Java Print Method: System.out.println
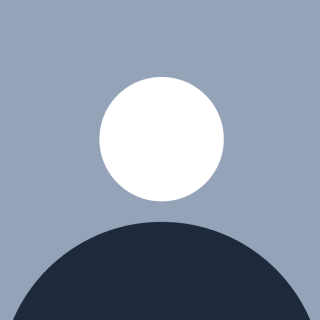
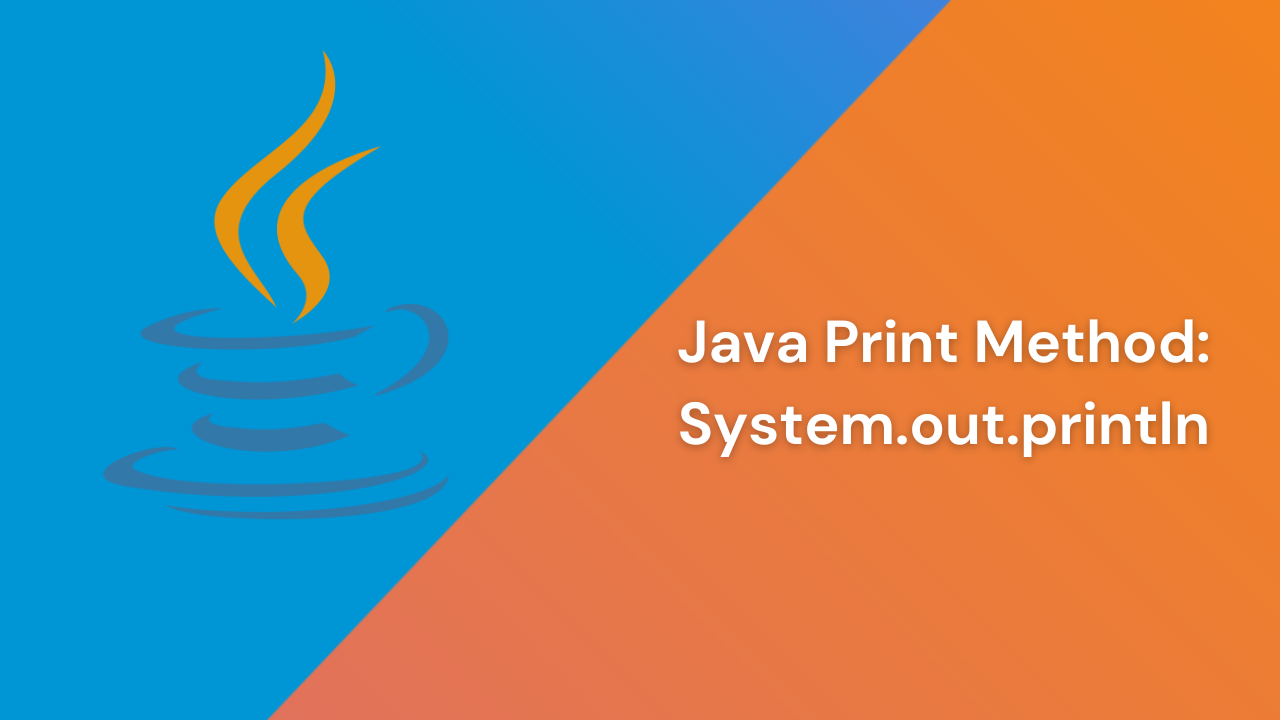
System.out.println
in Java is a method used to print output to the console. It involves three main components: the System
class, the out
field, and the println
method. The System
class provides standard input, output, and error streams, among other utilities. The out
field is a static final instance of PrintStream
, initialized by the JVM using a native method. The println
method in PrintStream
is overloaded to handle various data types and prints the provided string followed by a new line. Understanding its internal workings reveals Java's efficient design for handling standard output.
Introduction
System.out.println
is one of the most commonly used methods in Java for printing output to the console. Despite its frequent use, many developers are not fully aware of its internal workings. This article aims to provide a detailed explanation of the System.out.println
method, its components, and the underlying mechanisms that make it function.
To Print "Hello, World!"
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Define a class: Create the
HelloWorld
class.Declare the
main()
method: Ensure the method ispublic static
.Handle command-line arguments: Use the
String[] args
parameter.Use
System.out
: To print to the console.
Components of System.out.println
When you write System.out.println("Hello, World!");
, you are using three main components:
System: A final class in the
java.lang
package.out: A static final field of the
System
class, which is an instance ofPrintStream
.println: A method of the
PrintStream
class.
The System
Class
The System
class is a part of the java.lang
package and contains several useful class fields and methods. It cannot be instantiated because its constructor is private. This class provides various facilities such as:
Standard input, output, and error output streams.
Access to externally defined properties and environment variables.
Methods for loading files and libraries.
Utility methods for quickly copying a portion of an array.
The out
Field
The out
field is a static and final instance of PrintStream
. It is declared in the System
class as follows:
public final static PrintStream out = null;
Initially, out
is set to null
. However, it is later initialized by the JVM when the System
class is loaded. This initialization is done using a native method, which we'll discuss later.
The PrintStream
Class
PrintStream
is a class that provides methods to print various data values conveniently. The println
method is overloaded to handle different types of data, such as strings, integers, and booleans. Here is a simplified version of the println
method:
public void println(String x) {
synchronized (this) {
print(x);
newLine();
}
}
This method ensures that the string x
is printed to the console, followed by a new line.
Initialization of System.out
The out
field in the System
class is initialized by the Java Virtual Machine (JVM) using a native method. This process involves the Java Native Interface (JNI), which allows Java code to interact with native applications and libraries written in other languages like C or C++.
The setOut
Method
The System
class has a method called setOut
, which is used to reassign the standard output stream. Here is the method definition:
public static void setOut(PrintStream out) {
setOut0(out);
}
This method calls another method, setOut0
, which is a native method:
private static native void setOut0(PrintStream out);
The Native Method
The actual implementation of setOut0
is written in C and is part of the JVM. This method is responsible for setting the out
field to an instance of PrintStream
. The native method ensures that the out
field is properly initialized to handle output operations.
How println
Works
When you call System.out.println
, the following sequence of events occurs:
Class Loading and Initialization: The
System
class is loaded by the JVM, and its static fields, includingout
, are initialized. Theout
field is set to an instance ofPrintStream
via thesetOut0
native method.Setting the
out
Field: ThesetOut0
native method sets theout
field to an instance ofPrintStream
. This instance is configured to handle standard output operations.Calling
println
: Theprintln
method of thePrintStream
instance is called. This method is responsible for printing the provided string to the console. Theprintln
method is overloaded to handle different types of data, such as strings, integers, and booleans. Here is a simplified version of theprintln
method:public void println(String x) { synchronized (this) { print(x); newLine(); } }
print(x): This method prints the string
x
to the console.newLine(): This method adds a new line after the printed string.
Conclusion
Understanding the internal workings of System.out.println
can give you a deeper appreciation of Java's design and its interaction with the underlying system. The combination of Java code and native methods allows for efficient and flexible handling of standard output, making System.out.println
a powerful tool for developers. By delving into its components and initialization process, developers can gain insights into Java's robust architecture and improve their coding practices.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer