Java main() Method
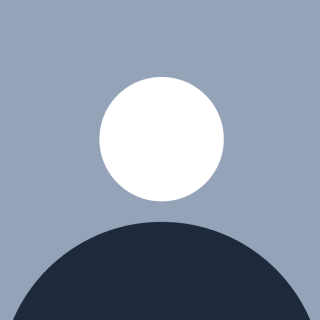
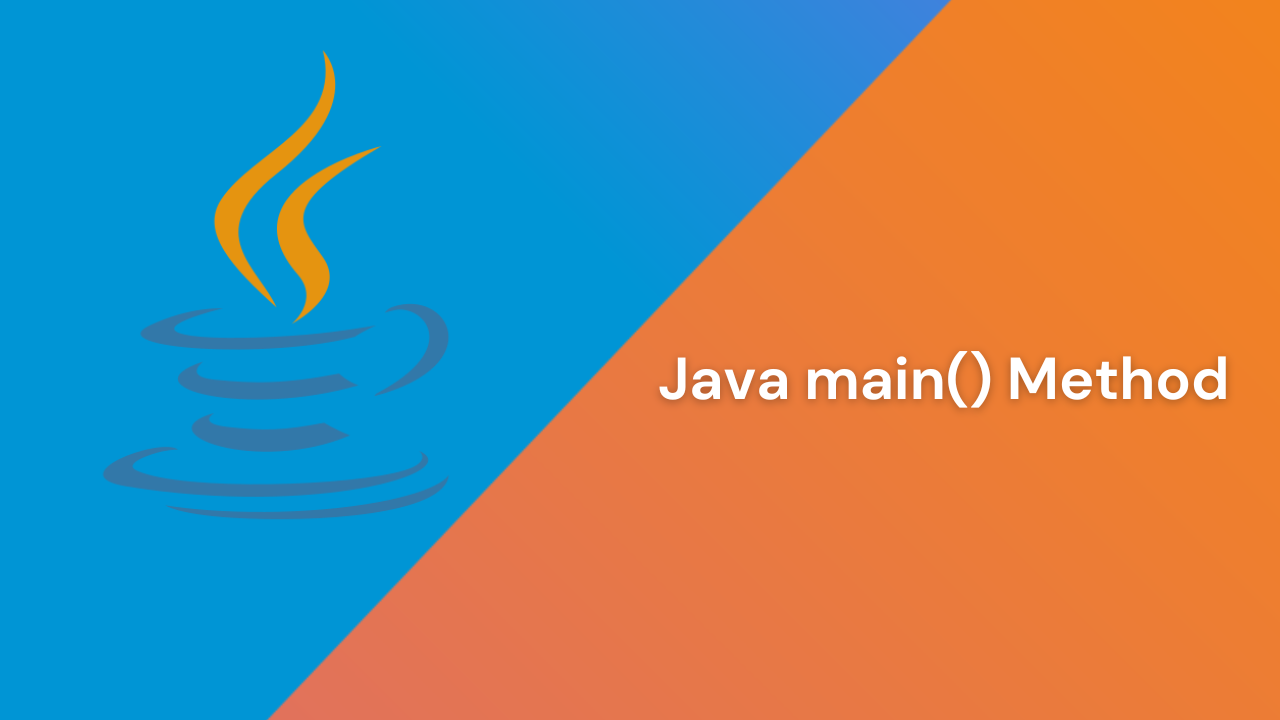
The main()
method in Java is the entry point for any standalone application, called by the JVM to start program execution. It must be public, static, and void, with a String[]
parameter for command-line arguments. The method's syntax is strict, but some variations are allowed, such as different orders of modifiers and using varargs. Overloading the main()
method is possible, but the JVM will only call the standard signature. The main()
method can also be inherited or hidden in subclasses. Understanding its requirements is essential for writing and running Java programs.
