Importance of Frontend Integration for a Senior Web Developer

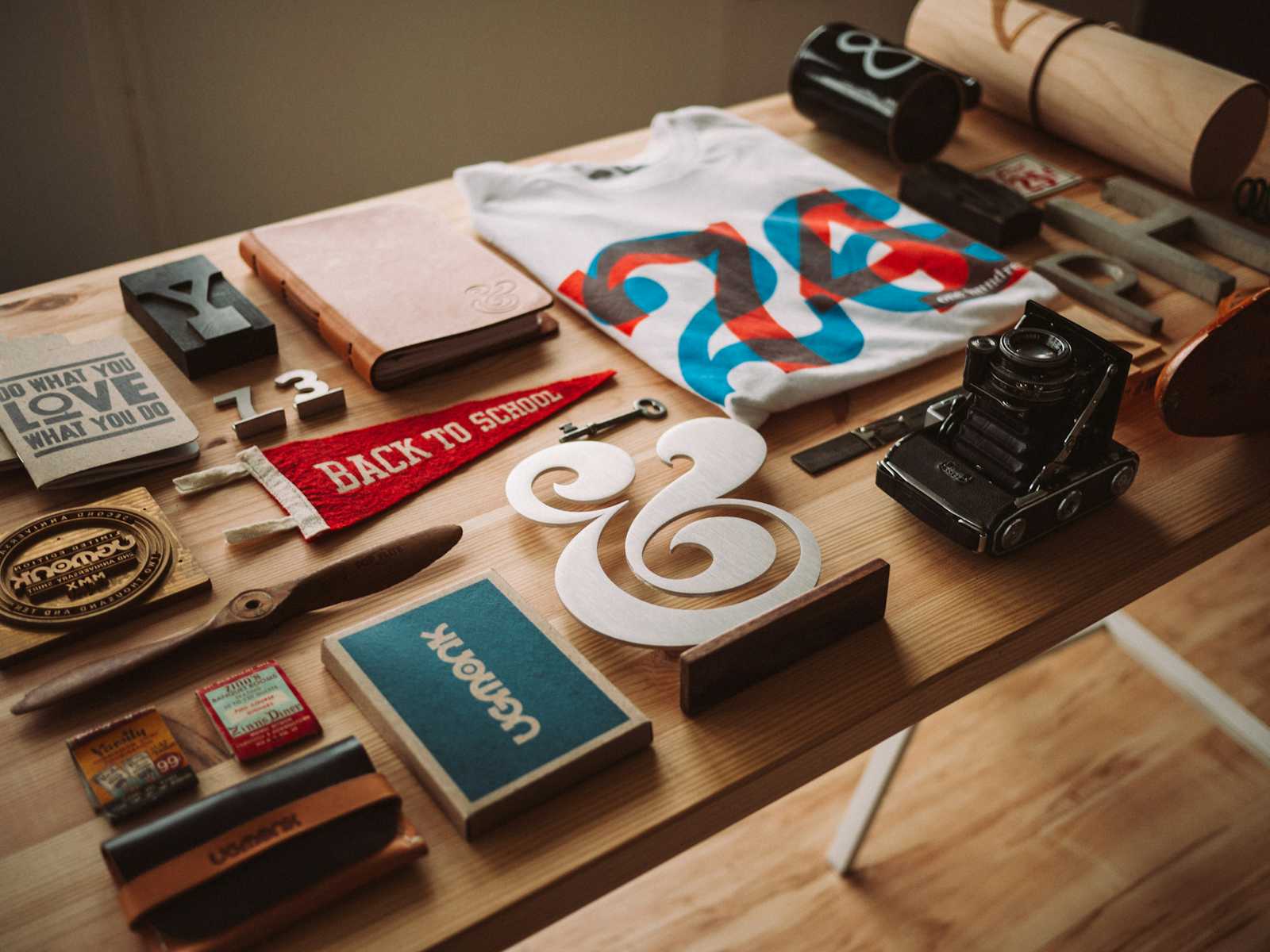
Understanding of Frontend Technologies:
A senior PHP or Laravel developer is often required to work with frontend developers or handle full-stack responsibilities. Understanding frontend technologies like React, Vue.js, or Angular allows for better collaboration and more efficient development of interactive applications.
Real-Life Scenario:
Imagine you're building an e-commerce platform where the frontend is built with Vue.js to create a highly dynamic user experience. As a senior Laravel developer, you'd need to build a RESTful API (or GraphQL) to serve the necessary data (products, orders, etc.) to Vue.js components.
Example:
In Laravel, you might expose an endpoint to retrieve product data:
// Routes in Laravel API
Route::get('/api/products', [ProductController::class, 'index']);
// ProductController in Laravel
public function index() {
return ProductResource::collection(Product::all());
}
On the frontend side, Vue.js would make an HTTP request to this endpoint and display the products dynamically. Your understanding of Vue.js will help you ensure that the data format and response structure are optimal for this frontend framework, making integration smoother.
Vue.js Example:
Here’s a Vue.js component that fetches products from the Laravel API and displays them.
<template>
<div>
<h1>Products</h1>
<ul v-if="products.length">
<li v-for="product in products" :key="product.id">{{ product.name }} - ${{ product.price }}</li>
</ul>
<p v-else>Loading products...</p>
</div>
</template>
<script>
export default {
data() {
return {
products: []
};
},
mounted() {
// Fetch products when the component is mounted
fetch('http://your-laravel-api-url/api/products')
.then(response => response.json())
.then(data => {
this.products = data.data; // Assuming your API response has a "data" property
})
.catch(error => console.error('Error fetching products:', error));
}
};
</script>
This Vue.js component calls the Laravel API as soon as it is mounted, processes the JSON response, and renders a list of products.
