How to Remove Background from Images Using Node.js
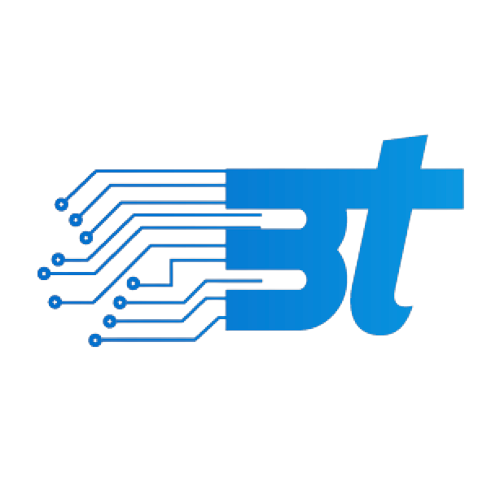
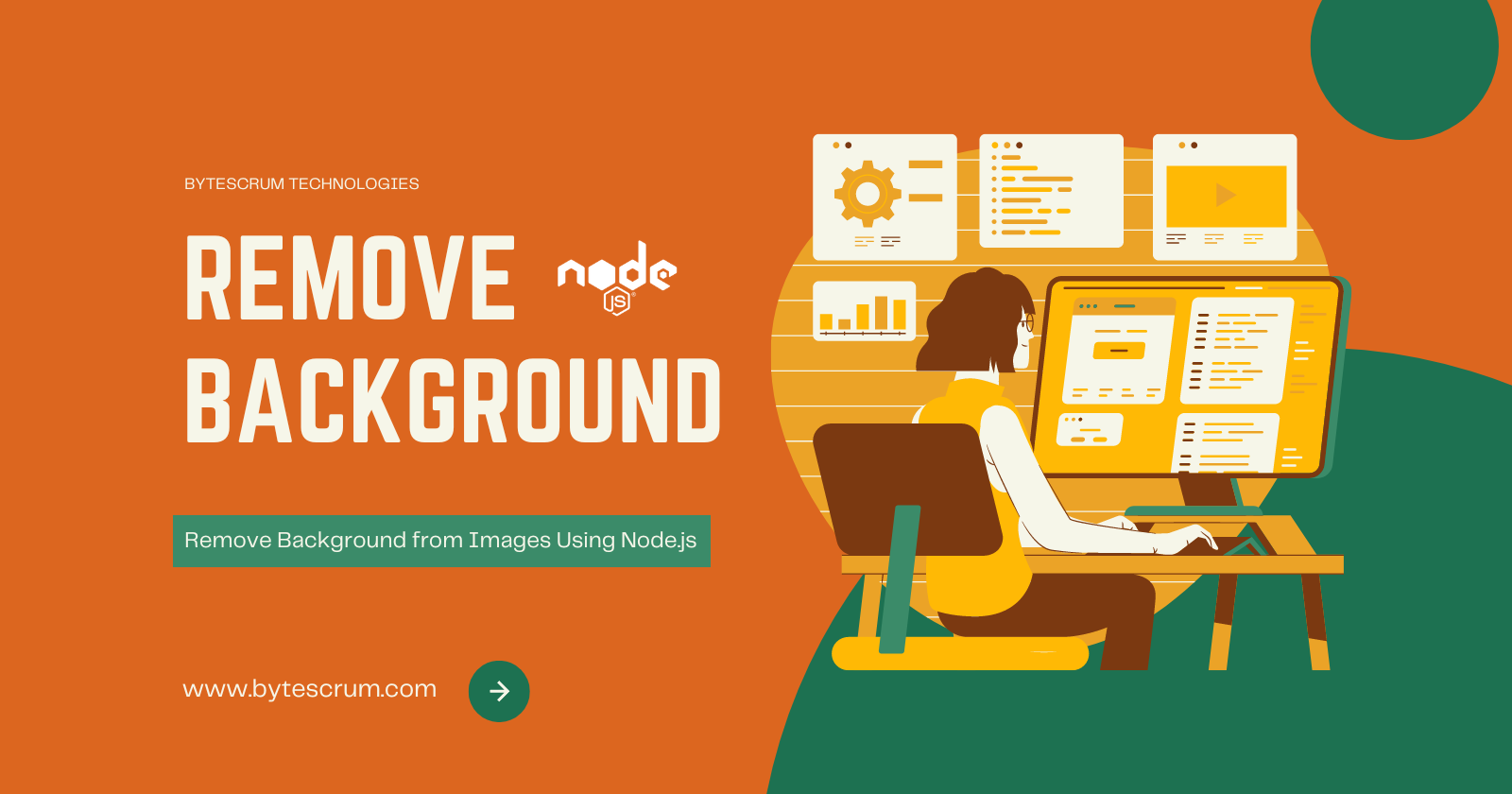
Removing the background from an image has become a widely required task, especially in e-commerce, graphic design, and content creation. Whether you're creating product photos, banners, or social media content, a clean and transparent background often makes the images more appealing. Luckily, with Node.js, you can automate this process programmatically.
In this blog, we'll walk through how to remove the background from images using Node.js, leveraging various libraries and APIs to help streamline the process.
1. Prerequisites
Before diving into the code, ensure that you have the following set up:
Node.js: You can download and install Node.js from here.
A Code Editor: Such as VSCode, Sublime, or any IDE of your choice.
Basic Knowledge of Node.js: You should be familiar with basic Node.js concepts such as
npm
, modules, and async/await.An Image to Test With: You’ll need at least one image with a distinguishable foreground and background for testing.
2. Choosing an Approach
There are several methods to remove backgrounds in Node.js. The approach you take will depend on your specific use case, image type, and desired output. Here are the most common ways:
Using Remove.bg API: A third-party service specifically designed to remove backgrounds from images.
