Android Learning Path with XML
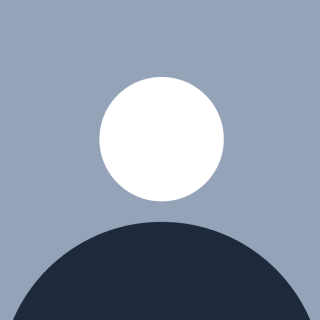
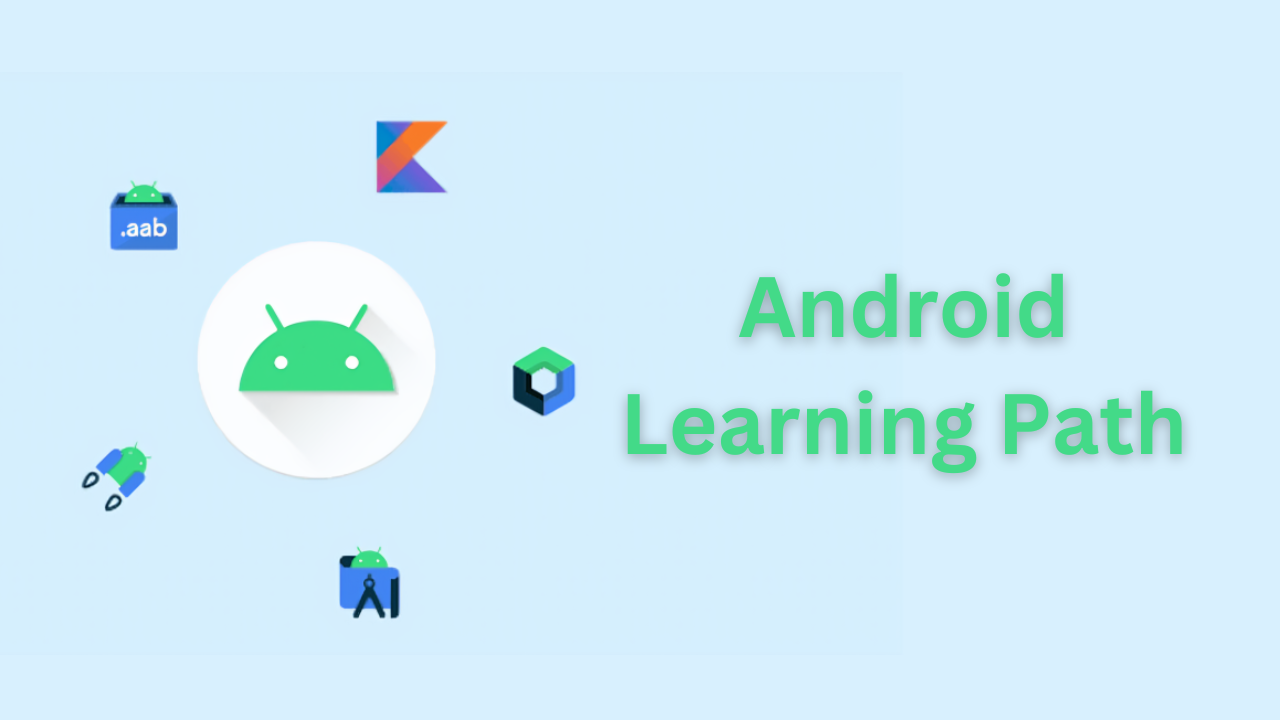
Basics
Android Layouts, Views, Chains, and Guidelines
Different types of layouts (LinearLayout, RelativeLayout, ConstraintLayout, etc.)
Use Views and ViewGroups
Chains and Guidelines in ConstraintLayout for complex UI designs
Activity and Data Passing
Activity lifecycle
Pass data between activities using Intents and Bundles
Fragment and Data Passing
Fragment lifecycle
Pass data between fragments and activities
Intent
Differentiate between explicit and implicit intents
Use intents to start activities and services
RecyclerView
RecyclerView component for displaying lists of data
ViewHolders, Adapters, and LayoutManagers
Notification
Create and manage notifications
Notification channels and importance levels
Alert Dialogs
Create and display alert dialogs
Different types of dialogs (e.g., AlertDialog, DatePickerDialog)
Spinner (Drop-down Menu)
Use Spinner for drop-down menus
Populate Spinner with data
Toolbar Menu
Add and manage items in the Toolbar menu
Handle menu item clicks
Swipeable Tab Bar
- Implement swipeable tabs using ViewPager and TabLayout
Bottom Navigation
- Implement bottom navigation using BottomNavigationView
Navigation Drawer
- Create a navigation drawer for app navigation
Drag and Drop
- Implement drag and drop functionality in your app
Runtime Permission
- Request and handle runtime permissions
Android MultiThreading
Thread, Runnables, and Callables
- Basics of threading and how to use Runnables and Callables
Handlers and Loopers
- Use Handlers and Loopers for message handling
Executors and ThreadPool
- Manage threads using Executors and ThreadPools
AsyncTask
- Use AsyncTask for background operations (Note: Deprecated in API level 30)
RxJava
- Use RxJava for reactive programming
WorkManager
- Use WorkManager for background tasks
AlarmManager
- Schedule tasks using AlarmManager
Coroutines
- Use Kotlin Coroutines for asynchronous programming
Services
Background Service / Started Service
- Create and manage background services
Foreground Service
- Create and manage foreground services
Bound Service
- Create and manage bound services
Android Interface Definition Language (AIDL)
- Use AIDL for inter-process communication
IntentService / JobIntentService
- Use IntentService and JobIntentService for background tasks
JobScheduler
- Use JobScheduler for scheduling background tasks
Broadcast Receivers
Normal Broadcast Receiver
Use sendBroadcast()
Implicit and explicit broadcasts
Dynamic and static registration
Ordered Broadcast Receiver
Use sendOrderedBroadcast()
Implicit and explicit broadcasts
Dynamic and static registration
Sticky Broadcast
- Note: Deprecated in API 21
Local Broadcast
Use LocalBroadcastManager
Implicit and explicit broadcasts
Dynamic registration
Content Provider
- Use Content Providers for data sharing between applications
Data Persistence
SharedPreferences
- Store and retrieve simple data using SharedPreferences
Room Database
Use Room for database management
Entities, DAOs, and database migrations
SQLite
- Basics of SQLite database management
DataStore
- Use DataStore for data persistence
Android Networking
Volley
- Use Volley for network operations
OkHttp
- Use OkHttp for HTTP requests
Retrofit
- Use Retrofit for network operations and API calls
Ktor
- Use Ktor for asynchronous network operations
Architecture Patterns
MVC (Model — View — Controller)
- Basics of the MVC architecture pattern
MVP (Model — View — Presenter)
- MVP architecture pattern
MVVM (Model — View — ViewModel)
- Implement the MVVM architecture pattern using ViewModel and LiveData
Seign Pattern
- Seign pattern and its use cases in software architecture
Dependency Injection
Basics of Dependency Injection
- Principles of Dependency Injection (DI) and its benefits
Dagger
- Use Dagger for dependency injection in Android
Hilt
- Use Hilt, a dependency injection library built on top of Dagger, for simpler DI in Android applications
Koin
- Use Koin, a lightweight dependency injection framework for Kotlin
Design Patterns
Singleton Pattern
- Implement the Singleton pattern to ensure a class has only one instance
Factory Pattern
- Factory pattern for creating objects without specifying the exact class
Observer Pattern
- Use the Observer pattern to allow objects to be notified of changes in other objects
Builder Pattern
- Builder pattern for constructing complex objects step by step
Adapter Pattern
- Use the Adapter pattern to allow incompatible interfaces to work together
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer