How to Publish Your First NPM Package: A Beginner's Tutorial

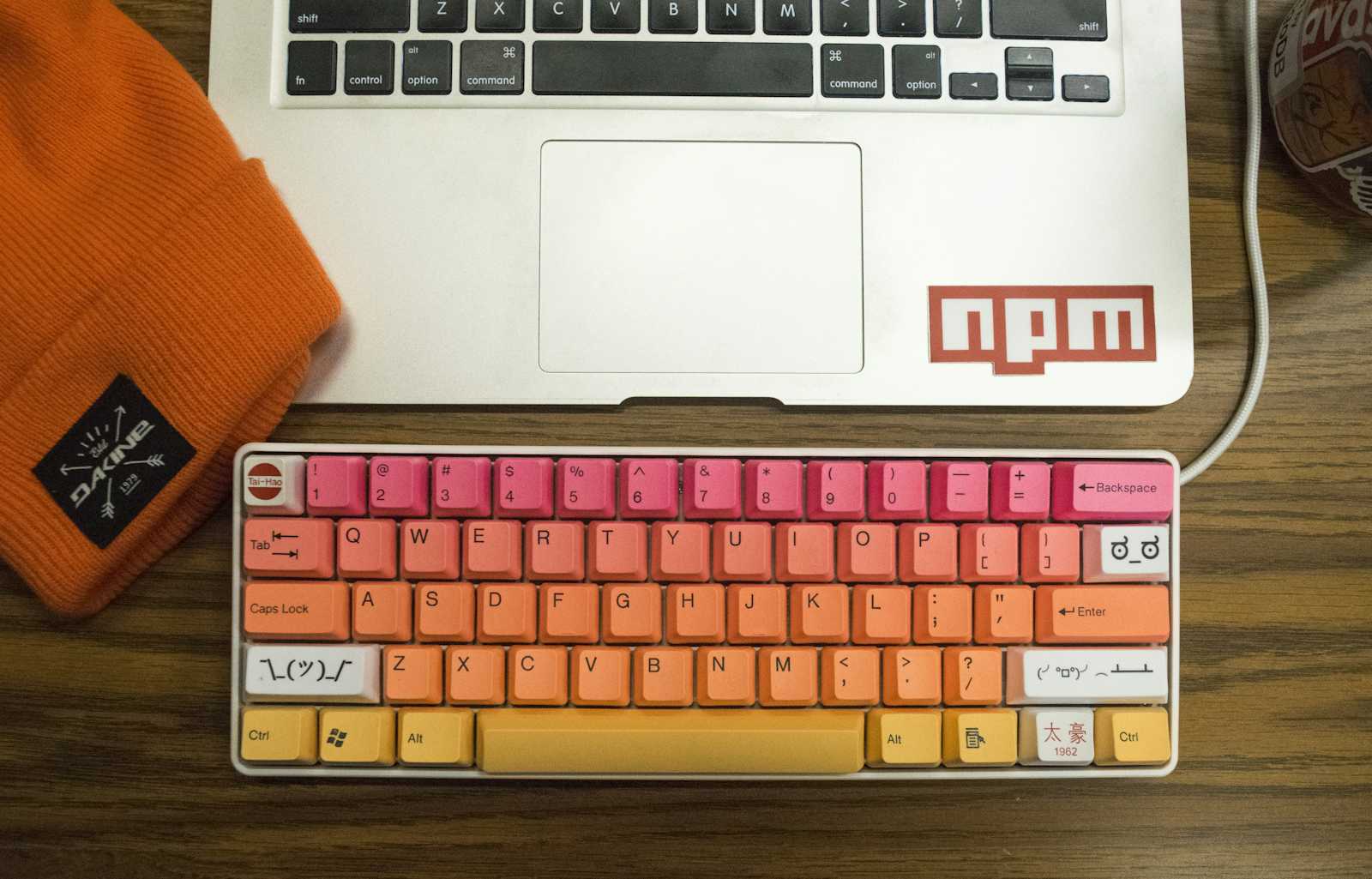
Prerequisites
Node.js (version 14 or higher) installed on your system
NPM (version 6 or higher) installed on your system
A GitHub account (optional but recommended)
A code editor or IDE of your choice
Creating a New NPM Package
Open your terminal or command prompt and navigate to the directory where you want to create your package.
Run the following command to create a new directory for your package:
mkdir my-package
Navigate into the newly created directory:
cd my-package
Initializing the Package
Run the following command to initialize a new NPM package:
npm init
Follow the prompts to fill in the required information:
package name
: The name of your package (e.g.,my-package
).version
: The initial version of your package (e.g.,1.0.0
).description
: A brief description of your package.entry point
: The main file of your package (e.g.,index.js
).test command
: The command to run your tests (e.g.,npm test
).git repository
: The URL of your GitHub repository (optional).keywords
: Keywords related to your package (e.g.,javascript
,utility
).author
: Your name and email address.license
: The license under which your package is released (e.g.,MIT
).
Writing the Package Code
Create a new file called
index.js
in the root of your package directory:touch index.js
Open the
index.js
file in your code editor and add the following code:function greet(name) { console.log(Hello, ${name}!); } module.exports = greet;
Save the file.
Testing the Package
Create a new file called
test.js
in the root of your package directory:touch test.js
Open the
test.js
file in your code editor and add the following code:const greet = require('./index'); describe('greet', () => { it('should print a greeting message', () => { const message = 'Hello, John!'; console.log = jest.fn(); greet('John'); expect(console.log).toHaveBeenCalledWith(message); }); });
Save the file.
Building the Package
Open your
package.json
file and add the following script:"scripts": { "build": "echo 'Building package...'" }
Run the following command to build your package:
npm run build
Publishing the Package
Create an NPM account if you haven't already.
Run the following command to login to your NPM account:
npm login
Follow the prompts to enter your NPM username, password, and email address.
Run the following command to publish your package:
npm publish
Follow the prompts to confirm that you want to publish your package.
Updating the Package
Make changes to your package code.
Update the version number in your
package.json
file.Run the following command to publish the updated package:
npm publish
Example Use Case
To use your newly published package in another project, run the following command:
npm install my-package
Then, in your JavaScript file, import and use the package:
const greet = require('my-package');
greet('John'); // Output: Hello, John!
That's it! You've successfully created and published an NPM package.
Subscribe to my newsletter
Read articles from Pratham Agrawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
