Unlocking the Shell: Essential Scripting Techniques - Part 1
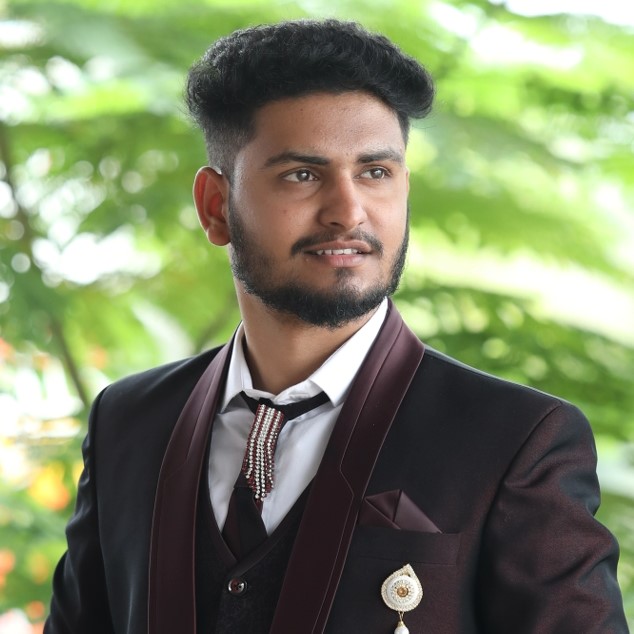
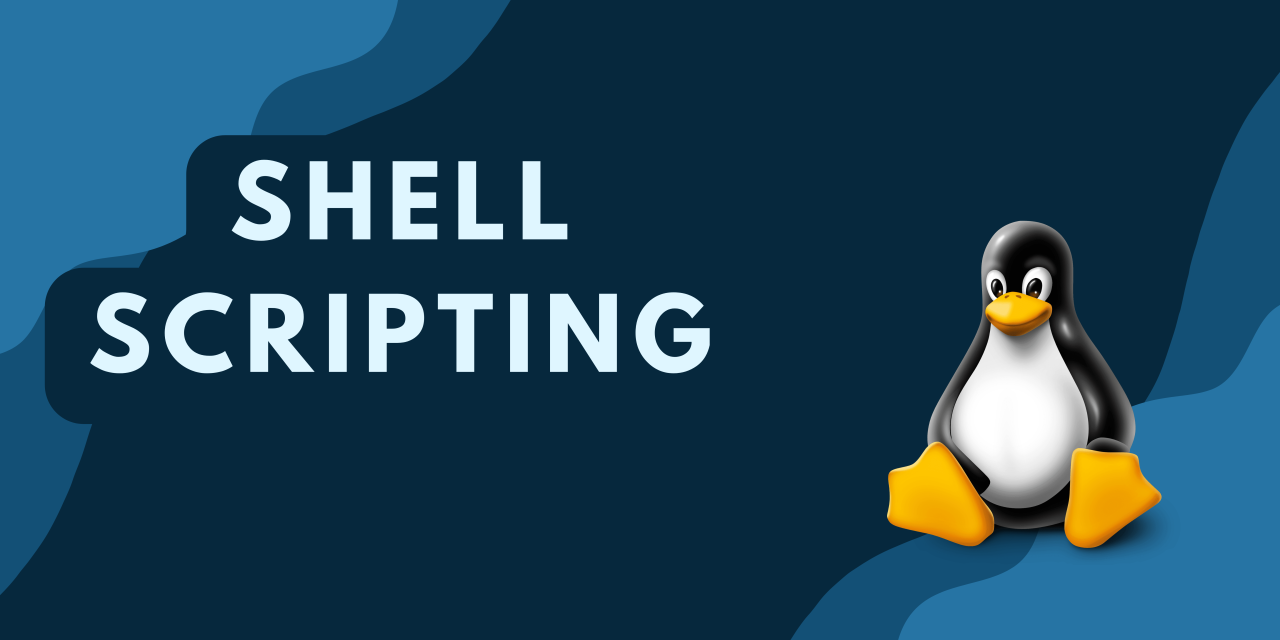
Introduction to Linux Shell and Shell Scripting
When we use any major operating system, we usually interact with the shell without even knowing it. For instance, when we use Ubuntu or other Linux versions, we use the terminal to talk to the shell. In this article, we'll look at Linux shells and shell scripting. But first, let’s learn a few important terms:
Kernel
Shell
Terminal
Getting Started with Shell Scripting
Shell scripting is a way to automate tasks in Unix or Linux by writing commands in a text file. These commands are read by a shell, like Bash, which runs them one after another.