Using OpenCV and Image Processing: OpenCV is a powerful library for computer vision tasks, and it can be used for background removal.
Using Pixel Manipulation with Sharp: Sharp is an image processing library that allows pixel manipulation, which can help in basic background removal.
3. Using Remove.bg API
Remove.bg is a popular API that provides high-quality background removal using AI. It’s the easiest way to remove backgrounds but requires an API key.
Step 1: Install Required Packages
First, let’s install axios
for making HTTP requests and fs
to handle file system operations:
npm install axios fs
Step 2: Set Up the Remove.bg API
Next, sign up for an API key from Remove.bg and create a new project in your codebase.
const axios = require('axios');
const fs = require('fs');
require('dotenv').config(); // If using environment variables
const removeBackgroundFromImage = async (inputPath, outputPath) => {
const apiKey = process.env.REMOVEBG_API_KEY; // Set this key in your .env file
const formData = {
image_file: fs.createReadStream(inputPath),
size: 'auto',
};
try {
const response = await axios({
method: 'post',
url: 'https://api.remove.bg/v1.0/removebg',
data: formData,
headers: {
'X-Api-Key': apiKey,
...formData.getHeaders()
},
responseType: 'arraybuffer'
});
fs.writeFileSync(outputPath, response.data);
console.log('Background removed successfully!');
} catch (error) {
console.error('Error removing background:', error.message);
}
};
removeBackgroundFromImage('input.jpg', 'output.png');
Explanation:
Input Image: We use
fs.createReadStream()
to read the image file.API Call: We send a POST request to Remove.bg API with the image data.
Output: The result is saved as a transparent PNG file.
Pros:
High-quality background removal.
Easy to implement.
Requires no image processing expertise.
Cons:
Requires an API key.
Limited free usage (subscription-based).
4. Using OpenCV and Image Processing
For those who prefer an open-source, offline solution, OpenCV is a powerful library that can handle a wide variety of image processing tasks, including background removal.
Step 1: Install OpenCV
First, install the Node.js bindings for OpenCV.
npm install opencv4nodejs
Step 2: Basic Image Background Removal
Here’s an example of removing a background using color thresholding:
const cv = require('opencv4nodejs');
const path = require('path');
// Load the image
const image = cv.imread(path.resolve(__dirname, 'input.jpg'));
// Convert the image to HSV (Hue, Saturation, Value) format
const hsvImg = image.cvtColor(cv.COLOR_BGR2HSV);
// Define the color range for the background (e.g., white or a specific color)
const lowerWhite = new cv.Vec(0, 0, 200);
const upperWhite = new cv.Vec(180, 20, 255);
// Create a mask where white colors are detected
const mask = hsvImg.inRange(lowerWhite, upperWhite);
// Invert the mask to keep the foreground
const maskInv = mask.bitwiseNot();
// Apply the mask to the original image to remove the background
const foreground = image.copyTo(new cv.Mat(), maskInv);
// Save the output image
cv.imwrite(path.resolve(__dirname, 'output.png'), foreground);
console.log('Background removed using OpenCV!');
Explanation:
HSV Conversion: The image is converted to the HSV color space to detect specific color ranges more effectively.
Masking: We create a mask that isolates the background (typically based on its color).
Foreground Extraction: By inverting the mask, we retain the foreground and discard the background.
Pros:
Fully offline.
More control over the image processing.
Open-source and free.
Cons:
Requires image processing expertise.
May not handle complex backgrounds as well as AI-powered solutions.
5. Using Pixel Manipulation with Sharp
Sharp is an image processing library that’s fast and supports various image manipulations. It can be used for basic background removal by manipulating pixel values.
Step 1: Install Sharp
npm install sharp
Step 2: Basic Background Removal Using Thresholding
Here’s how you can remove a simple background using sharp
:
const sharp = require('sharp');
const removeBackgroundWithSharp = async (inputPath, outputPath) => {
try {
// Read the image and apply a threshold
const { data, info } = await sharp(inputPath)
.threshold(200) // Adjust threshold value for background detection
.toBuffer({ resolveWithObject: true });
// Save the processed image with the background removed
await sharp(data, { raw: info })
.png()
.toFile(outputPath);
console.log('Background removed using Sharp!');
} catch (error) {
console.error('Error processing image with Sharp:', error);
}
};
removeBackgroundWithSharp('input.jpg', 'output.png');
Explanation:
Thresholding: This technique turns pixels above a certain brightness (typically background) into white and retains the rest.
Output: The resulting image is saved as a PNG with transparency.
Pros:
Easy to implement.
Supports many image formats.
Lightweight and fast.
Cons:
Works best with simple backgrounds.
May not be suitable for complex images.
Conclusion
Each method has its pros and cons, so choose the one that best fits your project's needs. With Node.js and the power of image processing libraries, you can integrate background removal seamlessly into your workflow.
Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
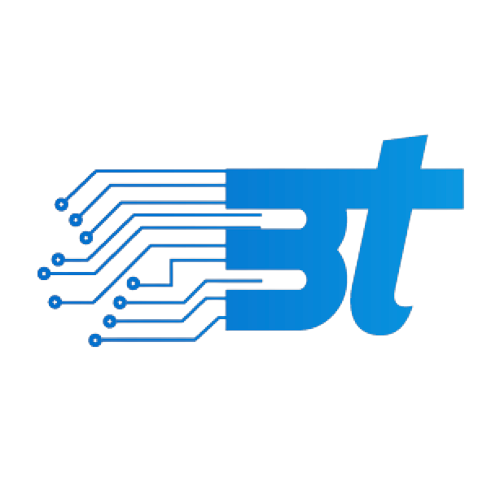
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.