How to Create Fake API JSON Data for Frontend Testing Using Golang

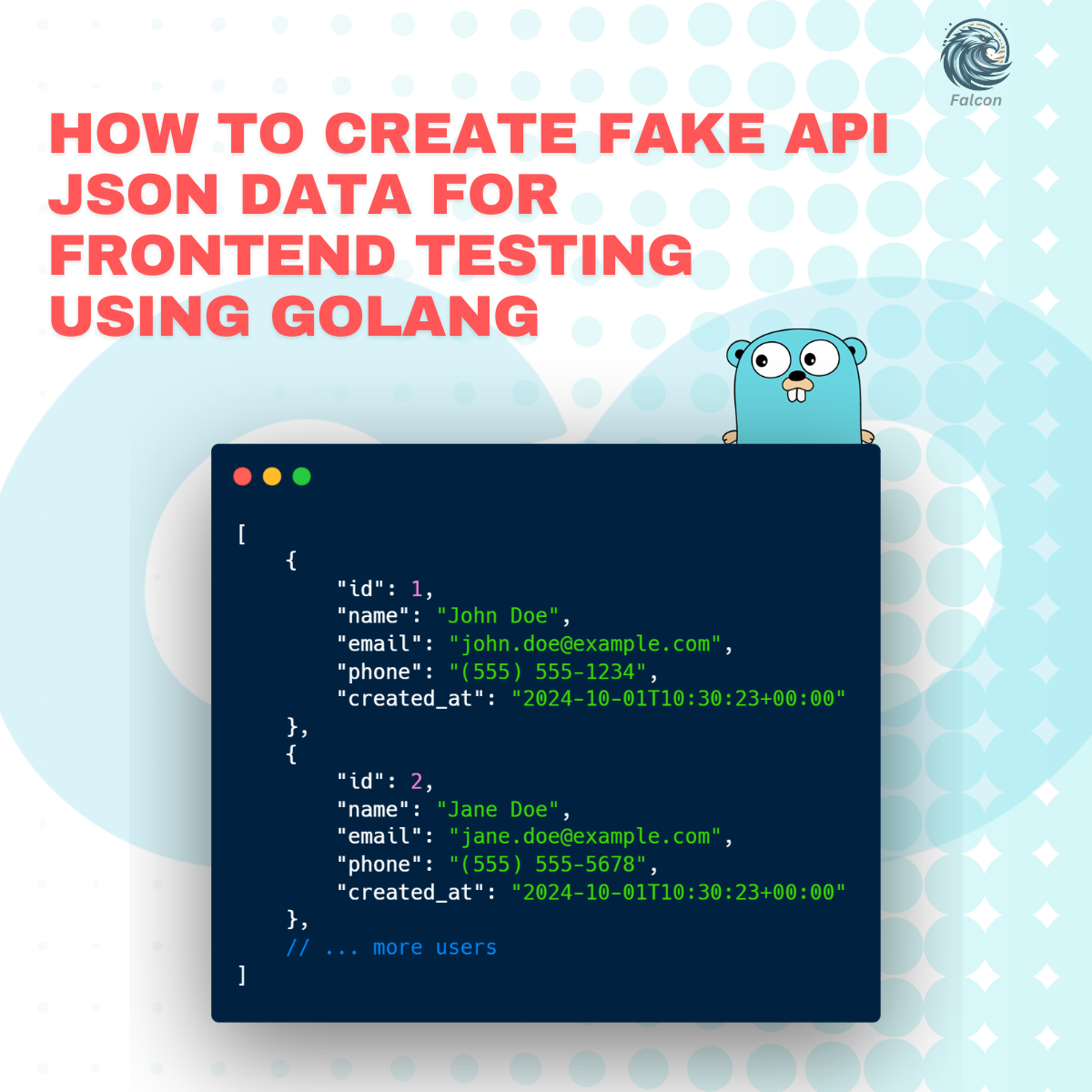
Testing frontend applications often requires mock data to simulate API calls before the backend is ready or when you need static data for development. In this article, I’ll show you how to create a simple API in Golang that serves fake JSON data, ideal for frontend testing.
We will be using the bxcodec/faker
package to generate realistic fake data and Go’s built-in HTTP server for serving the API. Let’s dive in!
Prerequisites
To follow this guide, you’ll need:
Go installed on your system. You can download it from the official site.
A code editor (VS Code, GoLand, etc.).
Basic knowledge of Go programming.
Step 1: Set Up Your Project
First, create a directory for your project and navigate into it:
mkdir fake-api && cd fake-api
Initialize a new Go module:
go mod init fake-api
Next, install the faker
package, which helps generate realistic fake data:
go get github.com/bxcodec/faker/v3
Step 2: Create a Simple HTTP Server
Now that the project is set up, let’s create a simple HTTP server in Go. This server will return a list of fake users as JSON.
Code for a Basic Fake User API:
package main
import (
"encoding/json"
"log"
"net/http"
"time"
"github.com/bxcodec/faker/v3"
)
// User represents a simple user model
type User struct {
ID int `json:"id"`
Name string `json:"name"`
Email string `json:"email"`
Phone string `json:"phone"`
CreatedAt string `json:"created_at"`
}
// generateFakeUser generates a fake user with realistic data
func generateFakeUser(id int) User {
return User{
ID: id,
Name: faker.Name(),
Email: faker.Email(),
Phone: faker.Phonenumber(),
CreatedAt: time.Now().Format(time.RFC3339),
}
}
// fakeUsersHandler handles the endpoint to return a list of fake users
func fakeUsersHandler(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
// Generate 50 fake users
numUsers := 50
users := make([]User, numUsers)
for i := 0; i < numUsers; i++ {
users[i] = generateFakeUser(i + 1)
}
// Encode the users slice to JSON and send the response
json.NewEncoder(w).Encode(users)
}
func main() {
// Create HTTP routes
http.HandleFunc("/api/users", fakeUsersHandler)
// Start the HTTP server
log.Println("Starting server on :8080...")
log.Fatal(http.ListenAndServe(":8080", nil))
}
Explanation of the Code:
User Struct: This struct defines the structure of the fake user JSON data.
generateFakeUser()
: This function creates a new user with realistic fake data using thefaker
package. It includes a fake name, email, phone number, and address.fakeUsersHandler()
: This function handles the/api/users
endpoint. It generates 50 users and encodes them as JSON for the API response.HTTP Server: The
http.HandleFunc()
binds the/api/users
endpoint to thefakeUsersHandler()
function, andhttp.ListenAndServe()
starts the server on port8080
.
Step 3: Running the Server
Once you’ve written the code, you can run the server:
go run main.go
The server will start on http://localhost:8080
. You can visit http://localhost:8080/api/users
in your browser or use tools like Postman or curl
to test the API.
Example request using curl
:
curl http://localhost:8080/api/users
Sample Output
Here’s an example of what the JSON response might look like:
[
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com",
"phone": "(555) 555-1234",
"created_at": "2024-10-01T10:30:23+00:00"
},
{
"id": 2,
"name": "Jane Doe",
"email": "jane.doe@example.com",
"phone": "(555) 555-5678",
"created_at": "2024-10-01T10:30:23+00:00"
},
// ... more users
]
Each user has a unique id
, name, email, phone number, and address. You can use this data in your frontend application to simulate API calls.
Step 4: Customizing the API
You can easily customize this fake API to suit your needs:
Changing the Number of Users:
To return a different number of users, simply modify thenumUsers
variable in thefakeUsersHandler
function.Different Data Models:
You can create additional structs to model different kinds of data (e.g., products, blog posts) and create corresponding endpoints.More Realistic Data:
Thefaker
package supports generating more complex data, such as IP addresses, dates, and custom fields. Check out faker's documentation for more details.
Conclusion
By setting up a simple fake API in Golang, you can quickly generate realistic JSON data for frontend testing. This approach is great for testing your frontend components when the backend is not ready or if you need mock data for prototyping.
Feel free to expand this API with additional endpoints or data models. Happy coding!
Subscribe to my newsletter
Read articles from Sachin Borse directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
