Mastering Contexts in Go: Managing Concurrency with Ease
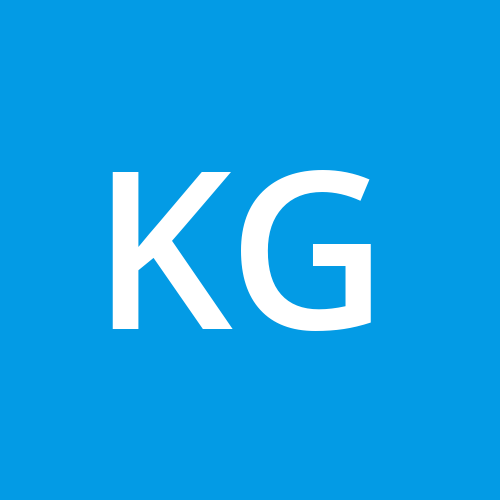
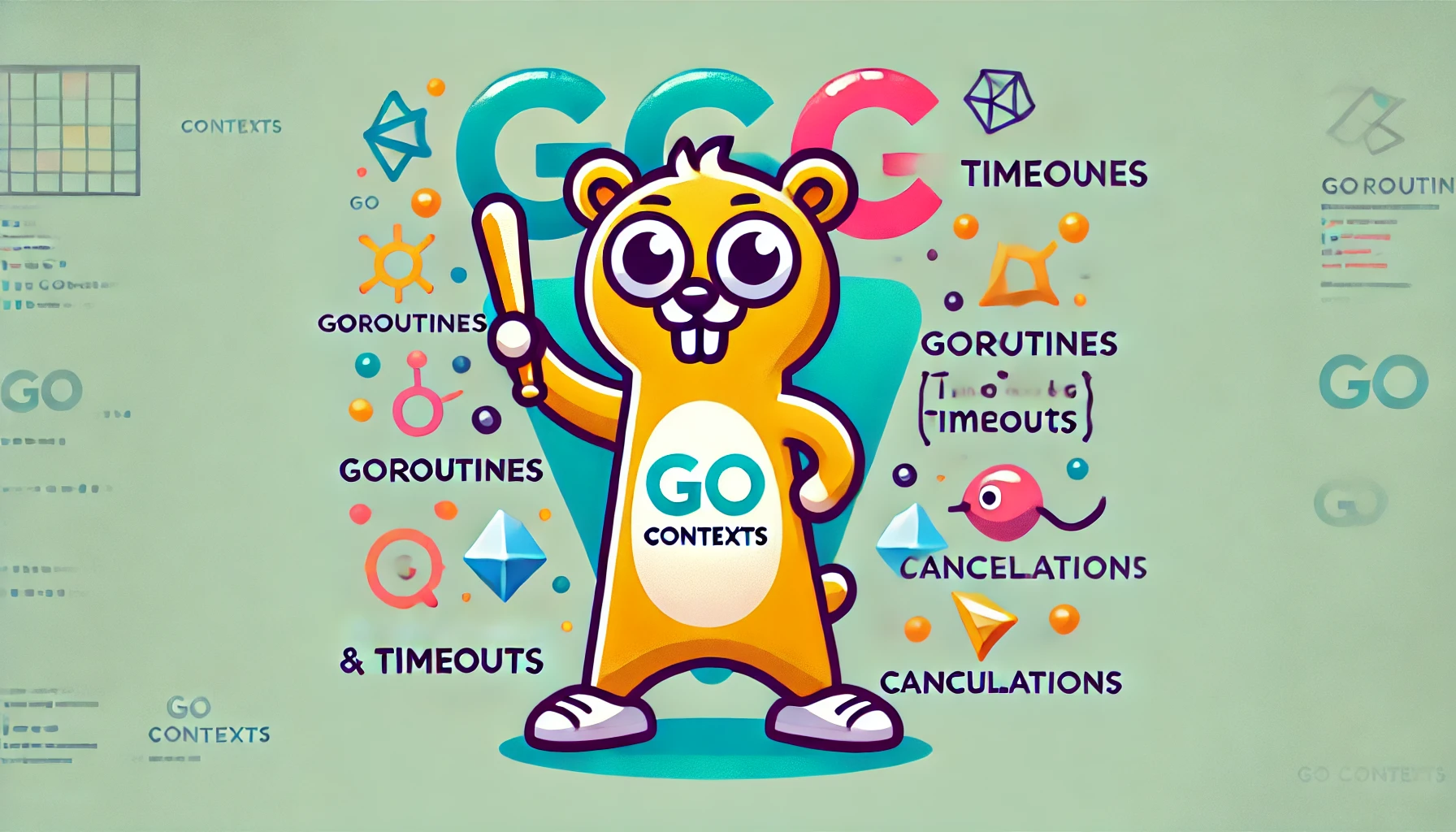
Concurrency is one of Go’s most powerful features, and at the heart of effective concurrency management lies the context
package. Whether you're handling web requests, database interactions, or other time-sensitive tasks, contexts allow you to manage the lifecycle of concurrent processes, passing data, managing timeouts, and handling cancellations in an elegant way.