React.js Example:
Here’s a React.js component that fetches products from the Laravel API.
import React, { useState, useEffect } from 'react';
function Products() {
const [products, setProducts] = useState([]);
useEffect(() => {
// Fetch products when the component is mounted
fetch('http://your-laravel-api-url/api/products')
.then(response => response.json())
.then(data => {
setProducts(data.data); // Assuming your API response has a "data" property
})
.catch(error => console.error('Error fetching products:', error));
}, []); // Empty array means this useEffect runs only once when the component mounts
return (
<div>
<h1>Products</h1>
{products.length > 0 ? (
<ul>
{products.map(product => (
<li key={product.id}>
{product.name} - ${product.price}
</li>
))}
</ul>
) : (
<p>Loading products...</p>
)}
</div>
);
}
export default Products;
This React component uses the useEffect
hook to call the Laravel API when the component is mounted and stores the products in state using useState
.
Full Stack Experience (Optional):
While full-stack experience isn't mandatory for a senior developer, it can be highly beneficial. Being able to handle both backend and frontend tasks makes you more versatile, especially in smaller teams or startups where you may need to build and maintain both layers.
Real-Life Scenario:
A startup is developing a SaaS product where you, as a senior Laravel developer, are responsible for both backend logic (user authentication, database management, etc.) and frontend functionality. If you have full-stack experience, you can directly work on integrating both parts without relying on a separate frontend team.
Example:
You might handle a React-based frontend while writing the backend API. Here’s how you could serve a login system in Laravel for a React frontend:
// Laravel API route for login
Route::post('/api/login', [AuthController::class, 'login']);
// AuthController logic
public function login(Request $request) {
$credentials = $request->only('email', 'password');
if (Auth::attempt($credentials)) {
$token = Auth::user()->createToken('AuthToken')->accessToken;
return response()->json(['token' => $token]);
} else {
return response()->json(['error' => 'Unauthorized'], 401);
}
}
The React frontend would consume this login endpoint and store the returned authentication token in local storage or cookies. A senior developer would also need to understand CORS (Cross-Origin Resource Sharing) issues that may arise during frontend-backend integration and configure Laravel accordingly.
Vue.js Example:
This Vue.js component allows a user to log in using the Laravel API.
<template>
<div>
<h1>Login</h1>
<form @submit.prevent="login">
<label>Email:</label>
<input type="email" v-model="email" required />
<label>Password:</label>
<input type="password" v-model="password" required />
<button type="submit">Login</button>
</form>
<p v-if="error">{{ error }}</p>
</div>
</template>
<script>
export default {
data() {
return {
email: '',
password: '',
error: ''
};
},
methods: {
login() {
fetch('http://your-laravel-api-url/api/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
email: this.email,
password: this.password
})
})
.then(response => response.json())
.then(data => {
if (data.token) {
localStorage.setItem('token', data.token);
alert('Login successful');
} else {
this.error = 'Login failed. Please check your credentials.';
}
})
.catch(() => {
this.error = 'An error occurred. Please try again.';
});
}
}
};
</script>
Here, the login form in Vue.js sends a POST request to the Laravel API with the user’s credentials. On successful login, the token is stored in localStorage
.
React.js Example:
This React.js component performs the login and handles the response.
import React, { useState } from 'react';
function Login() {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [error, setError] = useState('');
const handleLogin = (e) => {
e.preventDefault();
fetch('http://your-laravel-api-url/api/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
email,
password
})
})
.then(response => response.json())
.then(data => {
if (data.token) {
localStorage.setItem('token', data.token);
alert('Login successful');
} else {
setError('Login failed. Please check your credentials.');
}
})
.catch(() => setError('An error occurred. Please try again.'));
};
return (
<div>
<h1>Login</h1>
<form onSubmit={handleLogin}>
<label>Email:</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/>
<label>Password:</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
required
/>
<button type="submit">Login</button>
</form>
{error && <p>{error}</p>}
</div>
);
}
export default Login;
The React version behaves similarly: it uses useState
to manage form inputs and handles login logic inside the handleLogin
function, sending the user credentials via a POST request and saving the authentication token in localStorage
.
Summary:
In both Vue.js and React.js, the approach is similar:
Fetching Data (e.g., Products): Make an HTTP GET request to fetch data from the API and display it dynamically using the component’s state.
User Authentication (e.g., Login): Make an HTTP POST request to submit form data to the API, handle authentication, and store tokens locally.
Understanding both frontend frameworks allows a senior developer to integrate backend APIs effectively, ensuring smooth data flow and optimal performance across the full stack.
Why Are These Skills Important?
Seamless Communication Between Backend and Frontend: Understanding how frontend technologies work allows you to build APIs that align with frontend needs. You can structure your responses in a way that makes frontend development easier and more efficient.
Faster Troubleshooting: When issues arise, especially in data flow between frontend and backend, a senior developer who understands both sides can troubleshoot problems more quickly.
Improved Performance and User Experience: Knowing how to build an efficient API and understanding how frontend frameworks consume that data can significantly improve the performance and responsiveness of the application, leading to better user experiences.
These skills are critical in modern web applications where separation between frontend and backend has become more common due to the rise of Single Page Applications (SPAs) and headless CMS architectures.
Subscribe to my newsletter
Read articles from Dale Lanto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Dale Lanto
Dale Lanto
A passionate Full Stack and Backend Web Developer with 7+ years of experience, specializing in PHP, Laravel, and a range of modern web technologies. I enjoy solving complex problems, optimizing systems, and delivering efficient, maintainable code. Check out some of my work at dalelanto.netlify.app or explore my GitHub at github.com/dalelantowork.