Each shell script is saved with .sh
file extension e.g. Abc.sh
To check which shell you are using: echo $0
Why Do We Need Shell Scripts?
Shell scripts are useful for several reasons:
Automation: They help avoid repetitive tasks.
Routine Tasks: System administrators use them for backups and monitoring.
Enhanced Functionality: Shell scripts can add new features to the shell.
Advantages:
Familiar Commands: They use the same commands as the command line, making them easy for programmers.
Faster Writing: Creating scripts is quicker than typing commands one by one.
Quick Start and Debugging: Easy to start and debug interactively
Disadvantages:
Risk of Errors: A small mistake can cause problems.
Slower Performance: They may run slower compared to other languages.
Complex Tasks: Not ideal for large, complicated tasks.
Limited Data Structures: They don’t offer as many data structures as other scripting languages.
Let’s Get Started
What is Shebang
A shebang is a special line at the beginning of a script that tells the operating system which interpreter to use to run the script. It starts with #!
followed by the path to the interpreter, like #!/bin/bash
for a Bash script. In simple terms, the shebang tells your computer how to execute the script you’ve written.
First Script
Create a file names script.sh
#!/bin/bash
echo "Hello World!"
How to run a Script
Make sure script has execute permission rwx.
Comments
Using #
# This is a single line comment
--Multi-line Comment--
<<comment
...
your comment here
...
comment
Variables
To define variables in a shell script, Use below syntax:
Var_Name=value
Var_Name=$(Command_whose_op_will_be_stored_in_Var)
To Print the value of variable:
- echo $Var_Name
Constant Variable
Once you defined a variable and don’t wanna change it until end of the script.
- readonly Var_Name=”value”
#!/bin/bash
# Define a variable
Var1="Rohan"
Var2=$(pwd)
# Define a Constant Variable
readonly Id="123"
# Use the variable
echo "My name is $Var1"
echo "My working directory is $Var2"
echo "my ID is $Id"
Arrays
In shell scripts, arrays allow you to store multiple values in a single variable. It is a collection of items which are accessible using an index.
Defining Arrays
To define an array in a shell script, you can use parentheses ()
to enclose the values.
Example
#!/bin/bash
# Define an array
myList=( "Red" "Black" "Orange" )
# Access elements of the array using index
echo "First element: ${myList[0]}"
echo "Second element: ${myList[1]}"
# Get the length of the array
echo "Total elements: ${#myList[*]}"
# Loop through the array
echo "All elements:"
for ele in "${myList[*]}"; do
echo "$ele"
done
# Get specific values
echo "${myList[*]:1}" # Get value from 1st index
echo "${myList[*]:2:2}" # Get 2 value from index 2
# Update an Array
myList+=( 1 2 3 )
String Operations
Strings are sequences of characters that can be manipulated in various ways. You can perform operations like concatenation, substring extraction, and length calculation and many more.
Common String Operations
Concatenation: Joining two or more strings.
Length: Finding the length of a string.
Substring Extraction: Extracting a part of a string.
Replacing Substrings: Replacing part of a string with another.
Example
#!/bin/bash
# Define strings
str1="Hello"
str2="World!"
# Concatenation
myStr="$str1, $str2"
echo "Concatenated String: $myStr"
# Length of a string
length=${#myStr}
echo "Length of Greeting: $length"
# Substring extraction (first 5 characters)
substring=${myStr:0:5}
echo "Substring: $substring"
# Replace a substring
new_string=${myStr/World/Shell}
echo "Replaced String: $new_string"
#Upper-case
uppercase="${myStr^^}" # or $(echo "$myStr" | awk '{print toupper($0)}')
echo "Uppercase: $uppercase"
# Lower-case
lowercase="${myStr,,}" # or $(echo "$myStr" | awk '{print tolower($0)}')
echo "Lowercase: $lowercase"
User Interaction
User interaction in shell scripts refers to the way a script can communicate with users, allowing them to provide input or respond to prompts. This makes scripts more dynamic and flexible, enabling them to adapt based on user choices.
Common Methods of User Interaction
Reading Input: Using the
read
command to get input from the user.Prompts: Displaying messages that ask for user input.
Syntax:
read <Var_Name
read -p “Message” Var_Name
Example:
#!/bin/bash
# Prompt the user for their name
echo "What is your name?"
read name
# Greet the user
echo "Hello, $name!"
# Ask for their favorite color
read -p "Your City" City
echo "Your city is $City"
Arithmetic Operations
You can perform arithmetic operations using different methods, including built-in commands and external tools. The most common ways to do arithmetic in shell scripts are using expr
, (( ))
, and let
.