In this blog, we’ll dive deep into Go's context
package, learning:
What contexts are
How they simplify concurrent programming
Practical examples where contexts make a difference
How to implement context in your Go applications
Let’s get started!
What is a Context in Go?
At its core, a context in Go is a way to carry deadlines, cancellation signals, and request-scoped values across API boundaries and between goroutines. Contexts help manage the lifecycle of concurrent operations, providing a way to cancel or time out long-running processes and pass values between goroutines efficiently.
Why Do We Need context
?
Imagine you're writing a web server in Go that handles multiple requests concurrently. Each request triggers a series of goroutines—one for fetching data from a database, another for calling an external API, and perhaps one more for logging. What if:
A client disconnects mid-request?
A query takes too long and you want to cancel it to avoid wasted resources?
You want to pass a request ID to all functions spawned by a single request?
This is where Go’s context
package shines. It allows you to:
Signal cancellation to all goroutines related to a request when it's no longer needed.
Enforce timeouts to avoid long-running tasks.
Pass request-scoped data like authentication tokens or request IDs between functions.
Types of Context in Go
Go’s context
package provides four main types of contexts:
context.Background()
The root context, generally used when you don’t have an existing context to derive from.
Typically used at the start of a program.
context.TODO()
- A placeholder for when you’re unsure what context to use. Often used during development when you're planning to add a proper context later.
context.WithCancel(parentContext)
- Creates a new context from a parent context that can be canceled. This is useful for terminating goroutines when they're no longer needed.
context.WithTimeout(parentContext, duration)
- Creates a new context that is automatically canceled after a specified timeout.
context.WithDeadline(parentContext, deadline)
- Similar to
WithTimeout
, but allows you to specify an exact deadline (specific time) for cancellation.
- Similar to
How to Use Context: A Simple Example
Let’s start by creating a basic context to manage goroutines:
package main
import (
"context"
"fmt"
"time"
)
func main() {
// Create a parent context that will be canceled after 3 seconds
ctx, cancel := context.WithTimeout(context.Background(), 3*time.Second)
defer cancel() // Ensure the context is canceled to free up resources
// Start a goroutine and pass it the context
go doSomething(ctx)
// Wait for 5 seconds before the main function exits
time.Sleep(5 * time.Second)
}
func doSomething(ctx context.Context) {
for {
select {
case <-ctx.Done():
// If the context is canceled, exit the goroutine
fmt.Println("Context canceled:", ctx.Err())
return
default:
// Simulate doing some work
fmt.Println("Working...")
time.Sleep(1 * time.Second)
}
}
}
Output:
Working...
Working...
Working...
Context canceled: context deadline exceeded
Context in Real-World Applications
Now that we understand the basics, let’s explore some real-world scenarios where context is invaluable.
1. Handling HTTP Requests with Context
When building web servers, you may need to terminate long-running requests or carry request-specific data (e.g., authentication info) across function calls.
package main
import (
"context"
"fmt"
"net/http"
"time"
)
func main() {
http.HandleFunc("/data", func(w http.ResponseWriter, r *http.Request) {
// Create a context with a 2-second timeout for the request
ctx, cancel := context.WithTimeout(r.Context(), 2*time.Second)
defer cancel()
// Pass the context to a function handling data fetching
data, err := fetchData(ctx)
if err != nil {
http.Error(w, "Request timed out", http.StatusRequestTimeout)
return
}
fmt.Fprintf(w, "Data fetched: %s", data)
})
http.ListenAndServe(":8080", nil)
}
func fetchData(ctx context.Context) (string, error) {
// Simulate a long-running task
select {
case <-time.After(3 * time.Second):
return "Important Data", nil
case <-ctx.Done():
return "", ctx.Err() // Return an error if context is canceled
}
}
Explanation:
The context is created with a 2-second timeout for the HTTP request.
If the data-fetching function (
fetchData
) takes longer than 2 seconds, the context cancels the operation, and the server responds with aRequest timed out
message.If the request is completed in under 2 seconds, the data is returned successfully.
