How To Design REST APIs: A Comprehensive Guide

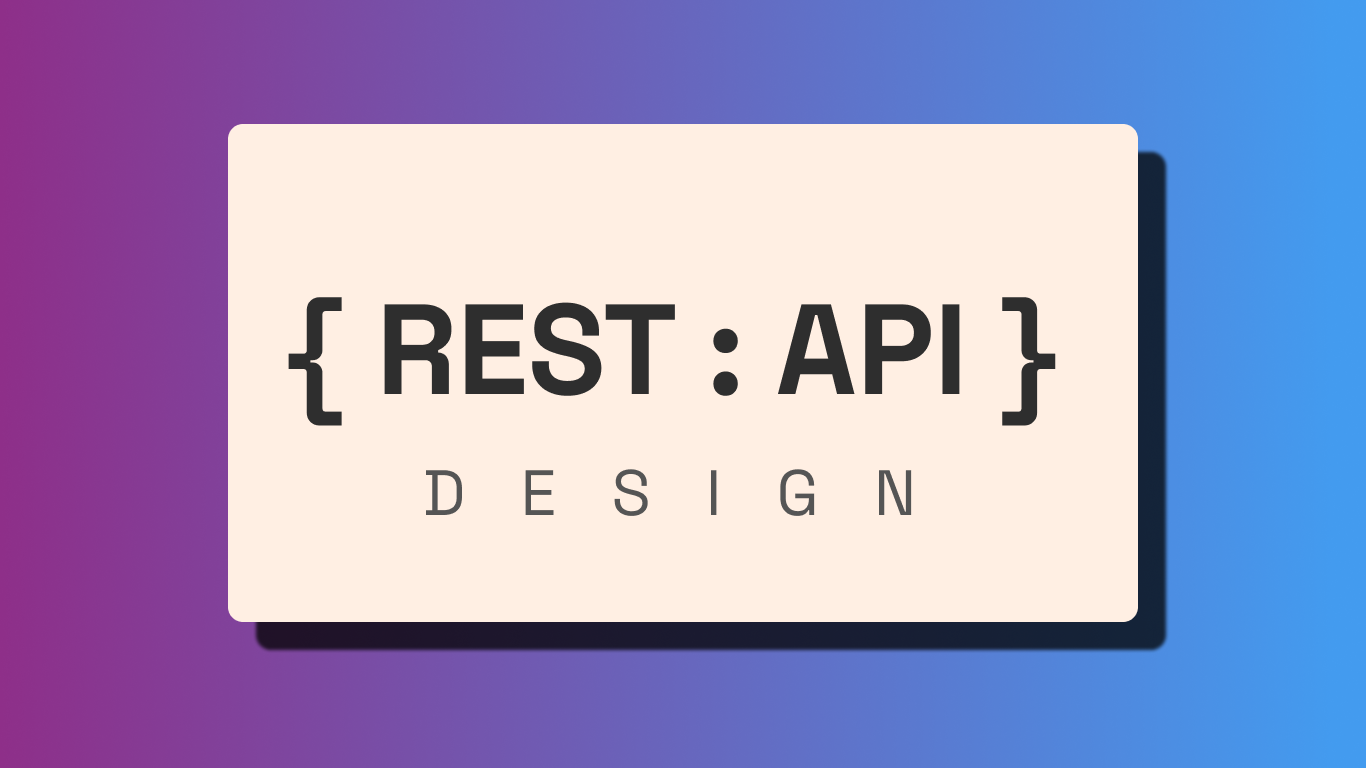
APIs have undeniably revolutionized the modern web landscape, fostering interoperability and modularity among applications. By enabling seamless communication and data exchange between different software systems, APIs have unlocked a realm of possibilities for developers worldwide. A prime example showcasing the transformative power of APIs is the Google Maps API, which provides developers with access to powerful geo-localization services, sparing them the need to develop such functionalities from scratch.
Numerous API types exist, ranging from SOAP, GraphQL, REST, gRPC, and more. However, in this article, we’ll center our discussion on REST, short for “Representational State Transfer,” which is the most prevalent and widely adopted API type in Web Development.
This article was originally written by “Ajimsimbom Bong Mbuyongha” at: https://numericaideas.com/blog/how-to-design-rest-apis-a-comprehensive-guide/
Who Is This For?