Syntax of the main()
Method
The standard syntax of the main()
method is as follows:
public static void main(String[] args) {
// method body
}
Breakdown of the Syntax
public: The
main()
method must be public so that the JVM can access it from outside the class.static: The
main()
method must be static so that it can be called without creating an instance of the class. This is necessary because the JVM needs to call this method before any objects are created.void: The
main()
method does not return any value.main: This is the name of the method that the JVM looks for as the entry point of the program.
String[] args: This is an array of
String
objects that stores command-line arguments passed to the program.
Importance of the main()
Method
The main()
method is the starting point of any Java application. When you run a Java program, the JVM searches for the main()
method with the exact signature public static void main(String[] args)
. If it doesn't find this method, it throws a NoSuchMethodError
.
Example
Let's look at the following example:
public class Test {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In this example, the main()
method prints "Hello, World!" to the console. When you run this program, the JVM calls the main()
method to start the execution.
Common Questions and Misconceptions
Can We Change the Name of the main()
Method?
No, the name of the main()
method cannot be changed. The JVM specifically looks for the method named main
with the exact signature public static void main(String[] args)
.
Why is the main()
Method Public?
The main()
method is public so that the JVM can access it from outside the class. If it were not public, the JVM would not be able to call it, and the program would not run.
Why is the main()
Method Static?
The main()
method is static because it needs to be called without creating an instance of the class. The JVM calls the main()
method before any objects are created, so it must be static.
Why is the Return Type of the main()
Method Void?
The main()
method has a return type of void because it does not return any value to the JVM. The purpose of the main()
method is to start the execution of the program, not to return a value.
Can We Change the Parameter of the main()
Method?
The parameter of the main()
method must be a String
array. However, you can change the name of the parameter. For example, the following is valid:
public static void main(String[] args) {
// method body
}
Can We Overload the main()
Method?
Yes, you can overload the main()
method, but the JVM will only call the main()
method with the signature public static void main(String[] args)
. Other overloaded versions of the main()
method will not be called by the JVM.
public class Test {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
public static void main(int[] args) {
System.out.println("Overloaded main method");
}
}
In this example, the JVM will call the main(String[] args)
method, not the main(int[] args)
method.
Acceptable Variations
While the syntax of the main()
method is strict, some variations are acceptable:
Order of Modifiers: The order of
public
andstatic
can be interchanged.static public void main(String[] args) { // method body }
String Array Declaration: The
String
array can be declared in different forms.public static void main(String args[]) { // method body }
Varargs: You can use varargs instead of a
String
array.public static void main(String... args) { // method body }
Final, Synchronized, and Strictfp Modifiers: The
main()
method can also be declared withfinal
,synchronized
, andstrictfp
modifiers.public static final synchronized strictfp void main(String[] args) { // method body }
Additional Cases and Concepts
Case 1: Overloading the main()
Method
Overloading the main()
method is possible, but the JVM will always call the main(String[] args)
method.
public class Test {
public static void main(String[] args) {
System.out.println("String array main method");
}
public static void main(int[] args) {
System.out.println("Integer array main method");
}
}
In this example, even though there are two main()
methods, the JVM will call the one with the String[]
parameter.
Case 2: Inheritance and the main()
Method
Inheritance is applicable to the main()
method. If a child class does not have its own main()
method, it will inherit the main()
method from its parent class.
public class Parent {
public static void main(String[] args) {
System.out.println("Parent main method");
}
}
public class Child extends Parent {
// No main method
}
Running Child
will execute the main()
method from Parent
.
Case 3: Method Hiding with the main()
Method
If both the parent and child classes have a main()
method, the child's main()
method will hide the parent's main()
method.
public class Parent {
public static void main(String[] args) {
System.out.println("Parent main method");
}
}
public class Child extends Parent {
public static void main(String[] args) {
System.out.println("Child main method");
}
}
Running Child
will execute the main()
method from Child
, not Parent
. This is known as method hiding, not method overriding, because main()
is a static method.
