Understanding Spring Boot Annotations: A Comprehensive Guide

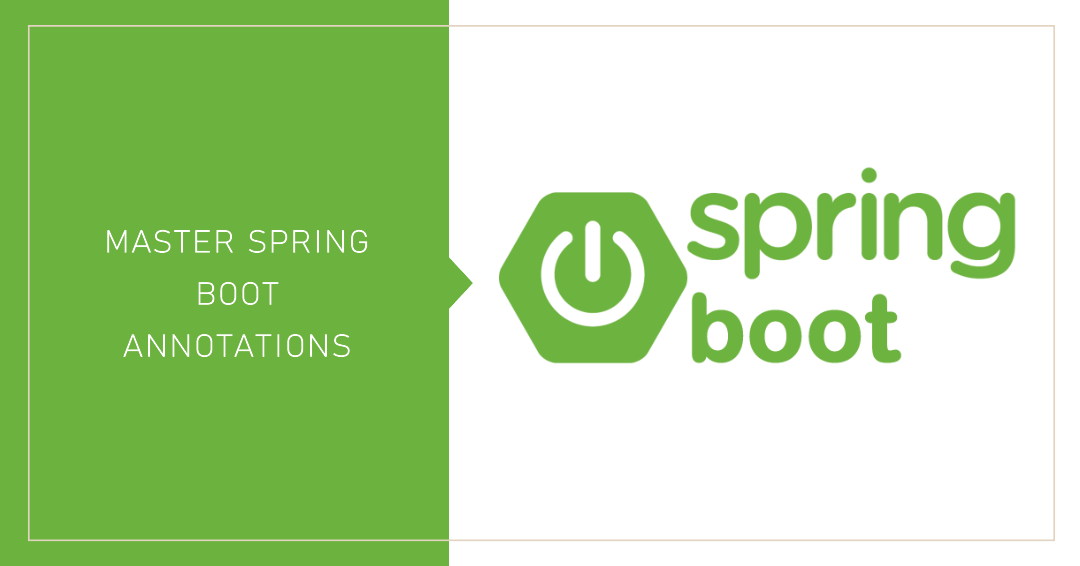
Understanding Spring Boot Annotations: A Comprehensive Guide
Spring Boot is widely known for simplifying Java application development, and one of its most powerful features is its extensive use of annotations. Annotations in Spring Boot significantly reduce boilerplate code by providing metadata to the Spring framework, allowing it to manage components automatically.
In this blog, we'll explore some of the most commonly used annotations in Spring Boot and how they make application development faster and more intuitive.
1. @SpringBootApplication
The @SpringBootApplication
annotation is the starting point of any Spring Boot application. It is used at the main class level, and it combines three annotations:
@Configuration
@EnableAutoConfiguration
@ComponentScan
Breakdown:
@Configuration
: Marks the class as a source of bean definitions for the Spring container.@EnableAutoConfiguration
: Enables Spring Boot’s auto-configuration mechanism to automatically configure your application based on the dependencies in your classpath.@ComponentScan
: Automatically scans the components in the specified package (or the package where the main class resides) and registers them in the Spring context.
Example:
@SpringBootApplication
public class MySpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
}
This annotation streamlines the configuration and startup process by eliminating the need for manual configuration of individual components.
2. @RestController
The @RestController
annotation is used to mark a class as a Spring MVC controller where every method returns a domain object instead of a view. It is a combination of @Controller
and @ResponseBody
.
Breakdown:
@Controller
: Indicates that the class is a web controller.@ResponseBody
: Ensures that the response from each method is written directly to the HTTP response body, typically used for REST APIs.
Example:
@RestController
public class MyController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
In this example, @RestController
tells Spring Boot that this class is responsible for handling HTTP requests, and the response will be returned as JSON or plain text instead of rendering a view.
3. @RequestMapping / @GetMapping / @PostMapping
Spring Boot simplifies request handling through specialized annotations like @RequestMapping
, @GetMapping
, @PostMapping
, and more.
- @RequestMapping: This is a versatile annotation that can map web requests to specific handler methods or classes. It supports HTTP methods like GET, POST, PUT, DELETE, etc.
- @GetMapping: Specifically maps HTTP GET requests to a method.
- @PostMapping: Specifically maps HTTP POST requests to a method.