This article is tailored primarily for beginners venturing into the world of API development and those seeking comprehensive guidance on designing REST APIs. While the content is structured to cater to individuals with limited experience in API design, seasoned professionals are also encouraged to engage with the material, offering insights and feedback drawn from their extensive expertise.
Importance of REST API Design
Just like we design a database schema for a database or design the User Interface(UI) of an application before building them, it’s essential to take the same approach when developing REST APIs. This helps ensure that we create APIs that are easy to use and also speeds up the development process because we’ve already mapped out our plan in advance. By thinking ahead and mapping out the structure of our APIs, we can make the development process smoother and create APIs that meet users’ needs more effectively.
This article assumes you have a basic understanding of how REST APIs work. Knowledge on how to implement a simple REST API would be a plus.
The points discussed in this article stem from my research and observations of prevalent practices in REST API implementation. They’re not strict rules, but rather widely accepted best practices that you can choose to adopt. Now, let’s get into the details.
REST API Structure
Let’s imagine we’re creating an API for a blogging platform where users can write articles and leave comments. This scenario will serve as the foundation for all our examples moving forward.
For example:
Users endpoint:
example.com/api/users
Articles endpoint:
example.com/api/articles
💡 Note: We haven’t mentioned the ‘Reviews’ endpoint yet, as it involves nesting, which we’ll cover later on.
Dynamic Parameters
While resource endpoints typically return arrays of entities, sometimes we only need to interact with a single entity. In such cases, we can use dynamic parameters. These parameters rely on an ID that uniquely identifies a resource.
For example:
Endpoint for a single User:
example.com/api/users/{id}
Endpoint for a single Article:
example.com/api/articles/{id}
Here, the curly braces indicate that the value within them is dynamic, representing the ID of the specific entity we want to access.
HTTP Methods
In REST APIs, we use HTTP request methods to indicate the actions we want to perform on the API. Common methods include GET, POST, PUT, and DELETE. These methods define how we interact with resources.
In our blogging example:
Use GET to retrieve a list of articles (
/articles
) or a specific article (/articles/{id}
).Use POST to create a new article (
/articles
).Use PUT to update an existing article (
/articles/{id}
).Use DELETE to remove a specific article (
/articles/{id}
).
Each HTTP method corresponds to a specific action we want to take on the API. These methods are integral parts of the HTTP protocol and are used to communicate our intentions to the server when making requests.
Nested Resources
Sometimes, we need to work with resources that are related to other resources. For instance, we might want to access the reviews associated with a specific article or the articles published by a particular user. In such cases, we can use nested routes to represent these relationships:
Reviews for an Article:
example.com/api/articles/{id}/reviews
Articles published by a User:
example.com/api/users/{id}/articles
However, it’s important to avoid excessive nesting, as it can lead to overly complex API endpoints. For example, instead of having a deeply nested structure like /api/users/{id}/articles/{id}/reviews
:
We can simplify it to: /api/articles/{id}/reviews
.
By doing so, we maintain a cleaner and more intuitive API interface, making it easier for developers to work with our API.
Pagination and Filtering
Often, we need to paginate our API endpoints to manage data efficiently and keep our system running smoothly. Additionally, clients may want to search for specific information or filter the results they receive. So, how do we accomplish this? The answer lies in query strings.
Query strings allow us to include additional information in a request using key-value pairs. This is particularly useful for implementing pagination and searching in APIs. Let’s take a look at some examples:
Pagination: To paginate results, we can specify the page number and the number of elements per page in the query string.
Example:
/api/articles?page=0&size=10
- Here,
page=0
indicates the first page, andsize=10
specifies that we want 10 articles per page.
- Here,
Filtering: We can filter results based on specific criteria by including relevant parameters in the query string.
Example:
/api/articles?title=api
- This query returns articles with “api” in their title.
Pagination + Filtering: We can combine pagination and filtering by including both pagination and filtering parameters in the query string.
Example:
/api/articles?page=0&size=10&title=api
- This query returns the first page of articles, with 10 articles per page, containing “api” in their title.
By leveraging query strings in this way, we can provide clients with the flexibility to paginate, search, and filter data according to their needs, enhancing the usability and effectiveness of our API.
Other Best Practices
Now, let’s explore additional considerations for API development beyond just the endpoint structure.
Status Codes
HTTP status codes indicate whether an HTTP request has been completed successfully. Using status codes is crucial in providing a concise way of communicating the results of a request, as each code describes exactly what happened or what action needs to be taken. Status codes are grouped into five classes:
Informational responses (
100
–199
)Successful responses (
200
–299
)Redirection messages (
300
–399
)Client error responses (
400
–499
)Server error responses (
500
–599
)
Some commonly used status codes in REST API responses include:
200 (OK): Indicates that the request was successful.
201 (Created): This shows that a new resource has been successfully created.
400 (Bad Request): Signifies that the request sent by the client is invalid or malformed.
401 (Unauthorized): Indicates that the client needs to authenticate itself to access the resource.
404 (Not Found): Shows that the requested resource does not exist.
500 (Internal Server Error): Indicates that an unexpected error occurred on the server.
You can learn more about status codes from the MDN Documentation
Response Design
Consistency in our API’s data format is key for clients to easily understand and handle the information they receive. While the specific format can vary based on our needs and API structure, maintaining uniformity across all endpoints is crucial. JSON and XML are common data representation formats used in REST APIs. JSON is the newer format, and more widely adopted because of its simplicity and readability. Here’s an example of the response data we might receive when making a GET request to example.com/api/users
in JSON:
{
"success": true,
"message": "Success",
"data": [
{
"id": 1,
"title": "Sample Article",
"content": "Lorem ipsum dolor sit amet..."
},
{
"id": 2,
"title": "Another Article",
"content": "Sed ut perspiciatis unde omnis iste..."
}
],
"pagination": {
"size": 10,
"page": 1,
"total": 5
},
"filters": {
"title": "api"
}
}
In this example:
“status” indicates if the request was successful (200) or not.
“message” provides a description corresponding to the status.
“data” contains the list of articles. If there’s an error, we maintain the same structure, providing a detailed error message in “data”.
“pagination” provides metadata for the total number of pages, current page, and number of items per page. This helps clients navigate through paginated results more efficiently.
“filter” is metadata for clients to understand how the filtering was done.
Ensuring consistency will simplify client-side parsing and improve overall usability.
API Versioning
User and business requirements evolve over time, and updating endpoints for an actively used API can cause disruptions and frustration for users. To avoid such issues, we implement API versioning.
API versioning allows us to maintain older implementations of our API while introducing updates in new versions. This ensures that existing applications relying on our API remain functional, while still allowing us to make necessary improvements. A common convention for API versioning is to include the version number in the endpoint URL:
Version 1 of the Articles Endpoint:
example.com/api/v1/articles
Version 2 of the Articles Endpoint:
example.com/api/v2/articles
By following this convention, we specify the version number immediately after the /api
route. Another approach is to specify the version number as a query string, providing flexibility in versioning strategies.
Authentication and Authorization
Authentication verifies the identity of users accessing an API, ensuring they are who they claim to be. This is typically done through credentials such as usernames and passwords, tokens, or API keys.
Authorization, on the other hand, determines what actions users are allowed to perform once they have been authenticated. It specifies the level of access granted to each user based on their role or permissions.
For example, in a blogging API:
Authentication ensures that only registered users can access their accounts.
Authorization determines whether a user can create, edit, or delete articles based on their role (e.g., admin, author, reader).
Documentation
Documentation is essential for ensuring that developers understand how to use an API effectively. It provides clear instructions on endpoints, request parameters, response formats, and error handling. Clear and comprehensive documentation simplifies API integration for developers. A standard widely used in documenting APIs is the OpenAPI Specification(formerly Swagger), other standards include API Blueprint and RAML.
Conclusion
In this guide, we’ve covered the importance of API design and discussed various conventions and best practices for designing REST APIs. We explored concepts such as dynamic parameters, HTTP methods, nesting, API versioning, documentation, authentication, and authorization. By following these guidelines, developers can create well-structured and user-friendly APIs that meet the needs of their applications and users. I hope this guide has provided you with a comprehensive overview of REST API design. If you have any feedback or suggestions, please feel free to leave them in the comments or reach out to me directly.
Thanks for reading this article. Like, recommend, and share if you enjoyed it. Follow us on Facebook, Twitter, and LinkedIn for more content.
Subscribe to my newsletter
Read articles from Ajimsimbom Bong M directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ajimsimbom Bong M
Ajimsimbom Bong M
I'm Ajim, a Cloud DevOps Engineer from Cameroon. I'm really into Cloud Native Solutions involving Microservices, Containers, and Kubernetes. Feel free to reach out to me if you wish to connect or collaborate on a project.