Expected Output Scenarios
When the request completes successfully (less than 2 seconds):
Data fetched: Important Data
When the request times out (after 2 seconds):
Request timed out
Go’s context orchestrating concurrency like a maestro.
2. Cancelling Database Queries
Imagine you are querying a database and a client cancels the request. You want to ensure that your database operations stop immediately to free up resources.
func queryDatabase(ctx context.Context, query string) (result string, err error) {
// Assume we're using a database driver that supports context cancellation
db, err := sql.Open("postgres", "your_connection_string")
if err != nil {
return "", err
}
// Use context with the query to manage cancellation
row := db.QueryRowContext(ctx, query)
err = row.Scan(&result)
if err != nil {
if err == sql.ErrNoRows {
return "", nil
}
// Handle the error (might be due to context cancellation)
return "", err
}
return result, nil
}
Explanation:
The QueryRowContext()
method from SQL drivers takes a context as its first argument, allowing the query to be canceled if the context is done. If the context is canceled (e.g., because the user disconnected or the timeout expired), the database query will be interrupted, freeing up resources.
Expected Output Scenarios
When the query successfully returns a result:
"Important Data"
When no rows are found (i.e., the query returns an empty result):
<empty>
When the context is canceled (e.g., due to a timeout or user intervention):
context canceled
When there is a database error (e.g., syntax error or connection issue):
pq: syntax error at or near "SELECT"
3. Managing Background Jobs with Context
In services running background tasks (e.g., sending emails, processing files), it’s important to ensure tasks can be canceled if the system shuts down or the task exceeds a given time limit.
func processTask(ctx context.Context, taskID string) {
for {
select {
case <-ctx.Done():
fmt.Println("Task", taskID, "canceled")
return
default:
// Simulate task processing
fmt.Println("Processing task", taskID)
time.Sleep(1 * time.Second)
}
}
}
Output:
Processing task 1
Processing task 1
Processing task 1
Task 1 canceled
Best Practices for Using Context in Go
While contexts are powerful, there are some best practices to keep in mind:
Pass context as the first parameter in function signatures.
- This keeps your API consistent and easy to read.
func fetchData(ctx context.Context) {}
Use
context.Background()
when starting the top-level context.- This is common for initializing contexts in the main function or the root of your application.
Avoid storing context in struct fields.
- Contexts should not be stored in long-lived variables or passed beyond the lifetime of their parent function.
Use
context.WithCancel()
to control the lifecycle of goroutines.- Ensure you cancel contexts when they are no longer needed to avoid resource leaks.
When you master Go's context and everything runs smoothly.
Conclusion
Go’s context
package is an essential tool for managing concurrency, coordinating goroutines, and ensuring efficient resource handling in time-bound operations. Whether you're dealing with HTTP requests, database queries, or long-running tasks, contexts can significantly simplify how you handle cancellations, timeouts, and data propagation across your application.
To dive deeper into Go’s context and concurrency patterns, here are some excellent resources for further reading:
Further Reading and Resources:
Go Official Documentation - Context Package
Explore the official Go documentation for thecontext
package, which provides in-depth examples and explanations.
Go Context Package DocumentationGo by Example - Context
Go by Example is a great website with clear, practical examples. This section covers context usage.
Go by Example - ContextGophercises - Go Coding Exercises
A series of Go programming exercises, including ones that explore concurrency and context.
GophercisesGo Blog - Go Concurrency Patterns
A great read on the official Go Blog about concurrency patterns, where context plays a crucial role.
Go Blog - Concurrency Patterns
These resources will help you build a stronger understanding of context and concurrency in Go. Whether you’re a beginner or an experienced developer, these materials can deepen your knowledge and provide further insight into managing complex, concurrent systems efficiently.
Subscribe to my newsletter
Read articles from Kartik Garg directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
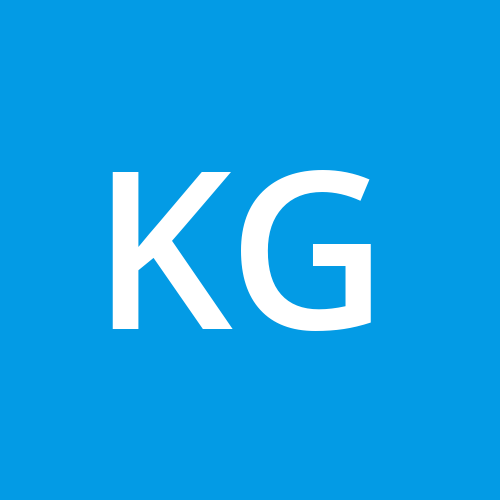