature-based systems might miss.
Limitations and Considerations: When K-Means Falls Short
While K-Means is powerful, it's not a one-size-fits-all solution. Understanding its limitations is crucial for effective application.
Sensitivity to Initial Centroids
The algorithm's results can vary depending on the initial centroid placement. To mitigate this:
Run the algorithm multiple times with different initializations.
Use techniques like K-Means++ for smarter centroid initialization.
Assumption of Spherical Clusters
K-Means assumes that clusters are spherical and equally sized. This can lead to poor results when:
Clusters have complex shapes
Clusters have significantly different sizes or densities
In such cases, consider alternatives like DBSCAN or Gaussian Mixture Models.
Handling Categorical Data
K-Means works natively with numerical data.
For categorical features:
Use techniques like one-hot encoding to convert categories to numerical values.
Consider specialized algorithms for categorical data, like K-Modes.
Scaling Sensitivity
K-Means is sensitive to the scale of features.
Always normalize your data before applying K-Means to ensure all features contribute equally to the distance calculations.
Data Preprocessing
Handle missing values:
Impute missing data or remove incomplete records.
K-Means doesn't handle missing values natively.
Feature scaling:
Normalize or standardize features to ensure equal contribution.
Use techniques like Min-Max scaling or Z-score normalization.
Dimensionality reduction:
For high-dimensional data, consider PCA or t-SNE before clustering.
This can improve performance and visualization.
Initialization Strategies
Multiple runs:
Run K-Means multiple times with different random initializations.
Choose the result with the lowest inertia.
K-Means++:
Use this advanced initialization method for better starting centroids.
It spreads out initial centroids, often leading to better results.
Conclusion
As we've explored, K-Means clustering is a powerful, versatile algorithm with applications across numerous domains.
Its simplicity belies its effectiveness in uncovering hidden patterns and structures in data.
From customer segmentation to image compression, from anomaly detection to data preprocessing, K-Means continues to be a fundamental tool in the data scientist's toolkit.
However, like any tool, its effectiveness depends on proper understanding and application.
By being aware of its strengths, limitations, and best practices, we can leverage K-Means to its full potential.
As data continues to grow in volume and complexity, K-Means is evolving, with innovations like Mini-Batch K-Means and GPU acceleration pushing the boundaries of what's possible.
Whether you're a seasoned data scientist or just beginning your journey, mastering K-Means clustering is a valuable step towards becoming a more effective and insightful data analyst.
So, the next time you're faced with a mountain of unstructured data, remember: within that chaos, K-Means might just help you find the order you're looking for.
PS: If you like this article, share it with others ♻️
Would help a lot ❤️
And feel free to follow me for articles more like this.
Subscribe to my newsletter
Read articles from Juan Carlos Olamendy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
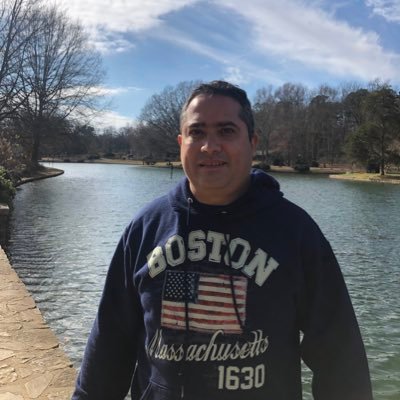
Juan Carlos Olamendy
Juan Carlos Olamendy
🤖 Talk about AI/ML · AI-preneur 🛠️ Build AI tools 🚀 Share my journey 𓀙 🔗 http://pixela.io