Example of @RequestMapping:
@RestController
@RequestMapping("/api")
public class ApiController {
@RequestMapping(value = "/greet", method = RequestMethod.GET)
public String greet() {
return "Hello from /api/greet";
}
}
Example of @GetMapping / @PostMapping:
@RestController
public class UserController {
@GetMapping("/users/{id}")
public User getUser(@PathVariable int id) {
// Logic to get the user by ID
return userService.getUserById(id);
}
@PostMapping("/users")
public User createUser(@RequestBody User user) {
// Logic to create a new user
return userService.createUser(user);
}
}
Using these annotations makes code more readable and better aligned with RESTful best practices.
4. @Autowired
@Autowired
is one of the core annotations in Spring’s dependency injection mechanism. It automatically injects the required dependencies into the class. It can be applied to constructors, fields, or setter methods.
Example:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(int id) {
return userRepository.findById(id).orElse(null);
}
}
Here, the UserRepository
is automatically injected into the UserService
class by Spring, ensuring that the repository instance is available when needed without manual instantiation.
5. @Component, @Service, @Repository
These annotations are used to mark classes as Spring beans, which are managed by the Spring container. These annotations serve specific purposes within the application architecture.
- @Component: A general-purpose annotation to mark any class as a Spring-managed component.
- @Service: A specialization of
@Component
, specifically used to indicate that a class provides business logic. - @Repository: A specialization of
@Component
used for data access logic, and it also provides exception translation from database-specific exceptions to Spring’sDataAccessException
.
Example:
@Component
public class NotificationService {
public void sendNotification(String message) {
// Logic to send a notification
}
}
@Service
public class OrderService {
private final NotificationService notificationService;
@Autowired
public OrderService(NotificationService notificationService) {
this.notificationService = notificationService;
}
public void processOrder(Order order) {
// Business logic to process an order
notificationService.sendNotification("Order processed");
}
}
@Repository
public interface UserRepository extends JpaRepository<User, Integer> {
// Custom database queries can be defined here
}
These annotations make it clear what role each class plays in the application and provide better modularity.
6. @Entity
@Entity
is used in Spring Data JPA to map a Java class to a database table. It is part of the JPA specification and is used to create an entity that can be managed by an ORM (Object-Relational Mapping) framework.
Example:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String name;
private String email;
// Getters and Setters
}
In this example, the User
class is mapped to a database table, where each field corresponds to a column.
7. @Transactional
@Transactional
is used to indicate that a method (or class) should be executed within a transaction. Spring will automatically manage the transaction boundaries for you, ensuring that all database operations within the method are executed within a single transaction.
Example:
@Service
public class BankService {
@Autowired
private AccountRepository accountRepository;
@Transactional
public void transferMoney(int fromAccountId, int toAccountId, double amount) {
Account fromAccount = accountRepository.findById(fromAccountId).get();
Account toAccount = accountRepository.findById(toAccountId).get();
fromAccount.setBalance(fromAccount.getBalance() - amount);
toAccount.setBalance(toAccount.getBalance() + amount);
accountRepository.save(fromAccount);
accountRepository.save(toAccount);
}
}
In this example, all the operations related to the money transfer will be wrapped in a transaction. If any exception occurs, Spring will roll back the entire transaction to ensure data consistency.
Conclusion
Spring Boot annotations provide a powerful mechanism to configure and manage various components of an application. They greatly reduce the complexity of manual configurations, making development more streamlined and maintainable. Whether you are handling dependency injection, managing database entities, or building REST APIs, understanding these annotations is key to mastering Spring Boot development.
In your next project, make sure to leverage these annotations to improve your productivity and code quality!
Subscribe to my newsletter
Read articles from Uttam Mahata directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Uttam Mahata
Uttam Mahata
An undergraduate student pursuing a Bachelor's degree in Computer Science and Technology at the Indian Institute of Engineering Science and Technology, Shibpur, I have developed a deep interest in data science, machine learning, and web development. I am actively seeking internship opportunities to gain hands-on experience and apply my skills in a formal, professional setting. Programming Languages: C/C++, Java, Python Web Development: HTML, CSS, Angular, JavaScript, TypeScript, PrimeNG, Bootstrap Technical Skills: Data Structures, Algorithms, Object-Oriented Programming, Data Science, MySQL, SpringBoot Version Control : Git Technical Interests: Data Science, Machine Learning, Web Development