Example
#!/bin/bash
# Define two numbers
num1=10
num2=5
# Using expr
sum_expr=$(expr $num1 + $num2)
echo "Using expr: Sum = $sum_expr"
# Using (( ))
difference=$((num1 - num2))
echo "Using (( )): Difference = $difference"
# Using let
let product=num1*num2
echo "Using let: Product = $product"
# Modulus using expr
remainder=$(expr $num1 % $num2)
echo "Using expr: Remainder = $remainder"
Conditional Statements
In shell scripting, conditional statements and loops are fundamental constructs that allow you to control the flow of your scripts. Here’s an overview of the available conditional statements and loops in shell scripts.
if-else Statement
Basic Syntax:
if [ condition ]; then # Code if condition is true else # Code if condition is false fi
elif Statement
Allows multiple conditions:
if [ condition1 ]; then # Code if condition1 is true elif [ condition2 ]; then # Code if condition2 is true else # Code if all conditions are false fi
Example:
#!/bin/bash number=10 if [ "$number" -gt 10 ]; then echo "The number is greater than 10." elif [ "$number" -eq 10 ]; then echo "The number is equal to 10." else echo "The number is less than 10." fi
case Statement
Useful for multiple options:
case variable in pattern1) # Code for pattern1 ;; pattern2) # Code for pattern2 ;; *) # Default case ;; esac
Example:
#!/bin/bash echo "Choose an option" echo "a = To see the current date" echo "b = list all the files in current dir" read choice case $choice in a) date;; b) ls;; *) echo "Non a valid input" esac
Looping Statements
for Loop
Iterates over a list of items:
for item in list; do # Code to execute for each item done
Example:
for i in {1..5}; do echo "Number $i" done
while Loop
Continues as long as a condition is true:
while [ condition ]; do # Code to execute while condition is true done
Example:
count=1 while [ $count -le 5 ]; do echo "Count is $count" ((count++)) done
until Loop
Continues until a condition is true (opposite of while):
until [ condition ]; do # Code to execute until condition is true done
Example:
count=1 until [ $count -gt 5 ]; do echo "Count is $count" ((count++)) done
Looping through Arrays
You can also loop through arrays:
# Define an array
array=(one two three)
# Loop through array
for item in "${array[@]}"; do
echo "$item"
done
Combining Conditions and Loops
#!/bin/bash
count=1
while [ $count -le 5 ]; do
if [ $count -eq 3 ]; then
echo "Three is my favorite number!"
else
echo "Count is $count"
fi
((count++))
done
Summary
This article introduces Linux Shell and Shell Scripting, covering essential concepts like the kernel, shell, and terminal. It explains how to get started with shell scripting, including creating and running scripts, using variables, arrays, and string operations. Additionally, it addresses user interaction, arithmetic operations, conditional and looping statements, and provides examples for each. For more scripting insights, connect with the author on LinkedIn: Rohan Tambat.
Contact
If you liked this post and would like to stay updated on my work, feel free to connect with me on LinkedIn: Rohan Tambat
Subscribe to my newsletter
Read articles from Rohan Tambat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
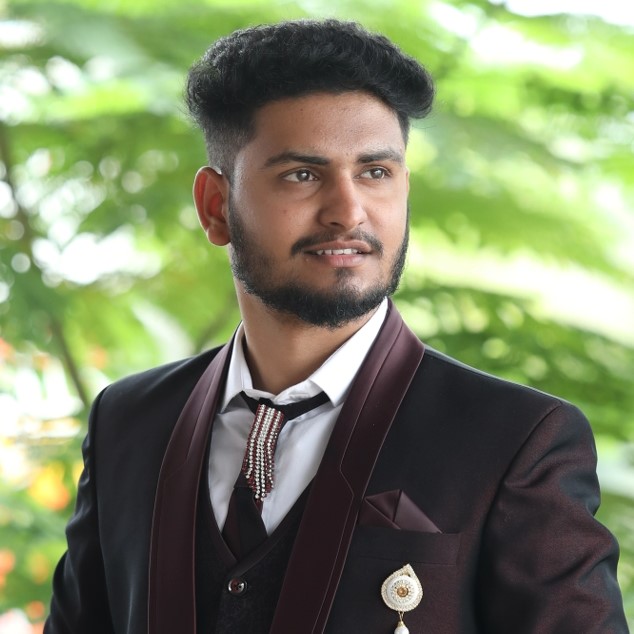
Rohan Tambat
Rohan Tambat
You can reach out to me on: rohantambat8@gmail.com