Simplified main()
Method in Java 21 and 22
The Java programming language has undergone significant simplification in recent versions, and one notable change is the transformation of the main()
method. Although this feature is still in preview mode until at least Java 23, it promises to streamline the way we write entry points for Java programs.
Traditional main()
Method
In earlier versions of Java, writing a simple "Hello World" program required a fair amount of boilerplate code. For example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In this traditional approach, you had to:
Define a class: The
HelloWorld
class.Declare the
main()
method: The method had to bepublic static
.Handle command-line arguments: The
String[] args
parameter.Use
System.out
: To print to the console.
In Java 21 and Java 22 (with preview features enabled), the code becomes much simpler:
void main() {
System.out.println("Hello world!");
}
Key Simplifications
No Class Declaration: You no longer need to define a class explicitly. The compiler implicitly creates a class for you.
No
public static
Modifiers: Themain()
method does not need to be declared aspublic static
. The method stands alone.No Explicit
System.out
Reference: Although the example still usesSystem.out.println
, future versions may further simplify this by allowing direct calls toprintln()
.
How It Works
Implicitly Declared Classes
For Java files without an explicit class declaration, the compiler will generate an "implicit class" named after the file (minus the .java
extension). For instance, compiling HelloWorld.java
will create HelloWorld.class
.
Characteristics of implicit classes include:
They reside in the unnamed package.
They are generally final and cannot be inherited.
They cannot implement interfaces or extend other classes.
They cannot be accessed by name from other classes.
Instance Main Methods
Instance main methods are non-static main
methods. Future Java versions will allow:
Non-static instance methods.
Methods with public, protected, or package-private visibility.
Methods with or without
String[]
parameters.
Examples include:
void main()
void main(String[] args)
public void main()
protected static void main(String[] args)
Static and non-static methods with the same signature, or methods with different visibility modifiers with the same signature, will lead to a compiler error. However, a main
method with a String[]
parameter and one without can coexist in the same file, with the JVM prioritizing the method with the String[]
parameter.
Benefits
Reduced Boilerplate: This simplification reduces the amount of boilerplate code, making it easier for beginners to write and understand Java programs.
Focus on Logic: Developers can focus more on the logic of their programs rather than the structure required to run them.
Future Prospects
Looking ahead to future versions like Java 23, the simplification may go even further, potentially eliminating the need for System.out
entirely:
void main() {
println("Hello world!");
}
This would make the code even more concise and beginner-friendly.
Summary
The main()
method in Java is the entry point for any standalone application, called by the JVM to start program execution.
It must be public, static, and void, with a String[]
parameter for command-line arguments.
public: Allows the JVM to access it from outside the class.
static: Enables calling without creating an instance of the class.
void: Indicates it doesn't return any value.
main: The method name the JVM looks for.
String[] args: Stores command-line arguments.
The main()
method is essential because the JVM looks for it to start your program. If it doesn't find it, you'll get a NoSuchMethodError
.
Common Questions:
Can we change the name of the
main()
method? No, the JVM specifically looks formain
.Why is it public? So the JVM can access it.
Why is it static? It needs to be called before any objects are created.
Why is the return type void? It doesn't return any value.
Can we change the parameter? Yes, but it must be a
String
array.Can we overload it? Yes, but the JVM will only call the standard signature.
Acceptable Variations:
Order of Modifiers:
public static
orstatic public
.String Array Declaration: Different forms are allowed.
Varargs: You can use varargs instead of a
String
array.Additional Modifiers:
final
,synchronized
, andstrictfp
are allowed.
Advanced Concepts:
Overloading: Possible, but the JVM calls the standard
main(String[] args)
.Inheritance: A child class can inherit the
main()
method.Method Hiding: A child class can hide the parent's
main()
method.
Conclusion
The main()
method is a fundamental part of any Java application. It serves as the entry point for program execution and must follow a specific syntax to be recognized by the JVM. Understanding the main()
method and its requirements is essential for writing and running Java programs successfully.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer